The difference between process oriented and object-oriented
sketch
- The process oriented idea is suitable for simple transactions that do not need cooperation. For example, simply driving a car does not need to cooperate with others.
- The object-oriented idea is suitable for complex transactions, and 1234 steps cannot be listed. Like how to build a car? Building a car is too complicated and requires a lot of cooperation.
summary
- Object oriented and process oriented are both ways of thinking to solve problems and ways of code organization.
- Process oriented can be used to solve simple problems.
- Solving complex problems: object-oriented is used in macro processing, and process oriented is still used in micro processing
Concepts of objects and classes
sketch
- Objects have different methods, properties, variables, etc
- Class can be regarded as a template or drawing. The system creates objects according to the definition of class.
- Class: call it class. Object: called object, instance.
- An object of a class is equivalent to an instance of a class. Same meaning.
Class definition
- Each source file must have one and only one public class, and the class name and file name must be consistent.
- A Java file can define multiple class es at the same time
- For a class, there are generally three common members: attribute field, method and constructor. All three members can define zero or more.
Code example: how classes are defined
// The classes defined below are empty and have no practical significance. You also need to define the specific information of the class. // Each source file must have one and only one public class, and the class name and file name must be consistent. public class SxtStu { } // A Java file can define multiple class es at the same time class Tyre{ } class Emage{ } class Try{ }
Code example: simple student class writing
// For a class, there are generally three common members: attribute field, method and constructor. All three members can define zero or more. public class SxtStu { // Properties (member variables) int id; String sname; int age; // method void study(){ System.out.println("I'm fishing and learning!"); } // Construction method SxtStu(){ } }
Attribute (field)
sketch
- Property is used to define the data or static characteristics contained in the class or class object.
- Attribute scope is the entire class body.
- When defining a member variable, you can initialize it. If not, Java initializes it with the default value.
Attribute definition format
[Modifier ] Property type property name = [Default value];
Member variable defaults
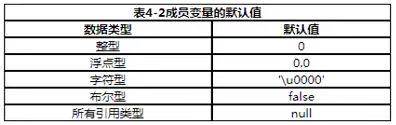
method
sketch
- Method is used to define the behavior characteristics and function implementation of the class or an instance of the class. For example, a student class can have the behavior characteristics or function realization of learning or playing games
- Methods are abstractions of the behavioral characteristics of classes and objects. Methods are very similar to procedure oriented functions.
- In process oriented, function is the most basic unit, and the program is composed of function calls.
- In object-oriented, the basic unit of the whole program is class, and the method is subordinate to class and object.
Method definition format
[Modifier ] Method return type method name(parameter list ){ // n statements }
Code example:
public class SxtStu { // method void study(){ System.out.println("I'm fishing and learning!"); } void play(){ System.out.println("I was playing games! LOL Mobile Games!"); } // Construction method SxtStu(){ } }
Code example: Call of complete object and class methods
- The program must have a main method as the entry for program execution
- Constructor: the object used to create this class. The construction method without parameters can be automatically created by the system.
- For a class, there are generally three common members: attribute field, method and constructor. All three members can define zero or more.
public class SxtStu { // Properties (member variables) int id; String sname; int age; // method void study(){ System.out.println("I'm fishing and learning!"); } void play(){ System.out.println("I was playing games! LOL Mobile Games!"); } // Constructor: the object used to create this class. The construction method without parameters can be automatically created by the system. SxtStu(){ } public static void main(String[] args) { // Defines the object that creates a class SxtStu stu = new SxtStu(); // Object calls the play() method stu.play(); // Object calls the study() method stu.study(); } }
Code example: simulate students using computers to learn
- Constructor: the object used to create this class. The construction method without parameters can be automatically created by the system.
- For a class, there are generally three common members: attribute field, method and constructor. All three members can define zero or more.
// Simulate students using computers to learn class Computer { String brand; // Computer brand } public class SxtStu1 { // Define attributes (member variables) int year; String sname; int age; // Define an object of Computer class in SxtStu1 class Computer comp; // Definition method void study() { System.out.printf("I'm using%s This brand of computer fishing learning!%n",comp.brand); } // Constructor: the object used to create this class. The construction method without parameters can be automatically created by the system. SxtStu1(){ } public static void main(String[] args) { // Object defining SxtStu1 class SxtStu1 stu1 = new SxtStu1(); // stu1 object calls the property (member variable) of SxtStu1 class and assigns a value stu1.year = 2021; stu1.sname = "Ah jun"; stu1.age = 0; // Object that defines the Computer class Computer comp1 = new Computer(); // The Computer1 object calls the attribute (member variable) of the Computer class and assigns a value comp1.brand = "Shenzhou God of war"; // stu1 object calls comp class attribute (member variable) and assigns value to comp1 stu1.comp = comp1; // Object call method stu1.study(); } }
Execution results:
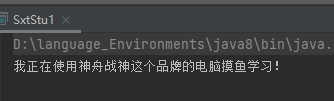
UML diagram example
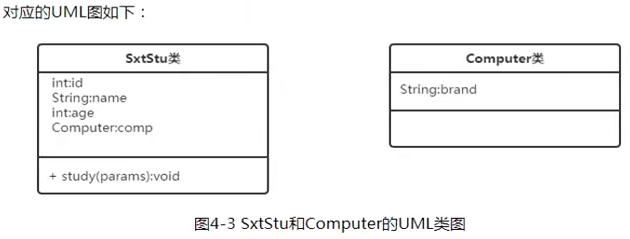