1, The same origin policy is a security policy for browsers
1. Same origin (i.e. same url): the protocol, domain name and port number must be exactly the same. (the request is from the same service)
2. Cross domain: it violates the homology policy, that is, cross domain.
3. ajax requests follow the same origin policy.
■ example of homology request (visit 127.0.0.1:9000 / server origin in the browser, and then click the button to send the homology request):
-
Processing of homologous requests by the server:
//3. Create routing rules (request is the requested message and response is the response message) app.get('/server-orign', (request, response) => { //Respond to a page response.sendFile(__dirname + '/Homology strategy.html'); }); app.get('/data', (request, response) => { //Response data response.send('Data returned by the server'); });
-
Client html:
const btn = document.getElementsByTagName('button')[0]; btn.onclick = function () { //console.log('test '); //Send ajax request const xhr = new XMLHttpRequest(); xhr.open('GET', '/data');//For homologous requests, the path can be abbreviated xhr.send(); xhr.onreadystatechange = function () { if(xhr.readyState === 4){ if(xhr.status >= 200 && xhr.status < 300){ console.log(xhr.response); } } } }
2, Solve cross domain (JSONP, CORS)
1. Unofficial solution JSONP:
(1) What is jsonp?
JSONP(JSON with Padding) is an unofficial cross domain solution that only supports get requests.
(2) How does jsonp work?
Some tags on Web pages are naturally cross domain, such as img link iframe script. JSONP uses script tags
The path src of script is used as the request path, and then only the data returned by the server needs to be encapsulated into js code
■ jsonp example 1:
- The server handles the response and returns the js code (the same as the external js code we usually introduce):
app.get('/jsonp', (request, response) => { //Response data response.send('console.log("hello jsonp")'); });
- Client processing:
<script src="http://127.0.0.1:9000/jsonp"></script>
■ jsonp example 2 (verify user name and display password):
-
Server processing:
//Server authentication user name app.all('/check', (request, response) => { //Response data // response.send('console.log("hello jsonp")'); const data = { name:'admin', password:'123456' }; let str = JSON.stringify(data); response.end(`handle(${str})`);//Call the handle method of the client (front end) });
-
Client processing:
user name: <input type="text" name="name"/> <p></p> <script> const input = document.getElementsByTagName('input')[0]; const p = document.querySelector('p'); //Declare a handle function to call the server to process the data returned by the server function handle(data) { input.style.border = "solid 1px #f00"; let username = input.value; //If the user name is correct, the data returned by the server will be displayed in the p tag if(username === data.name){ p.innerHTML = data.password; } } input.onblur = function () { //Send a request (jsonp) to the server to check whether the user exists const script = document.createElement('script'); //Set the src of the script tag to the url of ajax script.src = 'http://127.0.0.1:9000/check'; //Generate dom elements in the interface document.body.appendChild(script); } </script>
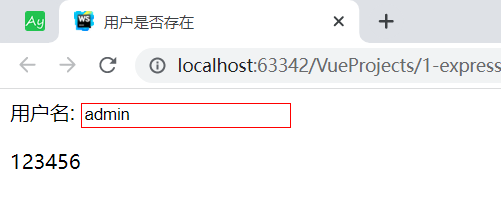
❀ jsop accesses the third-party interface across domains. The request is successful, but the data cannot be obtained?
From the above example, we can see that when the server is a third party (for example, visit Baidu's express interface: https://express.baidu.com/express/api/express),
Access the third-party interface through jsonp cross domain mode. The result: the request is successful, the status code is 200, but the response data cannot be obtained
- Reason 1: when the third-party service creates the routing rule, it is set that cross domain access is not allowed, so it prompts CORB and cannot get the data directly;
- Reason 2: I want to use src = 'request url' of the script tag to introduce the js code returned by the server response, but I don't know how the third-party server writes the response and what function is called back
- Summary: jsonp solves cross domain access. In addition to GET, it also needs the cooperation of the server to complete cross domain access
□ our own server is not set to allow cross domain access. By default, cross domain access is not allowed
□ however, I set some js codes to respond to the server setting request (the js code for the call of the handle function (the json object of data is used as a parameter))
□ on the client side, I introduce the js code returned by the server response through src = 'request url', and I know that the server response returns a call to a handle function, so I define a handle callback function on the client side (the parameter of the handle function is the data in json format).
2. Official solution CORS:
(1) CORS: cross origin resource sharing: cross domain resource sharing is allowed when the server sets the response header
(2) More about CORS: Cross source resource sharing (CORS) - HTTP | MDN (mozilla.org)
-
Cross domain request processing of server cors:
app.all('/cors', (request, response) => { //Set response header (allow cross domain) response.setHeader('Access-Control-Allow-Origin', '*'); // response.setHeader('Access-Control-Allow-Origin', 'http://127.0.0.1:9000); //Set response headers (allow custom request headers) response.setHeader('Access-Control-Allow-Headers', '*'); //Set response header (allow custom request mode) response.setHeader('Access-Control-Allow-Method', '*'); //Set response body response.send('hello cors'); });
-
Client processing:
<button>Click send ajax request</button> <script> const btn = document.getElementsByTagName('button')[0]; btn.onclick = function () { const xhr = new XMLHttpRequest(); xhr.open('get', 'http://127.0.0.1:9000/cors'); xhr.send(); xhr.onreadystatechange = function () { if(xhr.readyState === 4){ if(xhr.status >= 200 && xhr.status < 300){ console.log(xhr.response); } } } } </script>