Article catalog
Author: Gorit
Date: 2021/10/24
Blog post published in 2021: 22 / 30
1, Builder mode
When we walk into a city, we can see many tall buildings. These tall buildings are called Build in English.
To build a building, we need to lay the foundation, build the frame, and then build it from bottom to top. Generally, it is difficult to cover these complex structures in one breath. So we're going to build the parts that make up this object. Then assemble them in stages.
This section will learn about the instance Builder pattern of a complex structure of components
2, Example
2.1 example implementation function
We will write a "document" editor using Builder mode. A document should contain the following structure
● contains a title ● content (string) ● items with entries (list)
The Builder class defines the method to determine the document structure, and then the Director class uses this method to write a specific document.
The Builder class is an abstract class, which only declares abstract methods. Its subclasses determine the processing of specific written documents ● TextBuilder class: use plain text (ordinary string) to write documents ● HTMLBuilder class: use HTML to write documents
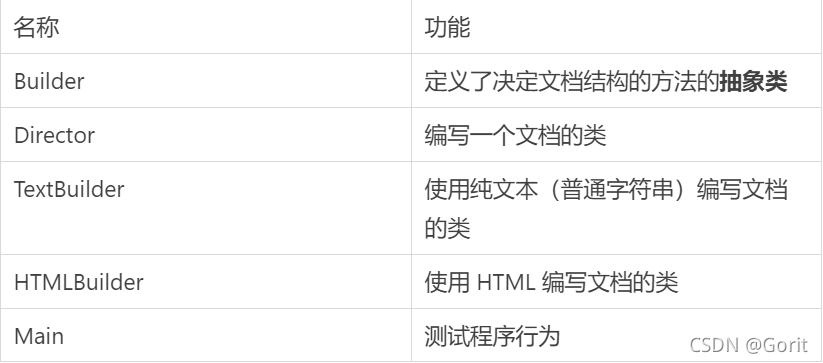
2.2 specific implementation
I have written the functions of each class into the code Builder class
package Builder; /** * An abstract class that declares a method for documenting * 1. makeTitle Write title * 2. makeString Write plain text * 3. makeTimes entry * 4. close How to complete documentation */ public abstract class Builder { public abstract void makeTtile(String title); public abstract void makeString(String str); public abstract void makeTimes(String[] items); public abstract void close(); } Director package Builder; /** * Use the methods declared in the Builder class to document */ public class Director { private Builder builder; public Director (Builder builder) { // A subclass of the Builder class is accepted this.builder = builder; // Save the subclass to the builder field } public void construct() { // Document builder.makeTtile("Greeting"); // title builder.makeString("From morning to afternoon"); // character string builder.makeTimes(new String[] { // entry "good morning", "Good afternoon" }); builder.makeString("night"); builder.makeTimes(new String[] { "Good evening", "good night", "bye" }); builder.close(); } }
TextBuilder
package Builder; /** * Writing documents in plain text */ public class TextBuilder extends Builder{ private StringBuffer buffer = new StringBuffer(); // The document content is saved in this field public void makeTtile(String title) { buffer.append("============================="); // Decorative line buffer.append("[" + title + "]\n"); buffer.append("\n"); } public void makeString(String str) { // Plain text string buffer.append('■' + str + '\n'); buffer.append('\n'); } public void makeTimes(String[] items) { for (int i = 0; i < items.length; i++) { buffer.append(" '"+ items[i] + "\n"); } buffer.append("\n"); } public void close() { buffer.append("============================="); } public String getResult() { // Edit completed document return buffer.toString(); // Convert StringBuffer to String } }
HTMLBuilder
package Builder; import java.io.FileWriter; import java.io.IOException; import java.io.PrintWriter; /** * Writing documents using HTML */ public class HTMLBuilder extends Builder{ private String filename; private PrintWriter writer; public void makeTtile(String title) { filename = title + ".html"; try { writer = new PrintWriter(new FileWriter(filename)); } catch (IOException e) { e.printStackTrace(); } writer.println(""</span> <span class="token operator">+</span> title <span class="token operator">+</span> <span class="token string">""); writer.println("" + title + "");// Output title } public void makeString(String str) { // String of the document in HTML writer.println("" + str + ""); // Use label output } public void makeTimes(String[] items) { // Output using ul and li Tags writer.println(""); for (int i = 0; i < items.length; i++) { writer.println("" + items[i] + ""); } writer.println(""); } public void close() { // Document complete writer.println(""); writer.close(); } public String getResult () { // Written document return filename; // Returns the file name } }
Main
package Builder; /** * The actual documentation is the Builder class */ public class Main { public static void main(String[] args) { // TextBuilder textBuilder = new TextBuilder(); // Director director = new Director(textBuilder); // director.construct(); // String result = textBuilder.getResult(); // System.out.println(result); HTMLBuilder htmlBuilder = new HTMLBuilder(); Director director = new Director(htmlBuilder); director.construct(); String result = htmlBuilder.getResult(); System.out.println(result); } }
2.3 operation results
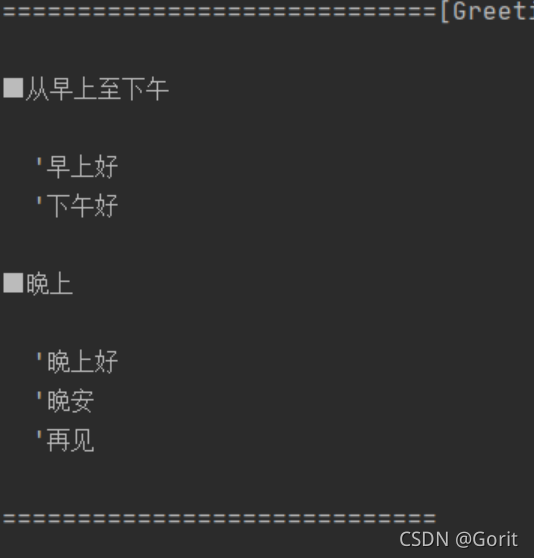
The second will generate the following HTML file
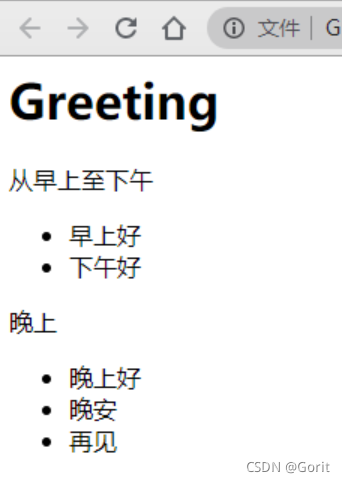
3, Roles in Builder mode
● Builder The Builder role is responsible for defining the API interface (API) used to generate the instance. The Builder role prepares the methods used to generate the instance.
● ConcreteBuilder (specific builder) ConcreteBuilder is a class (API) used to implement the interface of the Builder role. This defines the method actually called when generating an instance. At the same time, the method to obtain the final generated result is also defined in this class. The TextBuilder class and HTMLBuilder class in the sample program play this role
● Director (supervisor) The Director role is responsible for generating instances using the Builder role's interface (API). It does not depend on the ConcreteBuilder role. It only calls the methods defined in the Builder class
● Client (user) Main represents the role, using the Builder mode