preface
Last night, I visited GitHub and accidentally saw a P8 big man's algorithm brush notes. I felt that I had found a treasure! Some friends may have found it, but we still can't help Amway. I'm afraid some friends don't see it.
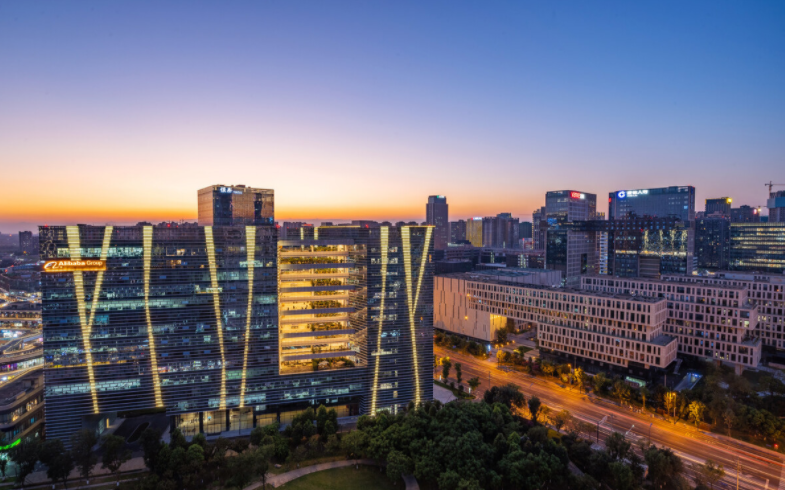
The puzzles and questions about the algorithm are often mentioned by friends. They are often asked in interviews and many businesses, but the algorithm must rely on a solid foundation and the amount of questions. The foundation of the algorithm is not solid. It is not only difficult to interview, but also much weaker than others in improving the performance of the code and controlling the programming language.
Therefore, it is difficult for students with weak algorithm foundation to pass the interview of large factories. However, only by brushing questions to improve the algorithm ability, the progress is too slow, and it is easy to lose focus. With the summary of this note, it is self-evident that it is of great help to the algorithm problem brushing of school recruitment and social recruitment. We decisively collect Amway's complete algorithm notes and think it is good for everyone Amway: 2021 algorithm real problem notes.
Java algorithm
1. Binary search
It is also called half search, which requires the sequence to be searched to be orderly. Each time, the value of the middle position is compared with the keyword to be queried. If the value of the middle position is larger than the keyword to be queried, the search process is cycled in the first half. If the value of the middle position is smaller than the keyword to be queried, the search process is cycled in the second half. Until it is found, there are no keywords to be checked in the sequence.
public static int biSearch(int []array,int a){ int lo=0; int hi=array.length-1; int mid; while(lo<=hi){ mid=(lo+hi)/2;//Middle position if(array[mid]==a){ return mid+1; }else if(array[mid]<a){ //Find right lo=mid+1; }else{ //Find left hi=mid-1; } } return -1; }
2. Bubble sorting algorithm
(1) Compare the two adjacent data before and after. If the previous data is greater than the subsequent data, exchange the two data.
(2) In this way, after traversing the 0th data to n-1 data of the array, the largest data will "sink" to the N-1 position of the array.
(3) N=N-1. If n is not 0, repeat the previous two steps, otherwise the sorting is completed.
