Preface
Text and pictures of the text come from the network for learning and communication purposes only. They do not have any commercial use. Copyright is owned by the original author. If you have any questions, please contact us in time for processing.
Author: Star Ango, AirPython
Target Scene
I believe that when you brush short video with shaking sound, you will always have the habit of approving and paying attention to the ladies with high facial values.
If brushing one brush at a time really takes a lot of time, it would save a lot of work if Python could help filter out high-looking ladies.
This article is based on the "Baidu Face Recognition" API, to help us identify the high-facial jitter lady sister, and then download to the mobile phone album.
Dead work
First, the project requires some precise manipulation of the page elements. You need to prepare an Android device in advance, activate the developer options, and turn on the two settings "USB debugging and pointer location" in the developer options.
In order to ensure that the adb command can work properly, you need to configure the adb development environment in advance.
Some elements in a page element cannot be retrieved using common attributes such as name. You may need to retrieve a complete UI tree and use Airtest to determine if a UI element exists.
# Installation Dependency pip3 install pocoui
In addition, the project will conduct face recognition on the video, get all the faces that appear, and then perform gender recognition and face value judgment.
This requires Baidu Cloud Background to register an application for face recognition and get a set of API Key and Secret Key values.
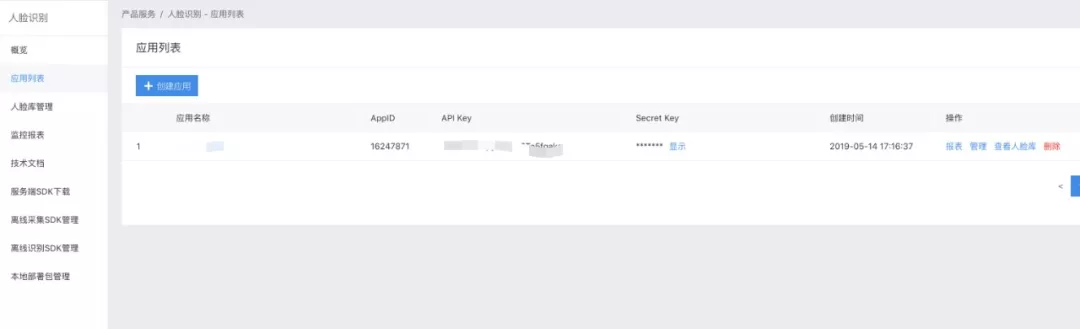
"access token" can then be obtained from the API documentation provided on the official website. Since ak has a validity period of one month, it only needs to be initialized once before normal face recognition can be performed using the face recognition interface.
appid = 'The one you registered for the app appid' api_key = 'The one you registered for the app ak' secret_key = 'The one you registered for the app sk' def get_access_token(): """ //Its access_token is usually valid for one month """ # This variable is assigned to itself API Key Value of client_id = api_key # This variable is assigned to itself Secret Key Value of client_secret = secret_key auth_url = 'https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id=' + client_id + '&client_secret=' + client_secret header_dict = {'User-Agent': 'Mozilla/5.0 (Windows NT 6.1; Trident/7.0; rv:11.0) like Gecko', "Content-Type": "application/json"} # Request Get To token Interface response_at = requests.get(auth_url, headers=header_dict) json_result = json.loads(response_at.text) access_token = json_result['access_token'] return access_token
Scripting
With the adb environment configured above, you can directly use the os module in python to execute the adb command to open the jitter App.
# Jitter App Application package name and initial Activity package_name = 'com.ss.android.ugc.aweme' activity_name = 'com.ss.android.ugc.aweme.splash.SplashActivity' def start_my_app(package_name, activity_name): """ //Open Application adb shell am start -n com.tencent.mm/.ui.LauncherUI :param package_name: :return: """ os.popen('adb shell am start -n %s/%s' % (package_name, activity_name))
Next, we need to take a screenshot of the currently playing video locally.It is important to note that the dithering video playback interface contains some cluttered elements such as the video creator's avatar and the BGM creator's avatar, which may cause some errors in the result of face recognition, so the image after screen capture needs to be "secondary clipped".
def get_screen_shot_part_img(image_name): """ //Get part of the phone screenshot :return: """ # screenshot os.system("adb shell /system/bin/screencap -p /sdcard/screenshot.jpg") os.system("adb pull /sdcard/screenshot.jpg %s" % image_name) # Open Picture img = Image.open(image_name).convert('RGB') # Original width and height of picture(1080*2160) w, h = img.size # Intercept parts, remove their avatars, other cluttered elements img = img.crop((0, 0, 900, 1500)) img.thumbnail((int(w / 1.5), int(h / 1.5))) # Save Locally img.save(image_name) return image_name
Now you can use the API provided by Baidu to get the face list of the above screenshots.
