Why generics
- The collection container class cannot determine what type of objects actually exist in this container in the design phase / declaration phase, so before JDK1.5, the element type can only be designed as Object, and after JDK1.5, generics can be used to solve it. At this time, except the element type is uncertain, other parts are determined, such as how to save and manage the element. Therefore, the element type is designed as a parameter, which is called genericity. Collection, List and ArrayList are type parameters, i.e. generics
2. Since JDK1.5, Java has introduced the concept of "Parameterized type", which allows us to specify the type of collection elements when creating a collection, such as: List < string >, which indicates that the list can only save objects of string type.
3.JDK1.5 rewrites all interfaces and classes in the collection framework and adds generic support for these interfaces and classes, so that type arguments can be passed in when declaring collection variables and creating collection objects Why use generics? Direct Object can also store data 1. Solve the security problem of element storage, such as commodity and drug labels, without making mistakes
2. Solve the problem of type coercion when obtaining data elements, for example, you don't have to identify goods and drugs every time you get them
3.Java generics can ensure that ClassCastException will not be generated at runtime if the program does not issue a warning at compile time. At the same time, the code is more concise and robust
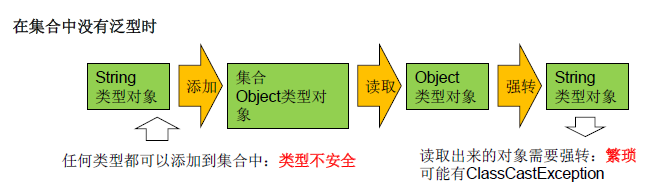
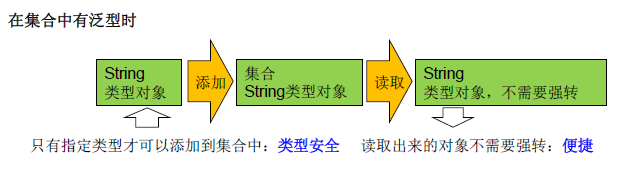
Use generics in Collections
ArrayList<Integer> list = new ArrayList<>();//Type inference list.add(78); list.add(88); list.add(77); list.add(66); //Traversal mode 1: //For (integer I: list) {/ / forced conversion is not required //System.out.println(i); //} //Traversal mode 2: Iterator<Integer> iterator = list.iterator(); while(iterator.hasNext()){ System.out.println(iterator.next()); } Map<String,Integer> map = new HashMap<String,Integer>(); map.put("Tom1",34); map.put("Tom2",44); map.put("Tom3",33); map.put("Tom4",32); //Add failed //map.put(33, "Tom"); Set<Entry<String,Integer>> entrySet = map.entrySet(); Iterator<Entry<String,Integer>> iterator = entrySet.iterator(); while(iterator.hasNext()){ Entry<String,Integer> entry = iterator.next(); System.out.println(entry.getKey() + "--->" + entry.getValue()); }
Custom generic structure
Custom generic class
1. A generic class may have multiple parameters. In this case, multiple parameters should be placed in angle brackets together. For example: < E1, E2, E3 >
2. The constructor of generic class is as follows: public GenericClass() {}.
The following is an error: public GenericClass() {}
3. After instantiation, the structure of the original generic location must be consistent with the specified generic type.
4. References with different generics cannot be assigned to each other.
5. Although ArrayList and ArrayList are two types at compile time, only one ArrayList is loaded into the JVM at run time
6. If a generic type is not specified, it will be erased. The types corresponding to the generic type are treated as Object, but they are not equivalent to Object. Experience: generic types should be used all the way. If not, they should not be used all the way.