public static void bubbleSort1(int [] a, int n){ int i, j; for(i=0; i<n; i++){//Represents n sorting processes. for(j=1; j<n-i; j++){ if(a[j-1] > a[j]){//If the preceding number is greater than the following number, it will be exchanged //Exchange a[j-1] and a[j] int temp; temp = a[j-1]; a[j-1] = a[j]; a[j]=temp; } } } }
3. Insert sort algorithm
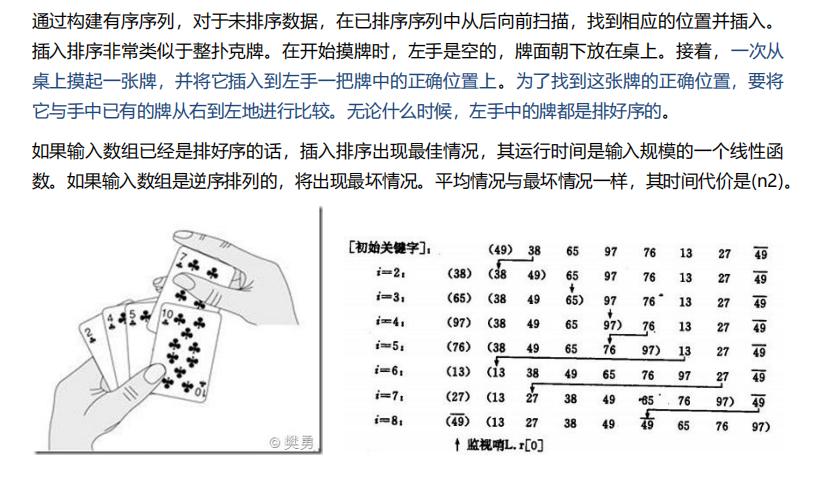
public void sort(int arr[]) { for(int i =1; i<arr.length;i++) { //Number of inserts int insertVal = arr[i]; //Position inserted (ready to compare with the previous number) int index = i-1; //If the number inserted is smaller than the number inserted while(index>=0&&insertVal<arr[index]) { //Will move arr[index] backward arr[index+1]=arr[index]; //Move the index forward index--; } //Put the inserted number in place arr[index+1]=insertVal; } }