def parse_face_pic(pic_url, pic_type, access_token): """ //Face recognition 5 Within seconds :param pic_url: :param pic_type: :param access_token: :return: """ url_fi = 'https://aip.baidubce.com/rest/2.0/face/v3/detect?access_token=' + access_token # call identify_faces,Get a list of faces json_faces = identify_faces(pic_url, pic_type, url_fi) if not json_faces: print('No human face recognized') return None else: # Return to all faces return json_faces
From the above list of faces, sisters with sex between 18 and 30 and facial value over 70 were selected.
def analysis_face(face_list): """ //Analyze face to determine if it meets the criteria 18-30 Between, female, face value greater than 80 :param face_list:List of recognized faces :return: """ # Can you find beautiful women with high looks find_belle = False if face_list: print('Total Recognition%d Face, start to identify if there is a beautiful woman below~' % len(face_list)) for face in face_list: # Judging male or female if face['gender']['type'] == 'female': age = face['age'] beauty = face['beauty'] if 18 <= age <= 30 and beauty >= 70: print('Pigment Value:%d,Pass, meet the criteria!' % beauty) find_belle = True break else: print('Pigment Value:%d,Fail, continue~' % beauty) continue else: print('Gender is male,Continue~') continue else: print('No face was found in the picture.') return find_belle
Because the video is played continuously, it is difficult to judge if there is a lady with a high face in the video by intercepting a frame of the video.
In addition, most short videos are played for "10s+". Here we need to do face recognition for each video multiple times until we recognize the lady sister with high facial value.
# Maximum recognition time for a video RECOGNITE_TOTAL_TIME = 10 # Number of identifications recognite_count = 1 # Face Removal from Current Video Screenshot while True: # Get screenshots print('Beginning%d Secondary Screenshot' % recognite_count) # Capture useful areas of the screen, filter the avatars of video authors, BGM Author's Avatar screen_name = get_screen_shot_part_img('images/temp%d.jpg' % recognite_count) # Face recognition recognite_result = analysis_face(parse_face_pic(screen_name, TYPE_IMAGE_LOCAL, access_token)) recognite_count += 1 # No. n Time after recognition recognite_time_end = datetime.now() # This video shows a lady with a high face if recognite_result: pass else: print('Timeout!!!This is an unattractive video!') # Jump out of inner loop break
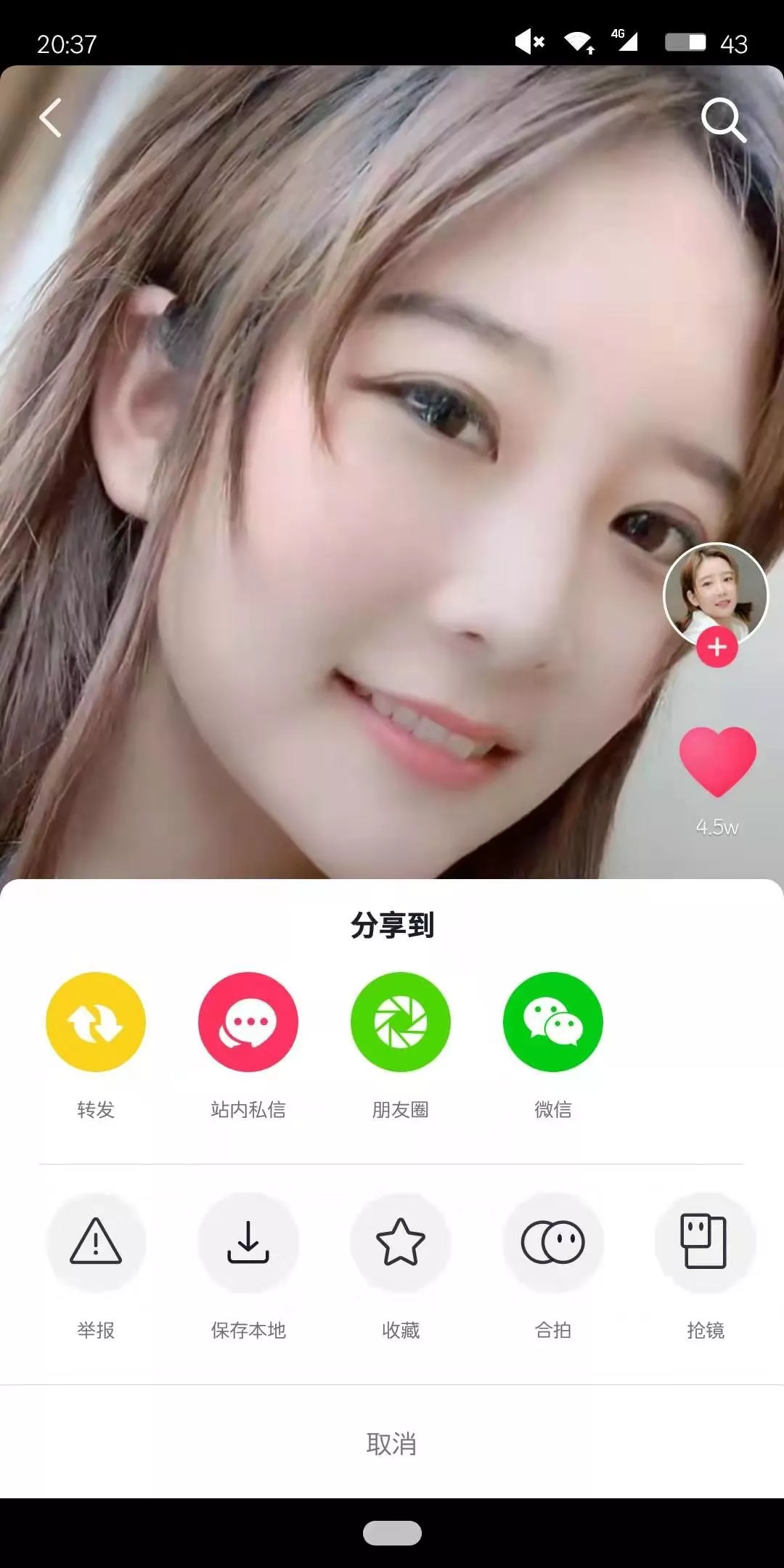
Get the coordinate locations of the Share and Save Local buttons, then click on the adb to download the video locally.
