Overview of packaging
Design idea of packaging
- Hide the internal complexity of the object, and only disclose simple interfaces to facilitate external calls, so as to improve the scalability and maintainability of the system
- High cohesion: the internal data operation details of the class are completed by themselves, and external interference is not allowed
- Low coupling: only a small number of methods are exposed for use
Implementation of encapsulation
public class Student {
private String name;
private int age;
public void setName(String n) {
name = n;
}
public String getName() {
return name;
}
public void setAge(int a) {
age = a;
}
public int getAge() {
return age;
}
}
Permission modifier
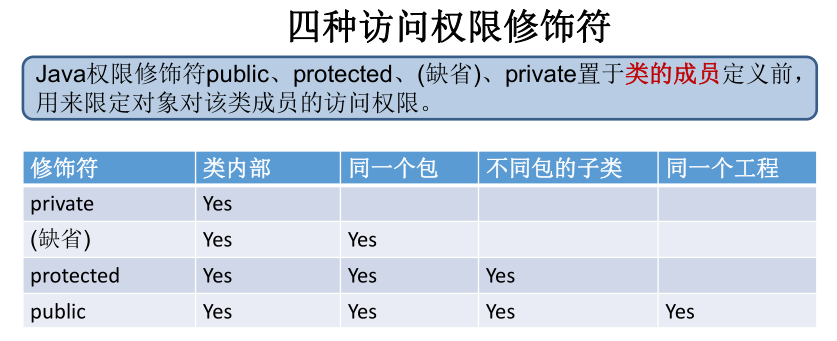
Constructor (Construct0r)
Function of constructor
- Initialize the instance object and assign the initial value to the member variable of the object
Example
public class Student {
private String name;
private int age;
// Nonparametric structure
public Student() {}
// Parametric structure
public Student(String n,int a) {
name = n;
age = a;
}
//Other codes are omitted here
}
be careful
- Java will automatically provide a parameterless constructor. Once the constructor is defined, the default parameterless constructor provided by Java will become invalid
- The constructor name must be the same as the class name in which it resides
- It has no return value, so void is not required
- Constructors cannot be modified by static, final, synchronized, Abstractct, or native
this keyword
The meaning of this
- This is used in the constructor to represent the example object being created, that is, who is new, this represents who
- This is used in the instance method to represent the object calling the method, that is, this represents who is calling
Standard JavaBean s
public class Student {
// Member variable
private String name;
private int age;
// Construction method
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
// get/set member method
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setAge(int age) {
this.age = age;
}
public int getAge() {
return age;
}
//List of other member methods
public String getInfo(){
return "full name:" + name + ",Age:" + age;
}
}
package
Function of package
- Avoid duplicate class names
- The classification organization manages a large number of classes
Common packages of JDK
- java.lang ---- contains some core classes of Java language, such as String, Math, Integer, System and Thread, and provides common functions
- java.io - contains classes that can provide multiple input / output functions
- java.util - contains some utility classes, such as collection framework class, date and time, array tool class, Arrays, text Scanner, Scanner, Random value generation tool, Random
- java.sql and javax SQL ---- contains the relevant classes / interfaces for JDBC database programming with Java
- java.net ---- contains classes and interfaces that perform network related operations
Declare the syntax format of the package
package Package name;
be careful
- Must be on the first line of source code
- A source file can only have one statement declaring a package
- Each word is lowercase and used between words separate
- Accustomed to using company domain name inversion
Classes that use other packages
Use the full name of the type
- java.util.Scanner input = new java.util.Scanner(System.in);
Import using import
import package.Class name;
import package.*;
import static package.Class name.Static member;
be careful
- Using Java Classes under Lang package can be used directly without import statement
- The import statement must be under package and above class
- When using classes with the same name in two different packages, for example: Java util. Date and Java sql. Date. One uses a full name and one uses a simple name
Example
package com.atguigu.test;
import java.util.Scanner;
import java.util.Date;
import com.atguigu.bean.Student;
public class Test{
public static void main(String[] args){
Scanner input = new Scanner(System.in);
Student stu = new Student();
String str = "hello";
Date now = new Date();
java.sql.Date d = new java.sql.Date(346724566);
}
}