Preliminary knowledge review
SSM review
Mybatis
Spring
spring mvc
Vue's core library only focuses on view layers to facilitate integration with third-party libraries or existing projects.
HTML + CSS + JS: View: show the user and refresh the data given by the background
Network communication: axios
Page Jump: Vue router
Status management: vuex
Vue-UI : ICE , Element UI
1, Front end core analysis
1. VUE overview
Vue (pronounced / vju /, similar to view) is a progressive framework for building user interfaces, which was released in February 2014. Unlike other large frameworks, Vue is designed to be applied layer by layer from bottom to top. Vue's core library only focuses on view layers, which is not only easy to start, but also easy to integrate with third-party libraries (such as Vue Router: jump, Vue resource: communication, vuex: Management) or existing projects
2. Front end three elements
HTML (structure): Hyper Text Markup Language, which determines the structure and content of web pages
CSS (presentation): cascading style sheets to set the presentation style of web pages and design the web page style in language.
JavaScript (behavior): it is a weak type scripting language. Its source code does not need to be compiled, but is interpreted and run by the browser to control the behavior of web pages
3. JavaScript framework
jQuery: the well-known JavaScript framework has the advantage of simplifying DOM operation, but the disadvantage is that DOM operation is too frequent, affecting the front-end performance; In the eyes of the front end, it is only used to be compatible with IE6, 7 and 8;
Angular: the front-end framework acquired by Google was developed by a group of Java programmers. It is characterized by moving the background MVC mode to the front-end and adding the concept of modular development. It is developed in cooperation with Microsoft using TypeScript syntax; Friendly to background programmers, not very friendly to front-end programmers; The biggest disadvantage is that the version iteration is unreasonable (for example, generation 1 - > generation 2, except for the name, there are basically two things; angular6 has been launched as of the time of publishing the blog)
React: produced by Facebook, a high-performance JS front-end framework; The feature is that a new concept [virtual DOM] is proposed to reduce real DOM operations and simulate DOM operations in memory, which effectively improves the front-end rendering efficiency; The disadvantage is that it is complex to use, because you need to learn an additional [JSX] language;
Vue: a progressive JavaScript framework. The so-called progressive means to gradually realize new features, such as modular development, routing, state management and other new features. It combines the advantages of Angular (Modular) and React (virtual DOM);
Axios: front end communication framework; Because the boundary of Vue is very clear, it is to deal with DOM, so it does not have communication capability. At this time, it is necessary to use an additional communication framework to interact with the server; Of course, you can also directly choose to use the AJAX communication function provided by jQuery;
Three front-end frameworks: Angular, React and Vue
2, First Vue program
1. What is MVVM
MVVM (model view ViewModel) is a software architecture design pattern developed by Ken Cooper and Ted Peters, architects of Microsoft WPF (used to replace WinForm, which was used to develop desktop applications) and Silverlight (similar to Java Applet, simply put, WPF running on browser). It is an event driven programming method to simplify the user interface. It was published by John Gossman (also the architect of WPF and Silverlight) on his blog in 2005.
MVVM originates from the classic MVC (modi view controller) mode. The core of MVVM is the ViewModel layer, which is responsible for transforming the data objects in the Model to make the data easier to manage and use. Its functions are as follows:
This layer performs bidirectional data binding with the view layer upward
Data interaction with the Model layer through interface requests
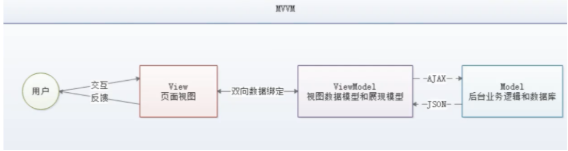
2. Why use MVVM
MVVM mode is the same as MVC mode. Its main purpose is to separate view and model. It has several advantages:
Low coupling: the view can be changed and modified independently of the Model, and a ViewModel can be bound to different views
In View, when the View changes, the Model can remain unchanged, and when the Model changes, the View can remain unchanged.
Reusability: you can put some View logic in a ViewModel to make many views reuse this View logic.
Independent development: developers can focus on the development of business logic and data (ViewModel), and designers can focus on page design.
Testability: the interface is always difficult to test, but now the test can be written for ViewModel.