def save_video_met(): """ :return: """ # share os.system("adb shell input tap 1000 1500") time.sleep(0.05) # Save Locally os.system("adb shell input tap 350 1700")
In addition, since the process of downloading video is a time-consuming operation, a "simulated wait" operation is required before the download progress dialog box disappears.
def wait_for_download_finished(poco): """ //Download from Click to download completely :return: """ element = Element() while True: # Because it is a dialog box, it cannot be used Element Class to determine if an element exists for accurate processing # element_result = element.findElementByName('Saving to Local') # Current Page UI Tree element information # Note: Element exceptions may be retrieved when saving, which needs to be thrown and the loop terminated # com.netease.open.libpoco.sdk.exceptions.NodeHasBeenRemovedException: Node was no longer alive when query attribute "visible". Please re-select. try: ui_tree_content = json.dumps(poco.agent.hierarchy.dump(), indent=4).encode('utf-8').decode('unicode_escape') except Exception as e: print(e) print('Exceptions, handled by download~') break if 'Saving to Local' in ui_tree_content: print('Still downloading~') time.sleep(0.5) continue else: print('Download complete~') break
Once the video has been saved locally, you can simulate a sliding up action and skip to the next video.Loop above to filter out all high-looking ladies and sisters and save them locally.
def play_next_video(): """ Next Video Slide from bottom to top :return: """ os.system("adb shell input swipe 540 1300 540 500 100")
During the scripting process of brushing videos one by one, you may encounter advertisements, which we need to filter.
def is_a_ad(): """ //Determine if an ad is on the current page :return: """ element = Element() ad_tips = ['Go play', 'To experience', 'Download now'] find_result = False for ad_tip in ad_tips: try: element_result = element.findElementByName(ad_tip) # Is an advertisement,Jump out directly find_result = True break except Exception as e: find_result = False return find_resul #I'm learning Python In the process, I often don't want to learn because there is no information or no guidance, so I intentionally prepared a group of 592539176, there are a large number of groups PDF Books and tutorials are free to use!No matter which stage of learning your partner can get their own information!
Result conclusion
Running the script above will automatically turn on the jitter, and perform face recognition for each small video several times until you recognize the high-looking sister, save the video locally, and then continue with the next short video.
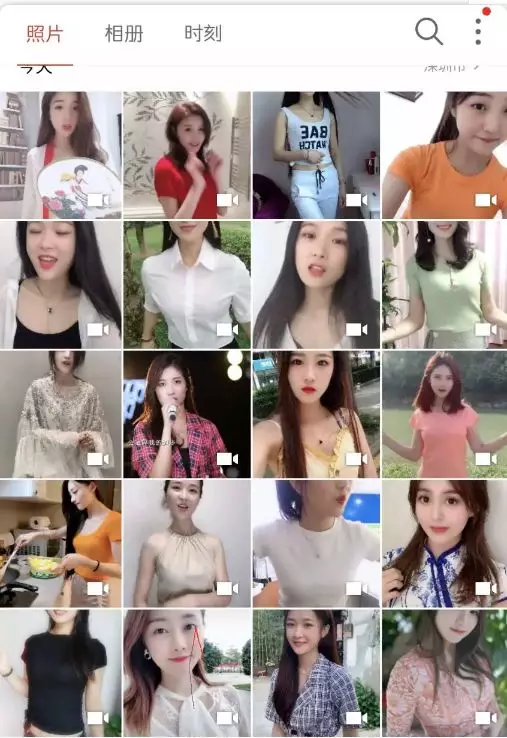
If you think the article is good, please share it.You must be my greatest encouragement and support.