* `LAYOUT_IN_DISPLAY_CUTOUT_MODE_SHORT_EDGES` * `LAYOUT_IN_DISPLAY_CUTOUT_MODE_NEVER` The default value is`LAYOUT_IN_DISPLAY_CUTOUT_MODE_DEFAULT`,The fringe area will not display content, and the value needs to be set to`LAYOUT_IN_DISPLAY_CUTOUT_MODE_SHORT_EDGES`
-
You can simulate the screen gap on any device or simulator running Android P as follows:
- Enable Developer options.
- In the Developer options screen, scroll down to the Drawing section and select Simulate a display with a cutout.
- Select the size of the notch screen.
-
Adaptation reference:
// Extend display area to bangs WindowManager.LayoutParams lp = window.getAttributes(); lp.layoutInDisplayCutoutMode = WindowManager.LayoutParams.LAYOUT_IN_DISPLAY_CUTOUT_MODE_SHORT_EDGES; window.setAttributes(lp); // Setting page full screen display final View decorView = window.getDecorView(); decorView.setSystemUiVisibility(View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_LAYOUT_STABLE);
The code that extends the display area to the bangs can also be implemented by modifying the Activity or application style, for example:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="AppTheme" parent="xxx"> <item name="android:windowLayoutInDisplayCutoutMode">shortEdges</item> </style> </resources>
Android O adapter
Because Google's official adaptation scheme was not launched until Android P, each manufacturer has its own implementation scheme on Android O devices.
I have mainly adapted Huawei, Xiaomi and oppo, all of which have given complete solutions. As for vivo, vivo gives the API for judging whether the bangs are displayed, but it is not necessary to set the bangs area to be displayed in the API, so there is no need to adapt.
Adapting Huawei Android O devices
Scheme I:
-
The specific methods are as follows:
<meta-data android:name="android.notch_support" android:value="true"/>
-
Effective for Application means that the system will not perform special downward movement of vertical screen scene or right movement of horizontal screen scene for all pages of the Application:
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:testOnly="false" android:supportsRtl="true" android:theme="@style/AppTheme"> <meta-data android:name="android.notch_support" android:value="true"/> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity>
-
Effective for Activity means that bangs can be adapted for a single page. The Activity system with this property set will not do special processing:
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:testOnly="false" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity> <activity android:name=".LandscapeFullScreenActivity" android:screenOrientation="sensor"> </activity> <activity android:name=".FullScreenActivity"> <meta-data android:name="android.notch_support" android:value="true"/> </activity> </application>
Scheme II
Effective for Application means that all pages of the Application will not be specially moved down in the vertical screen scene or right in the horizontal screen scene
my NotchScreenTool Scheme 2 is used in. If you need to target an Activity, you are recommended to modify it yourself.
-
Set the application window to use the bangs area on Huawei bangs screen mobile phones
/*Bang screen full screen display FLAG*/ public static final int FLAG_NOTCH_SUPPORT=0x00010000; /** * Set the application window to use the bangs area on Huawei bangs screen mobile phones * @param window Application page window object */ public static void setFullScreenWindowLayoutInDisplayCutout(Window window) { if (window == null) { return; } WindowManager.LayoutParams layoutParams = window.getAttributes(); try { Class layoutParamsExCls = Class.forName("com.huawei.android.view.LayoutParamsEx"); Constructor con=layoutParamsExCls.getConstructor(LayoutParams.class); Object layoutParamsExObj=con.newInstance(layoutParams); Method method=layoutParamsExCls.getMethod("addHwFlags", int.class); method.invoke(layoutParamsExObj, FLAG_NOTCH_SUPPORT); } catch (ClassNotFoundException | NoSuchMethodException | IllegalAccessException |InstantiationException | InvocationTargetException e) { Log.e("test", "hw add notch screen flag api error"); } catch (Exception e) { Log.e("test", "other Exception"); } }
