#User table
create table user(
id int auto_increment primary key, username nvarchar(20) null, password nvarchar(20) null, name nvarchar(20) null, gender nchar(10) null, age int null, address nvarchar(20) null, phone nvarchar(11) unique null, email nvarchar(30) null
);
#Student list
create table student(
id int auto_increment primary key, name nvarchar(20) null, gender nvarchar(10) null, age int null, classno nvarchar(20) null, phone nvarchar(11) unique null, email nvarchar(30) null
);
#Class table
create table class(
id int auto_increment primary key, cno nvarchar(10) null, classname nvarchar(30) null, department nvarchar(30) null
);
#Curriculum
create table course(
id int auto_increment primary key, courseno nvarchar(20) null, coursename nvarchar(20) null, type nvarchar(8) null, period int null, credit double null
);
User information table: User 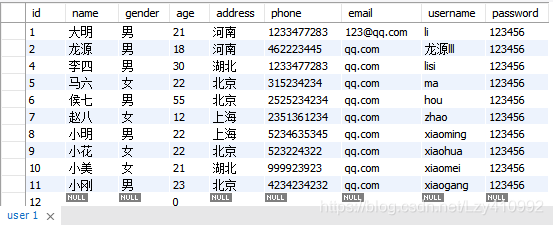 Student information sheet: Student 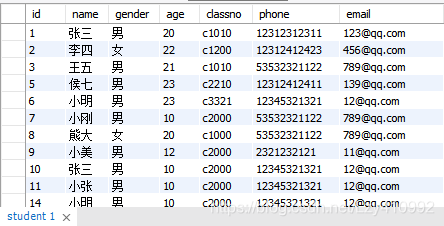 Class information table: Class 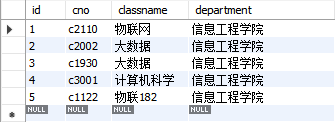 Course information sheet: Course 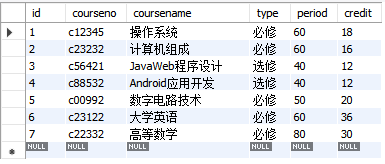 #### [] () function display: User login: 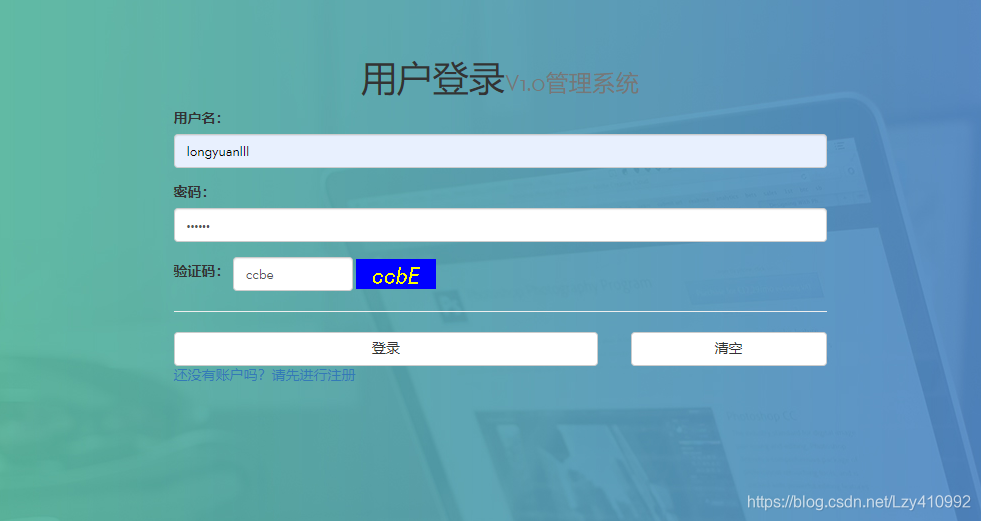 User registration: 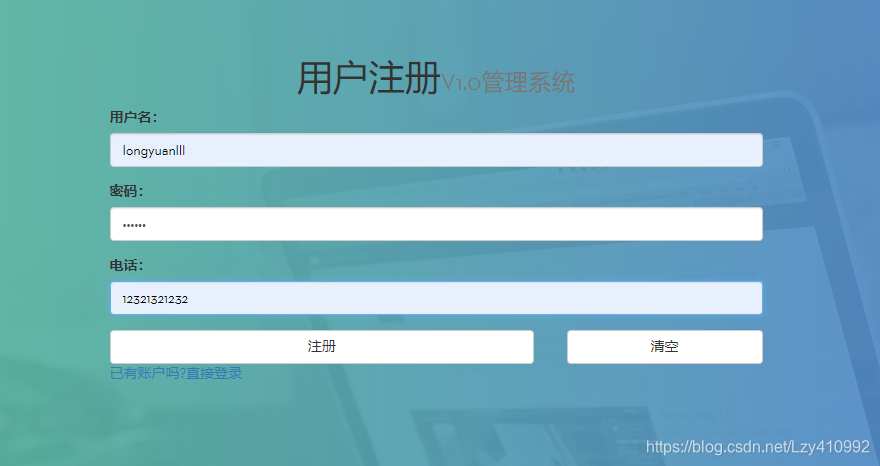 Login succeeded: 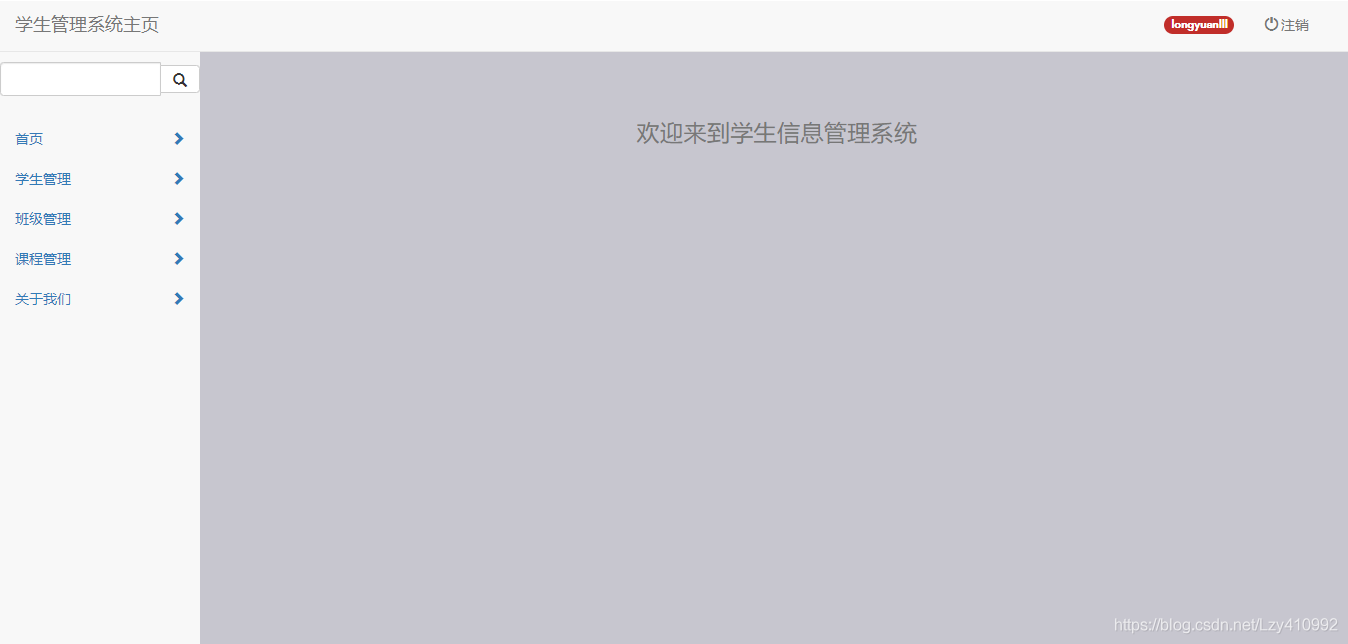 Click the user name in the upper left corner to improve your personal information: 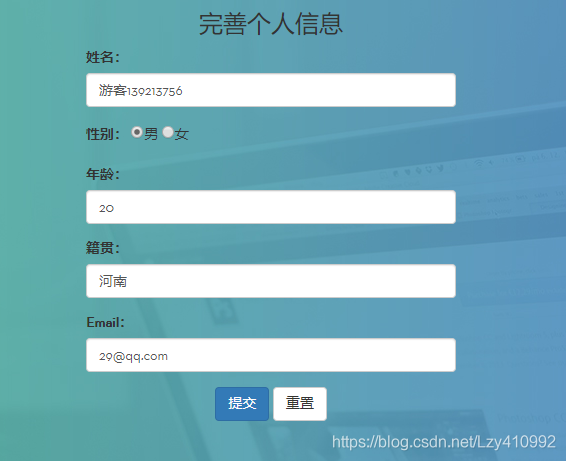 **The information management part realizes pagination display, addition, deletion, modification, query and batch deletion** Student information management: 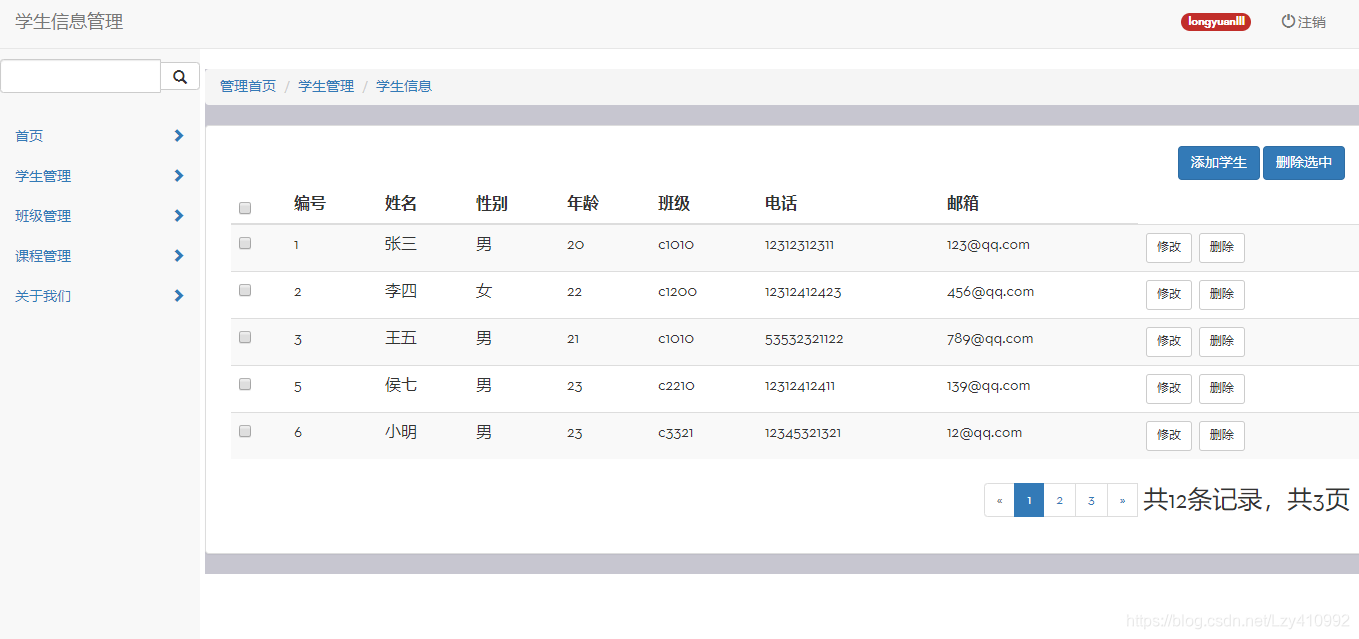 Class information management: 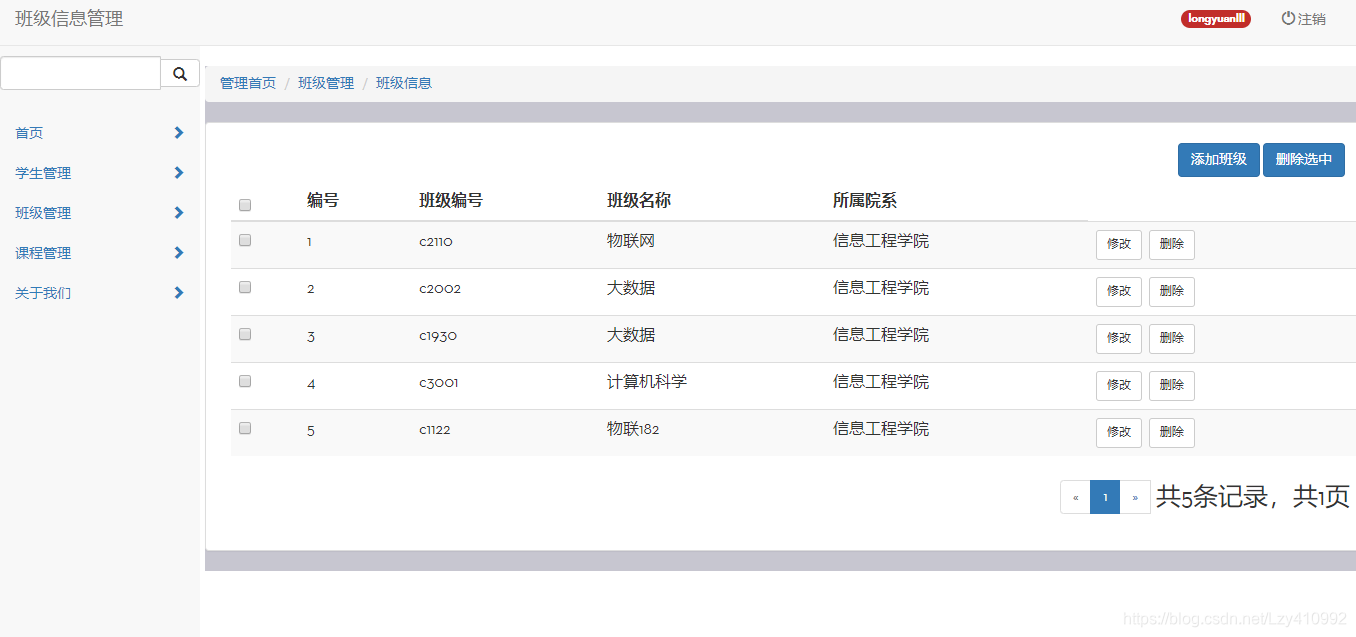 Course management: 