-
Class load
-
Load the bytecode content of the class file into memory, convert these static data into the run-time data structure of the method area, and then generate a Java Lang.class object
-
The class loader loads classes into memory. There are bootstrap classloader (provided by the JVM, written in C + +, which is responsible for the core library of the Java platform and cannot be obtained directly), extension classloader (responsible for packaging jars in the jre/lib/ext directory into the working Library), and system classloader (System Classloader) (responsible for packing classes and jars in the directory referred to in Java classpath, the most commonly used loader)
-
The parental delegation mechanism ensures that classes are loaded correctly
//Class loader demo public class ClassLoaderDemo { public static void main(String[] args) throws ClassNotFoundException { //Gets the loader of the system class ClassLoader systemClassLoader = ClassLoader.getSystemClassLoader(); System.out.println(systemClassLoader); //Gets the parent class loader -- > extension class loader of the system class loader ClassLoader parent = systemClassLoader.getParent(); System.out.println(parent); //Gets the parent class loader -- > root loader of the extension class loader ClassLoader parent1 = parent.getParent(); System.out.println(parent1); //Test which loader loads the current class ClassLoader classLoader = Class.forName("com.jayz.annotationDemo.ClassLoaderDemo").getClassLoader(); System.out.println(classLoader); //Test JDK built-in class loading classLoader = Class.forName("java.lang.Object").getClassLoader(); System.out.println(classLoader); //Gets the path where the system class loader loads System.out.println(System.getProperty("java.class.path")); } }
-
-
Class link
-
Merge the binary code of Java classes into the JVM runtime environment
-
Verification: ensure that the loaded class information conforms to the JVM specification and there is no security problem
-
Preparation: formally allocate memory for class variables (static) and set the default initial value of class variables. Memory will be allocated in the method area.
-
Resolution: the process of replacing the symbolic reference (constant name) in the virtual machine constant pool with a direct reference (address)
-
-
-
Class initialization
-
The process of executing a class constructor () method. The class constructor method is generated by the combination of the assignment actions of all class variables in the class automatically collected by the compiler and the statements in the static code block (not the constructor of the class object)
-
When initializing a class, if it is found that its parent class has not been initialized, the initialization of its parent class is triggered first
-
The virtual machine ensures that the () methods of a class are locked and synchronized correctly in a multithreaded environment.
-
Class initialization (active application of class) occurs, and the passive reference will not.
- Active reference: when the virtual machine starts, initialize the class where the main method is located first
-
new is an object of a class
-
Calling static methods and static members of a class
-
Using Java Methods in the lang.reflect package make reflection calls to classes
-
Initialize a class. If its parent class is not initialized, initialize the parent class first
-
Passive reference
-
When accessing a static domain, only the class that actually declares the domain will be initialized (for example, if the subclass references the static variable of the parent class, the subclass will not be initialized)
-
Defining a class reference through an array does not trigger the initialization of this class
-
Reference constants do not trigger initialization (constants are stored in the constant pool during the link phase)
-
-
public class InitiDemo { static { System.out.println("Main Loading of class"); } public static void main(String[] args) throws ClassNotFoundException { //1. Active reference //Son son = new Son(); // The main class is loaded, the parent class is loaded, and the child class is loaded // reflex //Class. forName("com.jayz.annotationDemo.Son");// The parent class of the main class is loaded, and the child class is loaded //Passive reference //System. out. println(Son.b);// The parent class of main is loaded 2 Son[] sons = new Son[5]; //Main class loading } } class Father{ static int b = 2; static{ System.out.println("The parent class is loaded"); } } class Son extends Father{ static { System.out.println("Subclass loaded"); m = 300; } static int m = 100; static final int M = 1; }
Type 2.3 information
Example: get class related information (method, property, constructor, parent class, interface, annotation)
//Get class information public class ClassInformation { public static void main(String[] args) throws ClassNotFoundException, NoSuchFieldException, NoSuchMethodException { Class c1 = Class.forName("com.jayz.annotationDemo.User"); //Get the name of the class System.out.println(c1.getName()); //Get package name + class name System.out.println(c1.getSimpleName()); //Get class name //Get the properties of the class Field[] fields = c1.getFields(); //Only public properties can be found fields = c1.getDeclaredFields(); // for(Field field:fields) { System.out.println(field); } //Gets the value of the specified property Field name = c1.getDeclaredField("name"); System.out.println(name); //Method to get class Method[] methods = c1.getMethods(); //Get all public methods of this class and its parent class for(Method method:methods) { System.out.println(method); } methods = c1.getDeclaredMethods(); //Get all methods of this class for(Method method:methods) { System.out.println(method); } //Get constructor method Constructor constructor = c1.getConstructor(); Constructor[] constructors = c1.getConstructors(); } }
Example: * * construct objects through reflection, call methods and properties (private, setAccessible(true))** Using reflection can affect program efficiency.
