1. Abstract Factory Pattern
Abstract Factory Pattern is to create other factories around one super factory. This super factory is also called the factory of other factories. This type of design pattern is a creation pattern, which provides the best way to create objects.
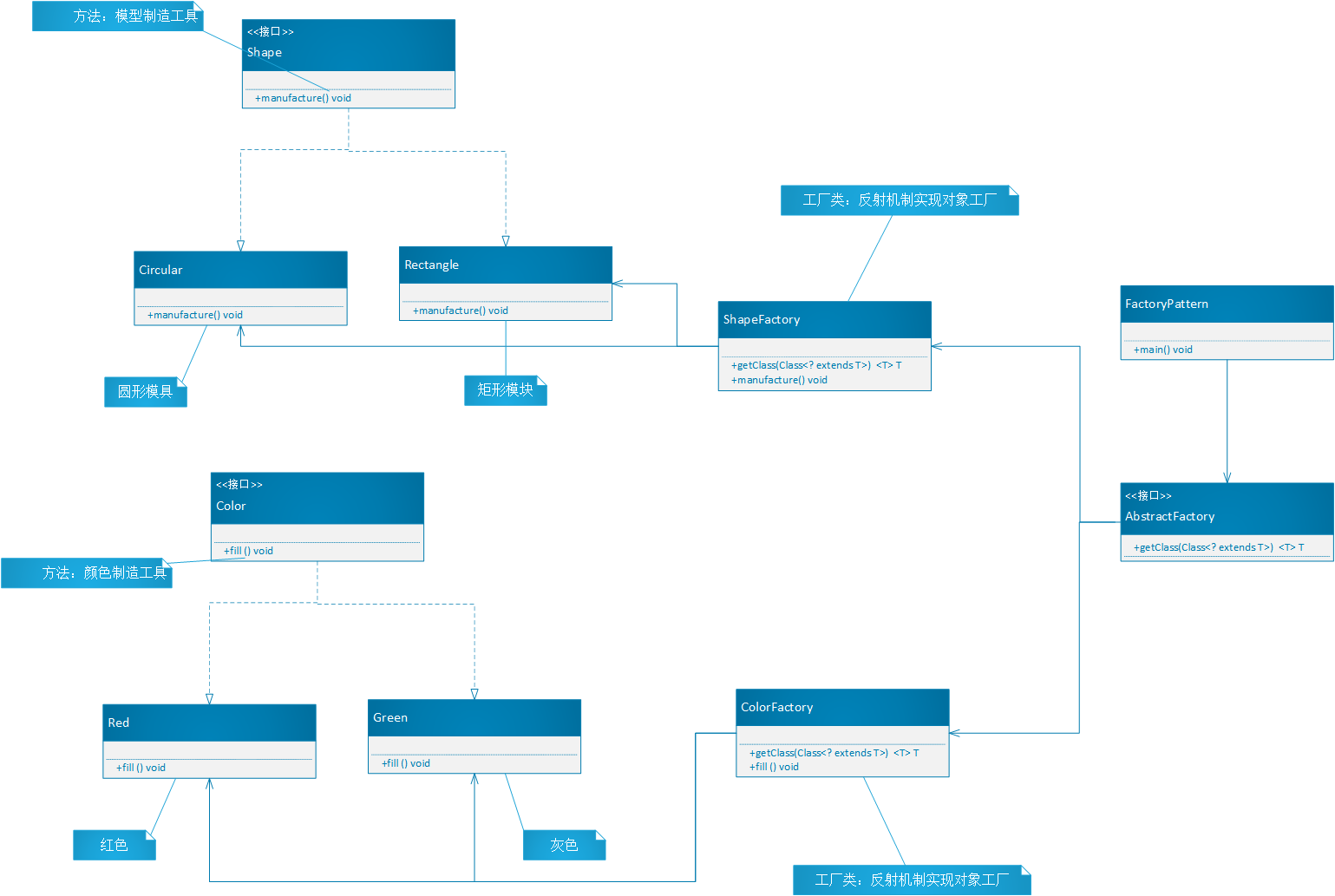
2. Code implementation
Scene: now we have to create two factories with one super factory, one factory to make mold and one factory to make pigment.
- Color factory:
public interface Color { void fill (); }
- Confidante:
import color.Color; public class Red implements Color{ @Override public void fill() { System.out.println("Make a red color"); } }
- Grey:
import color.Color; public class Green implements Color { @Override public void fill() { System.out.println("Make a gray color"); } }
- Mold factory (Shape):
public interface Shape { /** * Make various shape models */ void manufacture(); }
- Round:
public class Circular implements Shape { @Override public void manufacture() { System.out.println("I'm a round mold!"); } }
- Rectangle:
public class Rectangle implements Shape { @Override public void manufacture() { System.out.println("I'm a rectangular mold"); } }
- Colorfactory:
import color.Color; public class ColorFactory implements AbstractFactory{ Color obj = null; @Override public Color getClass(Class clazz){ try { obj = (Color) Class.forName(clazz.getName()).newInstance(); } catch (InstantiationException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } return obj; } public void fill(){ this.obj.fill(); } }
- Shapefactory:
import shape.Shape; public class ShapeFactory implements AbstractFactory{ Shape obj = null; @Override public Shape getClass(Class clazz){ try { obj = (Shape) Class.forName(clazz.getName()).newInstance(); } catch (InstantiationException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } return obj; } public void manufacture() { this.obj.manufacture(); } }
- AbstractFactory:
public interface AbstractFactory { <T> T getClass(Class<? extends T> clazz); }
- Factorypattern:
import color.Color; import color.impl.Green; import color.impl.Red; import shape.impl.Circular; import shape.impl.Rectangle; import shape.Shape; public class FactoryPattern { public static void main(String[] args) { AbstractFactory shapeFactory = new ShapeFactory(); Shape circular = shapeFactory.getClass(Circular.class); circular.manufacture(); Shape rectangle = shapeFactory.getClass(Rectangle.class); rectangle.manufacture(); AbstractFactory colorFactory = new ColorFactory(); Color green = colorFactory.getClass(Green.class); green.fill(); Color red = colorFactory.getClass(Red.class); red.fill(); } }
- Operation result:

- Advantage
When multiple objects in a product family are designed to work together, it can ensure that clients always use only the objects in the same product family. - Disadvantages:
Product family extension is very difficult. To add a product of a series, you need to add code in both abstract Creator and concrete Creator. - Usage scenario:
1. QQ for skin, a whole set together. 2. Generate programs for different operating systems.
(summary reference: rookie tutorial)