1. Introduction
webdriver has three methods to judge the status of elements: isEnabled, isSelected and isDisplayed. isSelected has been briefly introduced in the previous content. isSelected means to check whether an element is selected. It is generally used in the check box (multi-choice or single choice). isDisplayed means to check what?
2.isDisplayed() source code
/** * Is this element displayed or not? This method avoids the problem of having to parse an * element's "style" attribute. * * @return Whether or not the element is displayed */ boolean isDisplayed();
As can be seen from the comments in the source code above, the isDisplay() method is used to determine whether page elements are displayed on the page. true is returned if it exists, false is returned if it does not exist.
3.isDisplay() usage
List<WebElement> targetElement = driver.findElements(By.xpath("xpath_your_expected_element")); try { if(targetElement>=1) { if(targetElement.isDisplayed()) { System.out.println("Element is present"); }else { System.out.println("Element is found, but hidden on the page"); } }else { System.out.println("Element not found on the page"); } }catch (NoSuchElementException e) { System.out.println("Exception in finding the element:" + e.getMessage()); }
4. Project practice
In Web driver automated testing, we often need to judge a scenario. For example, after we do some operations, we will trigger some reminders, some are correct reminders, and some are error reminders displayed in red font. How do we capture these fields in automation? What if we make test automation judgment. The isDisplay() method will be used here. Brother Hong uses the home page login example of Du Niang to judge whether the field "please fill in the verification code" appears.
4.1 test cases (Ideas)
1. Visit home page
2. Locate the login button on the home page and click
3. Pop up the login box, locate the SMS login button, and then click
4. Locate the mobile phone number input box, and then enter the mobile phone number
5. Locate the login button in the login box and click
6. Locate the "please fill in the verification code" and judge.
4.2 code design
The code is designed according to the test cases as follows:
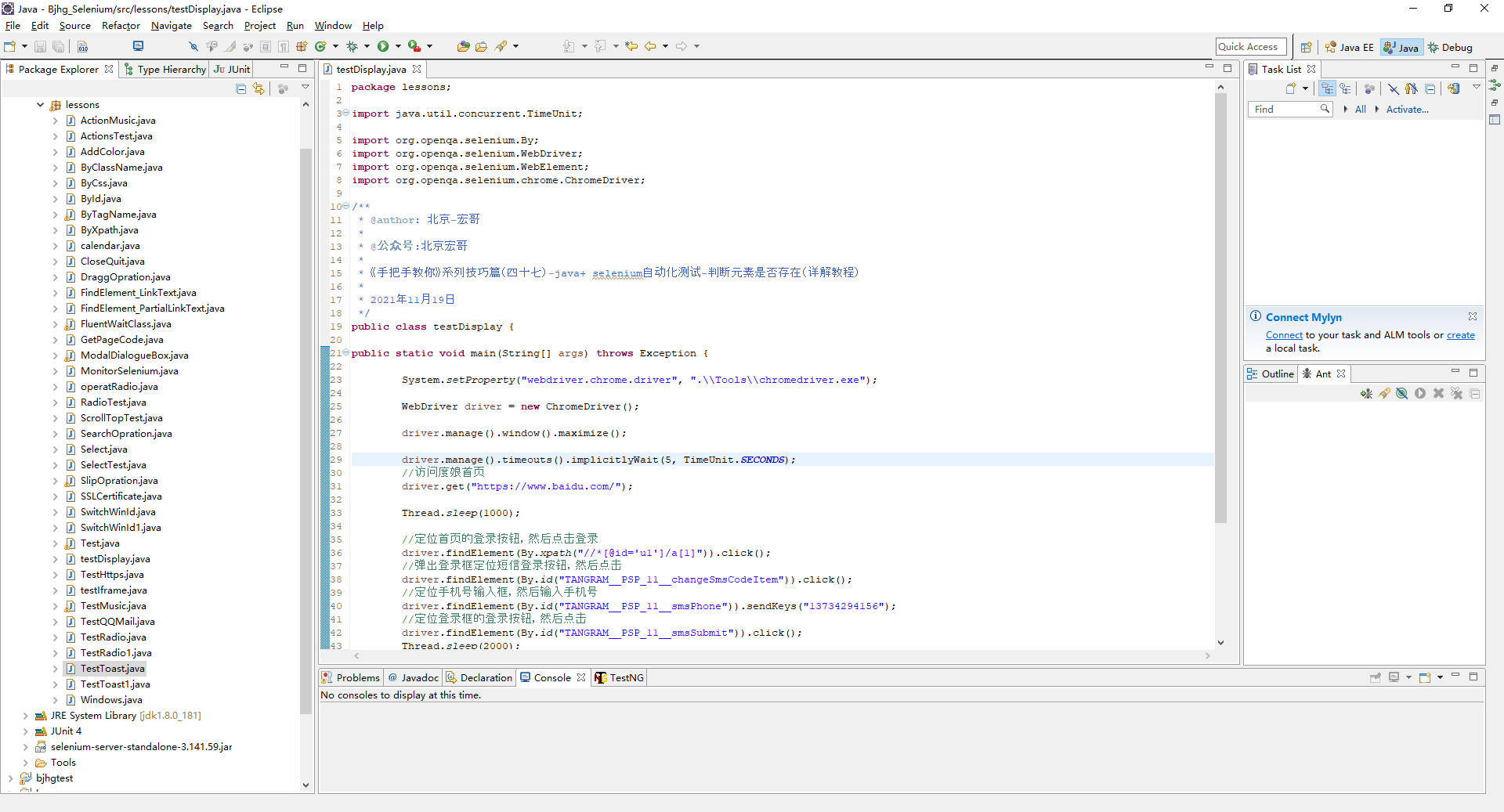
4.3 reference code
package lessons; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; /** * @author: Beijing - Hongge * * @Official account: Beijing, Hong brother * * <Teach you by hand series tips (47) - java+ selenium automated test - judge whether elements exist (detailed tutorial) * * 2021 November 19 */ public class testDisplay { public static void main(String[] args) throws Exception { System.setProperty("webdriver.chrome.driver", ".