P233 data structure
- Stack: data entering the stack model is called pressing stack, and data leaving the stack model is elastic stack. Stack is a last in first out model.
- Queue: the process of data entering the queue model from the back end is called entering the queue, and the process of leaving the queue is called leaving the queue. Queues are first in first out models.
- Array: query array is located by index. It takes the same time to query any data and has high query efficiency. When deleting data, the original data should be deleted, and each subsequent data should be moved forward, which has low deletion efficiency. Adding elements is the same. Each data after adding is moved back, and then adding elements is inefficient. Data is a model of fast query and slow addition and deletion.
- Linked list: linked list is a model with fast addition and deletion and slow query.
P235 characteristics of list set subclass
- ArrayList. The underlying data structure is array. The query is fast and the increase or decrease is slow
- LinkedList, the underlying data structure is link, which increases or decreases quickly and queries slowly
The unique method of linklist
P238 set overview and features
public static void main(String[] args) { Set<String> set = new HashSet<String>(); // The iteration sequence is not guaranteed set.add("hello"); set.add("world"); set.add("world"); // Does not contain duplicate elements set.add("java"); for(String s :set) System.out.println(s); }
P239 hash value! [here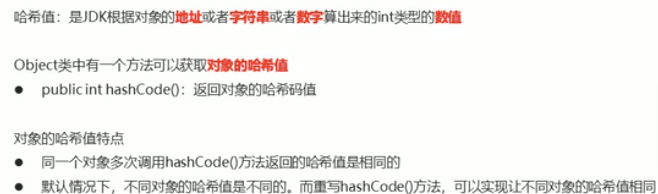
Insert picture description]( https://img-blog.csdnimg.cn/47cda787bed04956b359fb1b8f974434.png)
P240 HashSet set overview and features
P244 LinkedHashSet set overview and features
P245 TreeSet set overview and features
P246 natural sorting
That is, let the class implement the Comparable interface and override the compareTo(T o) method.
public class Student implements Comparable<Student>{ private String name; private int age; public Student(){ System.out.println("Nonparametric construction method"); } public Student(String name,int age){ this.name = name; this.age = age; System.out.println("Residual structure method"); } public void setName(String nanme) { this.name = name; } public String getName(){ return name; } public int getAge(){return age;} public void setAge(int a) { age = a; } public void show(){ System.out.println(name+"_" + age); } @Override public int compareTo(Student s) { int num = this.getAge() - s.getAge(); int num2 = num == 0?this.getName().compareTo(s.getName()):num; return num2; } }
P247 use of comparator
TreeSet<Student> ts = new TreeSet<Student>(new Comparator<Student>() { @Override public int compare(Student o1, Student o2) { int num = o1.getAge() - o2.getAge(); int num2 = num==0?o1.getName().compareTo(o2.getName()):num; return num2; } });
P250 generic overview
If no type is passed in, it defaults to the type of Object.
P251 generic class
public class Generic<T> { private T t; public T getT(){ return t; } public void setT(T t) { this.t = t; } }
Main function use
Generic<Integer> g2 = new Generic<Integer>(); g2.setT(30); System.out.println(g2.getT());
P252 generic method
for instance
public <T> void show(T t){ System.out.println(t); }
P253 generic interface
// Interface public interface GenericInterface<T>{ void show(T t); } //Interface implementation public class GenericInterfaceImpl<T> implements GenericInterface<T>{ @Override public void show(T t) { System.out.println(t); } } //Instance object in test case GenericInterface<String> gg = new GenericInterfaceImpl<String>(); gg.show("hello");
P254 type wildcard
// The following line of code reports an error because its upper limit is Number, so it cannot be the parent of Number //List<? extends Number> list4 = new ArrayList<Object>(); List<? extends Number> list5 = new ArrayList<Number>(); List<? extends Number> list6 = new ArrayList<Integer>(); // Limit lower limit List<? super Number> list7 = new ArrayList<Object>(); List<? super Number> list8 = new ArrayList<Number>(); // The following line of code reports an error. Anyway, it is an error //List<?super Number> list9 = new ArrayList<Integer>();
255 variable parameters
The last line actually means:
sum(int b,int... a);
//a is actually an array: call the method sum(10,20,30,40); public static int sum(int... a){ int sum = 0; for(int i:a) sum+=i; return sum; }
P256 use of variable parameters
List<String> list = Arrays.asList("hello","world","java"); //Cannot add or delete // list.add("jaa"); // list.remove("world"); list.set(0,"ss"); List<String> list2 = List.of("hello","world","java"); // Comments cannot be executed. They cannot be added, deleted or modified // list2.add("jaksdf"); //list2.remove("asdf"); //list.set(0,"ssa"); Set<String> set = Set.of("hello","world","java"); // Also, you cannot add remove
P257 Map
public static void main(String[] args) { Map<String,String> map = new HashMap<String,String>(); map.put("aaa","111"); map.put("bbb","222"); map.put("ccc","333"); System.out.println(map); System.out.println(map.remove("aaa")); System.out.println(map.containsKey("bbb")); System.out.println(map.isEmpty()); System.out.println(map.size()); map.clear(); System.out.println(map); }
Get function of Map collection
P260Map traversal method
//1. Get the collection of all keys Set<String> keySet = map.keySet(); for(String key:keySet){ String value = map.get(key); System.out.println(key+","+value); } //2. Traversal mode 2 Set<Map.Entry<String,String>> entrySet = map.entrySet(); for(Map.Entry<String,String> me :entrySet){ String key = me.getKey(); String value = me.getValue(); System.out.println(key+","+value); }
P267 Collections overview and usage
Collection is the top-level structure of a single column collection, and collections is a concrete class
List<Integer> list = new ArrayList<Integer>(); list.add(30); list.add(20); list.add(10); list.add(40); list.add(50); Collections.sort(list); Collections.reverse(list); //reversal Collections.shuffle(list); // Random permutation is often used to simulate shuffling
Sort classes
Sort by using the specified comparator.