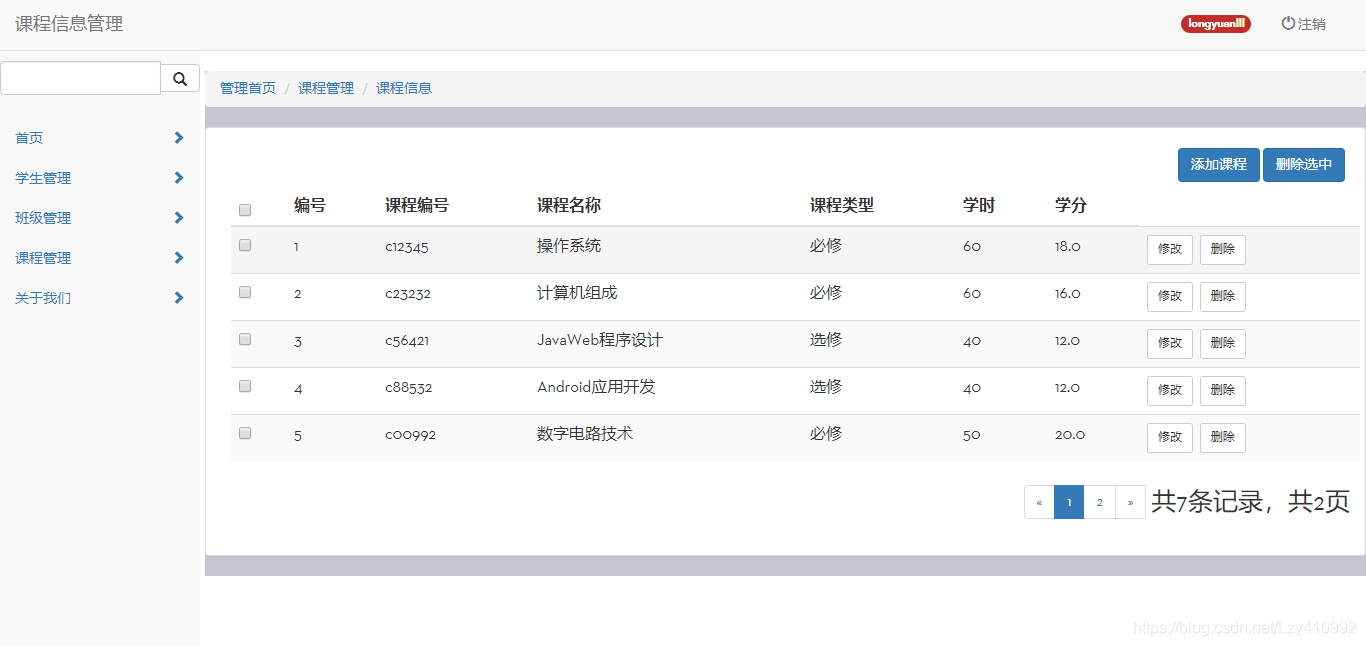 []( )Design steps ================================================================= There are many codes, and only the more important parts are shown: #### []( )1\. Database connection implementation The database connection part uses druid For database connection pool, first configure the database connection pool: druid.properties
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/mydb?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8
username=root
password=123456
initialSize=5
maxActive=10
maxWait=3000
Then write the database connection tool class:
/**
- The JDBC tool class uses the Durid connection pool
*/
public class JDBCUtils {
private static DataSource ds ; static { try { //1. Load configuration file Properties pro = new Properties(); //Use ClassLoader to load the configuration file and get the byte input stream InputStream is = JDBCUtils.class.getClassLoader().getResourceAsStream("druid.properties"); pro.load(is); //2. Initialize the connection pool object ds = DruidDataSourceFactory.createDataSource(pro); } catch (IOException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } } /** * Get connection pool object */ public static DataSource getDataSource(){ return ds; } /** * Get Connection object */ public static Connection getConnection() throws SQLException { return ds.getConnection(); }
}
Here we use JdbcTemplate To connect the database:
private JdbcTemplate template = new JdbcTemplate(JDBCUtils.getDataSource());
#### []( )2\. Implementation persistence layer (Dao) Write persistence layer interface:
/**
- User operated DAO
*/
public interface UserDao {
}
Implement persistence layer interface:
public class UserDaoImpl implements UserDao {
