In web development, we often encounter problems with international language processing, so how can we internationalize?
What can you get?
- Use springgmvc and thymeleaf for internationalization.
- Use springgmvc and jsp for internationalization.
- Use springboot and thymeleaf for internationalization.
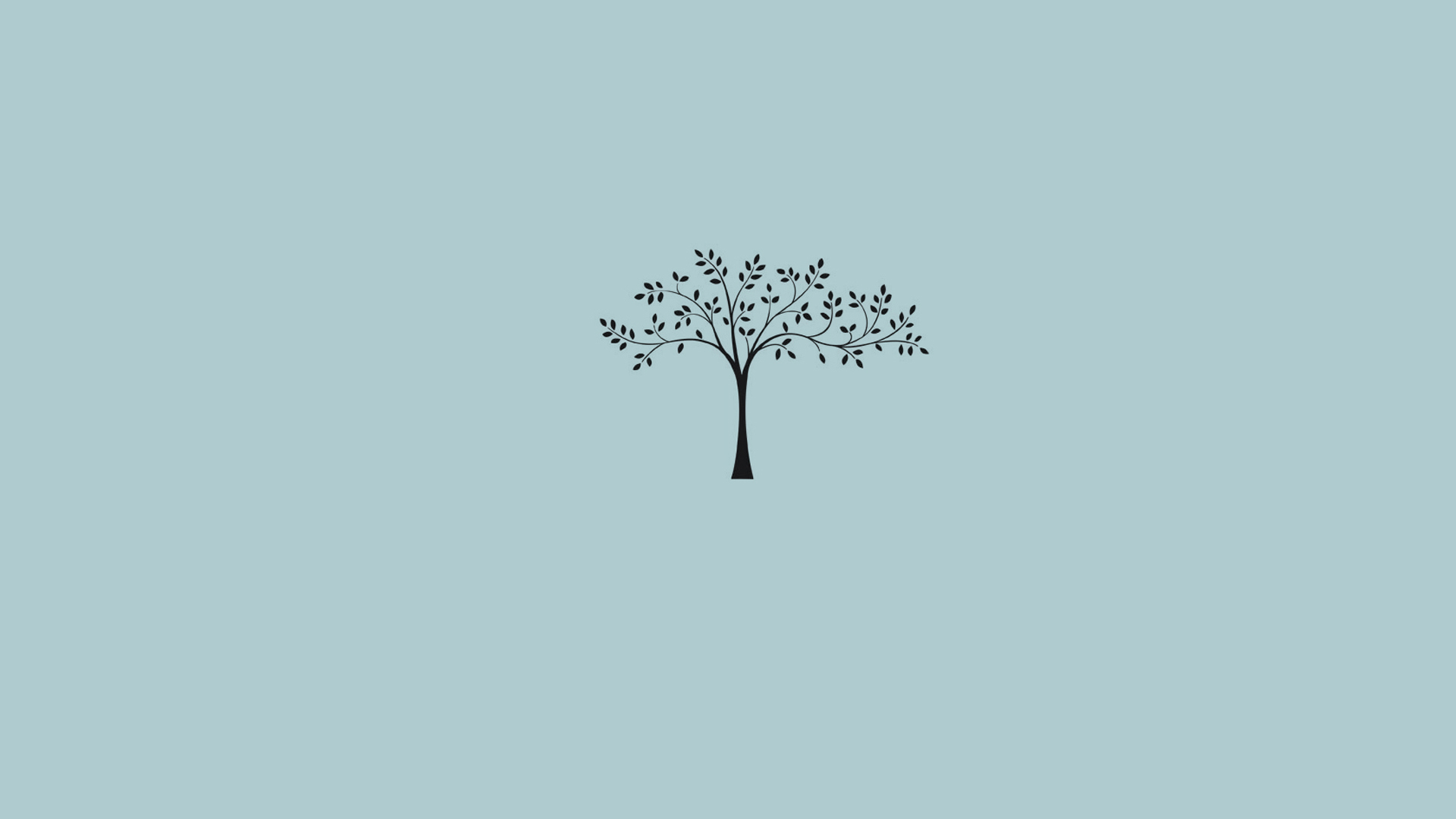
Concepts you must know
About i18n:
i18n (from English words)
The first and last characters I and N of the internationalization, 18 being the number of characters i n the middle), are short for "internationalization".In the field of information, internationalization (i18n) means that products (publications, software, hardware, etc.) can be adapted to the needs of different languages and regions without major changes.For programs, the interface can be displayed in different languages and regions without modifying the internal code.
