With the spring recruit approaching, it is always of great benefit to prospective graduates looking for a job or programmers who want to improve their own algorithms, internal mental skills and python programming ability in the workplace. Today, red stone found a good resource: a collection of interview questions implemented in Python!
This resource is called: Interview code practice Python! It contains hundreds of algorithmic interview questions, and all the answers are written in Python. With questions and answers, isn't it fast to learn~
Well, don't say much, just put the GitHub address of this interview collection:
https://github.com/leeguandong/Interview-code-practice-python
This project resource contains five real questions in total, namely: 2017 school recruitment real question, sword finger offer, Huawei machine test, machine test question and straight through BAT algorithm question.
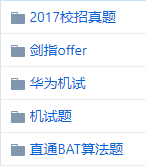
Next, let's take a look at the specific contents.
1. 2017 school enrollment
This part contains 37 real school recruitment questions in 2017.
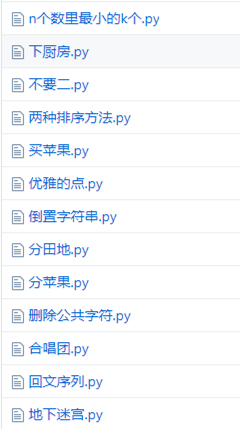
Each topic is equipped with a corresponding Python implementation. For example, let's take an interesting example: a restaurant py
# Select n batches from m batches of guests and set n tables n, m = [int(x) for x in input().split()] # Maximum number of people per table table = [int(x) for x in input().split()] # Respectively represent the number of guests in the i batch and the estimated consumption amount cus = [[int(x) for x in input().split()] for i in range(m)] # Arrange the tables according to the number of people you can hold from small to large table.sort() # Sort by the number of customers in each batch from small to large cus = sorted(cus, key=lambda x: x[0]) # Total amount money = 0 for i in range(n): # Hold the i th acceptable customer batch j temp = [] # Note that the length of cus is updated in the range for j in range(len(cus)): if cus[j][0] > table[i]: break temp.append(cus[j]) # Sort by consumption amount temp = sorted(temp, key=lambda x: x[1]) if temp: money += temp[-1][1] # This group of guests are already seated cus.remove() print(money)
2. Sword finger offer
This part contains 68 sword finger real questions.
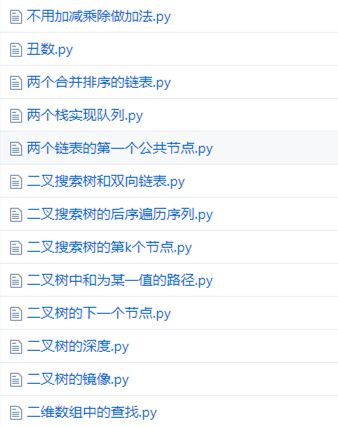
Look at the example: abnormal frog jumping py
''' Title: a frog can jump up 1 step or 2 steps at a time... It can also jump up n Level. Ask the frog to jump on one n How many jumping methods are there in all ''' ''' because n Steps, the first step is n Three jump methods: jump level 1, jump Level 2 and jump to n level Skip level 1, leaving n-1 Level, the remaining jump method is f(n-1) Skip Level 2, leaving n-2 Level, the remaining jump method is f(n-2) therefore f(n)=f(n-1)+f(n-2)+...+f(1) because f(n-1)=f(n-2)+f(n-3)+...+f(1) therefore f(n)=2*f(n-1) Then solve the sum of this infinite series. The correct answer should be: jump at least one at a time and jump at most n Yes, there are f(n)=2n-1 Seed jump method 29ms 5632k ''' # -*- coding:utf-8 -*- class Solution: def jumpFloorII(self, number): # write code here return 2**(number-1)
3. Huawei machine test
This section contains 41 Huawei computer test questions.
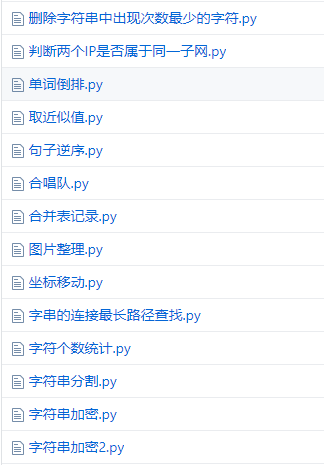
See example: password verification qualification program py
''' 1.Length exceeds 8 bits 2.Include upper and lower case letters.number.Other symbols,At least three of the above four 3.Substrings with the same length exceeding 2 cannot be repeated explain:Substring longer than 2 ''' # import re, sys # # for i in sys.stdin.readlines(): # print("OK" if len(i.strip()) > 8 and sum( # [1 if re.search(r"[A-Z]", i.strip()) else 0, 1 if re.search(r"[a-z]", i.strip()) else 0, # 1 if re.search(r"[0-9]", i.strip()) else 0, 1 if re.search(r"[^0-9a-zA-Z]", i.strip()) else 0]) > 2 and sum( # map(lambda c: i.strip().count(i.strip()[c:c + 3]) > 1, range(1, len(i.strip()) - 3))) == 0 else "NG") # If you think a little, you only need to judge whether the substring with length of 3 appears. Because if the substring length is 4, the substring length of 3 must be included. At the same time, it should be noted that, # The substring in this question refers to the overlapping of some substrings, # For example, Qw11111*ed cannot pass. Another thing to note is that when judging the substring, you only need to judge len(str)-3. import sys try: # Case, letters, def panchar(sss): standard = [0] * 4 for i in sss: # print(i) # 0 # 2 # 1 # A # b # print(len(sss)) # number if i.isdigit(): standard[0] = 1 # print(i.isdigit()) # a lowercase letter if i.islower(): standard[1] = 1 # Capitalize if i.isupper(): standard[2] = 1 # It's all letters, numbers, spaces if not i.isalpha() and not i.isdigit() and not i.isspace(): standard[3] = 1 if sum(standard) >= 3: return False return True # A string with the same length exceeding 2 cannot be repeated def zichuan(sss): for i in range(len(sss) - 3): zichuan_1 = sss[i: i + 3] zichuan_2 = sss[i + 1::] if zichuan_1 in zichuan_2: return True return False result = [] while True: line = sys.stdin.readline().strip() if line == '': break if len(line) <= 8: result.append('NG') # Upper and lower case letters Number Other symbols elif panchar(line): result.append('NG') elif zichuan(line): result.append('NG') else: result.append('OK') for i in result: print(i) except: pass # # Loop input, try catch # while True: # try: # x = input().split() # # # except: # pass
4. Machine test questions
This section contains 3 machine test questions.