4. Quick sorting algorithm
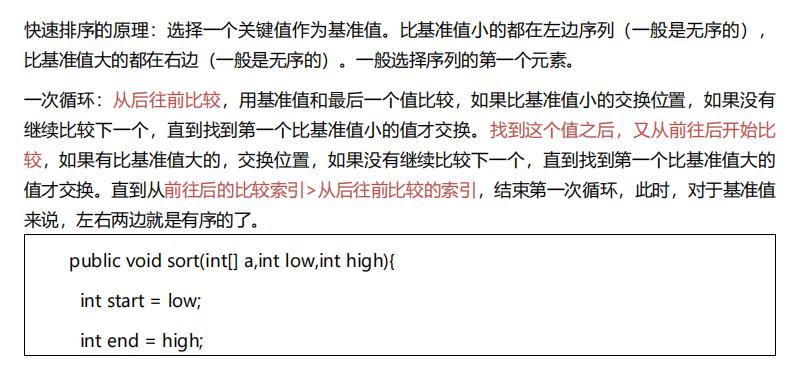
5. Hill sorting algorithm
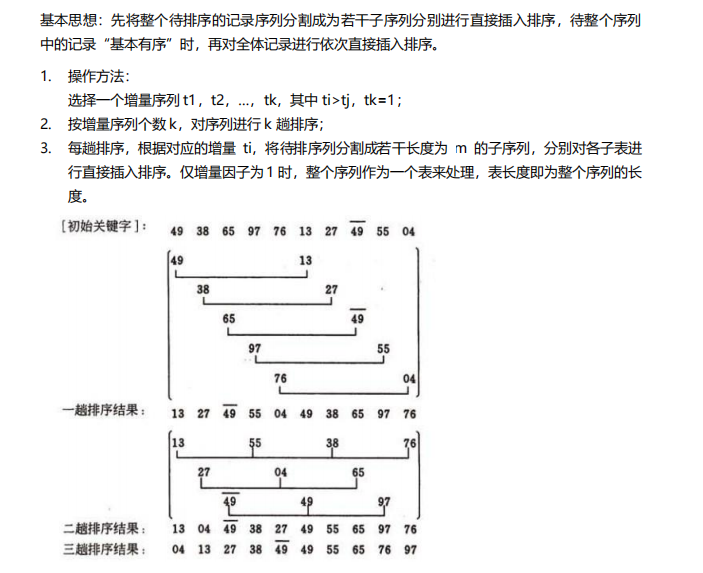
6. Merge sort algorithm
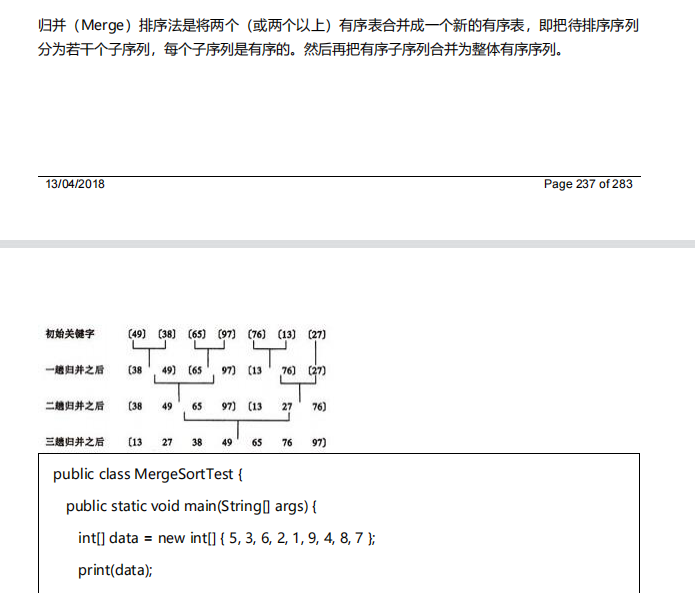
7. Bucket sorting algorithm

8. Cardinality sorting algorithm
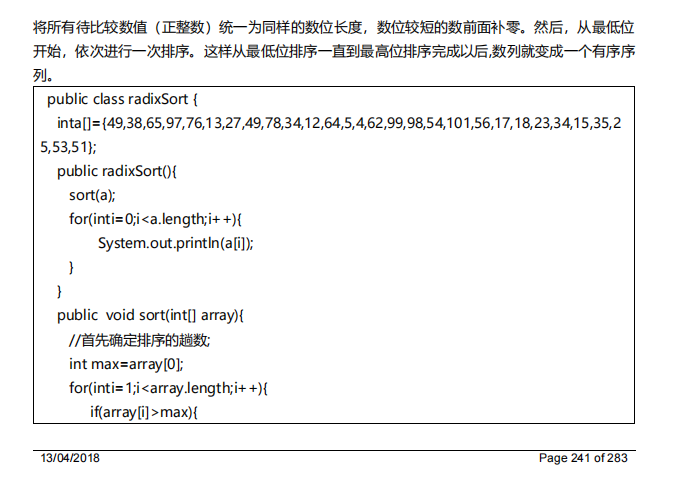
9. Backtracking algorithm
Backtracking algorithm is actually a search attempt process similar to enumeration. It is mainly to find the solution of the problem in the search attempt process. When it is found that the solution conditions are not met, it will "backtrack" back and try other paths.
10. Pruning algorithm
