welcome to the front end technology camp! If you are also a front-end learner or have the idea of learning from the front-end, follow me to attack the front-end from scratch.
we are committed to introducing front-end knowledge and our own shortcuts as detailed and concise as possible, which is also a record on the way of learning. Welcome to explore
1, JavaScript process control
Process control: controls the execution sequence of codes.
There is execution order in the process of program execution, and the functions to be completed can be realized by controlling the execution order of code
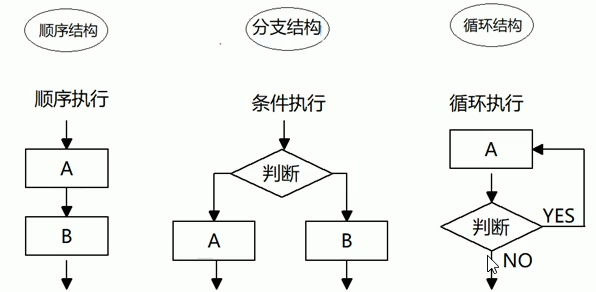
Sequential structure
The simplest and most basic process control has no specific syntax structure, and the program will be executed in the order of the code
Branching structure
In the process of executing code from top to bottom, different path codes are executed according to different conditions to get different results
if statement:
Syntax structure of if statement
if (Conditional expression) { Execute statements; }
Execution idea: if the conditional expression result in if is true, execute the execution statement in curly braces; If false, the execution statement in curly braces will not be executed, and the code after the if statement will be executed
//Case: entering an Internet cafe /* var age = prompt('Please enter your age: '; if (age>=18) { alert('Welcome ') } if(age<18) { alert('Minors on what net, go back and do your homework ') } */
if else statement:
Syntax structure of if else statement
if (Conditional expression) { Execute statement 1; } else { Execute statement 2; }
Execution idea: if the expression result is true, execute statement 1; otherwise, execute statement 2 and select one more to execute
var age = prompt('Please enter your age:'); if (age >= 18) { alert('Welcome'); } else { alert('What net do minors get on? Go back and do your homework'); }
If else statement:
If else statement syntax:
if (Conditional expression 1) { Execute statement 1; } else if (Conditional expression 2) { Execute statement 2; } else if (Conditional expression 3) { Execute statement 3; } else { Last statement; }
Execution idea: if expression 1 is satisfied, execute 1, and exit the entire if branch statement after execution
If expression 1 is not satisfied, judge whether statement 2 is satisfied, and so on. Select one more to execute. You can have as many conditions as you want in else if
var num = prompt('Please enter the time(1~24): '); if (num>=22) { alert('It's late at night. Pay attention to rest'); } else if (num>18) { alert('Good evening'); } else if (num>14) { alert('Good afternoon'); } else if (num>=10) { alert('Good noon'); } else if (num>6){ alert('good morning'); } else { alert('It's early morning'); }
Ternary expression: a formula composed of ternary operators (?:) is called a ternary expression. Can make some simple conditional choices
Syntax structure: conditional expression? Expression 1: expression 2
Execution idea: if the result of the conditional expression is true, the value of expression 1 will be returned; if false, the value of expression 2 will be returned
var num = 10; var result = num > 5 ? 'by the way' : 'no, it isn't'; //An expression has a return value console.log(result); //by the way
switch statement: used to execute different code based on different conditions. Select one from many. It is mainly used to set a series of specific value options for variables
Switch: switch, case: Option
Syntax structure:
switch(expression) { case value1: Execute statement 1; break; case value2: Execute statement 2; break; default: Execute the last statement; }
Execution idea: the value of the expression matches the value one by one. If the matching is successful, the case statement will be executed, otherwise the statement in default will be executed
Expressions are often written as variables;
When the variable matches the value in case, it must be congruent;
If there is no break in the current case, you will not exit the switch and continue to the next step
var num = 2; switch (num) { case 1: console.log('This is 1'); break; case 2: console.log('This is 2'); break; case 3: console.log('This is 3'); break; default: console.log('No matching results'); }
Differences between switch and if else statements:
Generally, they can be replaced with each other
switch: | if else if : |
---|---|
Generally, case is used to compare the determined value | More flexible, often used to judge the range |
It is more efficient to execute conditional statements directly to the program after conditional judgment | If there are multiple conditions, it is more efficient to judge multiple times and fewer branches |
Cyclic structure
Loop: repeats certain statements
