Definition: exceptions in Exception except RuntimeException and its subclasses.
Features: the Java compiler will check it. If such an exception occurs in the program, For example, ClassNotFoundException (the specified class exception is not found) and IOException (IO stream exception), either declare and throw it through throws or catch it through try catch, otherwise it cannot be compiled. In the program, this kind of exception is usually not customized, but directly use the exception class provided by the system. For this exception, we must manually add capture statements in the code to handle it.
[](
)4. Inspected anomaly and non inspected anomaly
All Java exceptions can be divided into checked exceptions and unchecked exception s.
[](
)Tested abnormality
The compiler requires an Exception that must be handled. During the operation of the correct program, it is often prone to abnormal conditions that meet the expectations. Once such an Exception occurs, it must be handled in some way. Except RuntimeException and its subclasses, all other exceptions belong to the checked Exception. The compiler will check such exceptions, that is, when the compiler checks that such exceptions may occur somewhere in the application, it will prompt you to handle this Exception - either catch it with try catch or throw it with the throw keyword in the method signature, otherwise the compilation will fail.
[](
)Non inspected abnormality
The compiler does not check and does not require exceptions that must be handled. That is, when such exceptions occur in the program, even if we do not try catch it and throw the exception with throws, the compilation will pass normally. Such exceptions include runtime exceptions (RuntimeException and its subclasses) and errors (errors).
[](
)Java exception keyword
• try – for listening. Put the code to be monitored (the code that may throw an exception) in the try statement block. When an exception occurs in the try statement block, the exception is thrown.
• catch – used to catch exceptions. Catch is used to catch exceptions that occur in the try statement block.
• finally – finally statement blocks are always executed. It is mainly used to recover the material resources (such as database connection, network connection and disk file) opened in the try block. Only after the execution of the finally block is completed, the return or throw statements in the try or catch block will be executed back. If the return or throw and other termination methods are used in the finally block, the execution will not jump back and stop directly.
• throw – used to throw an exception.
• throws – used in the method signature to declare the exceptions that the method may throw.
Java handles exceptions through object-oriented methods. Once the method throws an exception, The system automatically finds the appropriate Exception Handler according to the exception object (Exception Handler) to handle the exception, classify various exceptions, and provide a good interface. In Java, each exception is an object, which is an instance of the Throwable class or its subclass. When an exception occurs in a method, an exception object will be thrown. The object contains exception information, which can be caught by the method calling the object Exception and can be handled. Java exception handling is implemented through five Keywords: try, catch, throw, throws and finally.
In Java applications, exception handling mechanisms are divided into declaring exceptions, throwing exceptions and catching exceptions.
[](
)Declaration exception
Usually, you should catch those exceptions that know how to handle and pass on those exceptions that don't know how to handle. When passing an exception, you can use the throws keyword at the method signature to declare the exception that may be thrown.
be careful
-
Non check exceptions (Error, RuntimeException, or their subclasses) cannot use the throws keyword to declare the exception to be thrown.
-
If a compile time exception occurs in a method, it needs to be handled by try catch / throws, otherwise it will lead to compilation errors.
[](
)Throw exception
If you think you can't solve some exception problems and don't need to be handled by the caller, you can throw an exception.
Throw keyword is used to throw an exception of Throwable type inside a method. Any Java code can throw an exception through the throw statement.
[](
)Catch exception
The program usually does not report errors before running, but some unknown errors may occur after running, but it does not want to throw them directly to the upper level, so it needs to catch exceptions in the form of try... Catch... And then deal with them according to different exceptions.
[](
)How to select exception type
You can choose whether to catch an exception, declare an exception or throw an exception according to the following figure
[](
)Common exception handling methods
[](
)Throw an exception directly
Usually, you should catch those exceptions that know how to handle and pass on those exceptions that don't know how to handle. When passing an exception, you can use the throws keyword at the method signature to declare the exception that may be thrown.
private static void readFile(String filePath) throws IOException { File file = new File(filePath); String result; BufferedReader reader = new BufferedReader(new FileReader(file)); while((result = reader.readLine())!=null) { System.out.println(result); } reader.close(); }
[](
)Encapsulate the exception and throw it again
Sometimes we throw an exception from catch in order to change the type of exception. It is mostly used in multi system integration. When a subsystem fails, there may be multiple exception types, which can be exposed with a unified exception type without exposing too many internal exception details.