\\Tools\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //Visit Du Niang's home page driver.get("https://www.baidu.com/"); Thread.sleep(1000); //Locate the login button on the home page and click login driver.findElement(By.xpath("//*[@id='u1']/a[1]")).click(); //Pop up the login box, locate the SMS login button, and then click driver.findElement(By.id("TANGRAM__PSP_11__changeSmsCodeItem")).click(); //Locate the cell phone number input box and enter the cell phone number driver.findElement(By.id("TANGRAM__PSP_11__smsPhone")).sendKeys("13734294156"); //Locate the login button in the login box and click driver.findElement(By.id("TANGRAM__PSP_11__smsSubmit")).click(); Thread.sleep(2000); // Method 1 WebElement error_message = driver.findElement(By.xpath("//*[@ id ='tangram_psp_11_smserror 'and text() =' please fill in the verification code '] "); if(error_message.isDisplayed()){ System.out.println("Brother Hong! Element exists"); }else{ System.out.println("Hongge! Element does not exist"); } driver.quit(); } }
4.4 operation code
1. Run the code, right-click run as - > java appliance, and the console will output, as shown in the following figure:
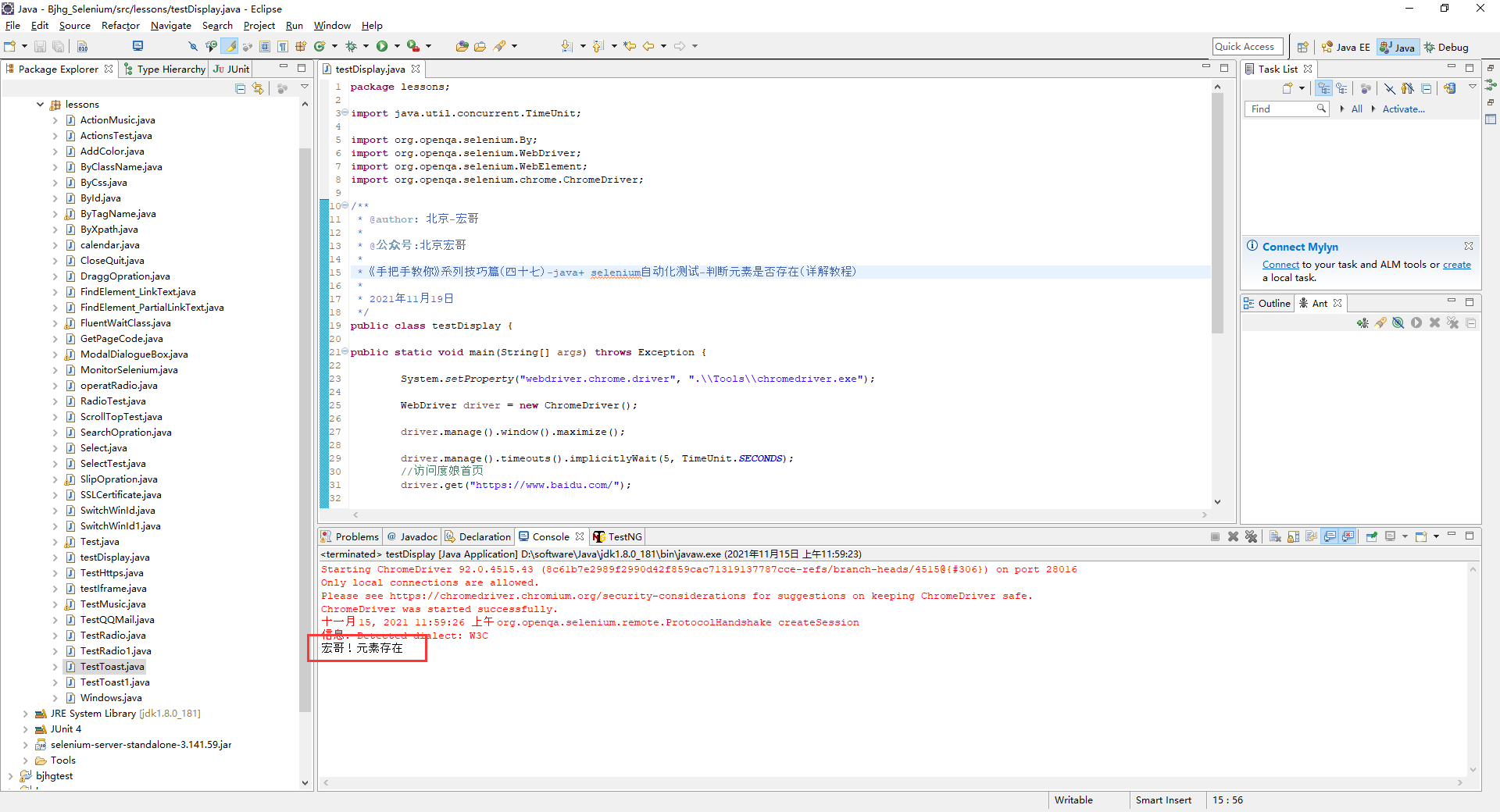
2. After running the code, the action of the browser on the computer side is shown in the following small video:
5. Method 2
