JavaScript operator
Operators commonly used in JS:
- Arithmetic operator
- Increment and decrement operators
- Comparison operator
- Logical operator
- Assignment Operators
Arithmetic operator
operator | describe | example |
---|---|---|
+ | plus | 10 + 20 = 30 |
- | reduce | 10 - 20 = -10 |
* | ride | 10 * 20 = 200 |
/ | except | 10 / 20 = 0.5 |
% | Remainder (modulus) | Returns the remainder of division 9% 2 = 1 |
The highest precision of floating-point number is 17 decimal places, but its precision is far lower than that of integer in arithmetic operation, so do not directly judge whether two floating-point numbers are equal
var result = 0.1 + 0.2;//The result is not 0.3, 0.300000000000000 4 console.log(0.07 * 100);//The result is not 7, but 7.000000000000001
Increment and decrement operators
Increment (+ +) and decrement (–)
In JS, increment (+ +) and decrement (–) can be placed before and after variables. When placed in front, we can call it pre increment (decrement) operator, and when placed after variables, we can call it post increment (decrement) operator
The increment decrement operator can only be used with variables
Pre increment operator
++Num is incremented in advance, similar to num = num + 1
First add 1 and then return the value
Post increment operator
Num + + pre increment, similar to num = num + 1
First return the original value, and then add 1
Examples
var a = 10; ++a; // ++a 11 a=11 var b = ++a + 2; // a=12 ++a = 12 console.log(b); // 14 var c = 10; c++; // c++ 11 c=11 var d = c++ + 2; // c + + returns 11 d=13 first, and then adds c = 12 console.log(d); // 13 var e = 10; var f = e++ + ++e; //E + + first returns 10 and then adds e=11 ++e first adds e=12 and then returns 12 console.log(f); // 22
Comparison operator
The comparison operator is the operator used when comparing two data. After the comparison operation, a Boolean value (true, false) will be returned as the result of the comparison operation.
Operator name Description case result
< less than sign 1 < 2 true
Greater than sign 1 > 2 false
=Greater than or equal to sign (greater than or equal to) 2 > = 2 true
< = less than or equal to sign (less than or equal to) 3 < = 2 false
==Equal sign (will transform) 37 == 37 true
!= Unequal sign 37= 37 false
=== !== The congruence value and data type are consistent 37 = = = '37' false
Operator name | explain | case | result |
---|---|---|---|
< | Less than sign | 1 < 2 | true |
> | Greater than sign | 1 > 2 | false |
>= | Greater than or equal to sign (greater than or equal to) | 2 >= 2 | true |
<= | Less than or equal to sign (less than or equal to) | 3 <= 2 | false |
== | Equal sign (transformation) | 37 == 37 | true |
!= | Unequal sign | 37 != 37 | false |
=== !== | Congruence requires consistent values and data types | 37 === '37' | false |
==Will transform, such as 18 = = '18', the result is true
Logical operator
Logical operators are operators used for Boolean operations, and the return value is also a Boolean value
Logical operator | explain | case |
---|---|---|
&& | "Logical and" is abbreviated as "and" | true && false |
|| | "Logical or", or "or" for short | true || false |
! | "Logical not", abbreviated as "not" | !true |
- Logic and: return true only when both sides are true, otherwise return false
- Logical or: return false only when both sides are false, otherwise both are true
- Logical non: also known as negation, it is used to take a value with the opposite Boolean value. For example, the opposite value of true is false
Short circuit operation (logic interrupt)
Principle: when there are multiple expressions (values) and the expression value on the left can determine the result, the value of the expression on the right will not be calculated
Logic and
- Syntax: expression 1 & & expression 2
- If the value of the first expression is true, expression 2 is returned
- If the value of the first expression is false, the expression 1 is returned