private static void readFile(String filePath) throws MyException { try { // code } catch (IOException e) { MyException ex = new MyException("read file failed."); ex.initCause(e); throw ex; } }
[](
)Catch exception
Multiple exception types can be caught in a try catch statement block, and different types of exceptions can be handled differently
private static void readFile(String filePath) { try { // code } catch (FileNotFoundException e) { // handle FileNotFoundException } catch (IOException e){ // handle IOException } }
The same catch can also catch multiple types of exceptions, separated by |
private static void readFile(String filePath) { try { // code } catch (FileNotFoundException | UnknownHostException e) { // handle FileNotFoundException or UnknownHostException } catch (IOException e){ // handle IOException } }
[](
)Custom exception
Traditionally, defining an exception class should include two constructors, a parameterless constructor and a constructor with detailed description (Throwable's toString method will print these details, which is very useful during debugging)
public class MyException extends Exception { public MyException(){ } public MyException(String msg){ super(msg); } // ... }
[](
)try-catch-finally
When an exception occurs in a method, the code after the exception will not be executed. If some local resources have been obtained before and need to be released, the code for releasing local resources needs to be called at the normal end of the method and in the catch statement, which seems cumbersome. finally statement can solve this problem.
private static void readFile(String filePath) throws MyException { File file = new File(filePath); String result; BufferedReader reader = null; try { reader = new BufferedReader(new FileReader(file)); while((result = reader.readLine())!=null) { System.out.println(result); } } catch (IOException e) { System.out.println("readFile method catch block."); MyException ex = new MyException("read file failed."); ex.initCause(e); throw ex; } finally { System.out.println("readFile method finally block."); if (null != reader) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } }
When calling this method, if an exception occurs when reading the file, the code will enter the catch code block, and then enter the finally code block; If no exception occurs when reading the file, it will skip the catch code block and directly enter the finally code block. Therefore, the code in fianlly will execute regardless of whether an exception occurs in the code.
If the catch code block contains a return statement, will the code in finally execute? Modify the catch clause in the above code as follows:
catch (IOException e) { System.out.println("readFile method catch block."); return; }
Invoke the readFile method to observe whether the finally clause executes when the return statement is called in the catch clause.
readFile method catch block. readFile method finally block.
It can be seen that even if the catch contains a return statement, the finally clause will still be executed. If finally also contains a return statement, the return in finally will overwrite the previous return
[](
)try-with-resource
In the above example, the close method in finally may also throw IOException, thus overwriting the original exception. JAVA 7 provides a more elegant way to realize the automatic release of resources. The automatically released resources need to be classes that implement the autoclosable interface.
private static void tryWithResourceTest(){ try (Scanner scanner = new Scanner(new FileInputStream("c:/abc"),"UTF-8")){ // code } catch (IOException e){ // handle exception } }
When the try code block exits, scanner is automatically called Close method, and put scanner The close method is placed in the finally code block. The difference is that if scanner If close throws an exception, it will be suppressed, and the thrown exception is still the original exception. The suppressed exceptions will be added to the original exceptions by the addsuppressed method. If you want to get the list of suppressed exceptions, you can call the getSuppressed method to get it.
Java exception common interview questions
[](
)1. What is the difference between error and Exception?
Error type errors are usually virtual machine related errors, such as system crash, insufficient memory, stack overflow, etc. the compiler will not detect such errors, and JAVA applications should not capture such errors. Once such errors occur, the application will usually be terminated and cannot be recovered by the application itself;
Errors of Exception class can be captured and processed in the application. Usually, when such errors are encountered, they should be processed so that the application can continue to run normally.
[](
)2. What is the difference between runtime exception and general exception (inspected exception)?
Runtime exceptions include the RuntimeException class and its subclasses, which represent the exceptions that may occur during the runtime of the JVM. The Java compiler does not check for runtime exceptions.
The detected Exception is an Exception in Exception except RuntimeException and its subclasses. The Java compiler checks for detected exceptions.
The difference between RuntimeException exception and checked exception: whether to force the caller to handle this exception. If the caller is forced to handle it, the checked exception is used. Otherwise, the non checked exception (RuntimeException) is selected. Generally speaking, if there are no special requirements, we recommend using RuntimeException exception.