List<Student> array = new ArrayList<Student>(); Collections.sort(array, new Comparator<Student>() { @Override public int compare(Student o1, Student o2) { int num = o1.getAge() - o2.getAge(); int num2 = num==0?o1.getName().compareTo(o2.getName()):num; return num2; } });
P272 File class overview and construction method
File class creation function
File class judgment and acquisition function
File f1 = new File("/home/usr/Code/JavaCode/test.txt"); System.out.println(f1); File f2 = new File("/home/usr/Code/JavaCode","test.txt"); System.out.println(f2); File f3 = new File("/home/usr/Code/JavaCode"); File f4 = new File(f3, "text.txt"); System.out.println(f4); //create a file public static void main(String[] args) throws IOException { File f1 = new File("/home/usr/Code/JavaCode/test1.txt"); System.out.println(f1.createNewFile());//If the file does not exist, create the file and return true; otherwise, return false File f2 = new File("/home/usr/Code/JavaCode/mkdirtest"); System.out.println(f2.mkdir());//If the directory does not exist, create a file and return true; otherwise, return false System.out.println(f2); File f3 = new File("/home/usr/Code/JavaCode/mkdirtest1/mkdirtest2/mkdirtest22"); System.out.println(f3.mkdirs()); File f4 = new File(f3, "text.txt"); System.out.println(f4); System.out.println(f1.mkdir()); // At this time, a directory will be created, even if the file name is test1 txt } //Common functions of File class File f1 = new File("test3.txt"); System.out.println(f1.createNewFile()); System.out.println(f1.isDirectory()); System.out.println(f1.isFile()); System.out.println(f1.exists()); System.out.println(f1.getAbsoluteFile()); System.out.println(f1.getPath()); System.out.println(f1.getName()); File f2 = new File("idea_test/src/ArraysPkg"); String[] strArry = f2.list(); for(String str:strArry){ System.out.println(str); } File[] f3 = f2.listFiles(); for(File file:f3){ System.out.println(file);//Absolute path output } //Delete file File f1 = new File("test1.txt"); System.out.println(f1.createNewFile()); System.out.println(f1.delete()); // Create directory File f2 = new File("test"); //System.out.println(f2.mkdir()); File f3 = new File("test/test1.txt"); //System.out.println(f3.createNewFile()); // If there is content in the directory to be deleted, you should delete the content first, and then delete the directory System.out.println(f2.delete());
Recursively traversing file names
public static void main(String[] args) { File srcFile = new File("idea_test"); getAllFilePath(srcFile); } public static void getAllFilePath(File srcFile){ File[] fileArray = srcFile.listFiles(); if(fileArray !=null){ for(File file:fileArray){ if(file.isDirectory()){ getAllFilePath(file); } else{ System.out.println(file.getAbsolutePath()); } } } }
P279 IO stream overview and classification
Byte stream write data
// Create byte output stream object: 1 Calling system functions to create files; 2. Create byte output stream object; 3. Make the byte output stream object point to the file FileOutputStream f1 = new FileOutputStream("test.txt"); f1.write(95); f1.close(); // release
Three forms of byte stream write data, additional write and line feed
public static void main(String[] args) throws IOException { // The second parameter is true, which allows each write of the file to be written to the end instead of the beginning FileOutputStream f1 = new FileOutputStream("test2.txt",true); f1.write(97); f1.write(98); f1.write(99); f1.write(100); f1.write(101); byte[] bys = {97,98,99,100,101,102,103}; byte[] bys2 = "abcde".getBytes(StandardCharsets.UTF_8); f1.write(bys); f1.write(bys2,0,3);//Starting from 0, write data with length of 3 f1.write("\n".getBytes(StandardCharsets.UTF_8)); // Line feed is required under windows \ r\n f1.close(); }
Byte stream data exception handling
public static void main(String[] args) { FileOutputStream f1 = null; try { // Create byte output stream object: 1 Calling system functions to create files; 2. Create byte output stream object; 3. Make the byte output stream object point to the file f1 = new FileOutputStream("test.txt"); f1.write(95); f1.close(); // release }catch (IOException e){ e.printStackTrace();; } finally { if(f1 !=null){ // z execute the clear operation to prevent the write exception from exiting successfully try{ f1.close(); }catch (IOException e){ e.printStackTrace(); } }}
