Handwriting adapter mode
Author: Gorit
Refer: graphic design mode
Blog post published in 2021: 2 / 50
Links to the original text - and integration of other series of articles source code
2, Adapter mode (add an adapter for reuse)
2.1 popular explanation of adapter mode
Explanation of adapter mode: in the program world, there are often situations where existing programs cannot be used directly, and appropriate transformation is required before they can be used. This design pattern used to fill the gap between "existing program" and "required program" is called adapter pattern My understanding: * * adapter mode can be compared to the relationship between plug and * * socket * *
2.2 types of adapter modes
- Class adapter pattern (using inherited adapters)
- Object adaptation mode (using delegated adapters)
2.3 implementation of adapter mode
2.3.1 using inherited adapters
We use the following example to enter a hello string and print out "hello" or (Hello). Represents a strong string and a weak string, respectively How to use the adapter pattern?
- Define a Banner class, including showWithParen() enclosed in string brackets and showWithAster() enclosed in string *. This represents our actual situation
- Define a Print interface, which includes the (bracketed) printbreak() and (marked with * sign) printStrong() methods respectively. We assume that this interface is similar to the requirements
- An adapter is required to implement the Print interface requirements through the Banner class. The PrintBanner class plays the role of this adapter
physical truth | Implement two kinds of Banner printing | Banner (showWithParen,showWithAster) |
---|---|---|
Requirements (Interface) | Print interface | Print interface (printbreak, printStrong) |
Adapter | printBanner | PrintBanner class |
Concrete implementation Banner class
/** * Banner class */ public class Banner { private String string; public Banner(String string) { this.string = string; } // brackets public void showWithParen() { System.out.println("(" + string + ")"); } // * public void showWithAster() { System.out.println("**" + string + "**"); } }
Print interface
// Requirement interface public interface Print { public abstract void printWeak(); public abstract void printStrong(); }
The subclass of PrintBanner class (core) inherits the parent class and obtains the method of the parent class
public class PrintBanner extends Banner implements Print{ public PrintBanner(String string) { super(string); } // brackets @Override public void printWeak() { showWithParen(); } // * @Override public void printStrong() { showWithAster(); } }
Main test class
public class Main { public static void main(String[] args) { Print p = new PrintBanner("Hello"); p.printStrong(); p.printWeak(); } }
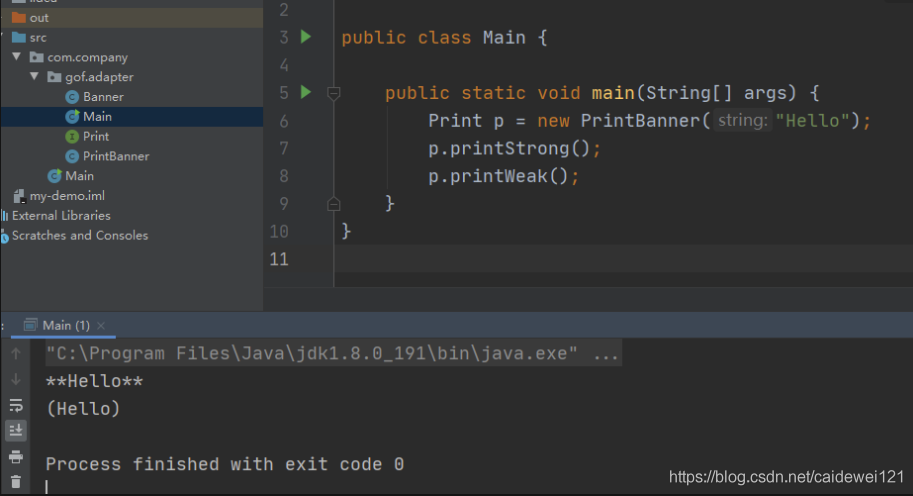
Here, we use Print to save the printBanner instance, hide the Banner class and the showWithxxx() method, and Main doesn't know how printBanner is implemented, so we don't need to modify the Main class
2.3.2 using delegated adapters
Delegate: a method that delegates the actual processing in a method to another instance Through a delegation relationship: when printbreak() of PrintBanner class is called, it is not handled by PrintBanner class itself, but handed over to showWithParen() of other instances (instances of Banner class) Print class
public abstract class Print { public abstract void printWeak(); public abstract void printStrong(); }
PrintBanner class (printbreak is handed over to Banner for processing)
public class PrintBanner extends Print { private Banner banner; public PrintBanner(String string) { this.banner = new Banner(string); } @Override public void printWeak() { banner.showWithParen(); } @Override public void printStrong() { banner.showWithAster(); } }
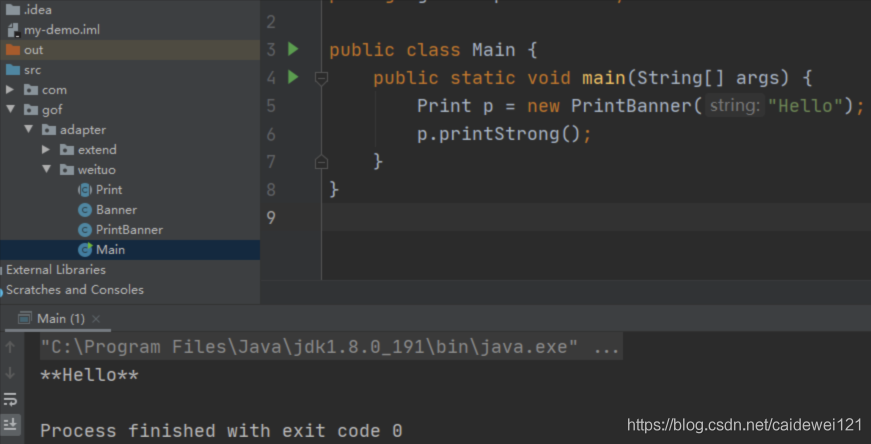
2.4 why use adapter mode
Using the Adapter pattern, you can adapt the existing code to the new interface API without modifying the existing code at all. In the Adapter pattern, you do not necessarily need ready-made code. You only need to know the function of the class to write a new class
2.5 adapter mode summary
- Use inheritance and delegate to implement the adapter pattern
- The Adapter is used to fill the gap between two classes with different interfaces (API s)