[](
)3. How does the JVM handle exceptions?
If an exception occurs in a method, the method will create an exception object and transfer it to the JVM. The exception object contains the exception name, exception description and the state of the application when the exception occurs. The process of creating an exception object and passing it to the JVM is called throwing an exception. There may be a series of method calls that eventually enter the method that throws an exception. The ordered list of this series of method calls is called the call stack.
The JVM will look up the call stack to see if there is any code that can handle exceptions. If so, call the exception handling code. When the JVM finds code that can handle exceptions, it passes the exception to it. If the JVM does not find a code block that can handle the exception, the JVM will transfer the exception to the default exception handler (the default handler is part of the JVM), which prints out the exception information and terminates the application.
[](
)4. What is the difference between throw and throws?
Exception handling in Java includes not only catching and handling exceptions, but also declaring and throwing exceptions. You can declare the exception to be thrown by the method through the throws keyword, or throw the exception object inside the method through the throw keyword.
The differences between the throws keyword and the throw keyword are as follows:
-
Throw keyword is used inside a method. It can only be used to throw an exception. It is used to throw an exception in a method or code block. Both checked and non checked exceptions can be thrown.
-
The throws keyword is used on a method declaration. Multiple exceptions can be thrown to identify the list of exceptions that may be thrown by the method. A method uses throws to identify the list of exceptions that may be thrown. The method calling the method must contain code that can handle exceptions. Otherwise, the corresponding exceptions must be declared with the throws keyword in the method signature.
[](
)5. What is the difference between final, finally and finalize?
-
final can modify classes, variables and methods. A modified class indicates that the class cannot be inherited, a modified method indicates that the method cannot be overridden, and a modified variable indicates that the variable is a constant and cannot be re assigned.
-
Finally is generally used in the try catch code block. When handling exceptions, we usually use the code method finally code block that must be executed, which means that the code block will be executed regardless of whether there is an exception or not. It is generally used to store some code that closes resources.
-
Finalize is a method belonging to the Object class, and the Object class is the parent class of all classes. In Java, it is allowed to use the finalize() method to clean up the objects before the garbage collector clears them from memory.
[](
)6. What is the difference between NoClassDefFoundError and ClassNotFoundException?
NoClassDefFoundError is an Error type exception caused by the JVM. You should not try to catch this exception.
The reason for this exception is that the JVM or ClassLoader cannot find the definition of a class in memory when trying to load it. This action occurs during runtime, that is, the class exists at compile time, but it cannot be found at runtime. It may be caused by mutation and deletion;
ClassNotFoundException is a checked exception, which needs to be explicitly captured and processed with try catch, or declared with the throws keyword in the method signature. When using class forName, ClassLoader. Loadclass or classloader When findsystemclass dynamically loads a class into memory, the exception will be thrown if the class is not found through the passed class path parameters; Another possible reason for throwing this exception is that a class has been loaded into memory by one class loader, and another loader tries to load it.
[](
)7. Which part of try catch finally can be omitted?
A: catch can be omitted
reason
More strictly speaking, try is only suitable for handling runtime exceptions, and try+catch is suitable for handling runtime exceptions + ordinary exceptions. In other words, if you only try to handle ordinary exceptions without catch, the compilation will not pass, because the compiler has a hard rule that if you choose to catch ordinary exceptions, you must use catch to display the declaration for further processing. There is no such provision for runtime exceptions at compile time, so catch can be omitted. You can add catch compiler to it.
In theory, the compiler doesn't like any code and thinks there may be potential problems, so even if you add try to all the code, the code will only add a layer of skin on the basis of normal operation at run time. However, once you add try to a piece of code, you explicitly promise the compiler to catch the exceptions that may be thrown by the code instead of throwing them up. If it is an ordinary exception, the compiler requires that it must be caught with catch for further processing; If the runtime exception is caught, then discarded and + finally swept, or catch caught for further processing.
As for adding finally, it is a "clean-up" process that must be carried out regardless of whether exceptions are caught or not.
[](
)8. In try catch finally, if the catch return s, will finally execute?
A: it will be executed before return.