In the era of globalization, internationalization is particularly important because potential users of products may come from all corners of the world.Usually associated with i18n is L10n (short for localization).
One: use springgmvc and thymeleaf for internationalization.
1. In the Spring MVC configuration file springmvc.xml for the project spring, you need to configure
- Resource File Binder ResourceBundleMessageSource
- SessionLocaleResolver (used to store Locale objects in Sessions for subsequent use)
- LocaleChangeInterceptor (used to get the locale information in the request, convert it to a Locale object, and get the LocaleResolver object).
<!-- Use ResourceBundleMessageSource Achieving Internationalized Resources--> <bean id="messageSource" class="org.springframework.context.support.ResourceBundleMessageSource"> <property name="basenames" value="messages"/> <property name="defaultEncoding" value="UTF-8"/> </bean> <bean id="localeResolver" class="org.springframework.web.servlet.i18n.SessionLocaleResolver"> <property name="defaultLocale" value="en_US"/> </bean> <mvc:interceptors> <bean class="org.springframework.web.servlet.i18n.LocaleChangeInterceptor"> <property name="paramName" value="lang"/> </bean> </mvc:interceptors>
2. Add method localeChange to the controller to handle internationalization and inject Bean instances of ResourceBundleMessageSource
@Autowired private ResourceBundleMessageSource messageSource; @GetMapping("/localeChange") public String localeChange(Locale locale){ String userName = messageSource.getMessage("userName",null,locale); String passWord = messageSource.getMessage("passWord",null,locale); System.out.println(userName+passWord); return "login"; }
3. Create international resource properties files messages_en_US.properties and messages_zh_CN.properties.
Note the naming format of these two files, otherwise parsing will fail.
And the two files I have here are located in my resources directory. When you create these two new files, they will be automatically archived for you. Don't think there's another layer on them. You can also build a folder along with them.
1,messages_en_US.properties userName=userName passWord=password 2,messages_zh_CN.properties userName=User name passWord=Password
4. Create a new html for the final display, using the thymeleaf template engine here. You can see another article of mine without the integration of springmvc and thymeleaf Integration of springmvc and thymeleaf
<!-- @Author: lomtom @Date: 2020/4/19 @Time: 16:51 @Email: lomtom@qq.com --> <!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>login</title> </head> <body> <h2 th:text="#{userName}+' '+#{passWord}"></h2> <a href="localeChange?lang=en_US">English</a> <a href="localeChange?lang=zh_CN">Chinese</a> </body> </html>
Final results:
2. Use springgmvc and jsp for internationalization.
The first three parts here are the same as above, the only difference is the display of the final view, using jsp, you need to use JSTL tag, where you need to introduce JSTL message tag.
That is, add <%@ taglib prefix="spring" uri="http://www.springframework.org/tags"%>
The value corresponding to the key in the resource properties file is then output through the key attribute of the <spring:message>element, as is the final jsp.
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="spring" uri="http://www.springframework.org/tags" %> <html> <head> <title>login</title> </head> <body> <p><spring:message code="userName"/></p> <p><spring:message code="password"/></p> <a href="localeChange?lang=en_US">English</a> <a href="localeChange?lang=zh_US">Chinese</a> </body> </html>
By clicking on the "Chinese" and "English" links in turn, you can see that the text displayed by the <spring:message>element can be dynamically displayed according to the language passed.
3. Use springboot and thymeleaf for internationalization.
No integration of springmvc with thymeleaf can be seen in another of my articles Integration of springmvc and thymeleaf
1. Create a configuration file to extract the messages to be displayed on the page
2. springboot is already configured with components and can be added
spring: # Let springboot manage configuration files messages: basename: i18n.login
3. Let the page be fetched (switch by default according to the browser language), using thymeleaf
<button type="submit" class="btn btn-default" th:text="#{login.btn}">Sign in</button>
4. Switch languages based on links
Principle: Internationalized locale (Regional Information Object), if customized, uses its own, not the system's
<a class="btn btn-sm" th:href="@{/index.html(l=zh_CN)}">[[#{login.zh}]]</a> <a class="btn btn-sm" th:href="@{/index.html(l=en_US)}">[[#{login.en}]]</a> <a class="btn btn-sm" th:href="@{/index.html(l=kor_KR)}">[[#{login.kor}]]</a>
5. Parser, because we set up our own region information object, we need to write our own parser.And inject into the container.