private JdbcTemplate template = new JdbcTemplate(JDBCUtils.getDataSource());
}
### []( )3\. Implement business layer (Service) Write business layer interface:
/**
- User managed service interface
*/
public interface UserService {
}
Implement business layer interface:
public class UserServiceImpl implements UserService {
private UserDao dao = new UserDaoImpl();
}
#### []( )4. Implementation of presentation layer functions Write presentation layer:
@WebServlet("/loginServlet")
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { /* * Code */ } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doPost(request, response); }
}
#### []( )5. Because there are too many presentation layer servlets, we can do simple extraction to write BaseServlet Class, and then by others servlet inherit
public class BaseServlet extends HttpServlet {
private static final long serialVersionUID = 1L; public void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { try { // Get request ID String methodName = request.getParameter("method"); // Gets the bytecode object of the specified class Class<? extends BaseServlet> clazz = this.getClass();//this here refers to inheriting the BaseServlet object // Get the bytecode object of the method through the bytecode object of the class Method method = clazz.getMethod(methodName, HttpServletRequest.class, HttpServletResponse.class); // Let the method execute method.invoke(this, request, response); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } }
}
#### []( )5. Write the corresponding front-end page: user\_login.jsp as an example
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<meta charset="utf-8"/> <meta http-equiv="X-UA-Compatible" content="IE=edge"/> <meta name="viewport" content="width=device-width, initial-scale=1"/> <title>Administrator login</title> <!-- 1. Import CSS Global style for --> <link href="css/bootstrap.min.css" rel="stylesheet"> <!-- 2. jQuery Import, 1 is recommended.9 Version above --> <script src="js/jquery-2.1.0.min.js"></script> <!-- 3. Import bootstrap of js file --> <script src="js/bootstrap.min.js"></script> <script type="text/javascript"> //Switching verification code function refreshCode(){ //1. Get verification code picture object var vcode=document.getElementById("vcode"); //2. Set its src attribute and add time stamp //2. Set its src attribute and add time stamp vcode.src = "${pageContext.request.contextPath}/checkCodeServlet?time="+new Date().getTime(); } </script>
<div class="container" style="width: 400px;"> <h3 style="text-align: center;">Administrator login</h3> <form action="${pageContext.request.contextPath}/loginServlet" method="post"> <div class="form-group"> <label for="user">user name:</label> <input type="text" name="username" class="form-control" id="user" placeholder="enter one user name"/> </div> <div class="form-group"> <label for="password">password:</label> <input type="password" name="password" class="form-control" id="password" placeholder="Please input a password"/> </div> <div class="form-inline"> <label for="vcode">Verification Code:</label> <input type="text" name="verifycode" class="form-control" id="verifycode" placeholder="Please enter the verification code" style="width: 120px;"/> <a href="javascript:refreshCode();"> <img src="${pageContext.request.contextPath}/checkCodeServlet" title="Can't see clearly, click refresh" id="vcode"/> </a> </div> <hr/> <div class="form-group" style="text-align: center;"> <input class="btn btn btn-primary" type="submit" value="Sign in"> </div> </form> <!