//Reflection operation public class ReflectionDemo02 { public static void main(String[] args) throws IllegalAccessException, InstantiationException, ClassNotFoundException, NoSuchMethodException, InvocationTargetException, NoSuchFieldException { //Get Class object Class c1 = Class.forName("com.jayz.annotationDemo.User"); //Construction object User u1 = (User)c1.newInstance(); //Call the parameterless constructor of the class. If a parameterless construct is defined and no parameterless construct is defined, this method cannot be used System.out.println(u1); //Creating objects through constructors Constructor constructor = c1.getConstructor(String.class, int.class, int.class); User u2 = (User)constructor.newInstance("jay", 01, 18); System.out.println(u2); //Call normal methods through reflection User u3 = (User)c1.newInstance(); Method setName = c1.getDeclaredMethod("setName", String.class); //invoke activation method setName.invoke(u3,"jay2"); System.out.println(u3.getName()); //Reflection operation properties User u4 = (User)c1.newInstance(); Field name = c1.getDeclaredField("name");//Insufficient permissions to access private properties //Close permissions name.setAccessible(true); name.set(u4,"jay3"); System.out.println(u4.getName()); } }
2.4 ORM (Object relation Mapping): use annotation and reflection to complete the mapping relationship between class and table structure
public class ORM_Demo { public static void main(String[] args) throws ClassNotFoundException, NoSuchFieldException { Class c1 = Class.forName("com.jayz.annotationDemo.Student01"); //Get annotations through reflection Annotation[] annotations = c1.getAnnotations(); for (Annotation annotation : annotations) { System.out.println(annotation); } //Gets