-
Clear the added Huawei bangs Flag and restore the application without bangs
/** * Set the application window to use the bangs area on Huawei bangs screen mobile phones * @param window Application page window object */ public static void setNotFullScreenWindowLayoutInDisplayCutout (Window window) { if (window == null) { return; } WindowManager.LayoutParams layoutParams = window.getAttributes(); try { Class layoutParamsExCls = Class.forName("com.huawei.android.view.LayoutParamsEx"); Constructor con=layoutParamsExCls.getConstructor(LayoutParams.class); Object layoutParamsExObj=con.newInstance(layoutParams); Method method=layoutParamsExCls.getMethod("clearHwFlags", int.class); method.invoke(layoutParamsExObj, FLAG_NOTCH_SUPPORT); } catch (ClassNotFoundException | NoSuchMethodException | IllegalAccessException |InstantiationException | InvocationTargetException e) { Log.e("test", "hw clear notch screen flag api error"); } catch (Exception e) { Log.e("test", "other Exception"); } }
Adapt to Xiaomi Android O device
-
Judge whether it is Liu Haiping
private static boolean isNotch() { try { Method getInt = Class.forName("android.os.SystemProperties").getMethod("getInt", String.class, int.class); int notch = (int) getInt.invoke(null, "ro.miui.notch", 0); return notch == 1; } catch (Throwable ignore) { } return false; }
-
Set display to fringe area
@Override public void setDisplayInNotch(Activity activity) { int flag = 0x00000100 | 0x00000200 | 0x00000400; try { Method method = Window.class.getMethod("addExtraFlags", int.class); method.invoke(activity.getWindow(), flag); } catch (Exception ignore) { } }
-
Get bangs width and height
public static int getNotchHeight(Context context) { int resourceId = context.getResources().getIdentifier("notch_height", "dimen", "android"); if (resourceId > 0) { return context.getResources().getDimensionPixelSize(resourceId); } return 0; } public static int getNotchWidth(Context context) { int resourceId = context.getResources().getIdentifier("notch_width", "dimen", "android"); if (resourceId > 0) { return context.getResources().getDimensionPixelSize(resourceId); } return 0; }
Adapt to oppoo devices
-
Judge whether it is Liu Haiping
@Override public boolean hasNotch(Activity activity) { boolean ret = false; try { ret = activity.getPackageManager().hasSystemFeature("com.oppo.feature.screen.heteromorphism"); } catch (Throwable ignore) { } return ret; }
-
Get the coordinates of the upper left and lower right corners of the bangs
/** * Get the coordinates of bangs * <p> * The attribute form is as follows: [ro.oppo.screen.heteromorphism]: [378,0:702,80] * <p> * The obtained value is 378,0:702,80 * <p> * <p> * (378,0)Is the coordinate of the upper left corner of the fringe area * <p> * (702,80)Is the coordinate of the lower right corner of the fringe area */ private static String getScreenValue() { String value = ""; Class<?> cls; try { cls = Class.forName("android.os.SystemProperties"); Method get = cls.getMethod("get", String.class); Object object = cls.newInstance(); value = (String) get.invoke(object, "ro.oppo.screen.heteromorphism"); } catch (Throwable ignore) { } return value; }
Oppo Android O models do not need to be displayed in the fringe area. As long as the application full screen is set, it will be displayed by default.
Therefore, Oppo models must be adapted.
