@RequestParam
@Requestparameter is often used to handle simple type bindings. Its principle is to obtain the parameter value through request.getParameter(). Because the request.getParameter() method is used to obtain parameters, you can process the values of parameters in the GET method or in the form in the post method; Submission methods: GET and post; Annotation has two attributes: value and required; Value is used to specify the id name of the value to be passed in (that is, the key of the request parameter corresponds to the value of the name of the form attribute). Required is used to indicate whether the parameter must be bound. The default is true, that is, the request parameter must carry the parameter. If not, an error will be reported;
example:
@PostMapping("/method12") public ApiResponse method12(@RequestParam(value = "id",required = true) int id, @RequestParam(value = "name",required = false)String name){ String s = id + "" + name; System.out.println(s); return ApiResponse.ok().data(s); }
Note: both get and post methods here can access parameters by putting them into Params. If it is a post request, put the parameters into form_ Both data and x-www-formdate-urlencoded can be received by the back end. Here @ RequestParam can be used in more than one interface method parameter
Interface method parameters do not use any comments: in fact, the default is to use the Request.getParameter() method to obtain parameters, but @ requestparameter is not displayed. However, there are differences. When @ requestparameter annotation is not used, the front-end request parameters can access the interface normally with or without @ requestparameter annotation, but the received parameter value is empty, The required value of @ RequestParam is the same as false. When @ RequestParam annotation is used and the required value is not specified as false, the front-end request must carry parameters, or an error will be reported directly
@RequestBody
@RequestBody is applicable to the front-end request with POST as the request mode, and the request parameters are placed in the request body in Json format. Therefore, when using @ RequestBody to receive data, it is generally submitted in POST mode, and the parameters are placed in the request body in Json format.
The request header content type of processed data: application / JSON, application / XML, etc. cannot be the content encoded by application/x-www-form-urlencoded;
Spring MVC mainly parses the data in HttpEntity by using HttpMessageConverters configured by HandlerAdapter, and then binds it to the corresponding bean.
Of course, in the back-end interface, @ RequestBody and @ RequestParam() can be used at the same time, but it should be noted that there can only be one @ RequestBody and multiple @ RequestParam().
Usage example:
@RequestBody + basic data List+json
@PostMapping("/method8") public ApiResponse method8(@RequestBody List<String> hobbies){ System.out.println(hobbies); return ApiResponse.ok().data(hobbies); }
@RequestBody + custom object List+json
@PostMapping("/method9") public ApiResponse method9(@RequestBody List<Dog> dogs){ System.out.println(dogs); return ApiResponse.ok().data(dogs); }
@RequestBody+map+json
@PostMapping("/method2") public ApiResponse method2(@RequestBody Map<String,Integer> map){ System.out.println(map.toString()); return ApiResponse.ok().dataMap(map); }
@RequestBody + entity class object + json
@PostMapping("/method4") public ApiResponse method4(@RequestBody Dog dog){ System.out.println(dog.toString()); return ApiResponse.ok().data(dog); }
@RequestBody+Vo encapsulates basic data and List and user-defined entity objects + json
@PostMapping("/method6") public ApiResponse method6(@RequestBody DemoVo demoVo){ System.out.println(demoVo.toString()); return ApiResponse.ok().data(demoVo); } ```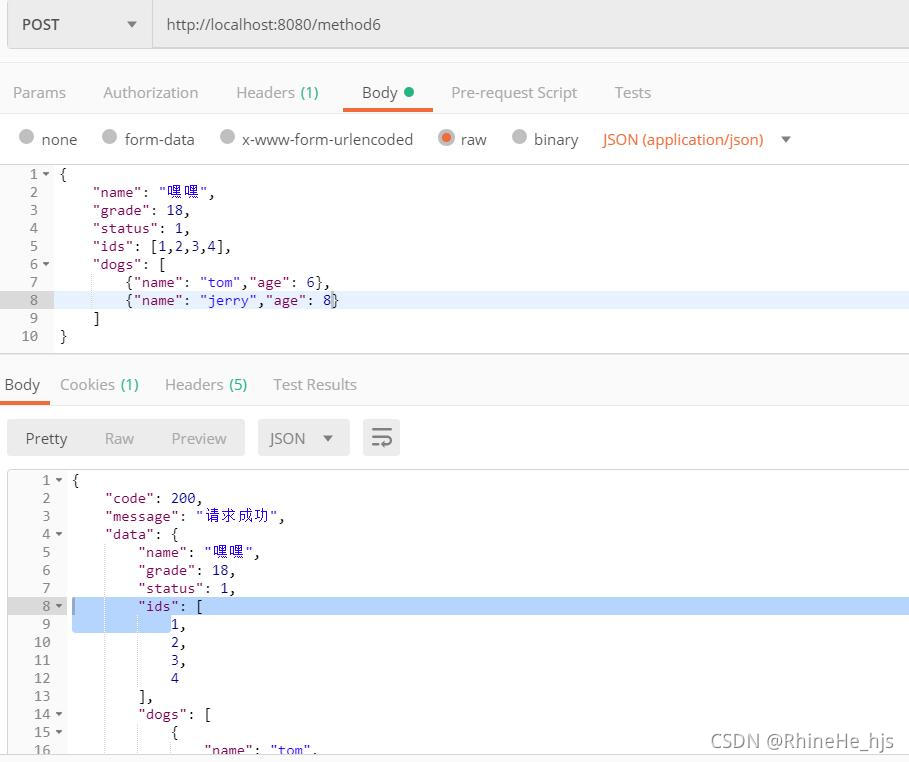 @RequestBody+Vo Encapsulate multiple custom entity objects+json ```java @PostMapping("/method10") public ApiResponse method10(@RequestBody UserVo userVo){ System.out.println(userVo); return ApiResponse.ok().data(userVo); }
@PathVariable+@RequestBody+ basic data and +json collection of the image collection and basic data simultaneous interpreting (the collection object can also be customized object here).
@PostMapping("/method/{id}")//The id parameter here is part of the request path public ApiResponse method7(@PathVariable("id") int id, @RequestBody List<String> hobbies){ String s = id + "" + hobbies; System.out.println(s); return ApiResponse.ok().data(s); }
What's the difference between @RequestParam+@RequestBody+ basic data and +json collection, object collection and object simultaneous interpreting (where the collection object can also be customized object) is not different from using @PathVariable, and both can use more @RequestParam or @PathVariable to reflect more data in the address bar.
@PostMapping("/method11") public ApiResponse method11(@RequestParam("id") int id, @RequestBody List<Dog> dogs){ String s = id + "" + dogs; System.out.println(s); return ApiResponse.ok().data(s); }
@RequestBody request parameter optimization
This involves using @ RequestBody to receive different objects
- Create a new entity and insert both entities. This is the simplest, but not "elegant".
- Accept the request body with map < string, Object > and deserialize it into each entity.
- Similar to method 2, but more elegant, implement your own HandlerMethodArgumentResolver reference resources