the value of the annotated value TableJay tableJay = (TableJay)c1.getAnnotation(TableJay.class); String value = tableJay.value(); System.out.println(value); //Gets the annotation specified by the class Field name = c1.getDeclaredField("name"); FieldJay fieldJay = name.getAnnotation(FieldJay.class); System.out.println(fieldJay.columnName()); System.out.println(fieldJay.length()); System.out.println(fieldJay.type()); } } @TableJay("db_student") class Student01{ @FieldJay(columnName = "db_id",type = "int",length = 10) private int id; @FieldJay(columnName = "db_name",type = "varchar",length = 3) private String name; @FieldJay(columnName = "db_age",type = "int",length = 10) private int age; public Student01(){} public Student01(int id, String name, int age) { this.id = id; this.name = name; this.age = age; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } ### last **If you need this, it's necessary for you to enter the factory redis Please praise videos, interview questions and technical documents** **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** I wish you all to enter the big factory as soon as possible and get a satisfactory salary and rank~~~come on. Thank you for your support!! 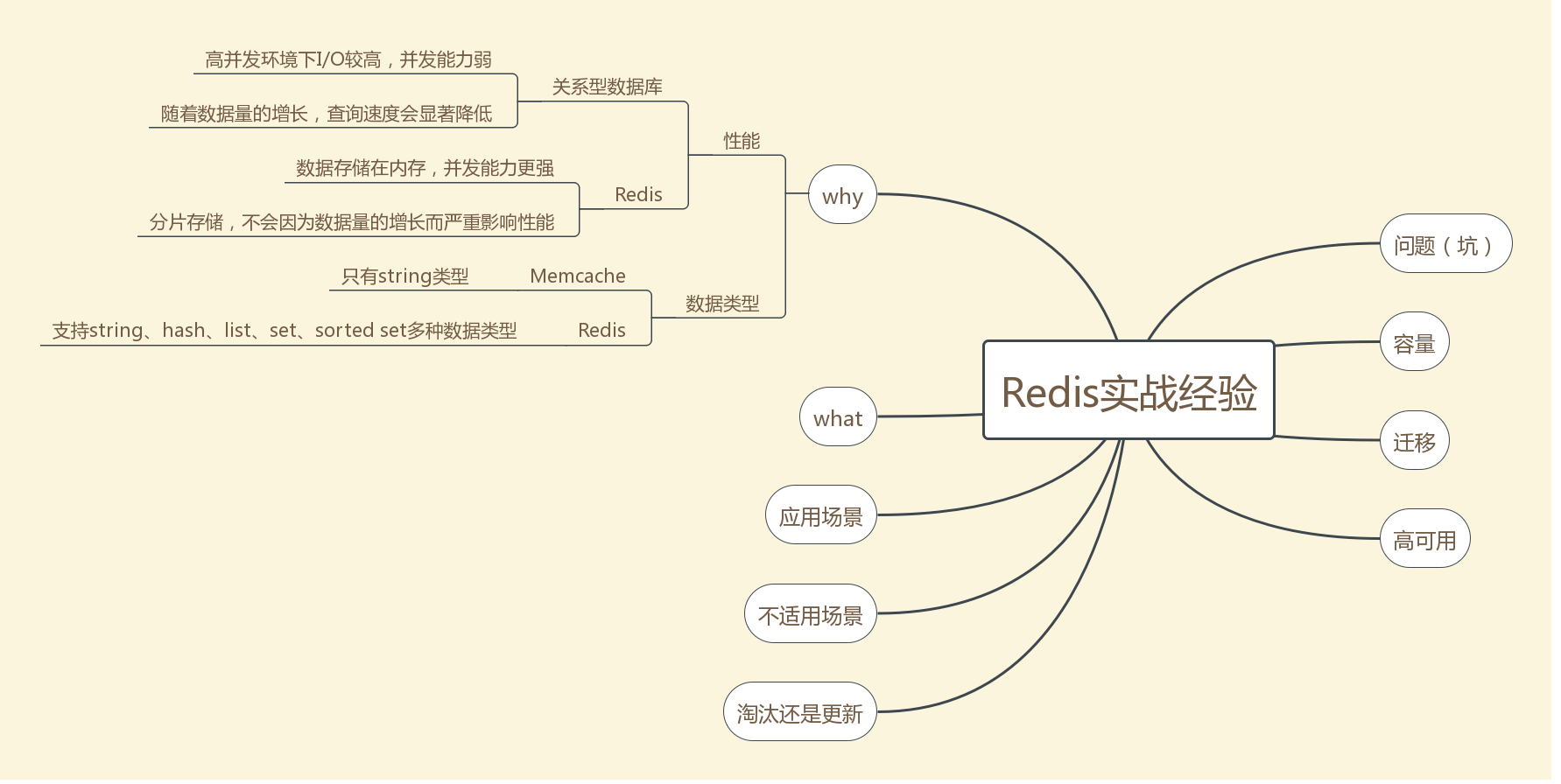 this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } ### last **If you need this, it's necessary for you to enter the factory redis Please praise videos, interview questions and technical documents** **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** I wish you all to enter the big factory as soon as possible and get a satisfactory salary and rank~~~come on. Thank you for your support!! [External chain picture transfer...(img-2FqktRPA-1630556331589)]