3. Vue is the implementer of MVVM mode
Model: the model layer, where JavaScript objects are represented
View: view layer, which represents DOM (element of HTML operation)
ViewModel: middleware connecting views and data, Vue JS is the implementer of ViewModel layer in MVVM. In MVVM architecture, data and view are not allowed to communicate directly, but only through ViewModel, which defines an Observer observer
ViewModel can observe the changes of data and update the content corresponding to the view
ViewModel can listen to changes in the view and notify data changes
So far, we understand, Vue JS is an implementer of MVVM. Its core is to realize DOM listening and data binding
Vue online cdn:
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
First vue program
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <!---view Layer template--> <div id="app"> {{message}} </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> var vm=new Vue({ el:"#app", data:{ message:"hello,vue!" } }); </script> </body> </html>
3, Vue basic syntax
1. v-bind
Now that the data and DOM have been associated, everything is responsive. We operate the properties of objects on the console, and the interface can be updated in real time.
We can use v-bind to bind element attributes!
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <p>Madness theory Java</p> <!--view Layer template--> <div id="app"> <span v-bind:title="message">Hover for a few seconds to view the prompt information of dynamic binding here!</span> </div> </body> <!--Import js--> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> var vm = new Vue({ el: "#app", data: { message: "hello,vue" } }) </script> </html>
2 if else
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <!---view Layer template--> <div id="app"> <h1 v-if="type==='A'">A</h1> <h1 v-else-if="type==='B'">B</h1> <h1 v-else-if="type==='C'">C</h1> <h1 v-else>D</h1> </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> var vm=new Vue({ el:"#app", data:{ type:'A' } }) </script> </body> </html>
3 for
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <li v-for="item in items"> {{item.message}}</li> </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> var vm=new Vue({ el:"#app", data:{ items:[ {message:'Madness theory Java'}, {message: 'Crazy God said the first paragraph'} ] } }) </script> </body> </html>
4 v-on event binding
<!DOCTYPE html> <html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>demo04</title> </head> <body> <div id="app"> <button v-on:click="sayHi">click Me</button> </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> var vm=new Vue({ el:"#app", data:{ message:"Madness theory Java" }, methods:{//Method must be bound in the method object, sayHi:function (event){ alert(this.message) } } }) </script> </body> </html>
4, Vue bidirectional binding v-model
1. What is bidirectional binding
Vue.js is an MVVM framework, that is, two-way data binding, that is, when the data changes, the view changes, and when the view changes, the data will change synchronously. This is also Vue The essence of JS.
It is worth noting that the data bidirectional binding we call must be for UI controls, and non UI controls will not involve data bidirectional binding. One way data binding is a prerequisite for using state management tools. If we use vuex, the data flow is also single, which will conflict with two-way data binding.
2. Why do you want to realize two-way binding of data
In Vue In. JS, if vuex is used, the data is actually one-way. The reason why it is two-way data binding is the UI control used. For us to process forms, Vue JS bidirectional data binding is particularly comfortable to use. That is, the two are not mutually exclusive, and a single item is used in the global data flow to facilitate tracking; Local data flow is bidirectional and easy to operate.
3. Use two-way data binding in the form
You can use the v-model instruction to create two-way data bindings on form < input >, < textarea > and < Select > elements. It automatically selects the correct method to update the element according to the control type. Although some magic, v-model is essentially just a syntax sugar. It is responsible for listening to user input events to update data, and some special processing for some extreme scenarios.
Note: v-model will ignore the initial values of the value, checked and selected attributes of all elements and always take the data of Vue instances as the data source. You should declare it in the data option of the component through JavaScript.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> Gender: <input type="radio" name="sex" value="male"v-model="checkedSex">male <input type="radio" name="sex" value="female"v-model="checkedSex">female <p> Who did you choose :{{checkedSex}} </p> </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> var vm=new Vue({ el:"#app", data:{ checkedSex:"" } }) </script> </body> </html>
The two-way binding is equivalent to your input. The page will display your input information at the same time
5, Vue components
Components are reusable Vue instances. In other words, they are a set of reusable templates, which are similar to JSTL's custom tags and Thymeleaf's th:fragment. Usually, an application is organized in the form of a nested component tree:
The component has two parameters, the first name and the second name. props defines a formal parameter to accept variables in items; Template is used to get the variable value in the formal parameter.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <jianghe v-for="item in items" v-bind:q="item"></jianghe> </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> Vue.component("jianghe",{ props:['q'], template:'<li>{{q}}</li>' }); var vm=new Vue({ el:"#app", data:{ items:["java","Linux","front end"] } }); </script> </body> </html>
6, Axios communication
1. What is Axios
Axios is an open source asynchronous communication framework that can be used in browser and NodeJS. Its main function is to realize AJAX asynchronous communication. Its functional features are as follows:
Create XMLHttpRequests from the browser
From node JS create http request
Support promise API [chain programming in JS]
Intercept requests and responses
Convert request data and response data
Cancel request
Automatically convert JSON data
The client supports defense against XSRF (Cross Site Request Forgery)
GitHub: https://github.com/ axios/axios
Chinese documents: http://www.axios-js.com/
2. Why use Axios
Due to Vue JS is a view layer framework, and the author (you Yuxi) strictly abides by SoC (principle of separation of concerns), so Vue JS does not contain the communication function of Ajax. In order to solve the communication problem, the author separately developed a plug-in named Vue resource. However, after entering version 2.0, the maintenance of the plug-in was stopped and the Axios framework was recommended. Use jQuery less because it operates Dom too often!
