The following GIF diagram shows the layout jump without a picture placeholder:
See the full example in DartPad
If you have cached a picture placeholder in your app, you can use FadeInImage Widgets to display placeholders.If you want to use a widget instead of a picture as a placeholder, you can use Image.frameBuilder Properties.
Image.frameBuilder Attribute is responsible for building a picture widget with four parameters:
- Context: build context
- Child:widget child element
- Frame: A number that codes the frame, if the picture is still loading, for null
- WasSynchronous Loaded : Boolean value, when the picture is loaded true
When implementing a placeholder widget, you first need to pass the WasSynchronous Loaded Check to see if the picture has been loaded, and if the loading is complete, return child . If not, use AnimatedSwitcher Widget to create a placeholder-to-picture gradient animation:
class ImageWidgetPlaceholder extends StatelessWidget { const ImageWidgetPlaceholder({ Key key, this.image, this.placeholder, }) : super(key: key); final ImageProvider image; final Widget placeholder; @override Widget build(BuildContext context) { return Image( image: image, frameBuilder: (context, child, frame, wasSynchronousLoaded) { if (wasSynchronousLoaded) { return child; } else { return AnimatedSwitcher( duration: const Duration(milliseconds: 500), child: frame != null ? child : placeholder, ); } }, ); } }
With placeholders, the layout never jumps back and forth again, and the pictures have a gradual effect:
See the full example in DartPad
Pre-cached pictures
If your app has a welcome screen before displaying the picture interface, you can call precacheImage Method to pre-cache pictures.
precacheImage(NetworkImage(url), context);
Take a look at the effect:
See the full example in DartPad
Disable navigation transition animation in Flutter web app
Navigation transition animations are commonly used when users switch pages, which is a good way to let users know where they are in a mobile app.However, such interactions are rarely seen on the web.So to improve awareness, you can disable transitional animation between pages.
By default, MaterialApp Different transition animations are used depending on the platform (Android slides up, iOS slides right (left).To override this default behavior, you need to create a custom PageTransitionsTheme Class.You can use the kIsWeb constant to determine if an app is running on the web.If so, by returning child To disable transitional animations:
