catalogue
5, Random reading or input of files:
VII Determination of the end of file reading:
7.1 wrong use of feof function:
7.2 # how to judge whether the file reading is finished:
5, Random reading or input of files:
The so-called random reading or input of files means that they can be read or input without following a certain order, such as:
Suppose there is a string in a file: abcdef,,. At the beginning of reading, the file pointer points to the character A. when the character is read, the file pointer will offset one back
Element, pointing to the character b until all the data in the file is read. When the file is operated continuously, the file pointer at a positioning position will be constantly changed. Press
Read in a certain order, so how can we do not read in a certain order?
5.1 fseek function:
int fseek ( FILE * stream, long int offset, int origin );
Function: move the file pointer to a specific location
The second parameter refers to the offset:
If the offset is a positive number, it represents a backward offset. If the offset is a negative number, it represents a forward offset in bytes
The third parameter refers to the starting position when offsetting:
There are three options for the starting position when offsetting:
SEEK_CUR: indicates the position of the current file pointer, that is, offset from the position of the current file pointer
SEEK_END: indicates the position at the end of the file, that is, offset from the position at the end of the file. At this time, the content in the file has ended. If you offset later, no matter how many times it is offset
All bytes will end because the file ends, so that the fgetc function will return an EOF, that is - 1. When using% c to print int integer - 1, it will print blank
SEEK_SET: indicates the starting position of the file, that is, offset from the starting position of the file. This position is the starting position of the file content. If you offset further, regardless of the offset
A few bytes will also cause the fgetc function to return an EOF, that is - 1, because the file ends. It can also be offset from the starting position of the file content
The solution is the end of the content in the file. The result is the same as that caused by the backward offset from the position at the end of the file. It is printed when using% c to print int integer - 1
All blank
Example:
In C language, the opening method has no absolute influence, which is determined by the function used later, so:
For fgets and fgetc functions, the general usage is: "r"
For fputc and fputs functions, the general usage is: "w"
For the fread function, "r" or "rb" is generally used
For the fwrite function, we usually use "w" or "wb"
However, it may cause some impact in C + +, which will be discussed later.
5.2 # ftell function:
Returns the offset of the current file pointer relative to the starting position of the file content
long int ftell ( FILE * stream );
The type of the return value of the ftell function is long int, that is, the return value represents the offset of the current file pointer relative to the starting position of the file content, and the unit is bytes.
Example:
5.3 rewind function:
void rewind ( FILE * stream );
Strings and characters written in binary form are the same as those written in text form. In essence, they are stored ASCII code values of each character. Other types of data are different
Like
Vi Text and binary files:
character All with ASCII code Value form storage , Numerical data Both can be used ASCII storage , you can also use Stored in binary form.
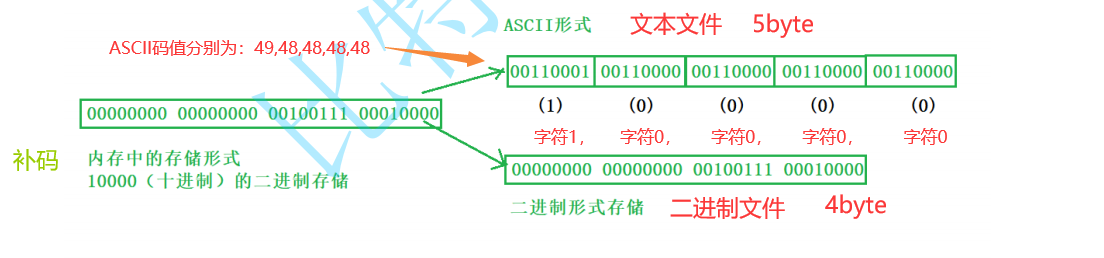
Test code:
VII Determination of the end of file reading:
7.1 wrong use of feof function:
7.2 # how to judge whether the file reading is finished:
7.2.1 text documents:
7.2.2 binary file:
7.3 # use of feof function:
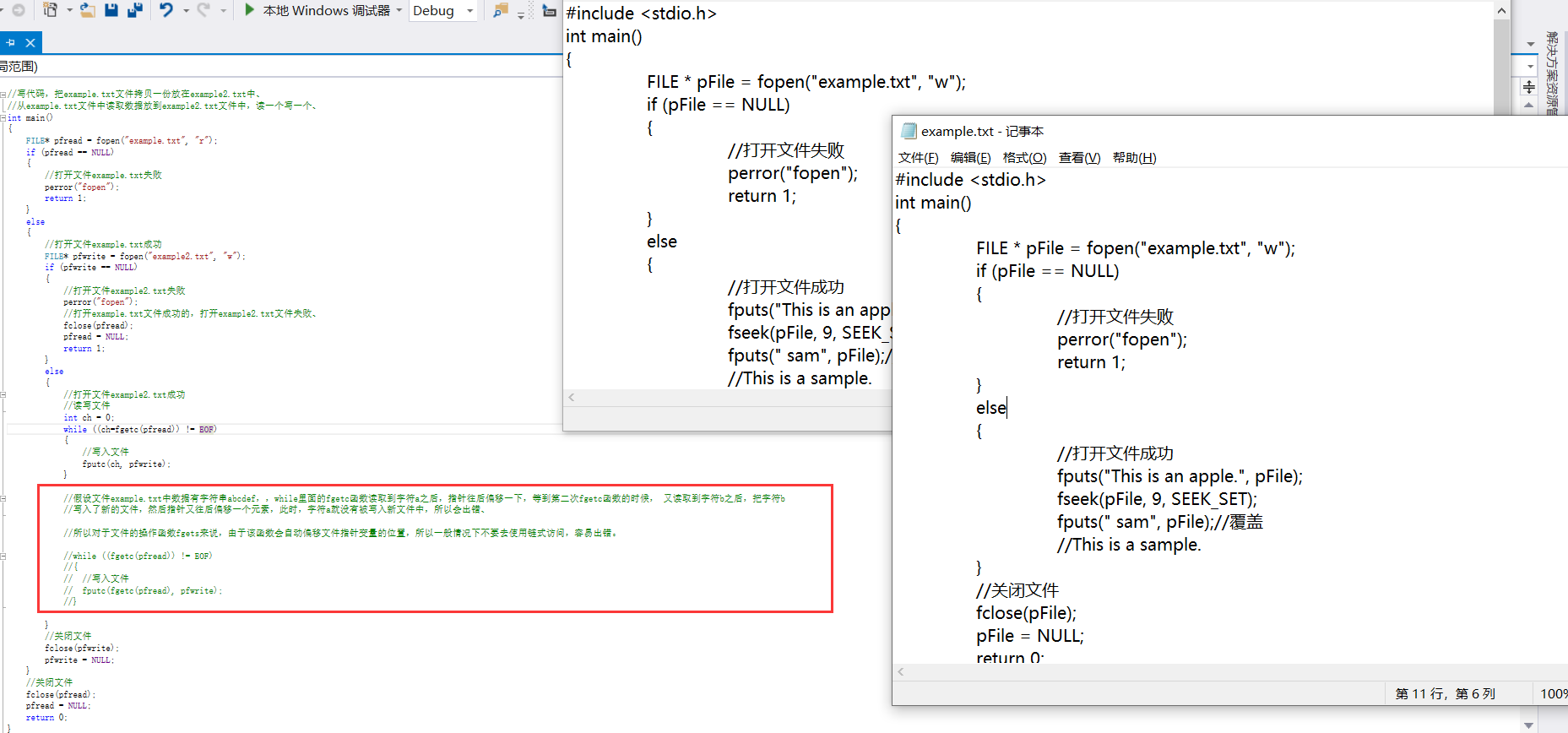
//Write code and put example Txt file and put it in example2 Txt //From example Txt file and put it into example2 Txt file, read one, write one int main() { FILE* pfread = fopen("example.txt", "r"); if (pfread == NULL) { //Open the file example Txt failed perror("fopen"); return 1; } else { //Open the file example Txt successful FILE* pfwrite = fopen("example2.txt", "w"); if (pfwrite == NULL) { //Open the file example2 Txt failed perror("fopen"); //Open example Txt file successfully, open example2 Txt file failed fclose(pfread); pfread = NULL; return 1; } else { //Open the file example2 Txt successful //Read write file int ch = 0; while ((ch=fgetc(pfread)) != EOF) { //write file fputc(ch, pfwrite); } //Suppose the file example The data in txt contains the string abcdef, while. After the fgetc function reads the character a, the pointer shifts back. When the second fgetc function reads the character b, the character b //A new file is written, and then the pointer is shifted back by one element. At this time, the character a is not written to the new file, so an error will occur //Therefore, for the file operation function fgets, because this function will automatically offset the position of the file pointer variable, generally do not use chain access, which is prone to error. //while ((fgetc(pfread)) != EOF) //{ // //Write file // fputc(fgetc(pfread), pfwrite); //} } //Close file fclose(pfwrite); pfwrite = NULL; } //Close file fclose(pfread); pfread = NULL; return 0; }
Example 2
1. ferror function:
int ferror ( FILE * stream );
If it is a read failure, that is, a read error, an int integer other than 0 is returned; otherwise, an int integer 0
2. feof function:
int feof ( FILE * stream );