Simulated Json data:
{ "name": "weg", "age": "18", "sex": "male", "url":"https://www.baidu.com", "address": { "street": "Wenyuan Road", "city": "Nanjing", "country": "China" }, "links": [ { "name": "bilibili", "url": "https://www.bilibili.com" }, { "name": "baidu", "url": "https://www.baidu.com" }, { "name": "cqh video", "url": "https://www.4399.com" } ] }
Load the template before rendering the data.
During initialization, instead of directly rendering data, vue objects are created first.
Then, the related events are executed through the callback function.
<!DOCTYPE html> <html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <div>{{info.name}}</div> <div>{{info.address.street}}</div> <a v-bind:href="info.url"></a> </div> <!--1.Import vue.js--> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <!--Import axios--> <script src="https://cdn.bootcdn.net/ajax/libs/axios/0.19.2/axios.min.js"></script> <script type="text/javascript"> var vm=new Vue({ el:"#app", data(){ return {//return executes the get method of axios //The return parameters of the request are appropriate and must be consistent with the json string info:{ name:null, address:{ street:null, city:null, country:null }, url:null } } }, mounted(){//Hook function axios.get("../data.json").then(response=>(this.info=response.data));//Get data, lambda expression, and the specific things to be executed after the arrow. } }); </script> </body> </html>
About v-bind instruction
v-bind is used to bind the href attribute of a tag. When a tag is clicked, it will jump to the corresponding url data in the corresponding vue https://www.baidu.com
3. Vue calculation properties
The key point of calculating attribute is attribute (attribute is a noun). Firstly, it is an attribute. Secondly, this attribute has the ability of calculation (calculation is a verb). Here, calculation is a function; Simply put, it is an attribute that can cache the calculation results (convert the behavior into a static attribute), that's all; Think of it as a cache!
Calculated attributes: the calculated attributes are cached
<!DOCTYPE html> <html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <p>Now1::{{currentTime1()}}</p> <p>Now2::{{currentTime2}}</p> </div> <!--1.Import vue.js--> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <!--Import axios--> <script src="https://cdn.bootcdn.net/ajax/libs/axios/0.19.2/axios.min.js"></script> <script type="text/javascript"> var vm=new Vue({ el:"#app", data:{ message:"Hello,kuangshen" }, methods:{ currentTime1:function (){//This is a method. Different from java, the method name in this vue is written in the front! return Date.now(); } }, computed:{//Calculate the attribute. The method name issued in methods cannot be duplicate. After the duplicate name, only the method in methods will be called currentTime2:function (){ return Date.now(); } } }) </script> </body> </html>
currentTime2 is a property, not a method. Therefore, do not add () to call it directly!
It can be seen that the calculation attribute will be recalculated only when it is changed. If it is not called again, it will not change, which can save the calculation attribute.
The currentTime1 method changes the value every time it is called. Just like the cache in mybatis.
Calculate the uncommon methods and cache them later to save time.
When a method is called, it needs to be calculated every time. Since there is a calculation process, there must be system overhead. What if the result does not change frequently? At this time, we can consider caching the results, which can be easily achieved by using the calculation attribute. The main feature of the calculation attribute is to cache the calculation results that do not change frequently, so as to save our system overhead;
7, Content distribution slot
In Vue In JS, we use the element as the exit to carry the distribution content. The author calls it a slot, which can be applied in the scenario of composite components;
In the developed page, set aside a slot in advance to facilitate future modification, dynamic modification and enhance reuse.