Loop body: a group of repeatedly executed statements. Whether to continue execution depends on the termination condition of the loop
Loop statement: a statement consisting of the loop body and the termination condition of the loop
for loop: repeats some code, usually related to counting
- for can execute different codes repeatedly, because the counter variable i exists, and i will change every time
- Statements can be nested in for:
- The for loop repeats some of the same operations
Syntax structure:
for (initialize variable;Conditional expression;Operation expression) { Circulatory body } //Initialization variable: a common variable declared with var, which is usually used as a counter //Conditional expression: used to determine whether to continue the execution of each cycle, which is the termination condition //Operation expression: it is the code executed at the end of each cycle. It is often used for counter variable update (increment or decrement)
//Code verification for (var age = 1; age <= 100; age++) { if (age == 1) { console.log('I'm one year old!'); } else if (age == 100) { console.log('I am a century old man!'); } else { console.log('I this year' + age + 'Years old'); } }
Double for loop:
Grammatical structure
for (Initialization variable of outer layer;Conditional expression of outer layer;Operation expression of outer layer) { for(Initialization variable of inner layer;Conditional expression of inner layer;Inner layer operation expression) { // Execute statements; } }
Implementation idea:
The inner loop can be regarded as the statement of the outer loop
The outer loop executes once, the inner loop executes all, and then executes the next round
//Code verification for (var i=1;i<=3;i++) { console.log('This is the second phase of the outer cycle' + i + 'second'); for (var j=1;j<=3;j++) { console.log('This is the second stage of the inner cycle' + j + 'second'); } }
while loop:
while syntax structure:
while (Conditional expression) { Circulatory body; }
Execution idea: when the result of the conditional expression is true, execute the loop body, otherwise exit the loop
//Code validation: // There are also counters and initialization variables var num = 1; while (num<=100) { console.log('N Mr. ness'); num++;// There should also be an operation expression to complete the counter update and prevent dead circulation }
do... while loop:
do... while syntax structure:
// do while loop do { Circulatory body; } while (Conditional expression)
Execution idea: execute the loop body first, and then judge the condition. If the condition expression is true, continue to execute the loop body, otherwise exit the loop
//Code validation: var i = 1; do { console.log('how are you'); i++; } while (i <= 100)
Summary of cycle structure:
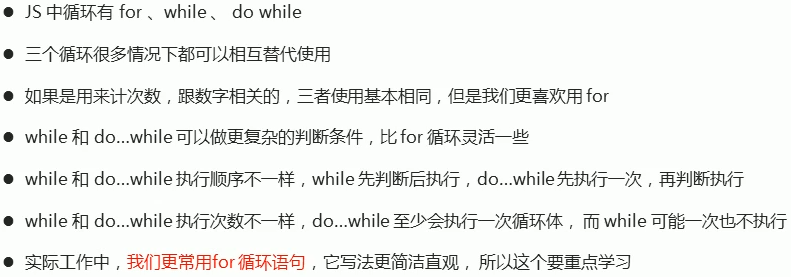
continue and break
Continue keyword is used to immediately jump out of this cycle and continue the next cycle
//The code after continue in the loop body will be executed less than once // Find the sum of integers between 1 and 100 except that they can be divided by 7 var sum = 0; for (var i = 1; i <= 100; i++) { if (i % 7 == 0) { continue; } sum += i; } console.log(sum);
The break keyword is used to jump out of the entire loop (end of loop)
//break for (var i=1;i<=5;i++) { if(i == 3) { break; } console.log('This is the second' + i); }
2, Array
Concept: it refers to a set of data, in which each data is called an element, and any type of element can be stored in the array
It can store a group of relevant data together and provide convenient access;
Arrays are an elegant way to store a set of data under a single variable name
Create array
1. Create an array with new
var array name = new Array()// A new empty array was created
2. Create an array using array literal (common)
var array name = [];
3. Any data type can be placed in the array, but separated by commas
Declaring an array and assigning values is called array initialization
Do not assign a value to the array name directly, otherwise there will be no array elements in it
var Array name = new Array();//A new empty array was created var arr = [1,2,'N brother',true];
basic operation
Gets the elements in the array
Index of array (subscript): the ordinal number (starting from 0) used to access array elements
//The array can access and modify the corresponding array elements through the index, and obtain the array elements through the array name [index]
var arr = [1, 2, 'N brother', true]; console.log(arr[2]);//Get 'N'
Traversal array
Traversal: each element in the array is accessed once from beginning to end
var arr =['N','brother','You are the one']; for (var i=0;i<3;i++) { console.log(arr[i]); }
Array length
Array length: array name. Length accesses the number (length) of array elements and dynamically monitors the number of array elements