Note: it is not good to change the return value in finally, because if there is a finally code block, the return statement in try will not immediately return the caller, but record the return value. After the finally code block is executed, return its value to the caller, and then if the return value is modified in finally, the modified value will be returned. Obviously, returning or modifying the return value in finally will cause great trouble to the program. C# directly uses compilation errors to prevent programmers from doing such dirty things. Java can also raise the syntax check level of the compiler to generate warnings or errors.
Code example 1:
public static int getInt() { int a = 10; try { System.out.println(a / 0); a = 20; } catch (ArithmeticException e) { a = 30; return a; /* * return a When the program reaches this step, this is not return a, but return 30; This return path is formed * However, it finds that there is finally, so continue to execute the finally content, a=40 * Return to the previous path again and continue to return 30. After forming the return path, a here is not a variable, but a constant 30 */ } finally { a = 40; } return a; }
Implementation result: 30
Code example 2:
public static int getInt() { int a = 10; try { System.out.println(a / 0); a = 20; } catch (ArithmeticException e) { a = 30; return a; } finally { a = 40; //If so, a return path is formed again. Since you can only return through one return, you can directly return 40 here return a; } }
Implementation result: 40
[](
)9. Class ExampleA inherits Exception and class ExampleB inherits ExampleA.
There are the following code snippets:
try { throw new ExampleB("b") } catch(ExampleA e){ System.out.println("ExampleA"); } catch(Exception e){ System.out.println("Exception"); }
What is the output of executing this code?
Answer:
Output: ExampleA. (according to the Richter substitution principle [where the parent type can be used, the child type must be used], the catch block that grabs the exception of type ExampleA can catch the exception of type ExampleB thrown in the try block)
Interview question - say the running results of the following code. (the source of this topic is the Book Java programming ideas)
class Annoyance extends Exception { } class Sneeze extends Annoyance { } class Human { public static void main(String[] args) throws Exception { try { try { throw new Sneeze(); } catch ( Annoyance a ) { System.out.println("Caught Annoyance"); throw a; } } catch ( Sneeze s ) { System.out.println("Caught Sneeze"); return ; } finally { System.out.println("Hello World!"); } } }
result
Caught Annoyance Caught Sneeze Hello World!
[](
)10. What are the common runtimeexceptions?
-
ClassCastException (class conversion exception)
-
Indexoutofboundsexception (array out of bounds)
-
NullPointerException (null pointer)
-
Arraystoreexception (data storage exception, inconsistent type when operating array)
-
There are also BufferOverflowException exceptions for IO operations
[](
)11. What are the common Java exceptions
java.lang.IllegalAccessError: illegal access error. This exception is thrown when an application attempts to access, modify the Field of a class or call its method, but violates the visibility declaration of the Field or method.
java.lang.InstantiationError: instantiation error. This exception is thrown when an application attempts to construct an abstract class or interface through Java's new operator
java.lang.OutOfMemoryError: out of memory error. This error is thrown when the available memory is insufficient for the Java virtual machine to allocate to an object.
java.lang.StackOverflowError: stack overflow error. This error is thrown when the level of an application recursive call is too deep, resulting in stack overflow or falling into an endless loop.
java.lang.ClassCastException: class modeling exception. Assuming that there are classes A and B (A is not the parent or child of B) and O is an instance of A, this exception is thrown when o is forcibly constructed as an instance of class B. this exception is often called A cast exception.
java.lang.ClassNotFoundException: class exception not found. This exception is thrown when the application attempts to construct a class according to the class name in string form, but cannot find the class file with the corresponding name after traversing the classpath.
java.lang.ArithmeticException: arithmetic condition exception. For example: integer division by zero, etc.
java.lang.ArrayIndexOutOfBoundsException: array index out of bounds exception. Thrown when the index value of the array is negative or greater than or equal to the array size.
java.lang.IndexOutOfBoundsException: index out of bounds exception. This exception is thrown when the index value accessing a sequence is less than 0 or greater than or equal to the sequence size.
java.lang.InstantiationException: instantiation exception. This exception is thrown when an attempt is made to create an instance of a class that is an abstract class or interface through the newInstance() method.
java.lang.NoSuchFieldException: property has no exception. This exception is thrown when a nonexistent property of a class is accessed.
