4. Enable threads to modify time
5. Thread synchronization to achieve stopwatch function
6. Run and debug
Hello, everyone, I'm the grey ape!
Today I share with you an electronic watch project developed using Java multithreading, which can realize the real-time display and modification of time in the electronic watch and the function of stopwatch.
The design sequence of Java spreadsheet design is from front-end interface to back-end class and thread design, and then the front and rear ends are combined. The following is the development process of electronic watch:
1. Front end interface design
=========
The front-end interface of the electronic watch is designed according to the JFrame form and Container, and the absolute positioning method is adopted to reasonably layout the controls such as time display, time modification and stopwatch display, so as to strive for beautiful and concise interface.
2. Add event listener for control
===========
After the interface design is completed, the work is to add function listening to the corresponding controls. Here, the ActionListener interface is called, and the actionPerformed method is rewritten. Listening is added to the three buttons "confirm modification", "start stopwatch" and "pause", and events are added to the corresponding listening, So that the corresponding event can be triggered when the button is clicked. The following is a rewrite of the actionPerformed method
@Override public void actionPerformed(ActionEvent e) { // If the confirm modification button is clicked if (e.getSource() == amend_JB) { //Gets the value of the drop-down box String hour_amend = hourAmend.getSelectedItem().toString(); String minute_amend = minuteAmend.getSelectedItem().toString(); String second_amend = secondAmend.getSelectedItem().toString(); //JOptionPane.showMessageDialog(null, "modification succeeded!"); isThreadShow = false; //Set the thread flag to False and abort the thread //Displays the modified value hourShow.setText(hour_amend); minuteShow.setText(minute_amend); secondShow.setText(second_amend); System.out.println("The modification time is:" + hour_amend + ":" + minute_amend + ":" + second_amend); threadAmend.start();//Start the thread with the modified run time } //If you click the button to start the stopwatch if (e.getSource() == second_JB) { //If the current stopwatch is in the start state, that is, it shows to stop timing if (second_JB.getText() == "Stop timing") { second_JB.setText("Start the stopwatch"); second_JB.setBackground(Color.yellow); //threadSecond.stop(); isStartSecond = false; } else { //If the current stopwatch is off second_JB.setText("Stop timing"); second_JB.setBackground(Color.RED); threadSecond.start();//Start stopwatch thread isStartSecond = true; } } //If the pause button is clicked if (e.getSource() == pause_JB) { if (pause_JB.getText() == "suspend") { pause_JB.setText("continue"); pause_JB.setBackground(Color.cyan); threadSecond.suspend(); //Pause the thread } else { pause_JB.setText("suspend"); pause_JB.setBackground(Color.RED); threadSecond.resume(); //Make the thread continue } } }
3. Real time display of time through the main thread
==============
After the listening function is added to the button control, the current time is displayed. The time is displayed by using the main thread, and the data is updated and displayed every second in the main thread. Here, the Date class is used to read the system time, and then the obtained time is normalized by using SimpleDateFormat. After that, the year, month The day, week and current time are displayed in the interface,
@Override public void run() { while (true) { // TODO Auto-generated method stub Date date = new Date(); //Instantiate time class objects //Normalize the currently acquired time SimpleDateFormat yearFormat = new SimpleDateFormat("yyyy"); SimpleDateFormat monthFormat = new SimpleDateFormat("MM"); SimpleDateFormat dayFormat = new SimpleDateFormat("dd"); SimpleDateFormat weekFormat = new SimpleDateFormat("EEEE"); SimpleDateFormat hourFormat = new SimpleDateFormat("HH"); SimpleDateFormat minuteFormat = new SimpleDateFormat("mm"); SimpleDateFormat secondFormat = new SimpleDateFormat("ss"); //Get the current year, month, day, hour, minute and second year = yearFormat.format(date); month = monthFormat.format(date); day = dayFormat.format(date); week = weekFormat.format(date); hour = hourFormat.format(date); minute = minuteFormat.format(date); second = secondFormat.format(date); hourShow.setText(hour); minuteShow.setText(minute); secondShow.setText(second); timeShow.setText(year + "year" + month + "month" + day + "day" + week); try { Thread.sleep(1000); //Let the thread execute every second } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } //If the thread aborts, it jumps out of the loop if (!isThreadShow) { break; } } }