What is a slot?
The vue official document mentions that components can distribute content through slots in the "component foundation". How is the slot used? What scenario does it solve? In the process of building a page, we usually extract the more common parts as a separate component, but when we actually use this component, it can not fully meet the requirements. I hope to add something to this component. At this time, we need to use slots to distribute content.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <div id="app"> <todo> <todo-title1 slot="todo-title" title="Madness theory"></todo-title1> <todo-items1 slot="todo-items" v-for="item in todoItems":item="item"></todo-items1> </todo> </div> <!--1.Import vue.js--> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <!--Import axios--> <script src="https://cdn.bootcdn.net/ajax/libs/axios/0.19.2/axios.min.js"></script> <script type="text/javascript"> Vue.component("todo",{//Define a component with \ no line breaks //Slot slot template: '<div>\ <slot name="todo-title"></slot>\ <ul>\ <slot name="todo-items"></slot>\ </ul>\ <div>' }); Vue.component("todo-title1",{ props:['title'], template: '<div>{{title}}</div>' }); Vue.component("todo-items1",{ props:['items'], template:'<li>{{items}}</li>' }) var vm=new Vue({ el:"#app", data:{ todoItems:['Madness theory Java','Crazy God said','Madness theory Linux'] } }) </script> </body> </html>
slot name is the insertion point name!
< todo title1 slot = "todo title" title = "crazy God" > < / todo title1 >
Todo title1 corresponds to todo title1. The < slot name = "todo title" > < / slot > \ defines an insertion point called todo title, and the specific insertion component name is todo title1
Therefore, the meaning of the above line of code is: when the insertion point of todo title is encountered, the component todo titlle1 is executed. Title = "crazy God said" is the incoming parameter of the component execution
In vue, we should know that the front end, vue and component are parallel. In the < todo title1 > tag, pass relevant parameters to the component.
<todo-items1 slot="todo-items" v-for="(item,index) in todoItems" v-bind:item1="item" v-bind:index1="index" v-on:remove="removeItems(index)"></todo-items1>
In this code, index1 is the parameter in the component, and index is the parameter obtained through traversal.
8, Custom event content distribution
Through the above code, it is not difficult to find that the data item is in the Vue instance, but the deletion operation needs to be completed in the component. How can the component delete the data in the Vue instance? At this point, parameter transfer and event distribution are involved. Vue provides us with the function of customizing events, which helps us solve this problem;
Use this$ Emit ('custom event name ', parameter)
vue focus view layer
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> </head> <body> <div id="app"> <todo> <todo-title slot="todo-title" v-bind:name="title"></todo-title> <todo-items slot="todo-items" v-for="(item,index) in todoItems" v-bind:item="item" v-bind:index="index" v-on:remove="removeItems(index)"></todo-items> </todo> </div> <!--1.Import vue.js--> <script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script> <script> //The slot component must be defined in the front, or there will be no data Vue.component("todo", { template: '<div>\ <slot name="todo-title"></slot>\ <ul>\ <slot name="todo-items"></slot>\ </ul>\ <div>' }); Vue.component("todo-title", { //attribute props: ['name'], template: '<div>{{name}}</div>' }); Vue.component("todo-items", { props: ['item','index'], template: '<li>{{index}}---{{item}} <button @click="remove">delete</button></li>', methods: { remove: function (index) { // this.$emit custom event distribution this.$emit('remove',index) } } }); let vm = new Vue({ el: "#app", data: { //title title: "Library Series Books", //list todoItems: ['Romance of the Three Kingdoms', 'The Dream of Red Mansion', 'Journey to the West', 'Water Margin'] }, methods: { removeItems: function (index) { console.log("Deleted"+this.todoItems[index]+"OK"); this.todoItems.splice(index,1); } } }); </script> </body> </html>
IX vue introduction summary
Core, data driven, componentization
Advantages: it draws lessons from the modular development of AngulaJs and the virtual Dom of reat. The virtual Dom is to put the Dom operation into memory for execution:
Common attributes:
v-if
v-else-if
v-else
v-for
v-on binding time, short for@
Bidirectional binding of v-model data
v-bind binds parameters to components, abbreviated as:
Componentization:
Combined component slot
The internal binding event of the component needs to use this$ Emit ("event name", parameter);
Features of calculation attributes, cache calculation data
Following the principle of separation of Soc concerns, Vue is a pure view framework and does not include communication functions such as ajax. In order to solve the communication problem, we need to use the axios framework for asynchronous communication.
explain:
The development of Vue is given to NodeJS. The actual development adopts Vue cli scaffold development, Vue router routing, vuex state management and vue ui interface. We usually use elementUI (hungry Development) or ICE (Alibaba Development)
To quickly build front-end projects.