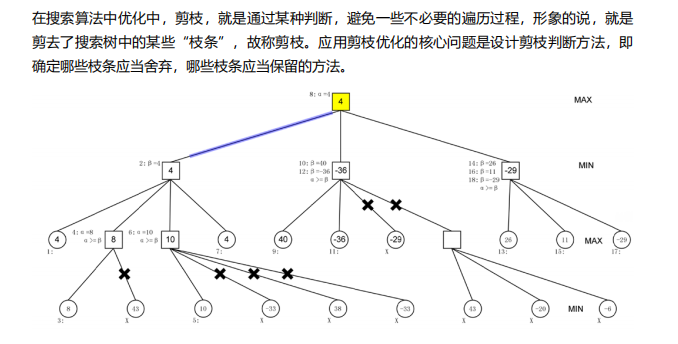
11. Minimum spanning tree algorithm
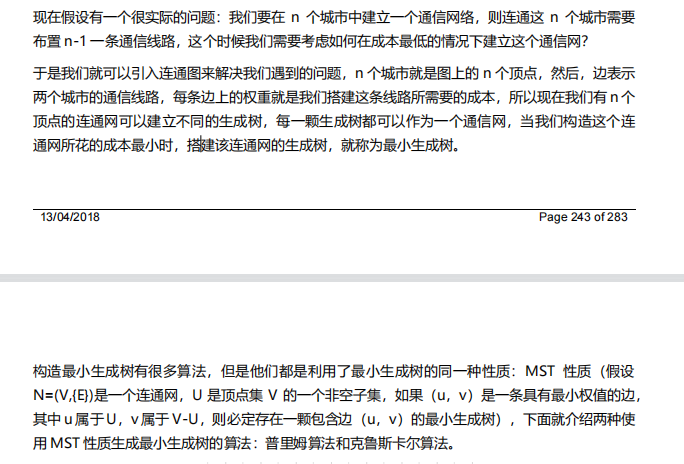
encryption algorithm
1,AES
Advanced encryption standard is the most common symmetric encryption algorithm (this encryption algorithm is used for encrypted transmission of wechat applet). Symmetric encryption algorithm uses the same key for encryption and decryption. The specific encryption process is as follows
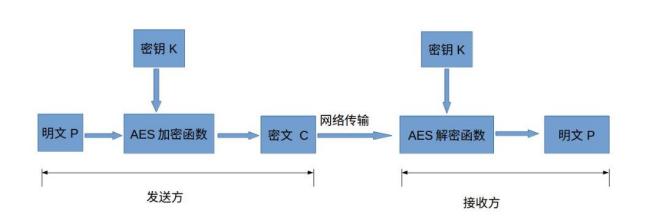
2,RSA
RSA encryption algorithm is a typical asymmetric encryption algorithm. It is based on the factorization of large numbers. It is also the most widely used asymmetric encryption algorithm
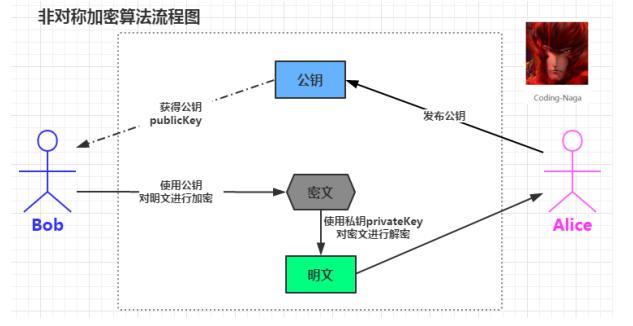
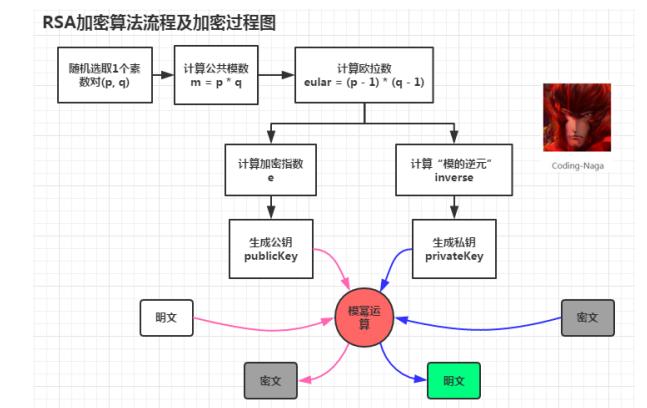