The second method is to first get the String, save it with a String variable, and then compare the two strings. In fact, this method has been used before, but brother Hong didn't point out that, just like the toast element in the previous article, directly locate and store it in the variable, and then print out its text. Are you friends or children's shoes.
5.1 code design
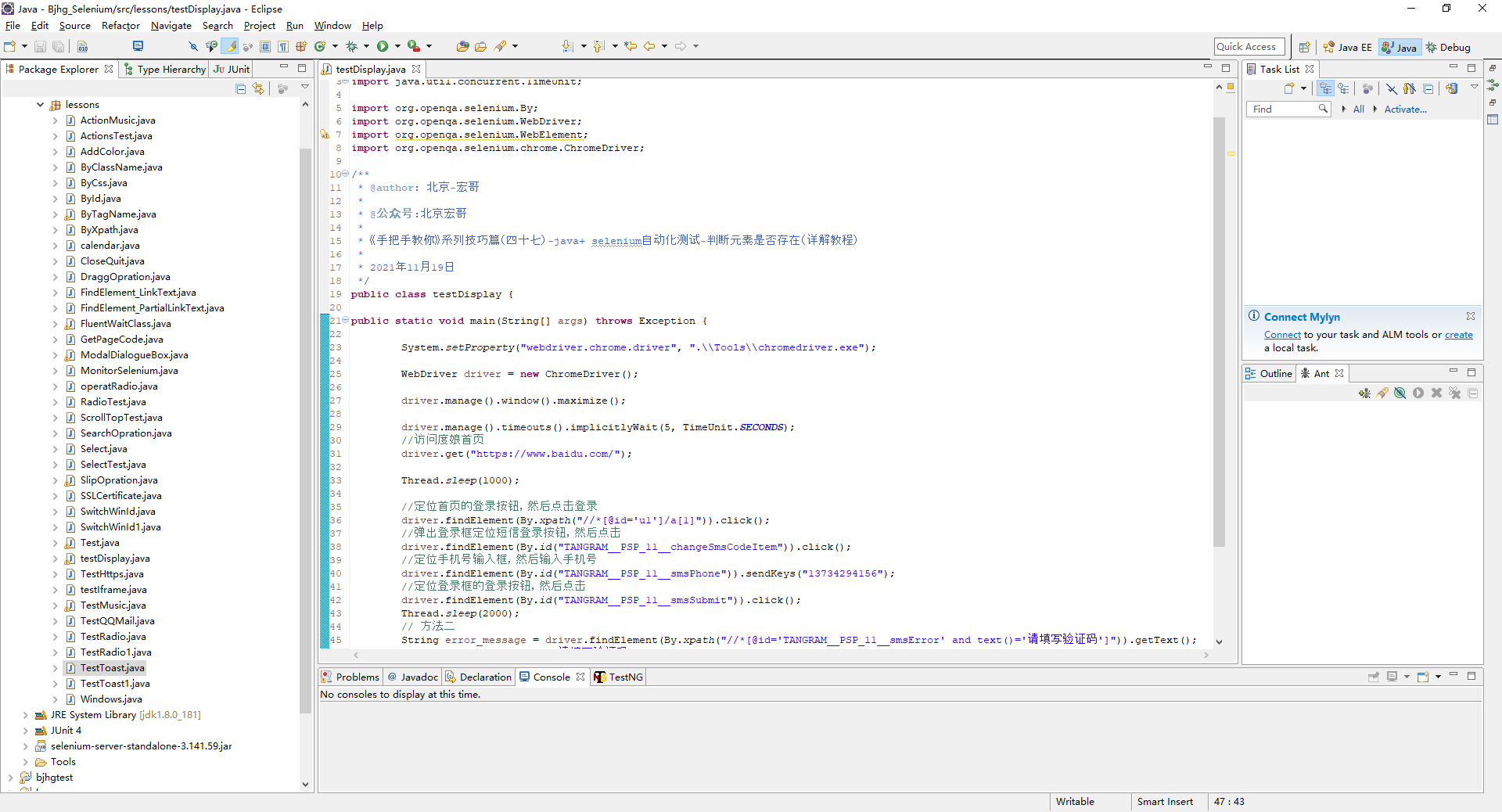
5.2 reference code
package lessons; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; /** * @author: Beijing - Hongge * * @Official account: Beijing, Hong brother * * <Teach you by hand series tips (47) - java+ selenium automated test - judge whether elements exist (detailed tutorial) * * 2021 November 19 */ public class testDisplay { public static void main(String[] args) throws Exception { System.setProperty("webdriver.chrome.driver", ".\\Tools\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //Visit Du Niang's home page driver.get("https://www.baidu.com/"); Thread.sleep(1000); //Locate the login button on the home page and click login driver.findElement(By.xpath("//*[@id='u1']/a[1]")).click(); //Pop up the login box, locate the SMS login button, and then click driver.findElement(By.id("TANGRAM__PSP_11__changeSmsCodeItem")).click(); //Locate the cell phone number input box and enter the cell phone number