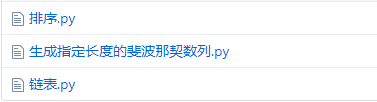
See example: sorting py
# https://blog.csdn.net/u012193416/article/details/78790448 # # Bubble sorting # # Time complexity O(n**2) space complexity O(1) # x = [int(i) for i in input().split(',')] # # # print(x) # # def mpsort(x): # n = len(x) # # print(n) # for i in range(n - 1): # for j in range(0, n - i - 1): # # print(x[j]) # if x[j] > x[j + 1]: # x[j], x[j + 1] = x[j + 1], x[j] # return x # # print(mpsort(x)) # # Select sort # # Time complexity O(n**2) space complexity O(1) # x = [int(i) for i in input().split(',')] # # def xzsort(x): # n = len(x) # for i in range(n - 1): # min = i # for j in range(i + 1, n): # if x[j] < x[min]: # min = j # x[i], x[min] = x[min], x[i] # return x # # print(xzsort(x)) # # Insert sort # # Time complexity O(n**2) space complexity O(1) # x = [int(i) for i in input().split(',')] # # def crsort(x): # n = len(x) # for i in range(1, n): # j = i # while j > 0: # if x[j] < x[j - 1]: # x[j], x[j - 1] = x[j - 1], x[j] # j -= 1 # else: # break # return x # # print(crsort(x)) # # Shell Sort # # Time complexity O(nlogn)-O(n**2) space complexity O(1) # x = [int(i) for i in input().split(',')] # # def shellsort(x): # n = len(x) # gap = n // 2 # # while gap > 0: # for i in range(gap, n): # j = i # while j > 0: # if x[j] < x[j - gap]: # x[j], x[j - gap] = x[j - gap], x[j] # j -= gap # else: # break # gap //= 2 # return x # # print(shellsort(x)) # # Quick sort # # Time complexity O(nlogn) space complexity O(logn)-O(n) # x = [int(i) for i in input().split(',')] # # def kpsort(x, first, last): # font = first # end = last # middle = x[first] # # if first >= last: # return # # while font < end: # while font < end and x[font] <= middle: # font += 1 # x[end] = x[font] # # while font < end and x[end] > middle: # end -= 1 # x[font] = x[end] # # x[font] = middle # # kpsort(x, first, font - 1) # kpsort(x, font + 1, last) # Merge sort # Time complexity O(nlogn) space complexity O(N) x = [int(i) for i in input().split(',')] def gbsort(x): length = len(x) if length <= 1: return x mid = length // 2 left = gbsort(x[:mid]) right = gbsort(x[mid:]) left_point, right_pointer = 0, 0 result = [] while left_point < len(left) and right_pointer < len(right): if left[left_point] <= right[right_pointer]: result.append(left[left_point]) left_point += 1 else: result.append(right_pointer) right_pointer += 1 result += left[left_point:] result += right[right_pointer] return result print(gbsort(x))
5. Straight through BAT algorithm problem
This part contains three blocks:
- Binary tree
- Stack and queue
- Linked list
Let's look at an example: inserting a new node into a ring linked list py
# The pointer gives the node value class Node(): def __init__(self, value=None): self.value = value self.next = None def insertnum(head, num): node = Node(num) if head == None: node.next = node return node node = head pre = node cur = node.next while cur != head: if pre.value > num and cur.value <= num: break pre = pre.next cur = cur.next # num is less than the node value, and pre only runs to the last node, node runway end node pre.next = node node.next = cur # It comes in order, and the returned head or node is determined in order return head if head.value < num else node node = Node() node.insertnum([[1, 2, 3], 5])
Finally, the best way to learn Python is to start with the underlying algorithm and go through the basic functions and structures. With sufficient understanding, you can directly brush LeetCode, etc. I hope this collection of Python interview questions is helpful to you!