7. If the generic structure is an interface or abstract class, the object of the generic class cannot be created.
8.jdk1.7, simplification of generics: ArrayList flist = new ArrayList < > ();
9. Basic data types cannot be used in the specification of generic types, but can be replaced by wrapper classes
10. The generic type declared on the class / interface represents a type in this class or interface. It can be used as the type of non-static attribute, parameter type of non-static method and return value type of non-static method. However, the generic type of class cannot be used in static method
11. Exception class cannot be generic
12. You cannot use new E [], but you can: E[] elements = (E[])new Object[capacity];
Reference: the ArrayList source code declares: Object[] elementData, not a generic parameter type array
13. The parent class has a generic type, and the child class can choose to keep the generic type or specify the generic type:
the subclass does not retain the generic type of the parent class: it is implemented on demand
no type erasure
specific type
subclasses retain the generics of the parent class: generic subclasses
all reserved
partial reservation
The subclass must be a "rich second generation". In addition to specifying or retaining the generics of the parent class, the subclass can also add its own generics
class Father<T1, T2> { } // Subclasses do not retain the generics of the parent class // 1) No type erase class Son1 extends Father {// Equivalent to class son extends father < object, Object > {} // 2) Specific type class Son2 extends Father<Integer, String> { } // Subclasses retain the generics of the parent class // 1) Keep all class Son3<T1, T2> extends Father<T1, T2> { } // 2) Partial retention class Son4<T2> extends Father<Integer, T2> { } class Father<T1, T2> { } // Subclasses do not retain the generics of the parent class // 1) No type erase class Son<A, B> extends Father{//Equivalent to class son extends father < object, Object > {} // 2) Specific type class Son2<A, B> extends Father<Integer, String> { } // Subclasses retain the generics of the parent class // 1) Keep all class Son3<T1, T2, A, B> extends Father<T1, T2> { } // 2) Partial retention class Son4<T2, A, B> extends Father<Integer, T2> { }
class Person<T> // Define variables using type T private T info; // Use T type to define the general method public T getInfo(){ return info; } public void setInfo(T info) { this.info = info; } // Use T type to define constructor public Person() { } public Person(T info) { this.info = info; }
Custom generic interface
Custom generic method
- Methods can also be generic, regardless of whether the class defined in them is generic or not. Generic parameters can be defined in generic methods. At this time, the type of parameters is the type of incoming data
- Format of generic methods
Exception thrown by [access permission] < generic > return type method name ([generic ID parameter name]) - The upper limit can also be specified when a generic method declares a generic type
public class DAO { public <E> E get(int id, E e) { E result = null; return result; } }
The embodiment of generics in inheritance
B is A subtype (subclass or sub interface) of A, while G is A class or interface with generic declaration, such as list interface. List < b > is not A subtype of list < A >
Use of wildcards
- Use type wildcard:?
For example: List <? >, map <?,? >
List <? > is the parent class of various generic lists such as list < string >, list < Object > - It is always safe to read the elements in the Object list of list <? >, because no matter what the real type of list is, it contains objects
- We can't write the elements in the list. Because we don't know the element type of c, we can't add objects to it
the only exception is null, which is a member of all types
Adding any element to it is not type safe
Collection<?> c = new ArrayList<String>();
c. add(new Object()); / / compile time error
Because we don't know the element type of c, we can't add objects to it. The add method has the type parameter E as the element type of the collection. Any parameter we pass to add must be a subclass of an unknown type. Because we don't know what type it is, we can't pass anything in
The only exception is null, which is a member of all types
On the other hand, we can call the get() method and use its return value. The return value is an unknown type, but we know that it is always an Object
//Note 1: compilation error: it cannot be used on generic method declaration, and < > in front of return value type cannot be used? public static <?> void test(ArrayList<?> list){ } //Note 2: compilation error: cannot be used on the declaration of generic classes class GenericTypeClass<?>{ } //Note 3: compilation error: it cannot be used for creating objects. The right side belongs to creating collection objects ArrayList<?> list2 = new ArrayList<?>();
<?>
Allow all generic reference calls
wildcard specifies the upper limit
Upper bound extensions: when used, the specified type must inherit a class or implement an interface, i.e<=
wildcard specifies the lower limit
Lower limit super: the type specified when using cannot be less than the class of the operation, that is >=
examples
<? Extensions Number > infinitesimal, Number]
Only reference calls with generic Number and Number subclasses are allowed
<? Super number > [number, infinity)
Only reference calls with generic Number and Number parent are allowed
<? extends Comparable>
Only generic calls are allowed for references to implementation classes that implement the Comparable interface
Generic application examples
Interview questions
1. What compile operations will report errors?
public class Test { public static void addstrings(List<? extends Object> list) { list.add(new A()); list.add(new Circle()); list.add(new GeometricObject()); list.add(new Object()); } public void addString(List<? super A> list) { list.add(new A()); list.add(new Circle()); list.add(new GeometricObject()); list.add(new Object()); } }