Adaptation summary
Based on the above functions, I organized it into a dependency Library: NotchScreenTool
Easy to use:
// Support display to fringe area NotchScreenManager.getInstance().setDisplayInNotch(this); // Get Liu Haiping information NotchScreenManager.getInstance().getNotchInfo(this, new INotchScreen.NotchScreenCallback() { @Override public void onResult(INotchScreen.NotchScreenInfo notchScreenInfo) { Log.i(TAG, "Is this screen notch? " + notchScreenInfo.hasNotch); if (notchScreenInfo.hasNotch) { ## last It is said that three years is a barrier for programmers. Whether they can be promoted or improve their core competitiveness is very critical in recent years. **With the rapid development of technology, what aspects should we start to learn in order to reach the level of senior engineer and finally advance to Android architect /technician? I summed up these five pieces;** > I have collected and sorted out the interview questions of Alibaba, Tencent, byte beat, Huawei, Xiaomi and other companies in recent years, and sorted out the interview requirements and technical points into a large and comprehensive report“ Android "Architect" interview PDF(In fact, it takes a lot more energy than expected), including the context of knowledge + Branch details. >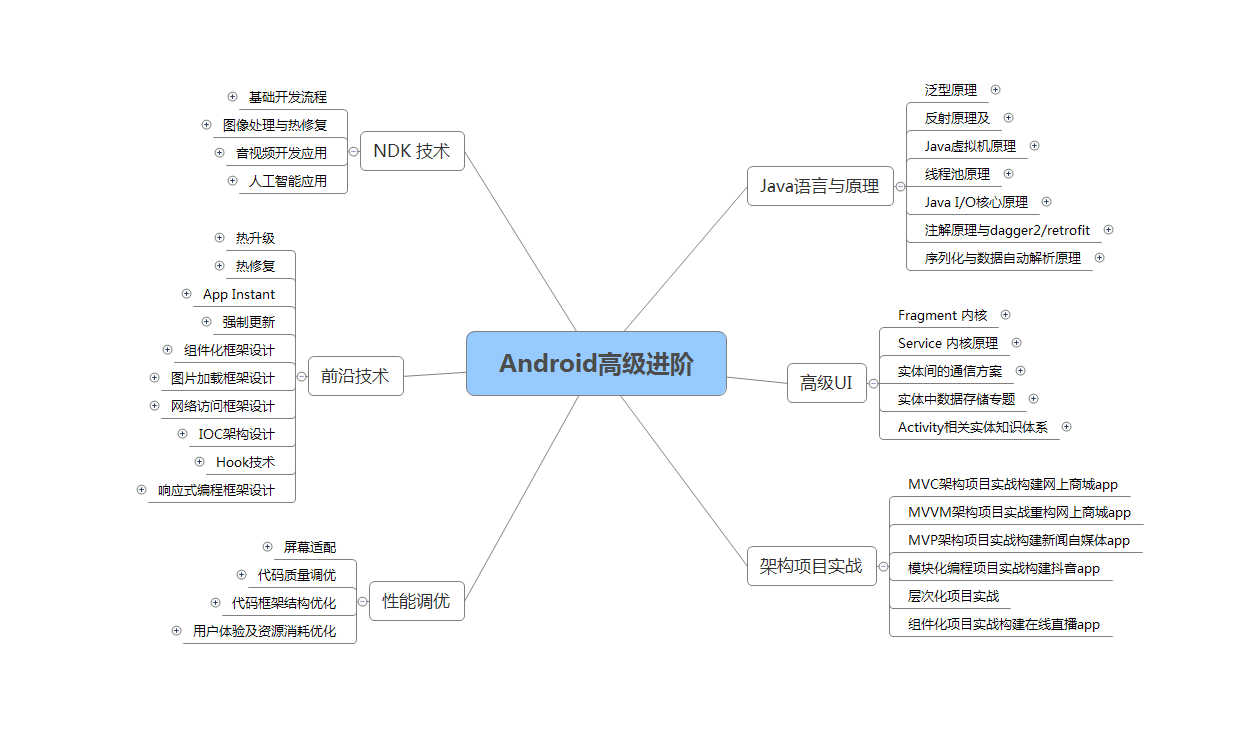 **Java Language and principles;** Big factory, small factory. Android The interview depends on whether you are familiar with it Java language > 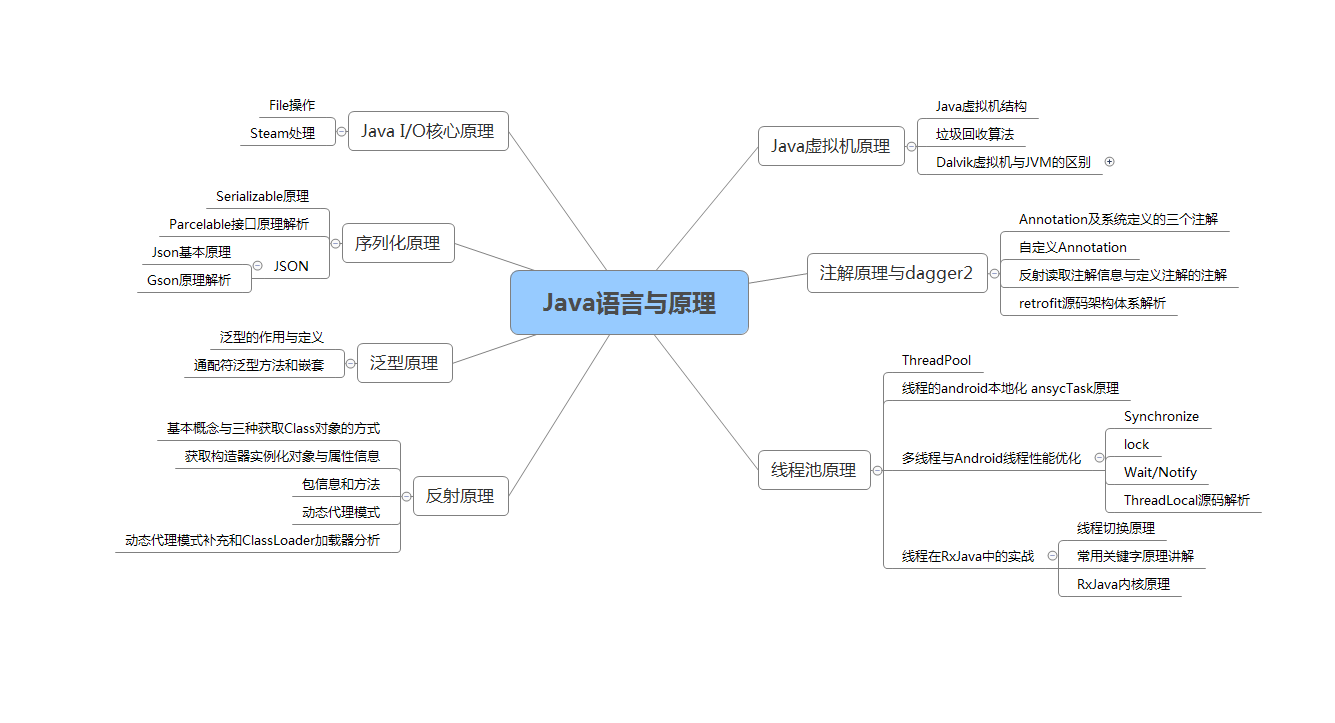 **senior UI And customization view;** custom view,Android Basic skills of development. > 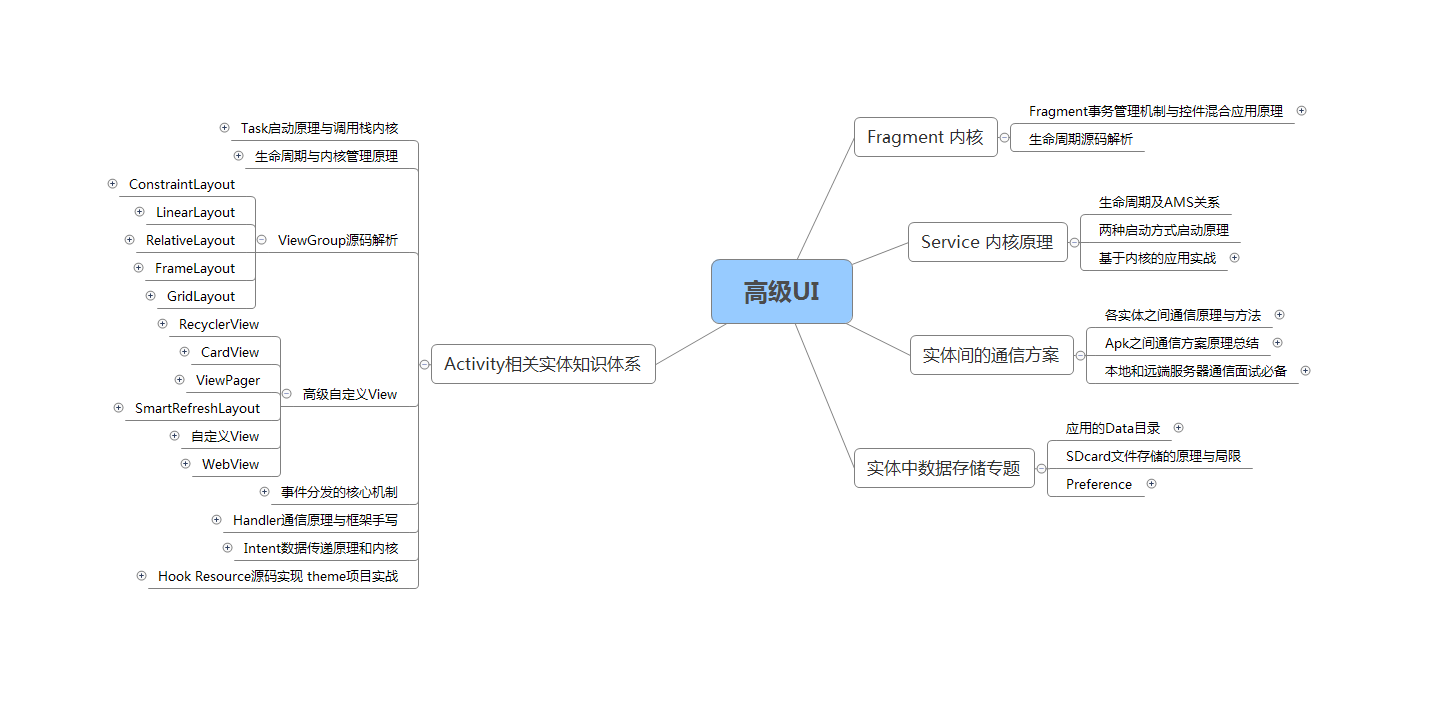 **Performance tuning;** Data structure, algorithm, design pattern. These are the key foundations and key points, which need to be skilled. > 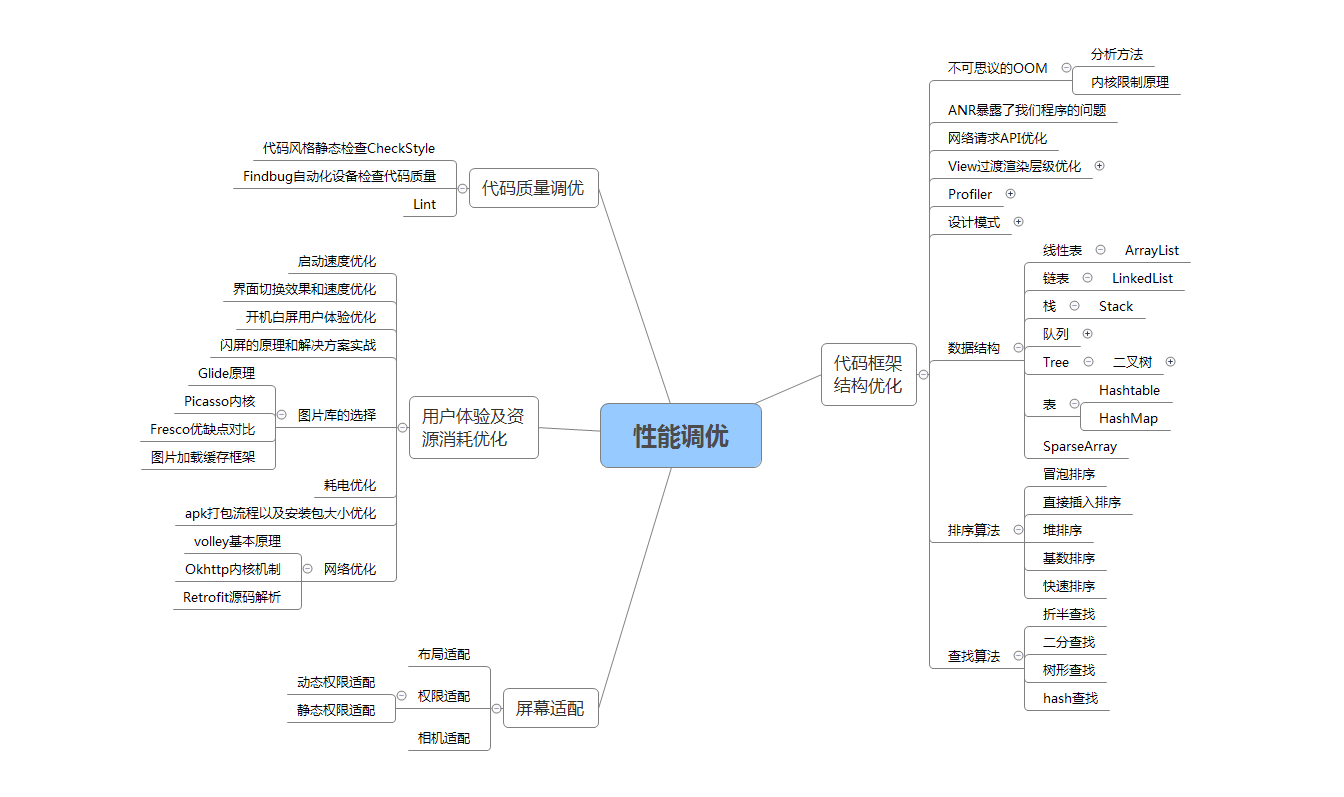 **NDK development;** The future direction, high salary will be. > 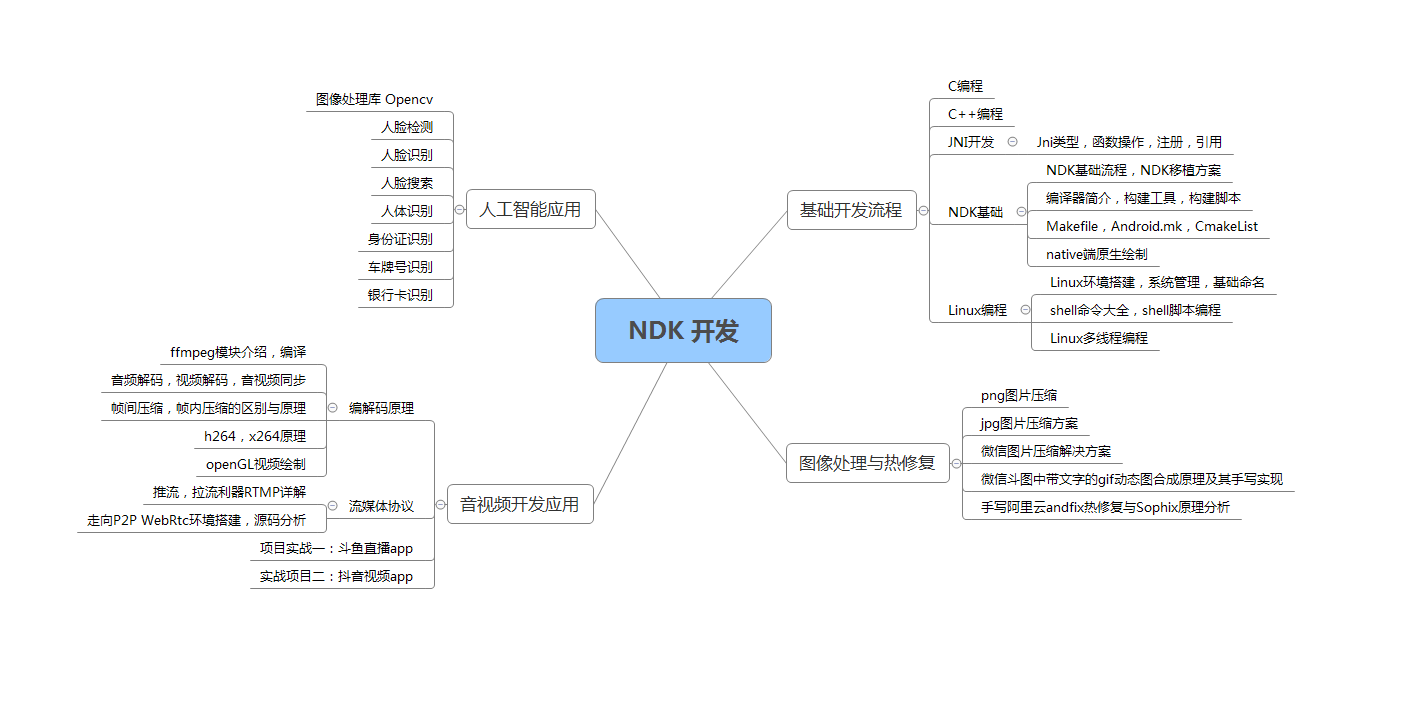 **Cutting edge technology;** Componentization, thermal upgrade, thermal repair, frame design > 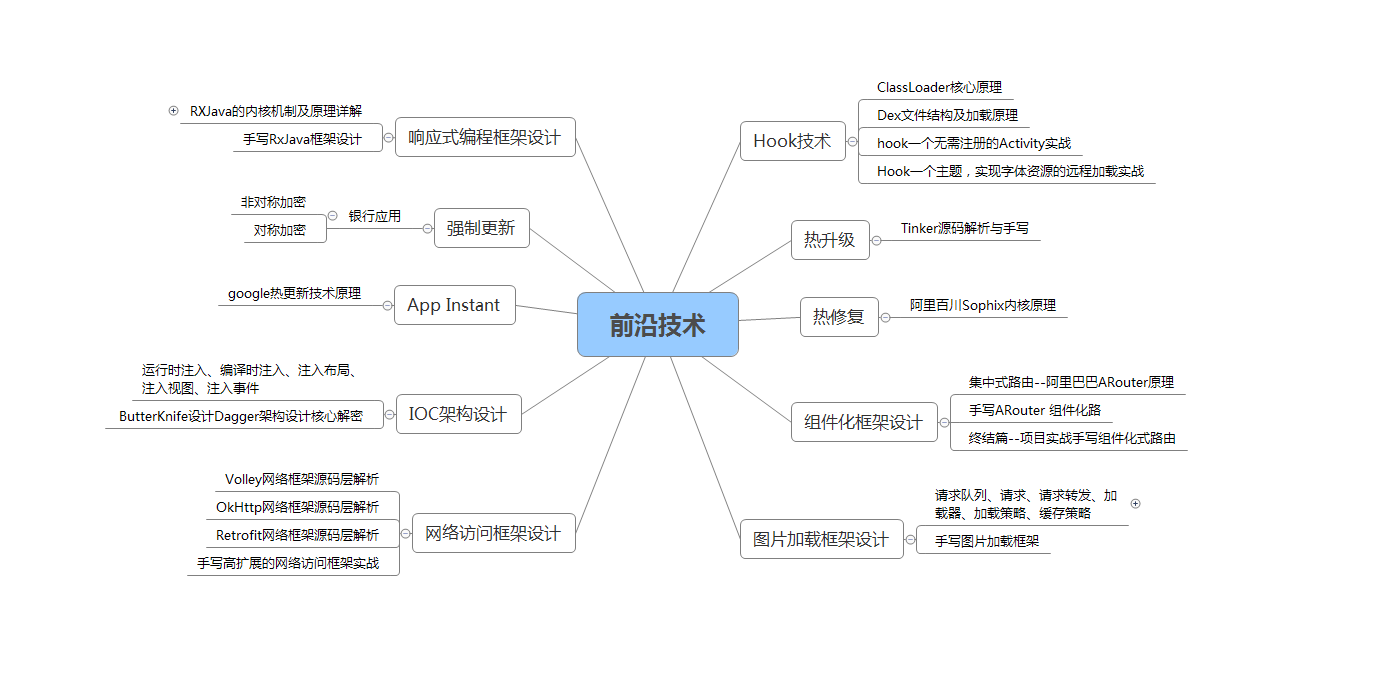 > Online learning Android There are a lot of materials, but if the knowledge learned is not systematic, only a superficial taste when encountering problems and no further research, it is difficult to achieve real technical improvement. I hope this systematic technical system has a direction reference for you. When I built these technical frameworks, I also sorted out the advanced tutorials of the system, which will be much more effective than my fragmented learning, CodeChina so **[CodeChina Open source project address: https://codechina.csdn.net/m0_60958482/android_p7](https://codechina.csdn.net/m0_60958482/android_p7)** Of course, it is not easy to learn and master these abilities. We all know how to learn and what the work intensity of being a programmer is, but no matter how busy the work is, we should still set aside two hours a week to study. **Within six months, you can see the change!** In fact, it is difficult to achieve real technological improvement. I hope this systematic technical system has a direction reference for you. When I built these technical frameworks, I also sorted out the advanced tutorials of the system, which will be much more effective than my fragmented learning, CodeChina so **[CodeChina Open source project address: https://codechina.csdn.net/m0_60958482/android_p7](https://codechina.csdn.net/m0_60958482/android_p7)** Of course, it is not easy to learn deeply and master these abilities. We all know how to learn and what the work intensity of being a programmer is, but no matter how busy the work is, we should still set aside two hours a week to study. **Within six months, you can see the change!**