If the end of the file is encountered, that is, the end of the file, an int integer other than 0 will be returned; otherwise, an int integer 0
1, Examples of text files:
#include <stdio.h> #include <stdlib.h> int main(void)//No reference { int c=0; // Note: int, not char, requires EOF FILE* fp = fopen("test.txt", "r"); if (!fp)//If the file fails to be opened, NULL pointer will be returned. NULL pointer is essentially 0. 0 means false,! 0, that is, if the logic is negative, it is true { //fail to open file perror("File opening failed"); return 1; } //The fgetc function will return EOF //For the file operation function fgetc, do not use chain access, which is prone to error while ((c = fgetc(fp)) != EOF) // Standard C I / O read file cycle { putchar(c); } //Determine the reason why the reading ended if (ferror(fp)) { puts("I/O error when reading"); } if (feof(fp)) { puts("End of file reached succ essfully"); } //Close file fclose(fp); fp = NULL; }
2, Examples of binary files:
#include <stdio.h> enum { SIZE = 5 }; int main(void) { double a[SIZE] = { 1., 2., 3., 4., 5. }; FILE *fp = fopen("test.bin", "wb"); // Binary mode must be used fwrite(a, sizeof *a, SIZE, fp); // Write array of double fclose(fp); double b[SIZE]; fp = fopen("test.bin", "rb"); size_t ret_code = fread(b, sizeof *b, SIZE, fp); // Read the array of double if (ret_code == SIZE) { puts("Array read successfully, contents: "); for (int n = 0; n < SIZE; ++n) printf("%f ", b[n]); putchar('\n'); } else { // error handling if (feof(fp)) printf("Error reading test.bin: unexpected end of file\n"); else if (ferror(fp)) { perror("Error reading test.bin"); } } //Close file fclose(fp); fp = NULL; }
VIII File buffer:
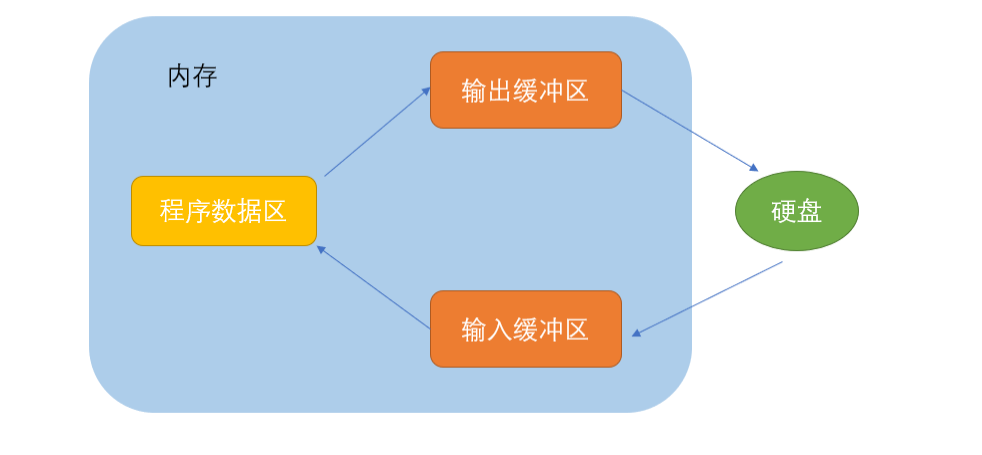
#include <stdio.h> #include <windows.h> //VS2013 WIN10 environment test int main() { FILE*pf = fopen("test.txt", "w"); fputs("abcdef", pf);//Put the code in the output buffer first and will not be written directly to the file printf("Sleep for 10 seconds-The data has been written. Open it test.txt File, no content found in the file\n"); Sleep(10000); printf("refresh buffer \n"); fflush(pf);//When the buffer is refreshed, the data of the output buffer is written to the file (disk) //Note: fflush cannot be used on higher version VS printf("Sleep for another 10 seconds-At this point, open again test.txt File, the file has content\n"); Sleep(10000); //Close file fclose(pf); //Note: fclose will also refresh the buffer when closing the file, even if it has not actively refreshed the buffer before. When closing the file, //The fclose function will automatically refresh the buffer once, remove the contents of the buffer, and then close the file. pf = NULL; return 0; //Therefore, file buffers exist }
Conclusion:
#define EXIT_FAILURE 1 #define EXIT_SUCCESS 0
You can simply think that both of them are constants of int integer