-- Error message box --> <div class="alert alert-warning alert-dismissible" role="alert"> <button type="button" class="close" data-dismiss="alert" > <span>×</span></button> <strong>${login_msg}</strong> </div> </div>
Operation screenshot: 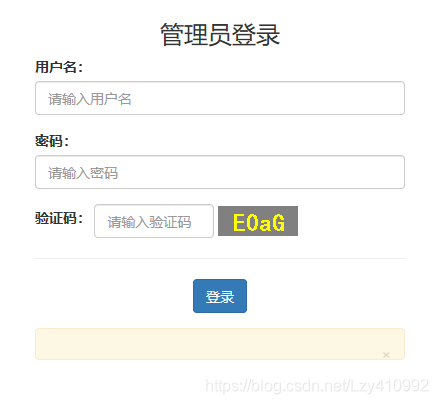 Test the login function and find the problem of Chinese garbled code (direct inheritance) HttpServlet No, inheritance BaseServlet (will appear) #### []( )6. Write filter to solve the problem of Chinese garbled code
@WebFilter("/*")
public class CharchaterFilter implements Filter {
protected String encoding; @Override public void destroy() { // Method stub automatically generated by TODO } @Override public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException { // Method stub automatically generated by TODO HttpServletRequest request=(HttpServletRequest)req; HttpServletResponse response=(HttpServletResponse)res; String method=request.getMethod(); if(method.equalsIgnoreCase("post")){ request.setCharacterEncoding("utf-8"); } response.setContentType("text/html;charset=utf-8"); chain.doFilter(request, response); } @Override public void init(FilterConfig arg0) throws ServletException { // Method stub automatically generated by TODO }
}
#### []( )7. Write a list page and implement the response function on the back-end code
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Website background management</title> <!-- Bootstrap --> <link rel="stylesheet" href="css/bootstrap.min.css"> <!-- jQuery (Bootstrap All of JavaScript All plug-ins depend on jQuery,So it must be put in front) --> <script type="text/javascript" src="js/jquery-2.1.1.min.js"></script> <!