driver.findElement(By.id("TANGRAM__PSP_11__smsPhone")).sendKeys("13734294156"); //Locate the login button in the login box and click driver.findElement(By.id("TANGRAM__PSP_11__smsSubmit")).click(); Thread.sleep(2000); // Method 2 String error_message = driver.findElement(By.xpath("//*[@ id ='tangram_psp_11_smserror 'and text() =' please fill in the verification code '] ") getText(); if(error_message.equals("Please fill in the verification code")){ System.out.println("Hiro! Element exists"); }else{ System.out.println("Hiro! Element does not exist"); } driver.quit(); } }
5.3 operation code
1. Run the code, right-click run as - > java appliance, and the console will output, as shown in the following figure:
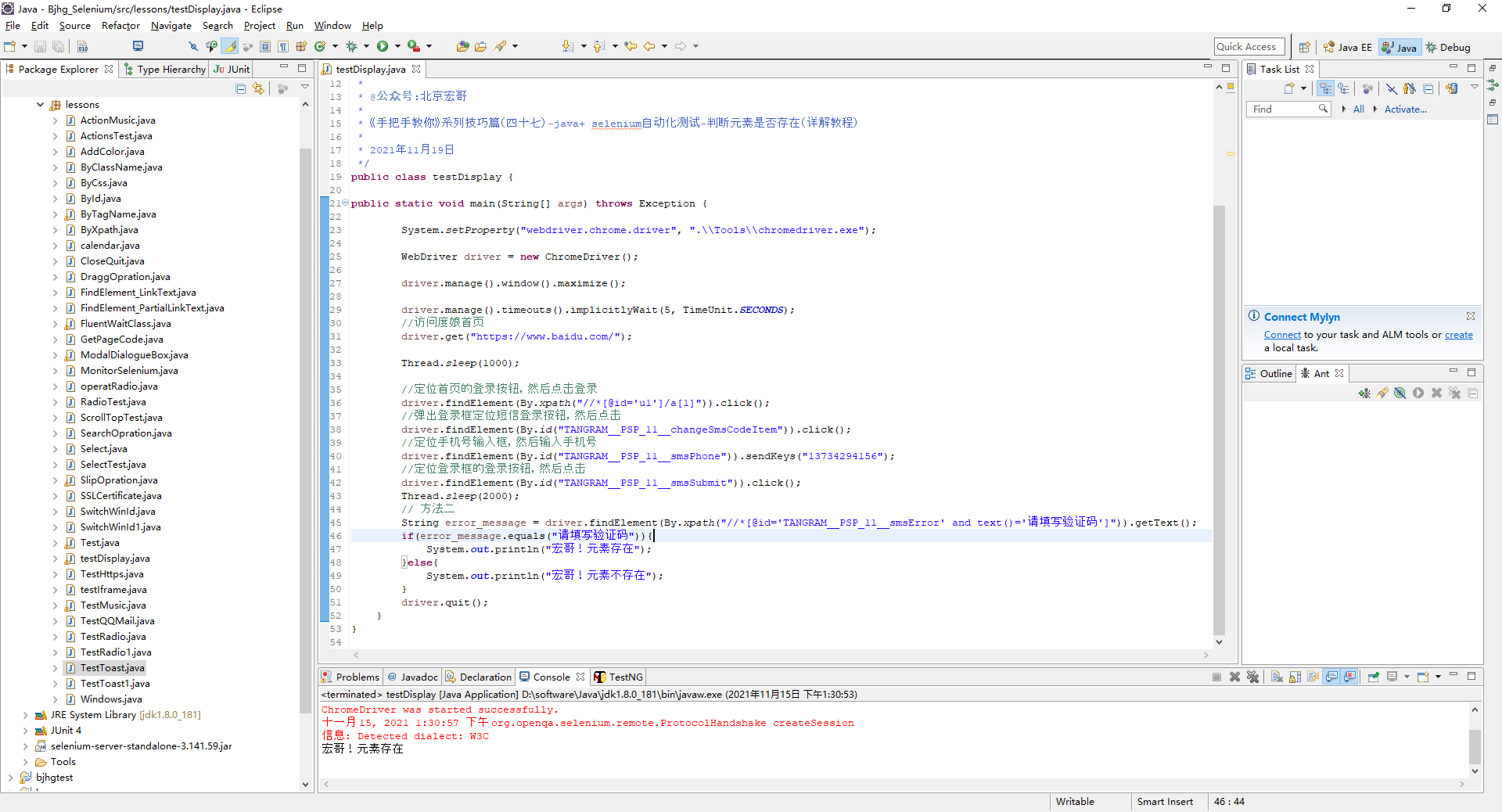
2. After running the code, the action of the browser on the computer side is shown in the following small video:
6. Summary
1.isDisplayed() function is used to determine whether an element exists on the page (the existence here is not visible to the naked eye, but the existence of HTML code. In some cases, the visibility of the element is hidden or the display attribute is none. We can't see it on the page, but there are actually some elements of the HTML page).
2. Use equals() and = =. The difference is that equals compares whether the contents are equal and = = compares whether the referenced variable addresses are equal.