var arr =['N','brother','You are the one']; console.log(arr.length);// 3
case
Sum and average the array:
var arr = [2,6,1,7,4]; var sum = 0; var average = 0; for (var i = 0; i<arr.length;i++) { sum += arr[i]; } average = sum/arr.length; console.log(sum,average);
Maximum:
//Maximum var arr1 = [2, 6, 1, 7, 4, 10, 25, 20]; var max = arr1[0]; for (var a = 1; a < arr1.length; a++) { if (arr1[a] > max) { max = arr1[a]; } } console.log('The maximum value is:' + max);
Convert array to string:
var sep = '!'; // Strings are separated by symbols var arr2 = ['red', 'blue', 'pink', 'skyblue']; var str = ''; //Create a new array to hold the string for (var b = 0; b < arr2.length; b++) { str += arr2[b] + sep; } console.log(str);
Add an element in the array: modify the length:
//Add elements in the array: 1. Modify the length var arr3 = ['N', 'That's it.', 'hehe', '1']; console.log(arr3.length); arr3.length = 5; // Modify the length to 5. No value is given. It is undefined by default //Add elements in the array: 2. Modify the index number and append arr3[4] = 'sir'; // Add console.log(arr3); arr3[3] = 'NAIS'; // Modify, change '1' to 'ness' console.log(arr3);
//Flip array
// Flip array var arr3 = ['of','I','yes','you']; var newArr3 = []; for (var c = arr3.length-1;c>=0;c--) { newArr3[newArr3.length] = arr3[c]; } console.log(newArr3);
Bubble sorting
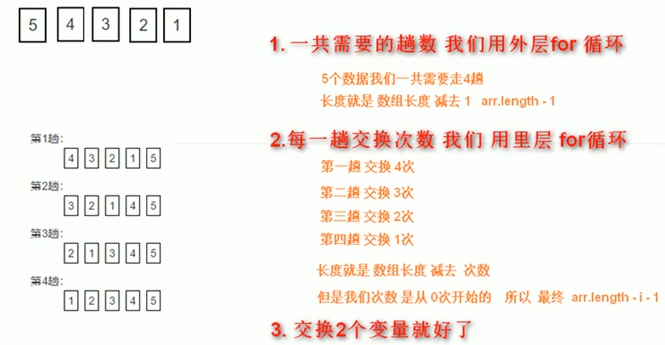
// Pairwise comparison var arr = [5, 4, 3, 2, 1]; for (var i = 0; i <= arr.length - 1; i++) { //Outer cycle times for (var j=0;j<=arr.length-i-1;j++) { // Inner layer cycle times //Internal exchange of two variables, the former and the latter element are compared if (arr[j] >arr[j+1]) { var temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } } } console.log(arr);
3, Interview questions
1, How does JS implement asynchrony?
JS engine is single threaded, but the reason why it can realize asynchrony lies in the event loop and task queue system.
Event cycle:
JS will create a loop similar to while (true). The process of executing the loop body every time is called Tick. The process of each Tick is to check whether there are events to be processed. If there are, take out the relevant events and callback functions and put them into the execution stack for execution by the main thread. The events to be processed will be stored in a task queue, that is, each Tick will check whether there are tasks to be executed in the task queue.
Task queue:
The asynchronous operation adds the relevant callback to the task queue. Different asynchronous operations are added to the task queue at different times, such as onclick, setTimeout and Ajax. These asynchronous operations are executed by the browser kernel's webcore. The browser kernel contains three web APIs, namely DOM Binding, network and timer modules.
onclick is handled by the DOM Binding module. When an event is triggered, the callback function will be immediately added to the task queue.
setTimeout is delayed by the timer module. When the time arrives, the callback function will be added to the task
In the queue.
ajax is handled by the network module. After the network request is completed and returned, the callback is added to the task queue.
Main thread:
JS has only one thread, which is called the main thread. The event loop is executed after the code in the execution stack in the main thread is executed. Therefore, if the code to be executed in the main thread takes too long, it will block the execution of event loops and asynchronous operations.
Only when the execution stack in the main thread is empty (that is, after the synchronous code is executed), will the event loop be carried out to observe the event callback to be executed. When the event loop detects an event in the task queue, it will take out the relevant callback and put it into the execution stack for execution by the main thread.
2, What is AJAX? How?
ajax is a technology that can refresh local web pages, which can refresh web pages asynchronously.
The implementation of ajax mainly includes four steps:
(1) Create the core object XMLhttpRequest;
(2) Use the open method to open the connection with the server;
(3) Send a request using the send method; (in case of "POST" request, the request header needs to be set additionally)
(4) Listen to the server response and receive the return value.
This issue ends here. Thank you for reading! If you have any questions, leave a message and reply in time
- Point praise and attention, and continuously update practical skills, popular resources, software tutorials, etc
- If you have any software, film and television tutorial resources, textual research materials and other needs, just leave a message