1,Create a new one LocaleResolver public class MyLocaleResolver implements LocaleResolver { @Override //Parse Region Information public Locale resolveLocale(HttpServletRequest httpServletRequest) { String l = httpServletRequest.getParameter("l"); Locale locale = Locale.getDefault(); if(!StringUtils.isEmpty(l)){ String[] split = l.split("_"); locale = new Locale(split[0],split[1]); } return locale; } @Override public void setLocale(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Locale locale) { } } 2,stay config File into container @Configuration public class MyMvcConfig implements WebMvcConfigurer { @Bean public LocaleResolver localeResolver(){ return new MyLocaleResolver(); } }
This was written too long, so you can paste out the code and study it yourself if you are interested:
1,html
<!-- User: Longtongtong Ouyang Date: 2019/12/22 Time: 16:42 --> <!DOCTYPE html> <html lang="ch" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>[[#{login.title}]]</title> <link rel="stylesheet" href="/webjars/bootstrap/4.4.1/css/bootstrap.css" th:href="@{/webjars/bootstrap/4.4.1/css/bootstrap.css}"> <script src="/webjars/jquery/3.3.1/jquery.js" th:src="@{/webjars/jquery/3.3.1/jquery.js}"></script> <script src="/webjars/bootstrap/4.4.1/js/bootstrap.js" th:src="@{/webjars/bootstrap/4.4.1/js/bootstrap.js}"></script> </head> <body> <div class="container"> <div class="row clearfix"> <div class="col-md-12 column"> <form class="form-horizontal" role="form" th:action="@{/user/login}" method="post"> <div class="form-group text-center"> <h1 class="h3 mb-3 font-weight-normal" th:text="#{login.tip}">Please sign in</h1> <p style="color: red" th:text="${msg}" th:if="${not #strings.isEmpty(msg)}"></p> </div> <div class="form-group"> <label for="username" class="col-sm-2 control-label" th:text="#{login.username}">Username</label> <div class="col-sm-10"> <input type="text" class="form-control" name="username" id="username" /> </div> </div> <div class="form-group"> <label for="password" class="col-sm-2 control-label" th:text="#{login.password}">Password</label> <div class="col-sm-10"> <input type="password" class="form-control" name="password" id="password" /> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <div class="checkbox"> <label><input type="checkbox" />[[#{login.remember}]]</label> </div> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <button type="submit" class="btn btn-default" th:text="#{login.btn}">Sign in</button> </div> </div> <div class="form-group text-center"> <p class="mt-5 mb-3 text-muted">@ 2019</p> <a class="btn btn-sm" th:href="@{/index.html(l=zh_CN)}">[[#{login.zh}]]</a> <a class="btn btn-sm" th:href="@{/index.html(l=en_US)}">[[#{login.en}]]</a> <a class="btn btn-sm" th:href="@{/index.html(l=kor_KR)}">[[#{login.kor}]]</a> </div> </form> </div> </div> </div> </body> </html>
2,properties
1,default login.btn=Sign in login.en=English login.kor=Korean login.password=Password login.remember=Remember me login.tip=Please login login.title=Sign in login.username=User name login.zh=Chinese 2,English login.btn=Sign in login.en=English login.kor=Korean login.password=Password login.remember=Remember me login.tip=Please sign in login.title=Login login.username=Username login.zh=Chinese 3,Korean login.btn=로그인 login.en=영어 login.kor=한글 login.password=암호 login.remember=저를 기억하세요 login.tip=로그인하십시오. login.title=로그인 login.username=사용자 이름 login.zh=중국어 4,Chinese login.btn=Sign in login.en=English login.kor=Korean login.password=Password login.remember=Remember me login.tip=Please login login.title=Sign in login.username=User name login.zh=Chinese
3,java
1,LocaleResolver /** * Zone information can be carried over the connection */ public class MyLocaleResolver implements LocaleResolver { @Override //Parse Region Information public Locale resolveLocale(HttpServletRequest httpServletRequest) { String l = httpServletRequest.getParameter("l"); Locale locale = Locale.getDefault(); if(!StringUtils.isEmpty(l)){ String[] split = l.split("_"); locale = new Locale(split[0],split[1]); } return locale; } @Override public void setLocale(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Locale locale) { } } 2,config @Configuration public class MyMvcConfig implements WebMvcConfigurer { @Bean public LocaleResolver localeResolver(){ return new MyLocaleResolver(); } }
4,yml
spring: # Let springboot manage configuration files messages: basename: i18n.login
problem
Description: Random code when displaying Chinese
Solution: Set the encoding format of the international resource properties file to UTF-8, or of course, set the entire project encoding format to UTF-8