How to define a structure type?
struct Student { int num; char name[2]; int age; }**;**
The first letter is capitalized, and the structure name must reflect the function of the structure (self annotation)
Function cannot be saved in structure:
Solution: save function pointer example:
struct Student { int num; char name[2]; int age; void (*func)(); }**;** void print() { peintf("hello world\n"); struct Student stu = {1,"zhangsan",12,**.func = print**};
Structure type definition variables:
struct Student stu;
initialization:
Pay attention to the problem of wild pointers, and directly assign values to array names (pointer constants)
struct Student stu = {1,"zhangsan",12}; struct Student stu2 ={ .num = 1, .name = "lisi", .age = 15 };
How to access members of structure variables?. - >
printf("stu.num = %d\n",stu2.num); printf("stu.name = %s\n",stu.name); struct Student *pstu = &stu;//*pstu = stu; printf("stu.num = %d\n",**(*pstu)**.num); printf("stu.num = %d\n",pstu->num);//(*pstu).num printf("stu.num = %d\n",(&stu)->num); struct Student stu3; scanf("%d",&(stu.num)); scanf("%d",stu.name); scanf("%d",&(stu.age));
Wild pointer processing:
struct Student
{
int num;
char *name;// char name[20];
int age;
void (*func)();
};
struct Student stu3;
scanf("%d",&(stu.num));
*stu.name = (char )malloc(sizeof(char) * 100);
if(NULL == stu.name)
{
printf("malloc error!\n");
exit(1);
}
scanf("%d",stu.name);
free(stu.name);
stu.name = NULL;
Assignment between structures: shallow copy
#include <stdio.h> #include <stdlib.h> #include <string.h> struct Student { int num; char name[20]; //char *name; int age; void (*func)(); }; void print() { printf("hello world!\n"); } int main(int argc, char **argv) { struct Student stu = {1, "zhangsan", 12, .func = print}; struct Student stu2 = { .num = 1, .name = "lisi", .age = 15, .func = print}; struct Student *pstu = &stu; //*pstr = stu; printf("stu.num = %d\n", stu2.num); printf("stu.name = %s\n", stu.name); printf("stu.num = %d\n", (*pstu).num); printf("stu.name = %s\n", (*pstu).name); printf("stu.num = %d\n", pstu->num); //(*pstu).num; printf("stu.num = %d\n", (&stu)->num); struct Student stu3; printf("input num:\n"); scanf("%d", &(stu3.num)); strcpy(stu3.name, "zhangsan"); printf("input age:\n"); scanf("%d", &(stu3.age)); printf("stu3.num = %d stu3.name = %s stu3.age = %d\n", stu3.num, stu3.name, stu3.age); struct Student stu4; stu4 = stu3; return 0; }
Deep copy: encapsulating functions
Precautions for use of structure:
Word alignment leads to empty memory and puts the same types together
Exceptions:
Members with a length greater than or equal to 4 bytes are aligned according to the maximum byte
Structure function: encapsulate data (package data) - abstract data
Structure array:
Flexible array: (implementation of variable length array in C language)
#include <stdio.h> struct node { int len; char src[0]; }; typedef struct node Array; int main(int argc,char **argv) { printf("sizeof(node) = %ld\n",sizeof(struct node)); Array a; a.len = 100; a.src = (char *)malloc (sizeof(char)* 100); a.src = (char *)realloc (sizeof(char)* Original byte length); return 0; }
union community:
union uses: defining types, defining variables and pointers, and initializing
union Student { int num; char ch; }; int main() { union Student stu = {.num = 1,.ch = 'a'}; union Student *pstu = &stu; printf("sizeof(stu) = %ld\n",sizeof(stu)); printf("stu.num = %d\n",stu.num);//???? printf("stu.ch = %c\n",spstu->num); return 0; }
struct VS union size:
union size:
The maximum byte length of the saved member is determined by the member; (maintain byte alignment like struct)
Storage method: all members share the same memory space (variable with the longest bytes) (data overwrite)
cpu properties:
Big endian byte order: the high byte is written at the low address
Small end byte order: the low byte is written at the low address
! [insert picture description here]( https://img-blog.csdnimg.cn/dcfb8c3f0a0d47e9bba81248f3607710.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5ZWl5Lmf5a2m5LiN5Lya55qE6I-c6bih,size_20,color_FFFFFF,t_70,g_se,x_16
Interview questions:
Using the common body to judge the byte order of the size end of the cpu?