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; class NoTransitionsOnWeb extends PageTransitionsTheme { @override Widget buildTransitions<T>( route, context, animation, secondaryAnimation, child, ) { if (kIsWeb) { return child; } return super.buildTransitions( route, context, animation, secondaryAnimation, child, ); } } ### End Successful interviews are inevitable, because I have done enough preparation before that, not only pure brushing, but also some brushing Android Core Architecture Advanced Knowledge Points,**For example: JVM,High concurrency, multithreading, caching, hot fix design, plug-in framework interpretation, component framework design, picture loading framework, network, design mode, design ideas and code quality optimization, program performance optimization, development efficiency optimization, design mode, load balancing, algorithms, data structure, advanced UI Promotion, Framework Kernel Resolution, Android Component Kernel, etc.** 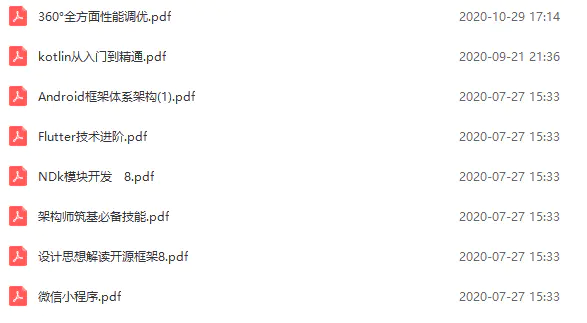 Not only learning documents, videos+Notes improve learning efficiency, but also stabilize your knowledge, forming a good and systematic knowledge system.Here, the author shares a video and information dissected from the perspective of architecture philosophy, which combs many years of experience in architecture and prepares for the latest recording in nearly 6 months. I believe this video can give you different inspiration and harvest. 