3,CRC
Cyclic redundancy check (CRC) is a hash function that generates a short fixed digit check code according to data such as network data packets or computer files. It is mainly used to detect or verify possible errors after data transmission or storage
4,MD5
MD5 often appears as a file signature. When downloading a file, we often see a file page with an extension of MD5 text or a line of characters. This line of characters is the value calculated by taking the whole file as the original data through MD5. After downloading the file, we can use the software to check the MD5 information of the file to calculate the downloaded file. Two results
Force deduction algorithm
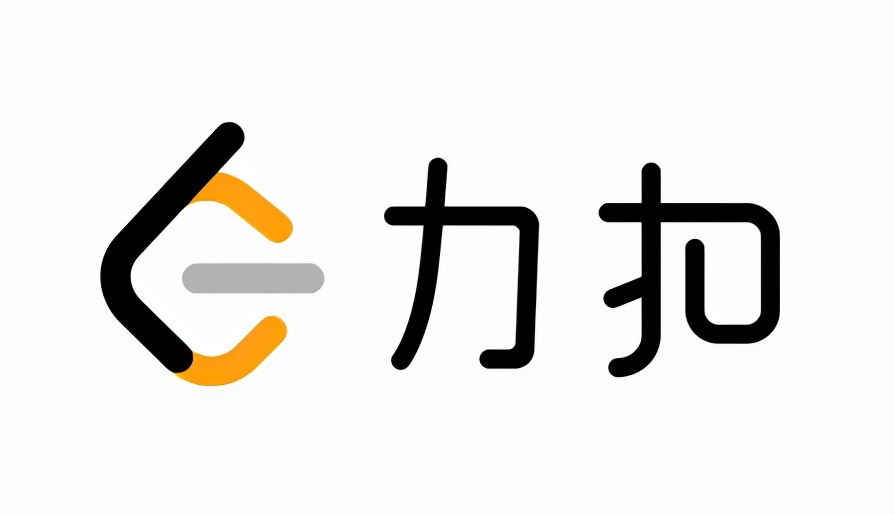