The size end can be verified without union:
int num = 0x12345678; char *ptr = # printf("%x\n",*ptr);
Function of union:
#include <stdio.h> union node { int len; void *ptr; char sex; }; void func(union node p,int flag) { switch(flag) { case 0: { printf("len = %d\n",p.len); break; } case 1: { printf("%d\n",*((int *)p.ptr)); break; } case 2: { printf("%c\n",p.sex)); break; } int main(int argc,char **argv) { union node p; p.len = 11; func(p,0); p.ptr = (void*)# func(p,1); p.sex='M'; func(p,2); return 0; } ```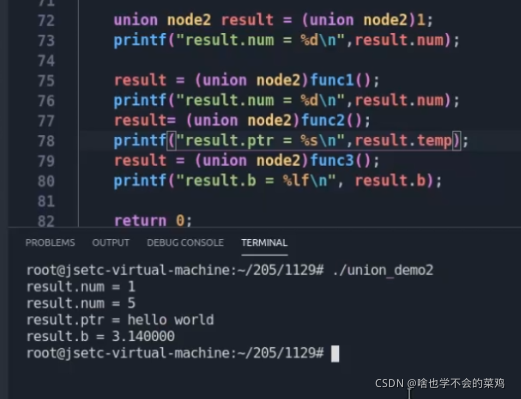 enum Enumeration: defines a series of integer macros 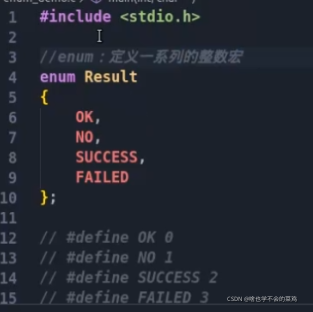 An enumerated variable is equivalent to an integer variable(4 byte) enum VS define enum Advantages: it is more convenient to define a series of integer macros; in the compilation stage define: Pretreatment, unsafe, fool replacement File operation: [https://www.cnblogs.com/saolv/p/7793379.html](https://www.cnblogs.com/saolv/p/7793379.html) FILE:field name pointer **fopen: **Open a file ```c #include <stdio.h> #include <stdlib.h> int main(int argc, char **argv) { FILE *fp; fp = fopen("/root/205/1129/a.txt","w+"); } if (fp == NULL) { printf("file a.txt error!\n"); exit(1); }
If the file does not exist, "w" "w +" "a" "a +" is created
**fclose: * * close a file
fclose(fp);
fwrite:
#include <stdio.h> #include <stdlib.h> #include <string.h> int main(int argc, char **argv) { FILE *fp; fp = fopen("/root/205/1129/a.txt","w+"); } if (fp == NULL) { printf("file a.txt error!\n"); exit(1); } char buffer [1024] = "hello world"; int ret = fwrite(buffer, 1, strlen(buffer), fp); printf("ret = %d\n", ret); fclose(fp); return 0; }
fread:
#include <stdio.h> #include <stdlib.h> #include <string.h> int main(int argc, char **argv) { FILE *fp; fp = fopen("/root/205/1129/a.txt","w+"); } if (fp == NULL) { printf("file a.txt error!\n"); exit(1); } char buffer [1024] = "hello world"; int ret = fwrite(buffer, 1, strlen(buffer), fp); printf("ret = %d\n", ret); memset(buffer, 0, sizeof(buffer)); ret = fread(buffer,ret,1,fp); buffer[ret] = '\0'; printf("read buffer = %s\n",buffer); fclose(fp); return 0; }
The result read is null
Solution: fseek
#include <stdio.h> #include <stdlib.h> #include <string.h> int My_fread(void *ptr, size_t size, size_t nmemb, FILE *fp) { int ret = fread(ptr,ret,1,fp); if (ret == 0) { printf("%d:fread a.txt error!\n",__LINE__); exit(1); } return ret; } int main(int argc, char **argv) { FILE *fp; fp = fopen("/root/205/1129/a.txt","a+"); } if (fp == NULL) { printf("file a.txt error!\n"); exit(1); } char buffer [1024] = "hello world"; int ret = fwrite(buffer, 1, strlen(buffer), fp); fwrite("\n",1,1,fp); if (ret == 0) { printf("fwrite a.txt error!\n"); exit(1); } printf("ret = %d\n", ret); memset(buffer, 0, sizeof(buffer)); fseek(fp, 0, SEEK_SET); //ret = fseek(fp, -ret, SEEK_CUR); if (ret == -1) { printf("fseek a.txt error!\n"); exit(1); } ret = My_fread(buffer, 1, 11, fp); buffer[ret] = '\0'; printf("read buffer = %s\n",buffer); fclose(fp); return 0; }
