Text outline
1. What is gradle
2. The characteristics of groovy language and its relationship with java
3. Why is your apk packing so slow
4. How to use gradle programming to solve practical problems in work
5. Advanced usage of gradle (gradle multi-channel quick packaging plug-in)
text
1. What is gradle?
Let's take a look at the explanation from Baidu Encyclopedia:
Gradle is an open source tool for project automation based on the concepts of Apache Ant and Apache Maven. It uses a Groovy based domain specific language (DSL) to declare project settings. At present, it also adds a Kotlin based DSL based on Kotlin language, abandoning various cumbersome configurations based on XML.
Mainly for Java applications. Currently, its supported languages are limited to Java, Groovy, Kotlin and Scala, and it is planned to support more languages in the future
Gradle is a JVM based build tool. It is a general and flexible build tool. It supports maven and Ivy warehouses and transitive dependency management without remote warehouses or POMs XML and Ivy XML configuration file, based on Groovy, and the build script is written in Groovy.
You know, Baidu Encyclopedia is to explain a simple thing that ordinary people can't understand. So let's take a look at the key points in the above paragraph.
Gradle is a Jvm based project automation build tool that can be programmed using Java, groovy, kotlin and scala languages. It is a DSL domain specific language, which is specifically used to build projects
Read and expand:
- Gradle is a tool used to build projects, which is reflected in the project directory of Android studio. It helps us to generate one or more apk files through a series of steps for the java source code files, resource files, dependency packages, common configuration files, etc. in the Android project. With apk files, we can publish them in the application market.
- Gradle is a programming framework, and Groovy is the common programming language of the framework. Since it is a language, you can write program logic. After writing, it will be compiled into bytecode and handed over to the JVM for operation.
- Besides gradle, there are also ant and maven, but they are rarely used and have gradually changed to gradle
The traces of Gradle in the Android studio project are shown in the following figure (this is a new project):
In the above figure, I marked 1-6. Now let's explain them one by one:
1. Gradle- Wrapper
The package box of gradle contains the jar package of gradle and the properties attribute Jar's bag contains class file, which explains why gradle's code is based on the JVM. It can be predicted that this jar package must contain java classes and various APIs generated after gradle compilation However, facts have also proved that my guess is not wrong.
In addition to Jar's bag, there is another one properties.
Here are some configuration information of gradle. The last one, distributionUrl, represents the download path of gradle toolkit
2. External build gradle
It is a global build configuration
3. setting.gradle
However, not only the module s to be compiled can be defined here, but also plug-in programming can be carried out.
4. gradle.properties
Global configuration of gradle
5. gradlew and gradlew bat
These two are executable files under different platforms. The former is sh format, Hill file, executable file under linux. The latter is a batch command under window.
###6. module internal build gradle
in summary
Gradle is a construction tool that runs through the whole android project. Gradle can be seen almost everywhere. As a senior developer, if you only know android java code and write xml, but don't know gradle programming, you may be at a loss when you encounter some problems in development (especially architectural problems).
2. The characteristics of groovy language and its relationship with java
Gradle's common programming languages include java, groovy, kotlin, scala, etc., but the most common is Google's default build language, groovy
So, understanding the features of groovy language can't be bypassed.
However, for developers who are proficient in (familiar with) Java, groovy and Java are based on the jvm and are compiled into bytecode to run in the jvm. On the basis of having a good background in Java, we don't need to learn the syntax word by word, but only need to summarize the parts that are different in the syntax between groovy and Java, so we can easily master it.
First give the official website of Groovy syntax:
https://www.w3cschool.cn/groovy/groovy_basic_syntax.html
As java developers, we can understand most of the syntax, but we need to use our brains for the following.
1. The comma at the end of the statement can be omitted
But if you want to add it, you won't report an error. I suggest you save it if you can
2. Guide Package
Groovy guides packages in the same way as java: import java Lang. *, but There will be a default guide package in gradle. Each XXX Gradle files, can
Instead of importing packages, use the following package classes directly.