4. Enable threads to modify time
==============
After displaying the system time, it is the operation of modifying the current time. The design idea here is to first obtain the modified time, then use break to jump out of the loop of the main thread, so as to end the operation of the main thread, and then display the read modified time in the label of displaying time, At the same time, start the threadAmend thread that continues to run after modification, and update it every second, so that the time can continue to execute on the basis of modification. The effect is as follows:
//Set threadAmend thread threadAmend = new Thread(new Runnable() { @Override public void run() { while (true) { int hourAmend = Integer.parseInt(hourShow.getText()); int minuteAmend = Integer.parseInt(minuteShow.getText()); int secondAmend = Integer.parseInt(secondShow.getText()); secondAmend++; //Judge and deal with the obtained time if (secondAmend == 60) { minuteAmend++; secondAmend = 0; } if (minuteAmend == 60) { hourAmend++; minuteAmend=0; } if (hourAmend == 24) { hourAmend = 0; minuteAmend = 0; secondAmend = 0; } //Display time hourShow.setText(Integer.toString(hourAmend)); minuteShow.setText(Integer.toString(minuteAmend)); secondShow.setText(Integer.toString(secondAmend)); try { threadAmend.sleep(1000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } # summary **That's it. It can be regarded as a summary of the interview during this period. I'll check the leaks and fill the vacancies. I wish myself good luck. I also hope that programmers who are looking for a job or intend to change jobs can see a little help or harvest from this article, and I'm satisfied. Think more and ask more why. I hope you will receive a satisfactory reply as soon as possible offer! The harder you work, the luckier you will be!** **The golden nine silver ten has passed. In terms of the current domestic interview mode, actively prepare for the interview and review the whole interview before the interview Java The knowledge system will become very important. It can be said responsibly that whether the review preparation is sufficient will directly affect your success rate. But many small partners suffer from not having the right data to review the whole process Java Knowledge system, or some small partners may not know where to review. I came across a collated information, whether from the whole Java Knowledge system, or from the perspective of interview, is a material with high technical content.** **[Interested friends can click here for free!](https://gitee.com/vip204888/java-p7)** 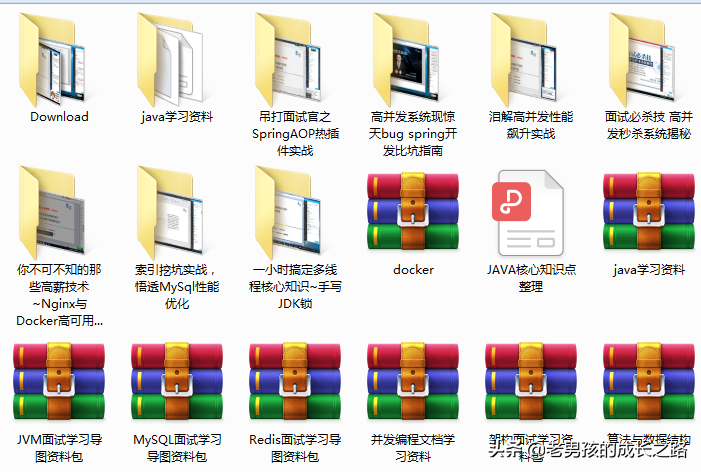 I am satisfied to see that this article can help or harvest a little. Think more and ask more why. I hope you will receive a satisfactory reply as soon as possible offer! The harder you work, the luckier you will be!** **The golden nine silver ten has passed. In terms of the current domestic interview mode, actively prepare for the interview and review the whole interview before the interview Java The knowledge system will become very important. It can be said responsibly that whether the review preparation is sufficient will directly affect your success rate. But many small partners suffer from not having the right data to review the whole process Java Knowledge system, or some small partners may not know where to review. I came across a collated information, whether from the whole Java Knowledge system, or from the perspective of interview, is a material with high technical content.** **[Interested friends can click here for free!](https://gitee.com/vip204888/java-p7)** [External chain picture transfer...(img-1Tf2RwT4-1628152905373)]