java.lang.NoSuchMethodException: method has no exception. This exception is thrown when a nonexistent method of a class is accessed.
java.lang.NullPointerException: null pointer exception. This exception is thrown when an application attempts to use null where an object is required. For example, call the instance method of null object, access the properties of null object, calculate the length of null object, throw null with throw statement, etc.
java.lang.NumberFormatException: number format exception. This exception is thrown when an attempt is made to convert a String to the specified numeric type, and the String does not meet the format required by the numeric type.
java.lang.StringIndexOutOfBoundsException: string index out of bounds exception. This exception is thrown when an index value is used to access characters in a string, and the index value is less than 0 or greater than or equal to the sequence size.
Java exception handling best practices
Handling exceptions in Java is not a simple thing. It is not only difficult for beginners to understand, but even some experienced developers need to spend a lot of time thinking about how to deal with exceptions, including what exceptions to deal with, how to deal with, and so on. This is why most development teams make some rules to regulate exception handling. These norms are often quite different between teams.
This article presents several exception handling best practices used by many teams.
[](
)1. Clean up resources in the finally block or use the try with resource statement
When using a resource like InputStream that needs to be closed after use, a common error is to close the resource at the end of the try block.
public void doNotCloseResourceInTry() { FileInputStream inputStream = null; try { File file = new File("./tmp.txt"); inputStream = new FileInputStream(file); // use the inputStream to read a file // do NOT do this inputStream.close(); } catch (FileNotFoundException e) { log.error(e); } catch (IOException e) { log.error(e); } }
The problem is that this code works only when there is no exception thrown. The code in the try code block will execute normally, and the resources can be shut down normally. However, there are reasons for using try code blocks. Generally, you call one or more methods that may throw exceptions, and you may throw an exception yourself, which means that the code may not execute to the last part of the try code block. As a result, you did not close the resource.
Therefore, you should put the clean-up code in finally, or use the try with resource feature.
[](
)1.1 using finally code blocks
Unlike the previous lines of try code blocks, finally code blocks are always executed. It will be executed after the try code block is successfully executed or after you handle the exception in the catch code block. Therefore, you can ensure that you clean up all open resources.
public void closeResourceInFinally() { FileInputStream inputStream = null; try { File file = new File("./tmp.txt"); inputStream = new FileInputStream(file); // use the inputStream to read a file } catch (FileNotFoundException e) { log.error(e); } finally { if (inputStream != null) { try { inputStream.close(); } catch (IOException e) { log.error(e); } } } }
[](
)1.2 try with resource syntax of Java 7
If your resource implements the autoclosable interface, you can use this syntax. Most Java standard resources inherit this interface. When you open a resource in the try clause, the resource will be automatically closed after the try code block is executed or after exception handling.
public void automaticallyCloseResource() { File file = new File("./tmp.txt"); try (FileInputStream inputStream = new FileInputStream(file);) { // use the inputStream to read a file } catch (FileNotFoundException e) { log.error(e); } catch (IOException e) { log.error(e); } }
[](
)2. Give priority to clear exceptions
The more explicit the exception you throw, the better. Always remember that your colleagues or you in a few months will call your method and handle the exception.
Therefore, it is necessary to ensure that as much information as possible is provided to them. This makes your API easier to understand. Callers of your methods can handle exceptions better and avoid additional checks.
Therefore, always try to find the class that best suits your exception event. For example, throw a NumberFormatException instead of an IllegalArgumentException. Avoid throwing an ambiguous exception.
public void doNotDoThis() throws Exception { ... } public void doThis() throws NumberFormatException { ... }
[](
)3. Document the exception
Documentation is also required when an exception is thrown on a method declaration. The purpose is to provide the caller with as much information as possible, so that exceptions can be better avoided or handled.
Add the @ throws declaration in Javadoc and describe the scenario of throwing exceptions.
public void doSomething(String input) throws MyBusinessException { ... }
[](
)4. Throw an exception using a descriptive message
When throwing an exception, you need to describe the problem and related information as accurately as possible, so that it can be easier for people to read whether printed in the log or in the monitoring tool, so as to better locate the specific error information and the severity of the error.
However, this does not mean that we should make a long speech on the error information, because the class name of Exception can reflect the cause of the error, so we only need to describe it in one or two sentences.