1. Reverse linked list=
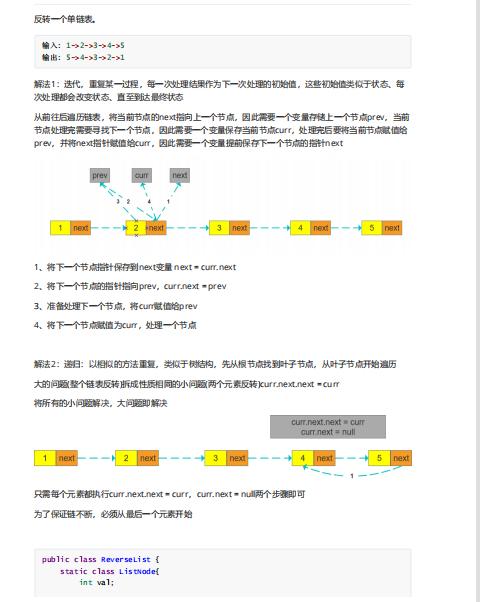
2. Count prime numbers within N

3. Find the central index of the array
If a subscript in the array is equal after the left and right elements, the subscript is the central index
Idea: first count the sum of the whole array, and then stack from the first element. The sum decreases the current element, and the stack increases the current element. You know that the two values are equal
public static int pivotIndex(int[] nums) { int sum1 = Arrays.stream(nums).sum(); int sum2 = 0; for (int i = 0; i<nums.length; i++){ sum2 += nums[i]; if(sum1 == sum2){ return i; } sum1 = sum1 - nums[i]; } return -1; }
4. Remove duplicates from the sort array
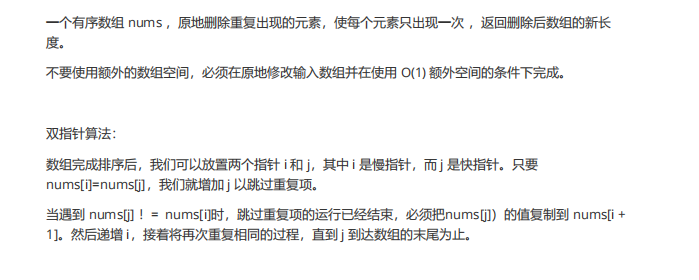