**feof() * * is a function to detect the file terminator on the stream. If the file ends, it returns a non-0 value, otherwise it returns 0
**EOF: * * there is a hidden character "EOF" at the end of the document. When the program reads it, it will know that the file has reached the end
**long ftell(FILE *stream); * * used to get the offset of the file pointer from the beginning of the file
#include <stdio.h> #include <stdlib.h> #include <string.h> int My_fread(void *ptr, size_t size, size_t nmemb, FILE *fp) { int ret = fread(ptr, 1, 11, fp); if (ret == 0) { printf("%d:fread a.txt error!\n", __LINE__); exit(1); } return ret; } int My_read_line(char *ptr, size_t size, size_t memb, FILE *fp) { int i = 0; char temp; while (!feof(fp)) { if (fread(&temp, 1, 1, fp) == 0) { printf("file fread error!\n"); } if (temp == '\n') { break; } ptr[i] = temp; i++; } ptr[i] = '\0'; return i; } int main(int argc, char **argv) { FILE *fp; //If the file does not exist, "w" "w+" "a" "a +" fp = fopen(argv[1], "a+"); if (fp == NULL) { printf("file a.txt error!\n"); exit(1); } #if 0 //fread \ fwrite char buffer[1024] = "hello world"; int ret = fwrite(buffer, 1, strlen(buffer), fp); fwrite("\n", 1, 1, fp); if (ret == 0) { printf("fwrite a.txt error!\n"); exit(1); } printf("ret = %d\n", ret); memset(buffer, 0, sizeof(buffer)); fseek(fp, 0, SEEK_SET); //ret = fseek(fp, -ret, SEEK_CUR); if (ret == -1) { printf("fseek a.txt error!\n"); exit(1); } ret = My_fread(buffer, 1, 11, fp); buffer[ret] = '\0'; printf("read buffer = %s\n", buffer); #endif char buffer[1024]; int ret; fseek(fp, 0, SEEK_SET); //Error: cannot read more than one character at a time. It may encounter a terminator, and fread will return 0 // if(fread(buffer, sizeof(buffer) - 1, 1, fp) == 0) // { // printf("fread file error!\n"); // exit(1); // } //Solution 1: // int i = 0; // char temp; // while(!feof(fp)) // { // if(fread(&temp, 1, 1, fp) == 0) // { // printf("file fread error!\n"); // } // buffer[i] = temp; // i++; // } // buffer[i] = '\0'; //Solution 2: // fseek(fp, 0, SEEK_END); // int file_size = ftell(fp); // fseek(fp, 0, SEEK_SET); // fread(buffer, file_size, 1, fp); while (!feof(fp)) { My_read_line(buffer, 1, sizeof(buffer) - 1, fp); printf("buffer = %s\n", buffer); } fclose(fp); return 0; }
3.36 points