import java.lang.* import java.util.* import java.io.* import java.net.* import groovy.lang.* import groovy.util.* import java.math.BigInteger import java.math.BigDecimal
3. Define variables
In addition to String a = "aaa" supported by java, it can also def a = "aaaa"
String s = "aaaaa" def s2 = "ssssss"
4. Circulation
for (int i in 1..10) {//Use for in to traverse 1 to 10 (including head and tail) println i } def dd= [1,2,3,4,5]//for in traversal array for(d in dd){ println d } def map = ["ken":21,"ju":12,"aaaaa":223] // for in traversal map for(d in map){ println d }
In addition, if you simply execute the same piece of code multiple times, you can also write this (this involves closures, which will be explained later):
10.times { variable -> println variable }
5. File IO
In addition to using java's standard IO stream to read and write files, Groovy can also be used to read and write files. The latter is simpler such as
new File("test.txt").eachLine { line -> println "line $line" } //Read each line //Read all contents of the file File f = new File("test.txt") println "All contents of the document \n$f.text" //Write the helloWorld string to test Txt file new File("test.txt").withWriter('utf-8', { writer -> writer.writeLine("hello world") })
In a word, the File class provided by Groovy can perform simple File operations, which is enough to meet our usual gradle programming IO stream operations. You can read more APIs https://www.w3cschool.cn/groovy/groovy_file_io.html
6. Closure
As a result, some people can't understand it The reason for gradle file is closure, which is a concept that does not exist in java (ramda expression is similar to closure, but not closure) It can be roughly understood as: closure = = anonymous code block
//Closure test def clo = { println("Nonparametric closure") }//Nonparametric closure clo() def clo1 = { a -> println("1 Closure of arguments:" + a) } clo1("parameter") def clo2 = { a, b, c -> println("Closure of multiple parameters $a $b $c") } clo2(1,2,3)
Closures can also be passed to a method as parameters:
task test() { //Closure test def clo = { println("Nonparametric closure") }//Nonparametric closure def clo1 = { a -> println("1 Closure of arguments:" + a) } def clo2 = { a, b,c -> println("Closure of multiple parameters $a $b $c") } //Closure as parameter testClo(clo) testClo1(clo1) testClo2(clo2) } void testClo(Closure clo) { println("=======Start testing closure 0=======") clo() } void testClo1(Closure clo) { println("=======Start testing closure 1=======") clo("Ha ha ha") } void testClo2(Closure clo) { println("=======Start testing closure 2=======") clo("Ah 1", 2, 3) }
#####Set / map + closure
task test() { def list = [1, 2, 3, 4, 5] list.each { a -> println(a) }//Specifies the parameter name list.each { println(it) } //Use the default parameter name it def map = ["zhou": 22, "li": 30, "han": 40] map.each { a -> println(a) }//Specifies the parameter name map.each { a -> println(a.key + "- "+ a.value) }//Specifies the parameter name map.each { println(it.key + "- "+ it.value) } //Use the default parameter name it }