Byte read data (single byte read and cyclic read)
FileInputStream f1 = new FileInputStream("test2.txt"); int by = f1.read(); System.out.println(by); System.out.println((char)by); //If the end of the file is reached, output - 1 while((by =f1.read())!=-1){ System.out.println((char)by); } f1.close();
Byte read input 2
FileInputStream f1 = new FileInputStream("test2.txt"); byte[] bys = new byte[5]; // Read 5 data at a time, F1 Read() returns the number of actual reads int len = f1.read(bys); System.out.println(len); System.out.println(new String(bys,0,len)); // Cyclic reading byte[] bys1 = new byte[1024]; int len2; while ((len2=f1.read(bys))!=-1){ System.out.println(new String(bys,0,len2)); } f1.close();
Byte buffer stream read / write data
// FileOutputStream fos = FileOutputStream("test2.txt"); BufferedOutputStream bos= new BufferedOutputStream(new FileOutputStream("test2.txt")); //write data bos.write("hello".getBytes(StandardCharsets.UTF_8)); bos.write("world\r\n".getBytes(StandardCharsets.UTF_8)); bos.close(); BufferedInputStream bis = new BufferedInputStream(new FileInputStream("test2.txt")); int by; while((by=bis.read())!=-1){ System.out.println((char)by); } //The second way byte[] bys = new byte[1024]; int len; while((len=bis.read(bys))!=-1){ System.out.println(new String(bys,0,len)); } bis.close();
P290 why does character stream appear
P291 coding table and GBK
P292 decoding problem in string
String s = "China"; byte[] bys = s.getBytes(StandardCharsets.UTF_8); // [-28, -72, -83, -27, -101, -67] byte[] bys2 = s.getBytes("GBK"); // [-42, -48, -71, -6] System.out.println(Arrays.toString(bys2)); String ss = new String(bys); // decode System.out.println(ss);
Encoding and decoding problems in P293 character stream
Note that the encoding of read and write streams should be the same.
FileOutputStream fos = new FileOutputStream("test.txt"); OutputStreamWriter osw = new OutputStreamWriter(fos,"UTF-8"); osw.write("China!!!!!!!!"); osw.close(); InputStreamReader isr = new InputStreamReader(new FileInputStream("test.txt"), "UTF-8"); int ch; while((ch=isr.read())!=-1){ System.out.println((char)ch); } isr.close();
Five ways of writing data in character stream
OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("test.txt")); osw.write(97); // osw.flush(); //The refresh stream is refreshed to the file, or when the close is completed, the close executes the refresh first and then close char[] chs = {'a','b','c','d','e'}; osw.write(chs); osw.write(chs,0,2); osw.write("hello"); osw.write("helllllllo",0,5); osw.close();
Two ways of reading data from character stream
It reads data in the same way as byte stream
InputStreamReader isr = new InputStreamReader(new FileInputStream("test.txt")); //way 1 int ch; while((ch=isr.read())!=-1){ System.out.println((char)ch); } //way 2 char[] chs=new char[1024]; int len; while((len=isr.read(chs))!=-1){ System.out.println(new String(chs,0,len)); } isr.close();
In P297, FileReader and FileWriter are also mentioned. No more..
P298 character buffer stream
FileWriter fw = new FileWriter("test.txt"); BufferedWriter bw = new BufferedWriter(fw); bw.write("hello\r\n"); bw.write("world"); bw.newLine(); bw.close(); BufferedReader br = new BufferedReader(new FileReader("test.txt")); String ss = br.readLine(); char[] chs = new char[1024]; int len; while((len=br.read(chs))!=-1){ System.out.println(new String(chs,0,len)); } br.close();
Unique function of character buffer stream
String ss = br.readLine(); // Read a line of read-only content, do not read newline symbols
bw.newLine(); // This is the best way to wrap lines, not \ r\n
A new reading method
String line; while((line=br.readLine())!=null){ System.out.println(line); }
P302 IO Flow Summary
P311 exception handling for copying files
P312 standard flow
in,out
InputStream is = System.in; /* int by; while((by=is.read())!=-1){ System.out.println((char)by); }*/ //The above method cannot read Chinese InputStreamReader isr = new InputStreamReader(is); BufferedReader br = new BufferedReader(isr); System.out.println("Please enter a string, including Chinese"); String line = br.readLine(); System.out.println("You entered:" + line); System.out.println("Please enter an integer:"); int i = Integer.parseInt(br.readLine()); System.out.println("The integer you entered is" + i);
out
PrintStream ps = System.out; ps.print("hello"); ps.print(100); ps.println("hello"); ps.print(100); ps.close();