public int removeDuplicates(int[] nums) { if (nums.length == 0) return 0; int i = 0; for (int j = 1; j < nums.length; j++) { if (nums[j] != nums[i]) { i++; nums[i] = nums[j]; } } return i + 1; }
5. Square root of x
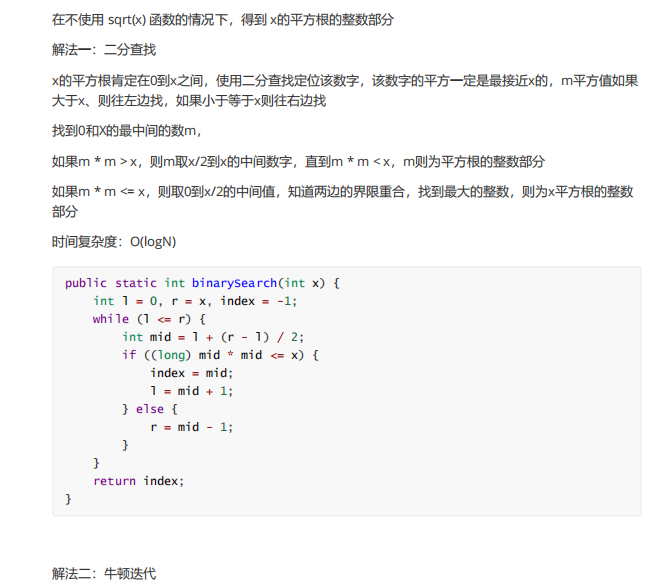
6. Maximum product of three numbers

7. Sum of two numbers
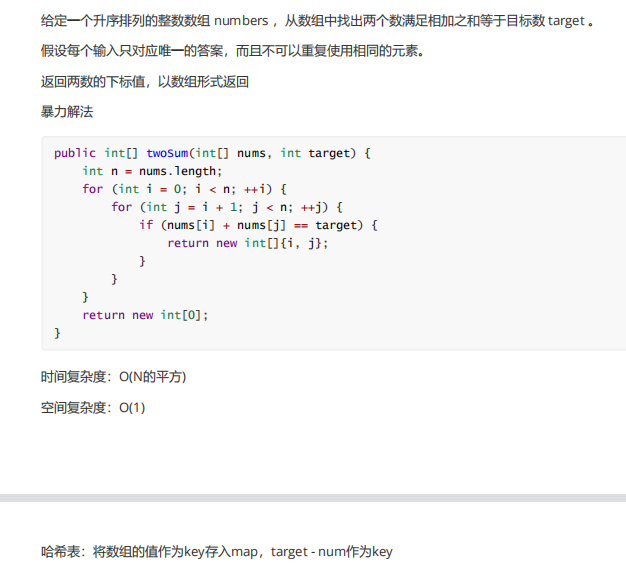
8. Fibonacci sequence
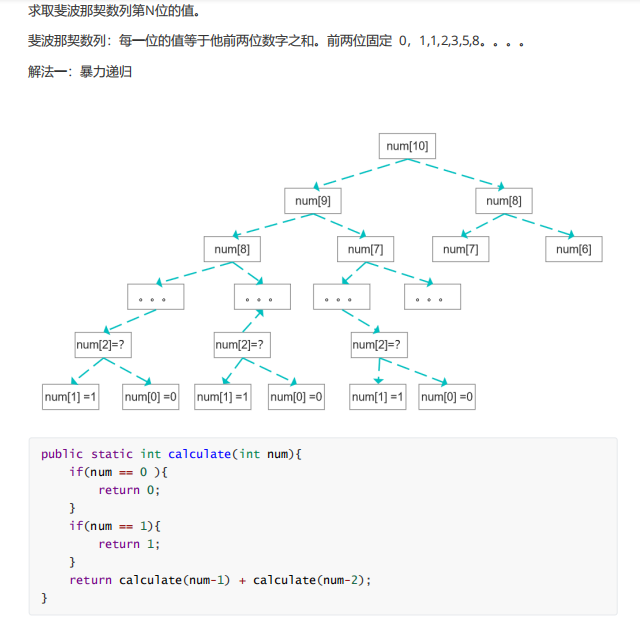
9. Circular linked list
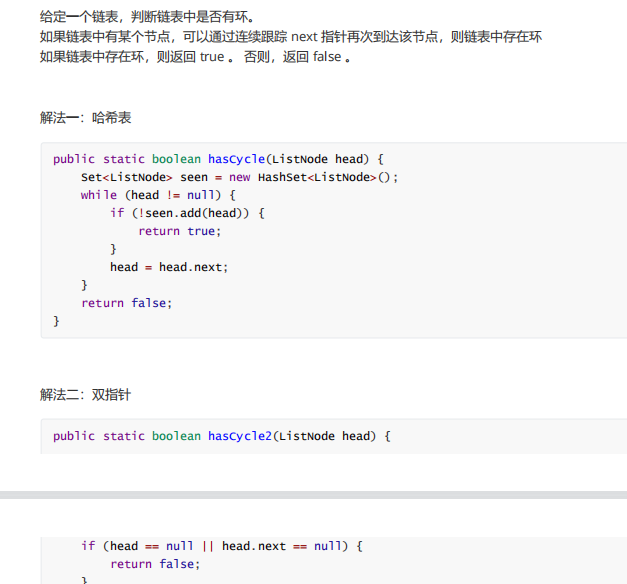
10. Arrange coins
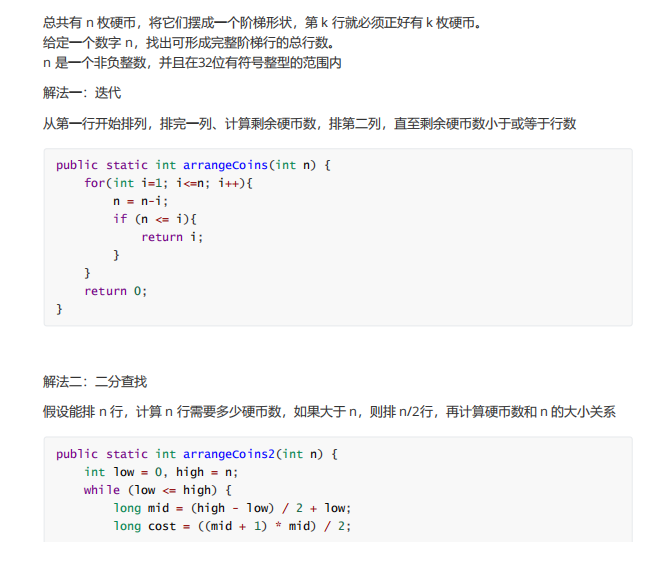
11. Number of provinces
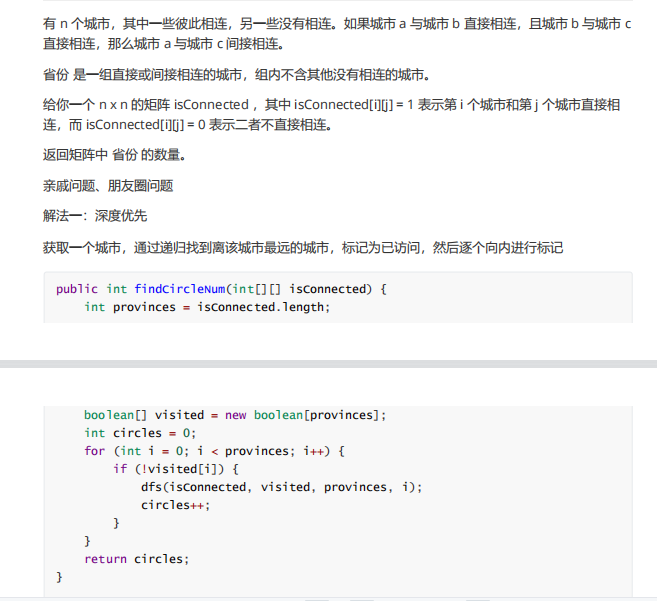
12. Forecast winner
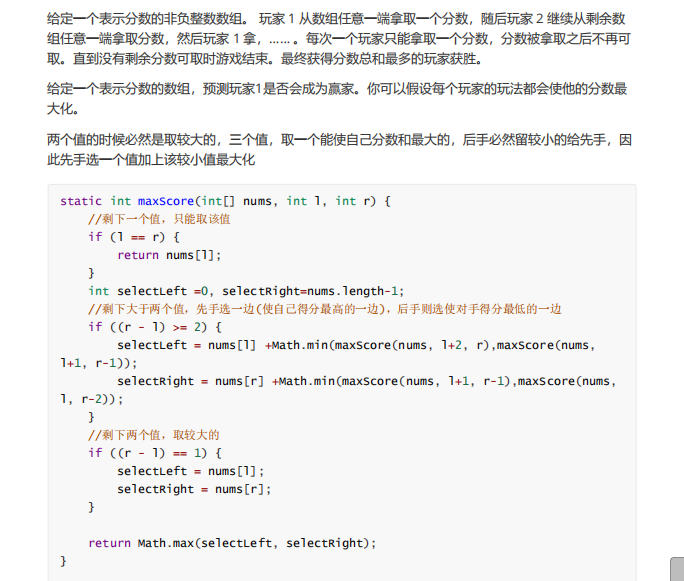
13. Champagne tower
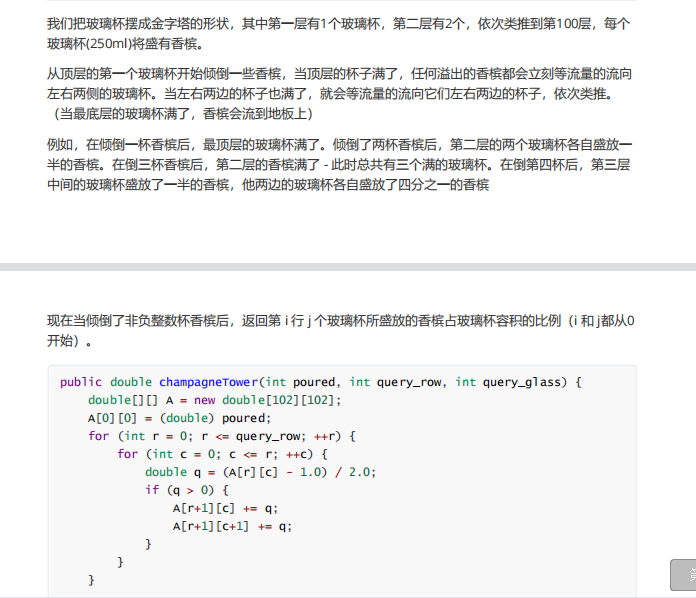