9, First Vue cli project
1. What is Vue cli
A scaffold officially provided by vue cli is used to quickly generate a vue project template;
The pre-defined directory structure and basic code are just like when we create Maven project, we can choose to create a skeleton project, which is the scaffold, and our development is faster;
Main functions:
Unified directory structure
Local debugging
Hot deployment
unit testing
Integrated packaging Online
2. Required environment
Node.js : http://nodejs.cn/download/
The next step is just to install it in your own environment directory
Git : https://git-scm.com/downloads
Mirror image: https://npm.taobao.org/mirrors/git-for-windows/
Confirm that nodejs is installed successfully:
cmd, enter node -v to check whether the publication number can be printed correctly!
Enter npm-v under cmd to check whether the publication number can be printed correctly!
This npm is a software package management tool, which is similar to apt software installation under linux!
npm is a package management tool in the JavaScript world and is a node JS platform's default package management tool. npm allows you to install, share, distribute code, and manage project dependencies.
Install node JS Taobao image accelerator (cnpm)
In this way, the download will be much faster~
# -g is the global installation npm install cnpm -g # If the installation fails, replace the source npm source with a Taobao image # Because npm installation plug-ins are downloaded from foreign servers, they are greatly affected by the network npm config set registry https://registry.npm.taobao.org # Then execute npm install cnpm -g
Installation location: C:\Users\Administrator\AppData\Roaming\npm
3. Install Vue cli
#Enter at the command desk cnpm install vue-cli -g #Check whether the installation is successful vue list
4. First Vue cli application
To create a Vue project, we randomly create an empty folder on the computer.
Here, I create a new directory D: \ project \ Vue study under disk D;
Create a vue application based on the webpack template
# myvue here is the project name, which can be named according to your own needs vue init webpack myvue
Select no all the way;
Initialize and run
cd myvue npm install npm run dev
After execution, the directory has many more dependencies
10, Webpack
WebPack is a module loader and packaging tool, which can process and use various resources, such as JS, JSX, ES6, SASS, LESS, pictures, etc. as modules.
npm install webpack -g npm install webpack-cli -g
Successful test installation: enter the following command, and the installation will be successful if the version number is output
webpack -v webpack-cli -v
1 what is webpack
In essence, webpack is a static module bundler for modern JavaScript applications. When webpack processes an application, it recursively builds a dependency graph containing each module required by the application, and then packages all these modules into one or more bundles
Webpack is the most popular front-end resource modular management and packaging tool at present. It can package many loosely coupled modules into front-end resources that meet the deployment of production environment according to dependencies and rules. You can also separate the code of the modules loaded on demand and load them asynchronously when they are actually needed. Through loader conversion, any form of resources can be used as modules, such as CommonsJS, AMD, ES6, CSS, JSON, CoffeeScript, LESS, etc;
with the tide of mobile Internet, more and more websites have evolved from web page mode to WebApp mode. They run in modern browsers and use new technologies such as HTML5, CSS3 and ES6 to develop rich functions. Web pages have not only completed the basic needs of browsers; WebApp is usually a SPA (single page application). Each view is loaded asynchronously, which causes more and more JS code to be loaded during page initialization and use, which brings great challenges to the front-end development process and resource organization.
the main difference between front-end development and other development work is that the front-end is based on multi language and multi-level coding and organization. Secondly, the delivery of front-end products is based on the browser. These resources run to the browser through incremental loading. How to organize these fragmented codes and resources in the development environment and ensure that they are fast and reliable on the browser Elegant loading and updating requires a modular system. This ideal modular system is a difficult problem that front-end engineers have been exploring for many years.
