The UI diagram of the scenario is as follows:
Main Start Menu Scenario:
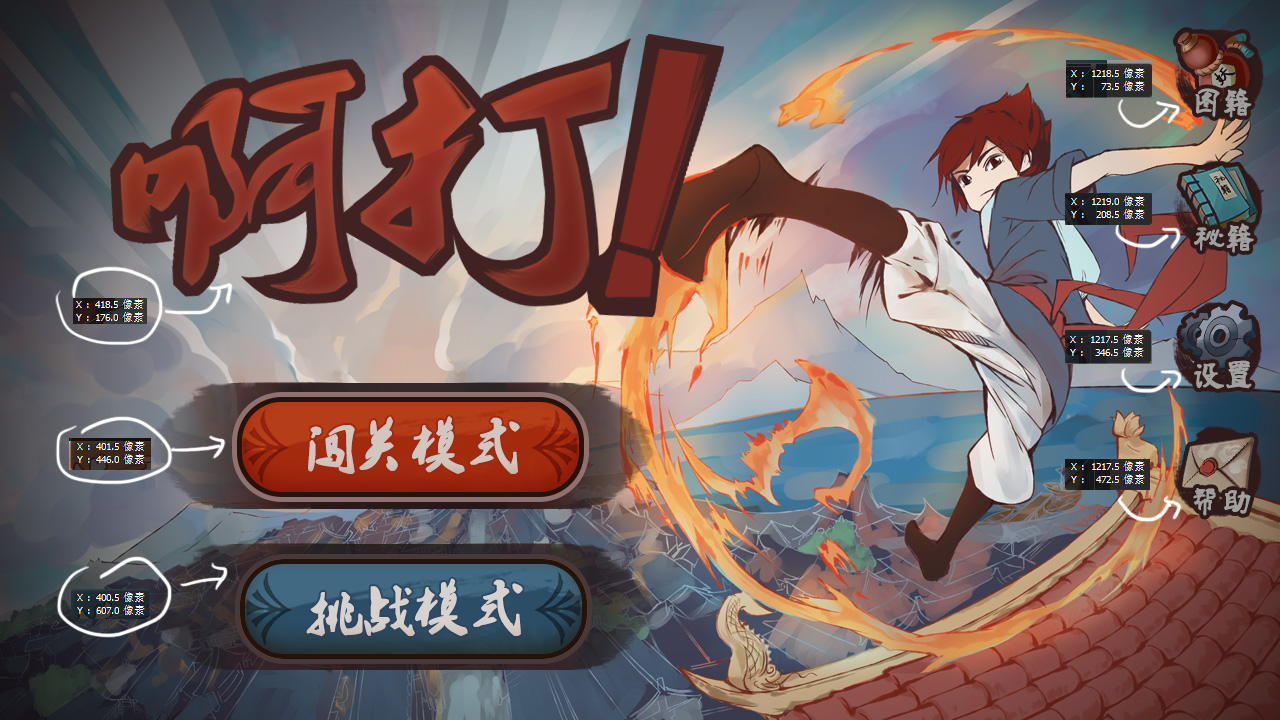
Secret Book Scene:
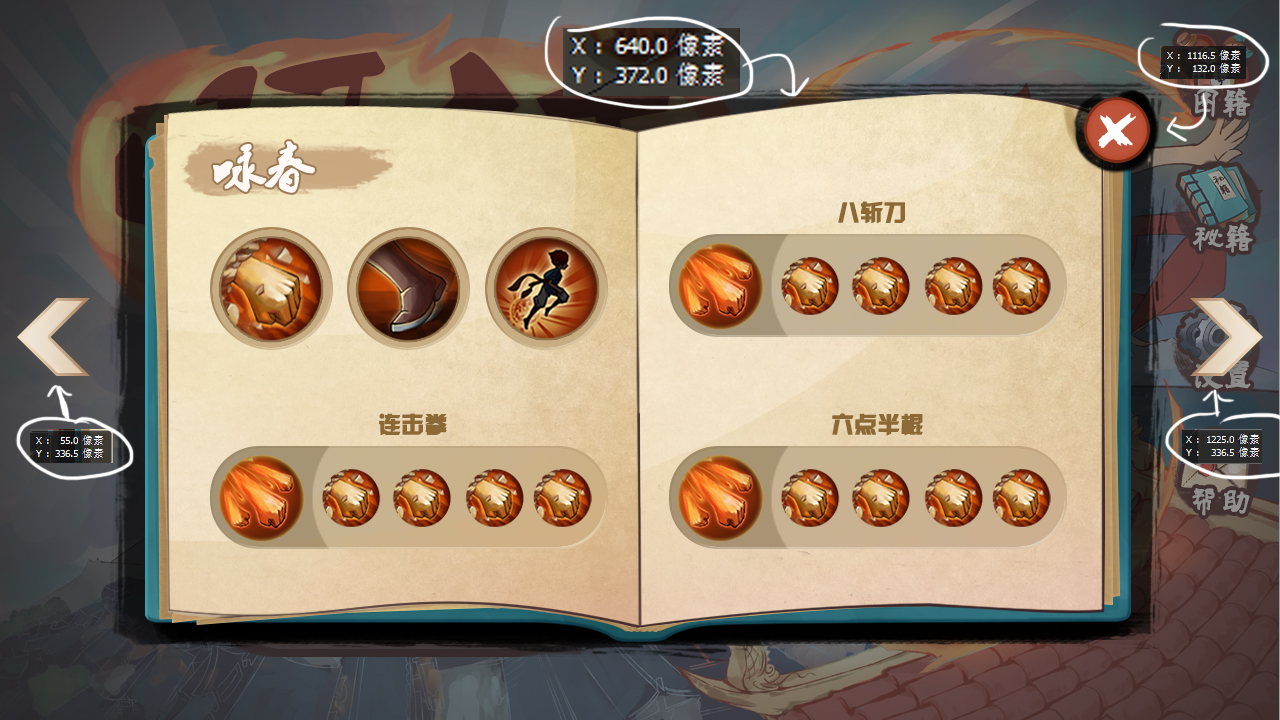
The main contents of this part are also three points:
Menu family and its membership
The characteristics of Menu and its members
Analysis and Implementation of Main Start Menu Scenarios
Below is the menu Menu and menu item MenuItem class diagram:
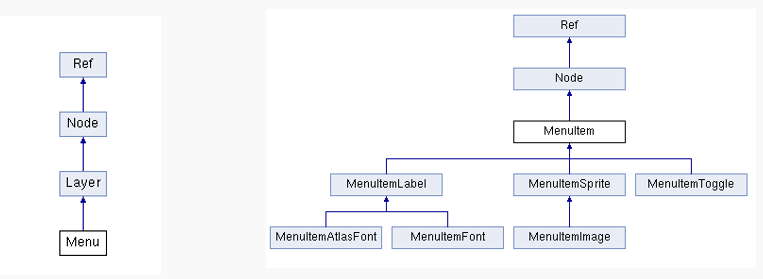
Their relationship is just like the name, one is the container Menu, the other is the content Item.
Here is a quotation:
Menu Menu is a Layer layer used to carry menu buttons. The child nodes in the layer can only be menu items MenuItem or its subclasses. Menu Item is usually created first, then menu Menu is generated with one or more menu items, and finally Menu is added to the current Layer layer. If you add MenuItem directly to the layer, it can also display normally, but it can not respond to the callback function, because Menu inherits to Layer, which inherits the related events of touch, while MenuItem only inherits from Node and does not respond to touch, so it can not call the callback function. Because CCMenu's parent class is Layer, the anchor is (0,0) and the anchor cannot be set. Menu's default origin coordinate is the positive center of the screen (winSize.width/2, winSize.height/2). MenuItem is added to Menu layer, so the position of MenuItem is offset from Menu layer. The offset of MenuItem relative to Menu is (0,0) by default, and the anchor of menu items is (0.5,0.5) by default. It is worth noting that Menu contains many sub-menu items. Each sub-menu item has a different location. If the location of Menu is defined, it will affect the location of all sub-menu items as the parent node. So we usually set Menu's position in Point Zero, and then set MenuItem's position (i.e., offset from the parent node) to locate the entire menu.
About menu items:
MenuItem inherits from Node, so its sub-class menu items can use the relevant operations of Node. MenuItem is the parent of all menu items. It is not recommended to use this class directly because it does not contain specific display functions. As the parent of other menu items, it provides three main functions: (1) Provides the status of the basic buttons: normal, selected, disabled. (2) Implementing the basic callback function mechanism for buttons. When the player clicks the product button, the corresponding callback function will be called to execute. (3) Touch menu items with automatic enlargement effect. Subcategories of menu items can be divided into three categories, a total of six: (1) Text menu items: Menu Item Label, Menu Item Atlas Font, Menu Item Font; (2) Picture menu items: MenuItemSprite, MenuItemImage; (3) Switch menu item: Menu Item Toggle.
And about the difference before each menu item here because the content is too much and no longer repeat, you can go to see his source code and official documents to obtain relevant knowledge, source code is the best learning material.
