Hello, I'm an advanced Java developer.
preface
This article mainly studies the BufferedInputStream class method, uses the BufferedInputStream class to read the content of the text file, and the BufferedOutputStream class to write the content and its common methods to the file. Next, let's learn together!
1, BufferedInputStream class method
1.BufferedInputStream is a buffered input stream, which can reduce the number of disk accesses and improve file reading performance. It is a subclass of FilterInputStream class.
2.BufferedInputStream class methods include:
(1)int available() method: used to return the number of unread bytes available in the input stream without blocking due to the next call to this InputStream method.
(2)void close() method: close this input stream and release all system resources associated with the stream.
(3)void mark(int readlimit) method: mark the current position of the input stream. The readlimit parameter is the number of bytes that the input stream is allowed to read before the mark position expires.
(4)boolean markSupported() method: test whether the input stream supports mark and reset methods.
(5)int read() method: reads a byte.
(6)int read(byte[] b, int off, int len) method: read multiple bytes into byte array b. parameter off is the array offset and parameter len is the length of the read data.
(7)void reset() method: resets the current position of the stream to the previously marked position.
(8)long skip(long n) method: skip the data in the stream. If the data is insufficient, skip the only bytes and return the number of skipped bytes.
2, BufferedInputStream class read(byte[] b, int off, int len) method
1.public int read(byte[] b, int off, int len) method: read multiple bytes into byte array b. parameter off is the array offset and parameter len is the length of the read data.
2. Implementation of read (byte [] B, int off, int len) method example:
(1) Create a test in the text folder Txt file and write "helloworld,java!" Content.
(2) Establish the input stream BufferedInputStream with a buffer size of 8 and read the first 5 bytes of the byte stream.
public class P09 { public static void main(String[] args) throws Exception { // TODO Auto-generated method stub //Create an input stream with a buffer BufferedInputStream in = new BufferedInputStream(new FileInputStream("text/test"), 8); //Read 5 bytes from byte stream byte temp[]=new byte[5]; //read(byte[] b, int off, int len) method in.read(temp,0,5); System.out.println("The first 5 bytes of the byte stream are:"+new String(temp)); } }
The running results are shown in the following figure:

3, BufferedInputStream class mark() and reset() methods
1.void mark(int readlimit) method: mark the current position of the input stream. The readlimit parameter is the number of bytes that the input stream is allowed to read before the mark position expires.
2.void reset() method: reset the current position of the stream to the position marked earlier.
3. Example implementation:
import java.io.*; public class P09 { public static void main(String[] args) throws Exception { // TODO Auto-generated method stub //Create an input stream with a buffer BufferedInputStream in = new BufferedInputStream(new FileInputStream("text/test"), 8); //Read 5 bytes from byte stream byte temp[]=new byte[5]; //read(byte[] b, int off, int len) method in.read(temp,0,5); System.out.println("The first 5 bytes of the byte stream are:"+new String(temp)); //Marking test in.mark(6); in.read(temp,0,5); System.out.println("The 6th to 10th bytes of the byte stream are:"+new String(temp)); //reset() method in.reset(); System.out.printf("reset The first byte read after is:%c", in.read()); } }
The running results are shown in the following figure:

4, BufferedOutputStream class
1.BufferedOutputStream class is a byte buffered output stream, which is a subclass of FilterOutputStream class.
2. The common methods of bufferedoutputstream class are as follows:
(1)void write(int b) method: write one byte at a time.
(2)void write(byte[] b,int off,int len) method: write len bytes from the offset off in the specified array b to the file output stream. The off parameter indicates the array offset, and Len indicates the number of bytes to be written.
(3)void flush() method: flushes the buffered output stream. This forces all buffered output bytes to be written out to the underlying output stream.
(4)void close() method: close this input stream and release all system resources associated with the stream.
3. Implementation example of bufferedoutputstream method:
import java.io.*; public class P10 { public static void main(String[] args) throws Exception { // TODO Auto-generated method stub //Create an output stream with a buffered stream BufferedOutputStream bos=new BufferedOutputStream(new FileOutputStream("text/test10")); //Write lowercase a letters to a text file bos.write(97); //Write "Java advanced learning exchange" in the text file bos.write("\nJava Advanced learning exchange\n".getBytes()); //Create a byte array byte[] bytes = {97,98,99,100,101}; //Starting from the offset 2 position is c, and the number of bytes written is obtained bos.write(bytes,2,2); //Flush buffer stream bos.flush(); //Close flow bos.close(); } }
The results of the operation are as follows:
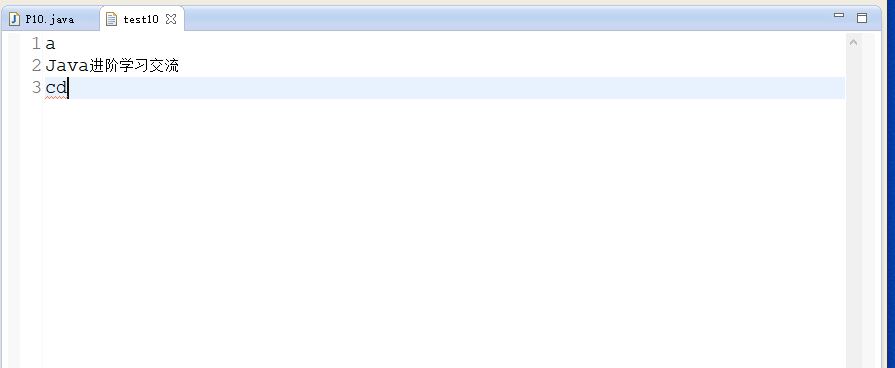
5, Summary
This article mainly introduces the BufferedInputStream class method and BufferedOutputStream class. This paper introduces the read(byte[] b, int off, int len) method, mark() and reset() method of BufferedInputStream, understands the usage of these methods through examples, and uses BufferedInputStream to read the content of text. BufferedOutputStream class is a byte buffered output stream, which is a subclass of FilterOutputStream class. BufferedOutputStream to write the contents of the text. I hope you can help you through the study of this article!