If a particular exception is thrown, its class name probably already describes the error. So you don't need to provide a lot of additional information. A good example is NumberFormatException. When you provide a String in the wrong format, it will be used by Java Thrown by the constructor of class lang.long.
try { new Long("xyz"); } catch (NumberFormatException e) { log.error(e); }
[](
)5. Give priority to capturing the most specific exceptions
Most ides can help you implement this best practice. When you try to catch less specific exceptions first, they report inaccessible code blocks.
The problem is that only the first catch block that matches the exception is executed. Therefore, if you catch the IllegalArgumentException first, you will never reach the catch block that should handle the more specific NumberFormatException, because it is a subclass of IllegalArgumentException.
Always catch the most specific exception class first, and add the less specific catch block to the end of the list.
You can see an example of such a try catch statement in the following code snippet. The first catch block handles all numberformatexceptions, and the second handles all illegalargumentexceptions that are not numberformatexceptions.
public void catchMostSpecificExceptionFirst() { try { doSomething("A message"); } catch (NumberFormatException e) { log.error(e); } catch (IllegalArgumentException e) { log.error(e) } }
[](
)6. Do not capture the Throwable class
Throwable is a superclass for all exceptions and errors. You can use it in the catch clause, but you should never!
If Throwable is used in the catch clause, it will catch not only all exceptions, but also all errors. The JVM throws an error indicating a serious problem that should not be handled by the application. Typical examples are OutOfMemoryError or StackOverflowError. Both are caused by conditions outside the control of the application and cannot be handled.
Therefore, it is best not to catch Throwable unless you are sure that you are in a special situation to handle errors.
public void doNotCatchThrowable() { try { // do something } catch (Throwable t) { // don't do this! } }