console.log(123 && 456);//456 console.log(0 && 456);//0 console.log(123 && 456 && 789);//789 console.log(0 && 1 + 2 && 456 * 789);//0
If the empty or negative is false, the rest is true, such as 0, '', null, undefined, NaN
Logical or
- Syntax: expression 1 | expression 2
- If expression 1 is true, expression 1 is returned
- If expression 1 is false, expression 2 is returned
console.log(123 || 456); //123 console.log(0 || 456); //456 console.log(123 || 456 || 789); //123 var num = 0; console.log(123 || num++);//123 console.log(num);//The final num result is 0, because the interrupt is not added with 1
Assignment Operators
Assignment Operators | explain | case |
---|---|---|
= | Direct assignment | var usrName = 'I am the value' |
+= ,-= | Add, subtract a number and then assign a value | var age = 10; age+=5;//15 |
*=,/=,%= | Multiply, divide, modulo and then assign values | var age = 2; age*=5; //10 |
Operator priority
priority | operator | order |
---|---|---|
1 | parentheses | () |
2 | Unary operator | ++ –- ! |
3 | Arithmetic operator | First * / later +- |
4 | Relational operator | > >= < <= |
5 | Equality operator | == != === !== |
6 | Logical operator | First & & then|| |
7 | Assignment Operators | = |
8 | Comma Operator | , |
Process control
There are three main types: sequential structure, branch structure and circular structure
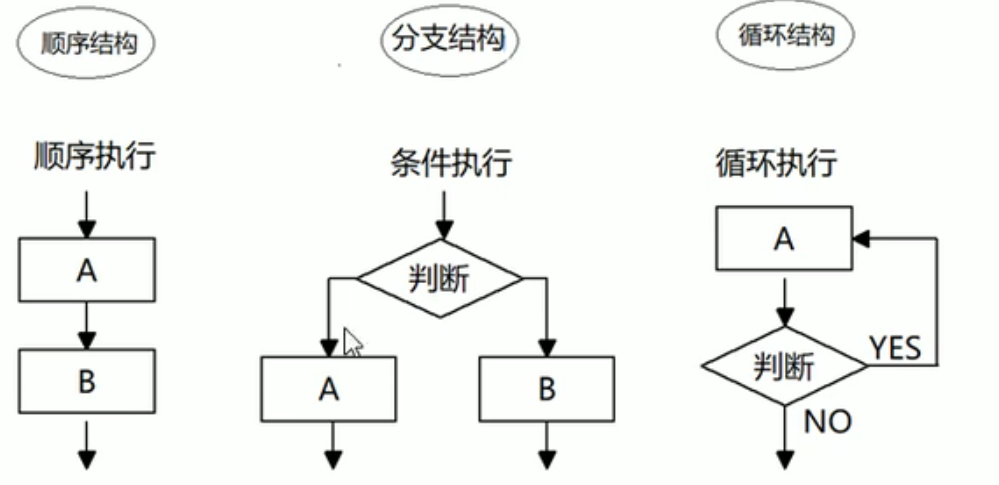
Branch process control
JS provides two branch structure statements:
- if statement
- switch
if statement
if (Conditional expression) { //Execute statement }
if else statement (double branch statement)
if (Conditional expression) { //Code executed when conditions are met } else { //Otherwise, execute the code }
if else if statement (multi branch statement)
if (Conditional expression 1) { //Statement 1 } else if (Conditional expression 2) { //Statement 2 } else if (Conditional expression 3) { //Statement 3 } else { //otherwise }
Ternary expression
Ternary expressions can also make some simple conditional choices. Expressions with ternary operators are called ternary expressions
-
Conditional expression? Expression 1: expression 2
-
If the conditional expression holds, the expression 1 is returned; otherwise, the expression 2 is returned
Example:
//Digital complement 0 //0 before less than 10 var num = prompt('Please enter 0~59 A number between'); var result = num < 10 ? '0' + num : num; alert(result);
switch Statements
switch(expression){ case value1: //Execute statement 1; break; case value2: //Execute statement 2; break; //... default: //Execute the last statement; (not executed on matching) }
-
The value of the expression and the value of the case must be congruent, and the value must be consistent with the data class
-
If there is no break in the current case, continue to execute the next case
Example:
// The user enters a fruit in the pop-up box. If there is, the price of the fruit will pop up. If there is no fruit, the "no fruit" will pop up var fruit = prompt('Please enter the apple you want to query'); switch (fruit) { case 'Apple': alert('The price of apple is 3.5 element/Kilogram'); break; case 'Banana': alert('The price of bananas is 3 yuan/Kilogram'); break; default: alert('There is no such fruit'); }
The difference between switch statement and if else statement
- Generally, it can be interchanged
- The switch statement is usually used to handle the case to compare the determined value, while the if else statement is more flexible and is often used to judge the range (greater than or equal to a certain range)
- The conditional statement of the program directly executed after the conditional judgment of the swit ch statement is not more efficient, while the if else statement has several conditions, you have to judge how many times
- When there are fewer branches, the execution efficiency of if else statement is higher than that of switch statement
- When there are many branches, the execution efficiency of switch statement is relatively high, and the structure is clear