StartLayer.h
#ifndef __StartLayer_H__ #define __StartLayer_H__ #include "cocos2d.h" #include "cocos-ext.h" #include "SimpleAudioEngine.h" USING_NS_CC; USING_NS_CC_EXT; class StartLayer : public Layer { public: virtual bool init(); static Scene* createScene(); CREATE_FUNC(StartLayer); private: //Start the button control of the main interface to monitor events void touchSet(Ref* pSender); void touchLib(Ref* pSender); void touchMiJi(Ref* pSender); void touchCG(Ref* pSender); void touchTZ(Ref* pSender); void touchClose(Ref* pSender); void touchHelp(Ref* pSender); //photogene Sprite* title; Sprite* bgPic; }; #endif
StartLayer.cpp
#include "cocos2d.h" #include "SimpleAudioEngine.h" #include "GlobalDefine.h" #include "StartLayer.h" #include "HelloWorldScene.h" using namespace cocos2d; using namespace CocosDenshion; Scene* StartLayer::createScene() { Scene* startScene = Scene::create(); StartLayer* layer = StartLayer::create(); startScene->addChild(layer); return startScene; } bool StartLayer::init() { if(!Layer::init()) { return false; } SpriteFrameCache::getInstance()->addSpriteFramesWithFile("pnglist/galleryLayer.plist"); SpriteFrameCache::getInstance()->addSpriteFramesWithFile("pnglist/monster.plist"); SpriteFrameCache::getInstance()->addSpriteFramesWithFile("pnglist/resultLayer.plist"); SpriteFrameCache::getInstance()->addSpriteFramesWithFile("pnglist/mapBg.plist"); SpriteFrameCache::getInstance()->addSpriteFramesWithFile("pnglist/mapMid.plist"); if(getBoolFromXML(MUSIC_KEY)) { float music = getFloatFromXML(MUSICVOL)*100.0f; aduioEngine->setBackgroundMusicVolume(getFloatFromXML(MUSICVOL)); if(SimpleAudioEngine::getInstance()->isBackgroundMusicPlaying()) { aduioEngine->pauseBackgroundMusic(); aduioEngine->playBackgroundMusic("Sound/startBGM.mp3",true); } else aduioEngine->playBackgroundMusic("Sound/startBGM.mp3",true); } else aduioEngine->pauseBackgroundMusic(); // Elf Initialization and Location Settings title = Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("Title.png")); title->setPosition(WINSIZE.width / 2 - 222, WINSIZE.height / 2 + 186); bgPic = Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("MainMenuBackground.png")); bgPic->setPosition(WINSIZE.width / 2, WINSIZE.height / 2); this->addChild(bgPic); this->addChild(title); // Button initialization and time binding auto helpItem = MenuItemSprite::create( Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("HelpNormal.png")), Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("HelpSelected.png")), CC_CALLBACK_1(StartLayer::touchHelp, this)); // Help auto tujiItem = MenuItemSprite::create( Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("PhotoGalleryNormal.png")), Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("PhotoGallerySelected.png")), CC_CALLBACK_1(StartLayer::touchLib, this)); // Atlas auto setItem = MenuItemSprite::create( Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("SetNormal.png")), Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("SetSelected.png")), CC_CALLBACK_1(StartLayer::touchSet, this)); // Set up auto mijiItem = MenuItemSprite::create( Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("CheatsNormal.png")), Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("CheatsSelected.png")), CC_CALLBACK_1(StartLayer::touchMiJi, this)); // Secret book auto chuangguanItem = MenuItemSprite::create( Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("EmigratedNormal.png")), Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("EmigratedSelected.png")), CC_CALLBACK_1(StartLayer::touchCG, this)); // Rush through customs auto tiaozhanItem = MenuItemSprite::create( Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("ChallengeNormal.png")), Sprite::createWithSpriteFrame(SpriteFrameCache::getInstance()->getSpriteFrameByName("ChallengeSelected.png")), CC_CALLBACK_1(StartLayer::touchTZ, this)); // Challenge tujiItem->setPosition(WINSIZE.width - 62, WINSIZE.height - 73); mijiItem->setPosition(WINSIZE.width - 62, WINSIZE.height - 209); setItem->setPosition(WINSIZE.width - 62, WINSIZE.height - 346); helpItem->setPosition(WINSIZE.width - 62, WINSIZE.height - 473); chuangguanItem->setPosition(WINSIZE.width / 2 - 240, WINSIZE.height / 2 - 86); tiaozhanItem->setPosition(WINSIZE.width / 2 - 240, WINSIZE.height / 2 - 250); auto menu = Menu::create(tujiItem,mijiItem, setItem, helpItem, chuangguanItem, tiaozhanItem, NULL); menu->setPosition(Point::ZERO); this->addChild(menu, 2); return true; } // Button event implementation void StartLayer::touchSet(Ref* pSender) { PLAYEFFECT; // Director::getInstance()->replaceScene(SetLayer::createScene()); } void StartLayer::touchLib(Ref* pSender) { PLAYEFFECT; // Director::getInstance()->replaceScene(TujiLayer::createScene()); } void StartLayer::touchMiJi(Ref* pSender) { PLAYEFFECT; //Director::getInstance()->replaceScene(MijiLayer::createScene()); } void StartLayer::touchCG(Ref* pSender) { if (getBoolFromXML(SOUND_KEY)) { aduioEngine->setEffectsVolume(getFloatFromXML(SOUNDVOL)); aduioEngine->playEffect("Sound/button.mp3"); } Director::getInstance()->replaceScene(HelloWorld::createScene()); } void StartLayer::touchTZ(Ref* pSender) { PLAYEFFECT; // Director::getInstance()->replaceScene(GateMapLayer::createScene()); } void StartLayer::touchHelp(Ref* pSender) { PLAYEFFECT; // Director::getInstance()->replaceScene(HelpLayer::createScene()); }
* See watermarking for image source