14. Tic tac toe
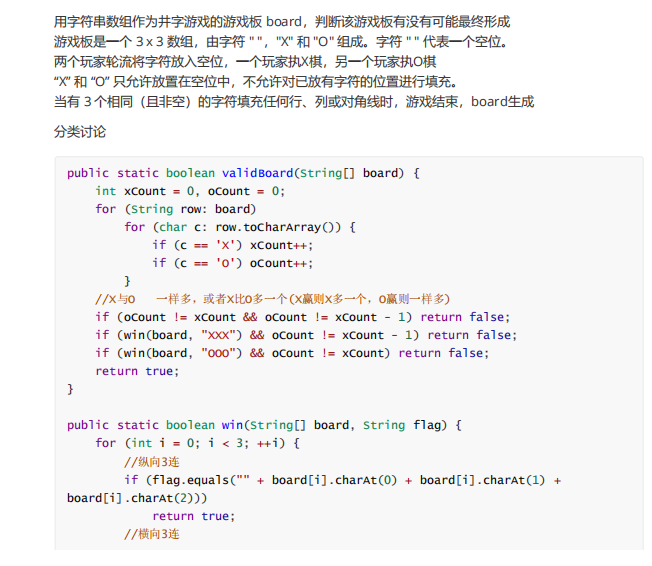
15. Looting
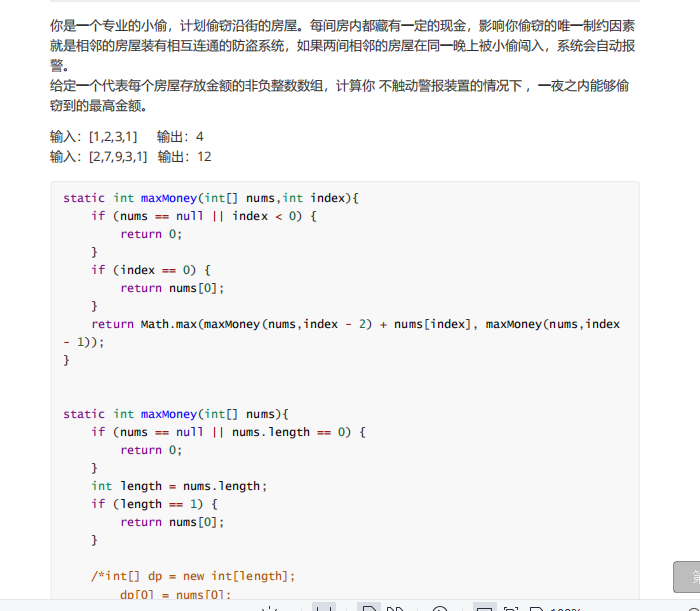
16. Advantage shuffle
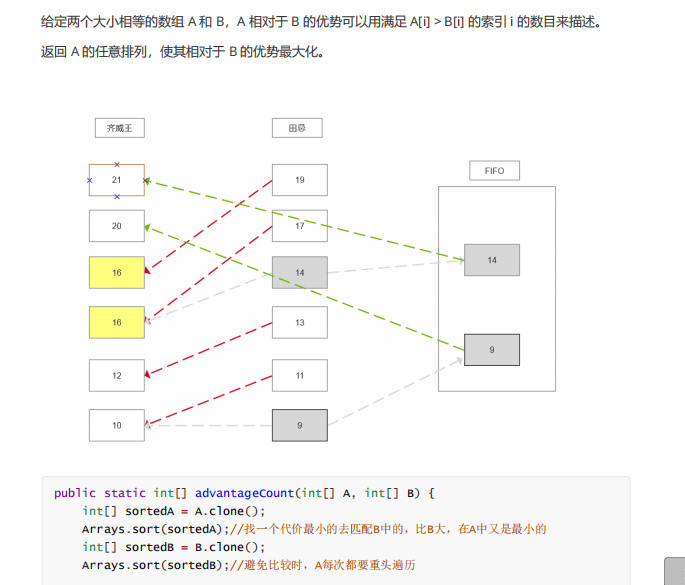
summary
In fact, many algorithm problems are not difficult in themselves. What is difficult is the detail logic. If there are no notes or explanations on these details, you may not want to understand them for several days. Once they are pointed out by others, you may be able to understand them in a few minutes. Therefore, collecting learning notes and learning together are very good learning methods, It can greatly improve their learning efficiency. Complete algorithm notes think it's good for Amway: 2021 algorithm real problem notes.