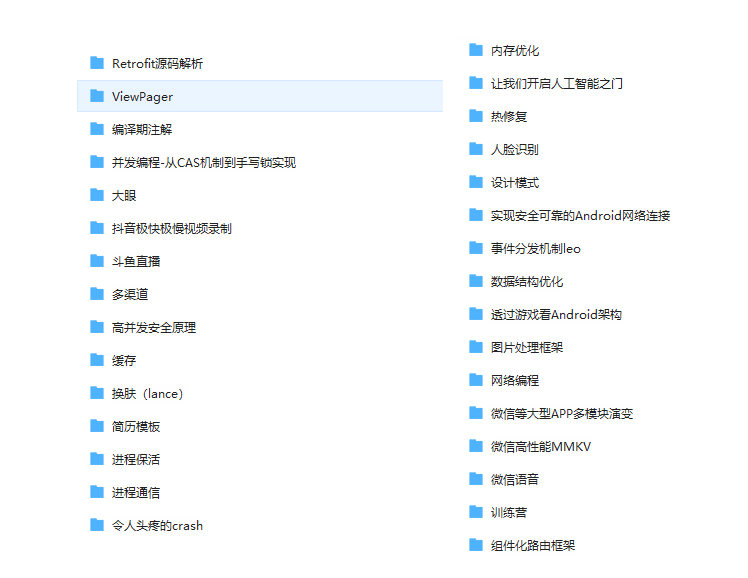 ##### Android Advanced Learning Database A total of ten themes, including Android Advance all learning materials, Android Advanced video, Flutter,java Basics, kotlin,NDK Modules, computer networks, data structures and algorithms, WeChat applets, interview question resolution, framework Source code! 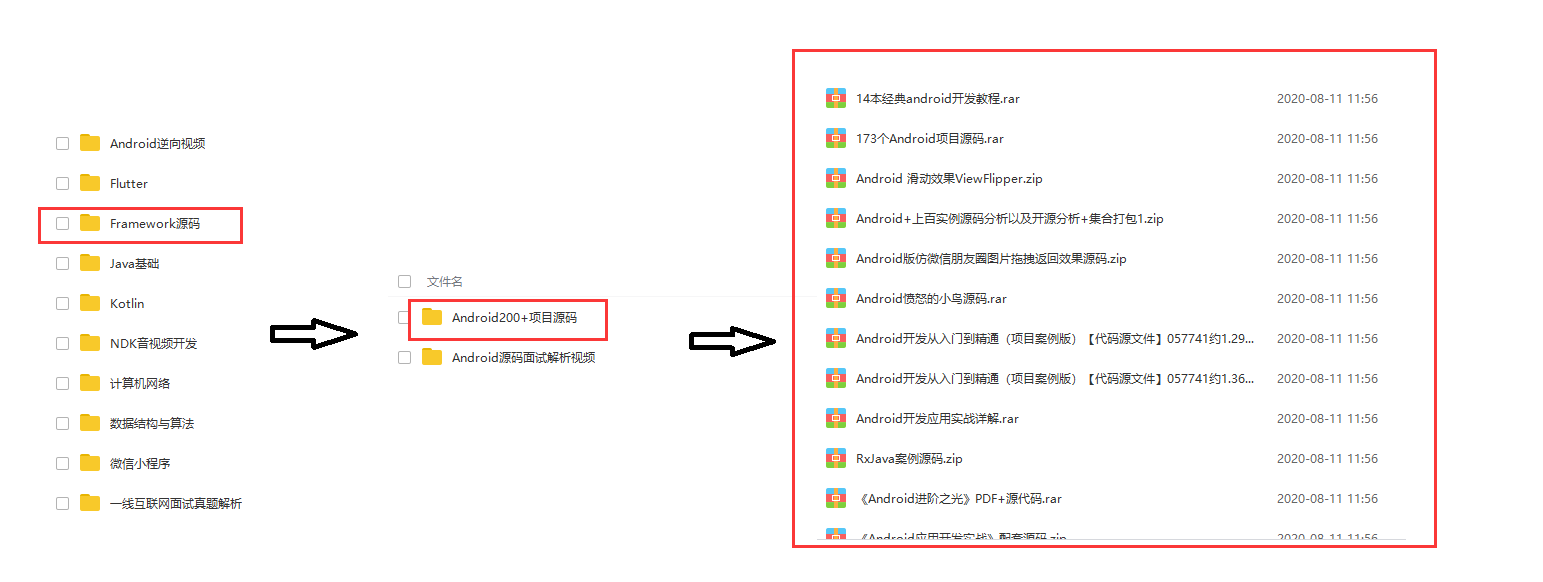 ##### True subject for factory interview PS: Twenty sets of 12th-line Internet companies previously collected for Autumn Entrance Android True interview questions (including BAT,Millet, Huawei, Meituan, Didi) and I organize myself Android Review notes (including Android Basic knowledge points, Android Expand your knowledge points, Android Source Parsing, Design Mode Summary, Gradle Summary of knowledge points, common algorithm questions.) 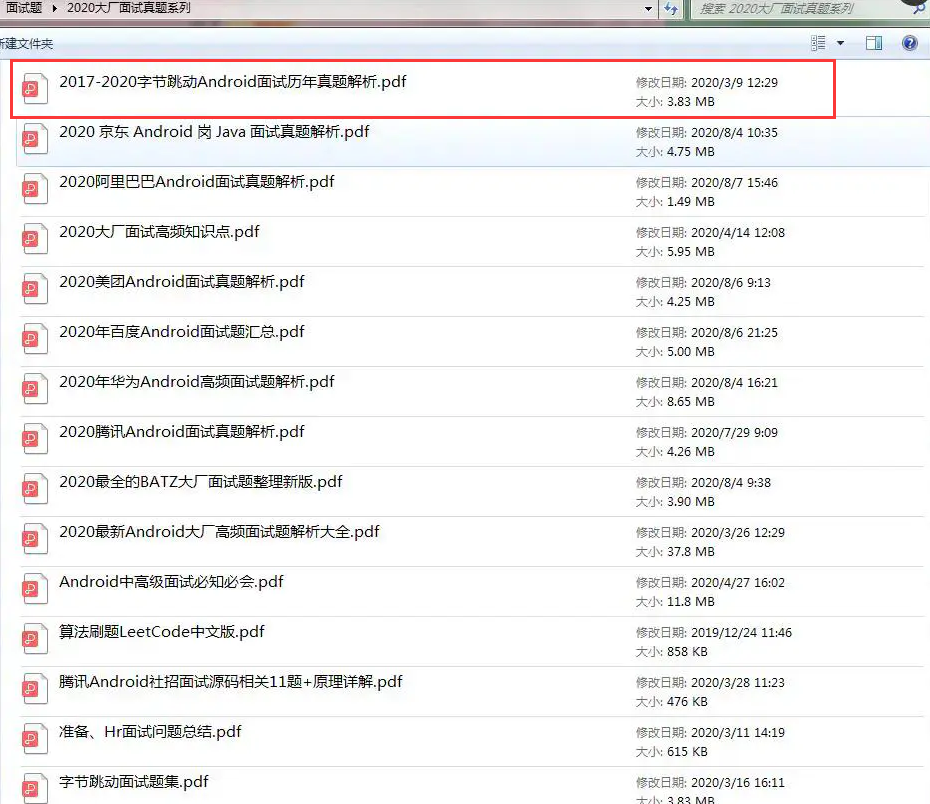 **<2017-2021 Byte bounce Android Interview Calendar Year True Topic Analysis** 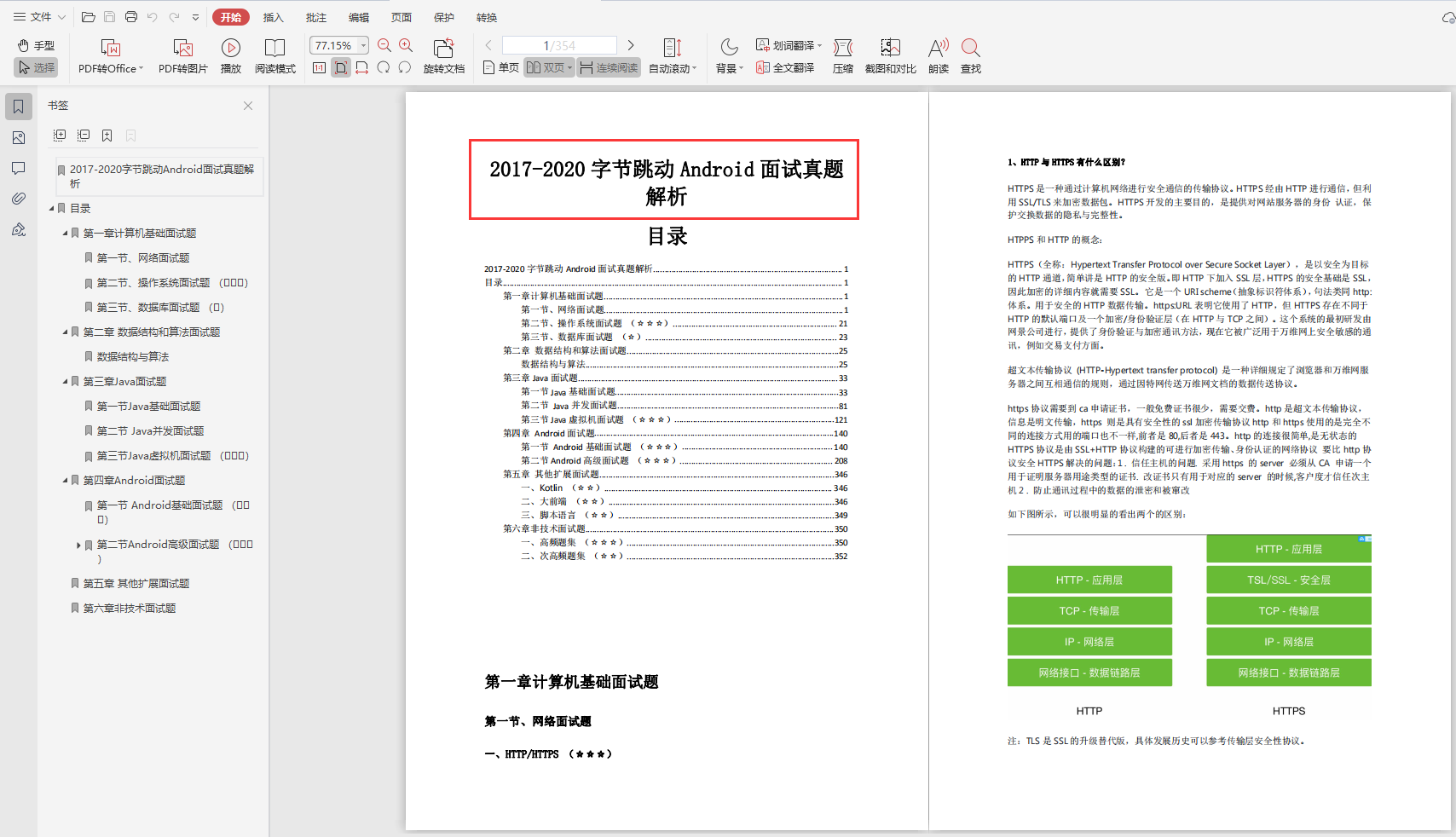 * ##### **[CodeChina Open Source Project: Android Learning Notes Summary + Mobile Architecture Video + Factory Interview True Topics + Project Actual Source](https://codechina.csdn.net/m0_60958482/android_p7)** 5641)] * ##### **[CodeChina Open Source Project: Android Learning Notes Summary + Mobile Architecture Video + Factory Interview True Topics + Project Actual Source](https://codechina.csdn.net/m0_60958482/android_p7)**