Special variables and methods in Flask
In Flask, some special variables and methods can be accessed directly in the template file.
config object:
config The object is Flask of config Object, that is app.config Object. {{ config.SQLALCHEMY_DATABASE_URI }}
request object:
It is the request object in Flask that represents the current request. The request object stores all the information of an HTTP request.
The common attributes of request are as follows:
attribute | explain | type |
---|---|---|
data | Record the requested data and convert it to a string | * |
form | Record the form data in the request | MultiDict |
args | Record the query parameters in the request | MultiDict |
cookies | Record cookie information in the request | Dict |
headers | Record the message header in the request | EnvironHeaders |
method | Record the HTTP method used by the request | GET/POST |
url | Record the URL address of the request | string |
files | Record the file requested to be uploaded | * |
{{ request.url }}
url_for method:
url_for() will return the URL corresponding to the incoming routing function, which is called app Route() is a function decorated by the route decorator. If the routing function we define has parameters, these parameters can be passed in as named parameters.
{{ url_for('index') }} {{ url_for('post', post_id=1024) }}
get_flashed_messages method:
Returns the list of information previously passed in through flash() in flash. Add the message represented by the string object to a message queue, and then call get_flashed_messages() method. The stored message will only be used once, that is, it can be used as the content of the message prompt box.
from flask import flash
# Method of transmitting parameters to Flash flash('Flash message 1') flash('Flash message 2') flash('Flash message 3')
{% for message in get_flashed_messages() %} {{ message }} {% endfor %}
After reading the above conceptual contents, let's take a look at the examples below to show the practical operation.
Sample code
1. Write view function
from flask import Flask, render_template, flash app = Flask(__name__) app.config["SECRET_KEY"] = "xhosd6f982yfhowefy29f" @app.route("/tpl", methods=["GET", "POST"]) def tpl(): # Method of transmitting parameters to Flash flash('Flash message 1') flash('Flash message 2') flash('Flash message 3') return render_template("tpl.html") @app.route('/hello1') def hello1(): return render_template('hello1.html') @app.route('/hello2') def hello2(): return render_template('hello2.html') if __name__ == '__main__': app.run(debug=True)
2. Write the module page TPL html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <p>config object: {{ config.SECRET_KEY }} <br></p> <p>request object: {{ request.url }} <br></p> <p> url_for method: <br> {{ url_for('hello1') }} <br> {{ url_for('hello2') }} <br> </p> </body> </html>
3. Write template page hello1 html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> get_flashed_messages method: <br> {% for message in get_flashed_messages() %} {{ message }} {% endfor %} </body> </html>
4. Write template page Hello 2 html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> get_flashed_messages method: <br> {% for message in get_flashed_messages() %} {{ message }} {% endfor %} </body> </html>
5. Test and view the direct use object of the template, and set the flash message storage
visit http://127.0.0.1:5000/tpl
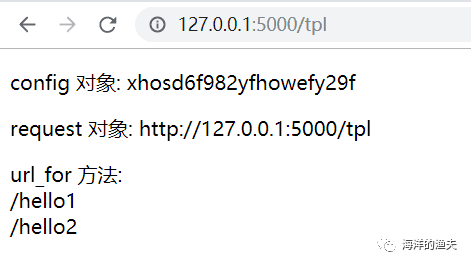
- Accessing hello1 consumption using flash messages
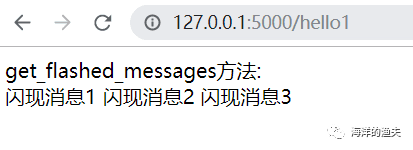
7. Refresh hello1 or visit the hello2 page to check whether the flash message exists
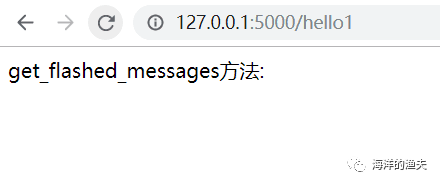
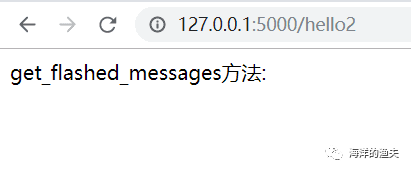
You can see that the flash message will only be displayed once. When refreshing or accessing other views, it will not appear again as long as it is consumed.
Based on this feature of flash, just like messages in Django, it is the most suitable message prompt box for switching pages.