7. Omit parentheses
In addition to closures, groovy's omission of parentheses is another reason that prevents us from understanding gradle files. So under what circumstances can groovy omit parentheses?
1. Parentheses of all top-level expressions can be omitted
2. When the closure is the last parameter of the top-level expression, the parentheses can be omitted
However, when a function is nested, if the function is parameterless, the parentheses cannot be omitted
what do you mean? The omission of parentheses is often related to closures / method calls. Let's take an actual case:
buildscript { repositories { google() jcenter() } dependencies { classpath 'com.android.tools.build:gradle:3.4.1' // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } }
The above paragraph can't be read at all according to the language habits of java. However, if we complete the brackets below, it will become like this:
buildscript({ repositories({ google() jcenter() }) dependencies({ classpath ('com.android.tools.build:gradle:3.4.1') // NOTE: Do not place your application dependencies here; they belong # summary The above knowledge points include the current mainstream application technologies of Internet enterprises and the advanced architecture knowledge that can make you a "pastry". Almost every note contains actual combat content. **Many people worry that learning is easy to forget. Here is a way to teach you, that is, repeat learning.** For example, if you are studying spring Annotation, suddenly found an annotation@Aspect,I don't know what it's for. You may check the source code or learn through a blog. It takes half an hour to finally understand it. You'll see it again next time@Aspect Now, you're a little depressed. It seems that you learned where you studied last time. You quickly opened the web page and learned it again in five minutes. **[Data collection method: stamp here for free](https://gitee.com/vip204888/java-p7)** From the comparison between half an hour and five minutes, we can find that learning more once is one step closer to mastering knowledge. 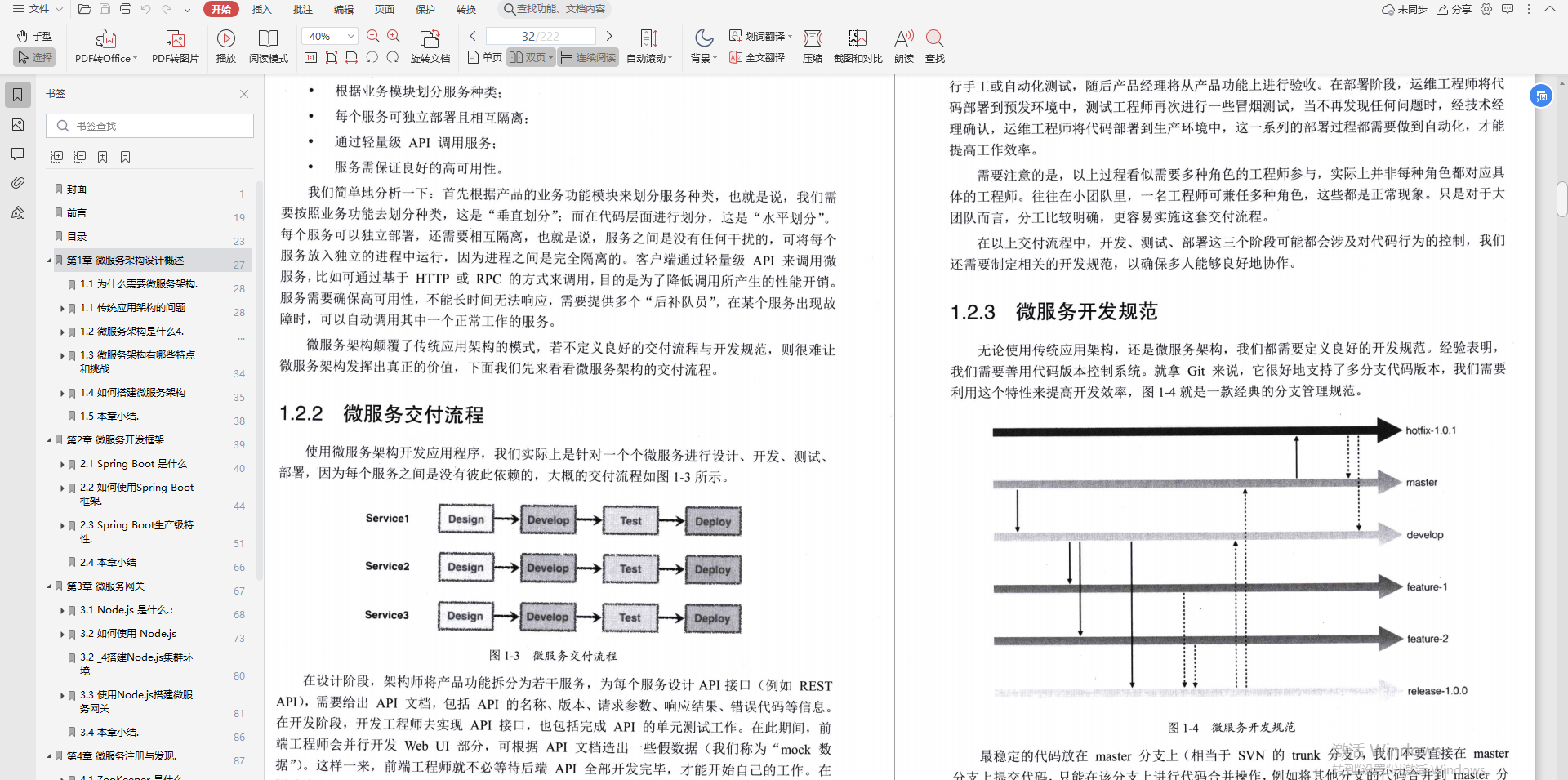 Human nature is easy to forget. Only by constantly deepening the impression and repeated learning can we really master it. Therefore, I recommend you to read many books several times. How can there be so many geniuses? He just read books several times more than you. Where to study, you quickly open the web page and learn it again in five minutes. **[Data collection method: stamp here for free](https://gitee.com/vip204888/java-p7)** From the comparison between half an hour and five minutes, we can find that learning more once is one step closer to mastering knowledge. [External chain picture transfer...(img-td6NlpVj-1628482496020)] Human nature is easy to forget. Only by constantly deepening the impression and repeated learning can we really master it. Therefore, I recommend you to read many books several times. How can there be so many geniuses? He just read books several times more than you.