-- load Bootstrap All of JavaScript plug-in unit. You can also load only a single plug-in as needed. --> <script type="text/javascript" src="js/bootstrap.min.js"></script> <style type="text/css"> @media (min-width: 768px) { #slider_sub{ width: 200px; margin-top: 51px; position: absolute; z-index: 1; height: 600px; } .mysearch{ margin: 10px 0; } .page_main{ margin-left: 205px; } } </style> <script> function deleteStudent(id){ //User safety tips if(confirm("Are you sure you want to delete?")){ //Access path location.href="${pageContext.request.contextPath}/student?method=delStudent&id="+id; } } window.onload = function(){ //Add a click event to the delete selected button document.getElementById("delSelectedStudent").onclick = function(){ if(confirm("Are you sure you want to delete the selected entry?")){ var flag = false; //Determine whether there is a selected item var cbs = document.getElementsByName("id"); for (var i = 0; i < cbs.length; i++) { if(cbs[i].checked){ //One entry is selected flag = true; break; } } if(flag){//There are entries selected //Form submission document.getElementById("form").submit(); } } } //1. Get the first cb document.getElementById("firstCb").onclick = function(){ //2. Get all CBS in the list below var cbs = document.getElementsByName("uid"); //3. Traversal for (var i = 0; i < cbs.length; i++) { //4. Set the checked status of these cbs[i] = firstcb checked cbs[i].checked = this.checked; } }
Feeling:
In fact, when I submit my resume, I don't dare to send it to Ali. Because Ali has had three interviews in front of him, he didn't get his resume, so he thought he hung up.