[](
)7. Don't ignore exceptions
Many times, developers are confident that they will not throw exceptions, so they write a catch block, but do not do any processing or log.
public void doNotIgnoreExceptions() { try { // do something } catch (NumberFormatException e) { // this will never happen } }
But the reality is that unexpected exceptions often occur, or it is uncertain whether the code here will be changed in the future (the code that prevents exception throwing is deleted). At this time, due to the exception being caught, it is impossible to get enough error information to locate the problem.
It is reasonable to record at least abnormal information.
public void logAnException() { try { // do something **Finally, attach a brain map prepared before the interview:** 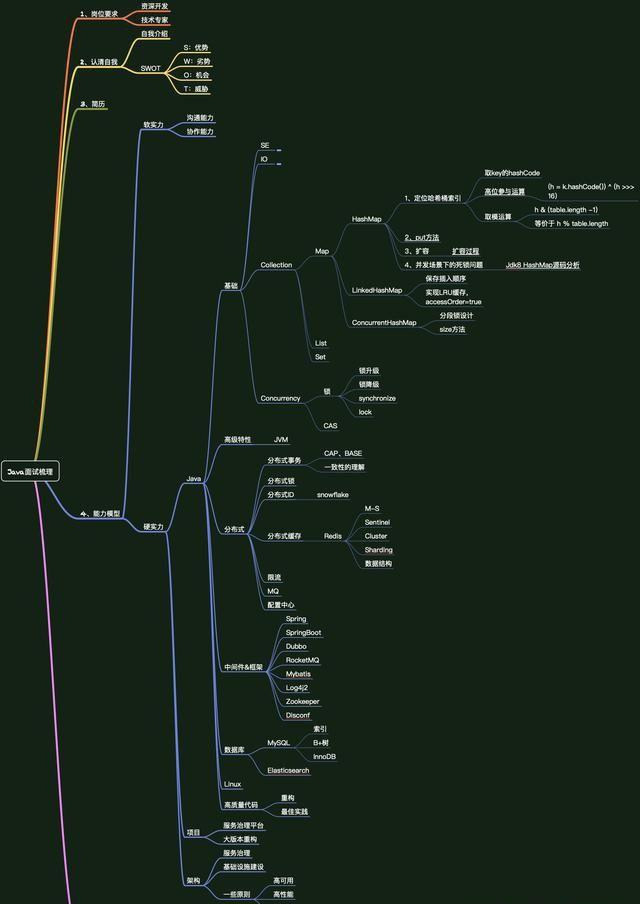 **Before the interview, you must brush the questions. In order to facilitate your review, I share a collection of personal interviews** * Java Core knowledge sorting 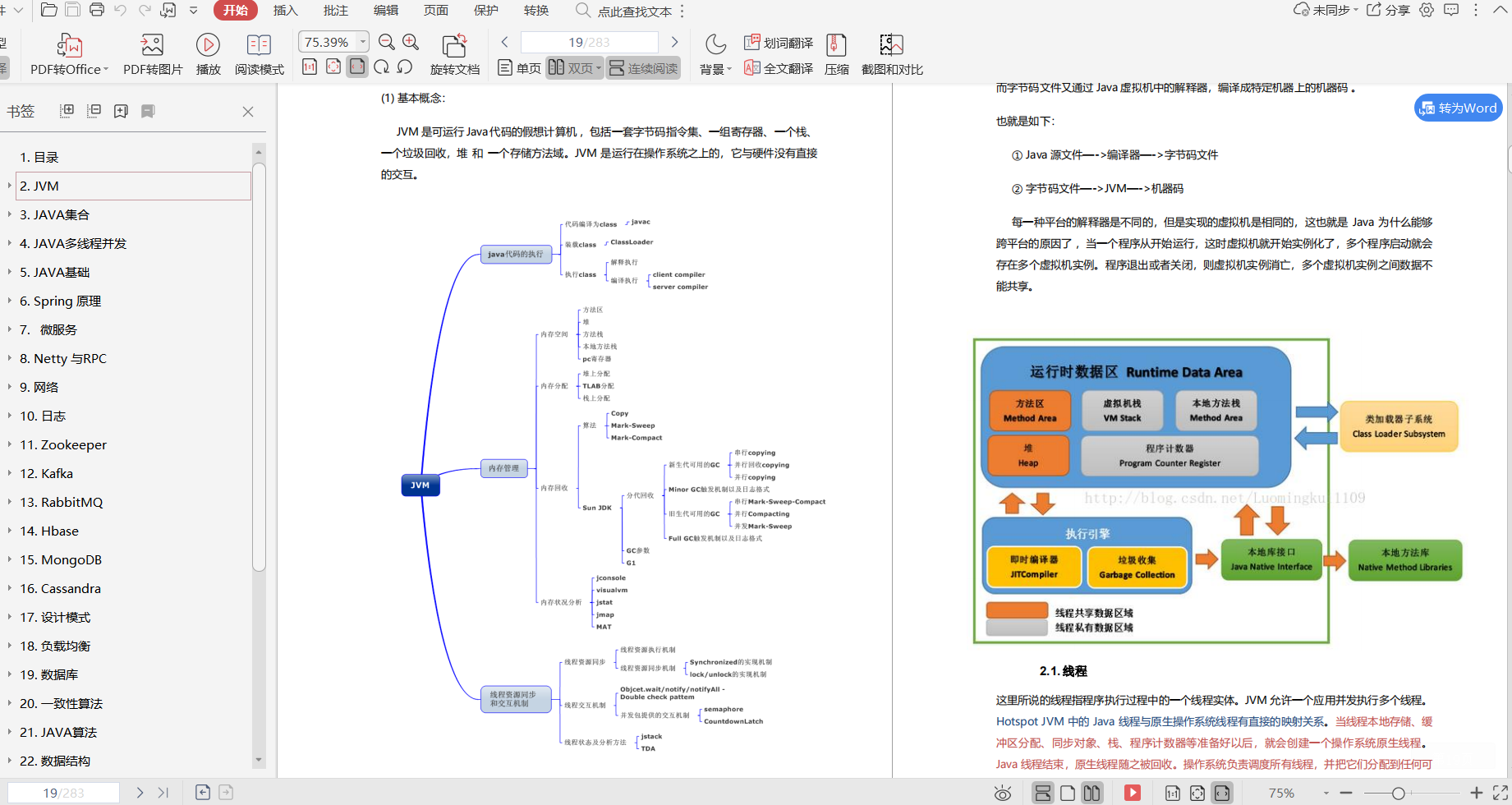 * Spring Family bucket (Practical Series) 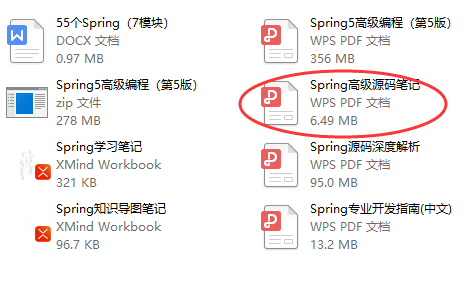 **Step3: Brush questions** Since it's an interview, I have to brush questions. In fact, I can't go anywhere after the Spring Festival. I brush a lot of interview questions myself, so I can know what knowledge points and high-frequency questions will be asked in the interview process. Therefore, brushing questions is a very important point in the early preparation of the interview. **The following is my private interview question bank:** 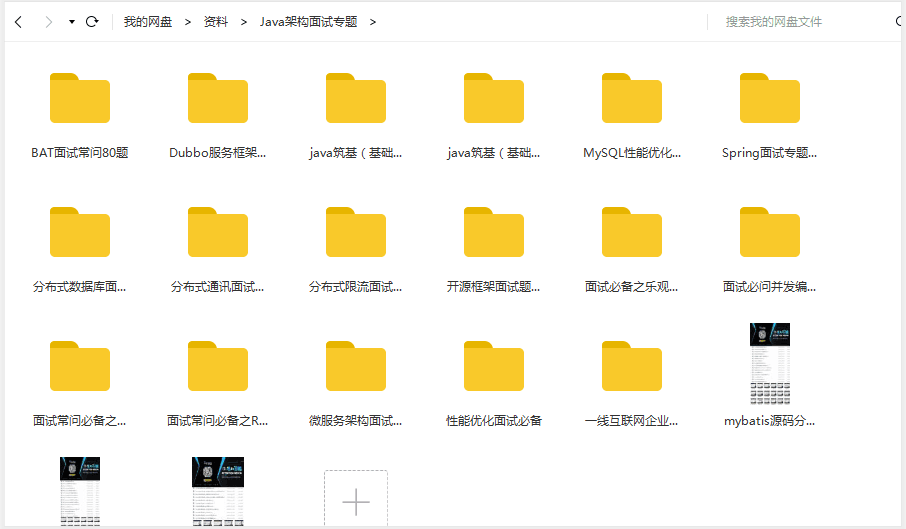 Many people lament that "learning is useless". In fact, the useless theory arises because what they want does not match what they have learned, which means that they have not learned enough. Whether studying or working, you should have initiative, so if you have a big factory dream, you should try your best to realize it. **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** try { // do something } catch (Throwable t) { // don't do this! } }
[](
)7. Don't ignore exceptions