2. Use Webpack
-
First create a package and open it by idea to generate one Idea file means that the document is handed over to idea
-
Create the modules package in idea, and then create hello js,hello. JS exposed interface is equivalent to a class in Java
//Expose a method exports.sayHi = function () { document.write("<h1>Madness theory ES6</h1>>") }
3. Create main js is regarded as the main entry of js, main js request hello js calls the sayHi() method
var hello = require("./hello"); hello.sayHi()
4. Create webpack config. In the home directory js , webpack-config. JS is equivalent to the configuration file of webpack
enrty request main JS file
Output is the location and name of the output
module.exports = { entry: './modules/main.js', output: { filename: './js/bundle.js' } }
-
Enter the webpack command on the idea command console (idea needs to be started by the administrator)
-
After completing the above operations, a dist file will be generated in the home directory, and the generated JS folder path is / dist / JS / bundle js
-
Create index. In the home directory HTML import bundle js
<html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script src="dist/js/bundle.js"></script> </head> <body> </body> </html>
11, Vue router routing
Vue Router is Vue JS official routing manager (path jump). It and Vue JS core deep integration makes it easy to build single page applications. The functions include:
Nested routing / view tables
Modular, component-based routing configuration
Routing parameters, queries, wildcards
Based on Vue View transition effect of JS transition system
Fine grained navigation control
Links with automatically activated CSS class es
HTML5 history mode or hash mode, automatically degraded in IE9
Custom scroll bar behavior
1. Installation
Test learning based on the first Vue cli; Check the node first_ Is there a Vue router in modules
Vue router is a plug-in package, so we still need to install it with npm/cnpm. Open the command line tool, enter your project directory, and enter the following command.
npm install vue-router --save-dev
Go to node after installation_ Modules path to see if there is Vue router information. If yes, it indicates that the installation is successful.
2. Vue router demo instance
Open the case generated by Vue cli with idea
Clean up unused things. The logo image under assert. The helloworld component defined by component. We use our own components
Clean up the code. The following is the app under the cleaned code src Vue and main JS and the index of the root directory html
main. The role of JS code is to automatically scan the routing configuration inside
// The Vue build version to load with the `import` command // (runtime-only or standalone) has been set in webpack.base.conf with an alias. import Vue from 'vue' import App from './App' import router from './router' import VueRouter from 'vue-router' Vue.config.productionTip = false /* eslint-disable no-new */ // Display declaration usage Vue.use(VueRouter) new Vue({ el: '#app', router, components: { App }, template: '<App/>' })
index.js code to configure routing information
import Vue from 'vue' import Router from 'vue-router' import HelloWorld from '@/components/HelloWorld' import Content from '../components/Content' import Main from '../components/Main' Vue.use(Router); export default new Router({ routes: [ { path: '/content', name:'content', component:Content }, { path: '/main', name:'main', component: Main } ] })
App.vue write relevant code and set the home page
< router link to = "/ content" > content page < / router link >
Router link to set the url link to jump to
<template> <div id="app"> <h1>Vue-router</h1> <router-link to="/main">home page</router-link> <router-link to="/content">Content page</router-link> <router-view></router-view> </div> </template> <script> export default { name: 'App' } </script> <style> #app { font-family: 'Avenir', Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; margin-top: 60px; } </style>
Main.vue
<template> <h1>home page</h1> </template> <script> export default { name: 'Main' } </script> <style scoped> </style>
Conten.vue
<template> <h1>Content page</h1> </template> <script> export default { name: 'Content' } </script> <style scoped> </style>
Two different vue components
Like the previous requestMapping, routing loads the vue component according to the request.
main.js using routing, index JS configure routing
The use effect is as follows:
12, vue + ElementUI
Create a new Vue cli project as before
1 create project
vue init webpack hello-vue
2 installation dependency. We need to install four plug-ins: Vue router, element UI, sass loader, and node sass
# Enter project directory cd hello-vue # Installing Vue router npm install vue-router --save-dev # Install element UI npm i element-ui -S # Installation dependency npm install # Install SASS loader cnpm install sass-loader node-sass --save-dev # Start test npm run dev
Npm command interpretation
npm install mod
npm install moduleName: install the module into the project directory
npm install -g moduleName: - g means to install the module globally. The specific location of the module depends on the location of npm config prefix
npm install moduleName -save: - save means to install the module in the project directory and write the dependencies in the dependencies node of the package file, - S is the abbreviation of this command
NPM install modulename - save dev: – save dev means to install the module in the project directory and write dependencies in the devdependences node of the package file, - D is the abbreviation of this command
After successful creation, open it with idea, delete the net things, and create views and router folders to store views and routes