Special thanks to the interviewer on one side for fishing me, giving me the opportunity, and recognizing my efforts and attitude. Comparing my facial Sutra with those of other big men, I'm really lucky. Others 80% strength, I may 80% luck. So for me, I will continue to redouble my efforts to make up for my lack of technology and the gap with the leaders of Keban. I hope I can continue to maintain my enthusiasm for learning and continue to work hard.
I also wish all students can find their favorite offer.
The preparations I made before the interview (brush questions, review materials, learning notes and learning routes of some big guys) have been sorted into electronic documents
//1. Get the first cb document.getElementById("firstCb").onclick = function(){ //2. Get all CBS in the list below var cbs = document.getElementsByName("uid"); //3. Traversal for (var i = 0; i < cbs.length; i++) { //4. Set the checked status of these cbs[i] = firstcb checked cbs[i].checked = this.checked; } }
Feeling:
In fact, when I submit my resume, I don't dare to send it to Ali. Because Ali has had three interviews in front of him, he didn't get his resume, so he thought he hung up.
Special thanks to the interviewer on one side for fishing me, giving me the opportunity, and recognizing my efforts and attitude. Comparing my facial Sutra with those of other big men, I'm really lucky. Others 80% strength, I may 80% luck. So for me, I will continue to redouble my efforts to make up for my lack of technology and the gap with the leaders of Keban. I hope I can continue to maintain my enthusiasm for learning and continue to work hard.
I also wish all students can find their favorite offer.
The preparations I made before the interview (brush questions, review materials, learning notes and learning routes of some big guys) have been sorted into electronic documents