Many times, developers are confident that they will not throw exceptions, so they write a catch block, but do not do any processing or log.
public void doNotIgnoreExceptions() { try { // do something } catch (NumberFormatException e) { // this will never happen } }
But the reality is that unexpected exceptions often occur, or it is uncertain whether the code here will be changed in the future (the code that prevents exception throwing is deleted). At this time, due to the exception being caught, it is impossible to get enough error information to locate the problem.
It is reasonable to record at least abnormal information.
public void logAnException() { try { // do something **Finally, attach a brain map prepared before the interview:** [External chain picture transfer...(img-S7xPOGdm-1630717868307)] **Before the interview, you must brush the questions. In order to facilitate your review, I share a collection of personal interviews** * Java Core knowledge sorting [External chain picture transfer...(img-padkgNhT-1630717868308)] * Spring Family bucket (Practical Series) [External chain picture transfer...(img-jJptKYVz-1630717868309)] **Step3: Brush questions** Since it's an interview, I have to brush questions. In fact, I can't go anywhere after the Spring Festival. I brush a lot of interview questions myself, so I can know what knowledge points and high-frequency questions will be asked in the interview process. Therefore, brushing questions is a very important point in the early preparation of the interview. **The following is my private interview question bank:** [External chain picture transfer...(img-kbxM2gAr-1630717868311)] Many people lament that "learning is useless". In fact, the useless theory arises because what they want does not match what they have learned, which means that they have not learned enough. Whether studying or working, you should have initiative, so if you have a big factory dream, you should try your best to realize it. **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** Finally, I wish you good health and get your favorite offer!