Go language learning checking and patching Day3
Zero. Preface
Because the author has a weak foundation and often encounters many confused problems when using Go language, the author is determined to check and fill in the gaps of Go language. This [Go language checking and filling in] series is mainly to help novice Gopher better understand the mistakes, key and difficult points of Go language. I hope you can like it, praise it and pay attention to it!
1, Comparison of structures
Let's first look at a section of code about structure comparison:
package main import "fmt" func main() { struct1 := struct { age int name string sex bool }{age: 18, name: "Boda", sex:false} struct2 := struct { age int name string sex bool }{age: 21, name: "Regan Yue", sex:true} if struct1 == struct2 { fmt.Println("struct1 == struct2") } struct3 := struct { age int people map[string]bool }{age: 31, people: map[string]bool{"Boda": false}} struct4 := struct { age int people map[string]bool }{age: 21, people: map[string]bool{"ReganYue": false}} if struct3 == struct4{ fmt.Println("struct3 == struct4") } }
Do you think there will be errors when compiling?
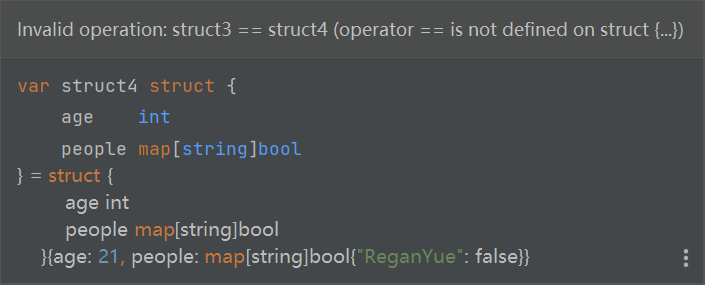

Ah, there's a big problem. It says that structures containing map s cannot be compared.
Yes, only structures containing bool type, numeric type, string, pointer, array and other types can be compared, while structures containing slice, map and function cannot be compared.
There are also several points worth noting:
- Structures can only compare whether they are equal, not their size.
- Only structures with equal attributes and consistent attribute order can be compared.
2, Several ways of accessing member variables through pointer variables
Look at this code first:
package main import "fmt" func main() { s1 := struct { name string age int64 }{name:"Regan",age:21} s2 := &s1 fmt.Println(s2.name) fmt.Println((*s2).name) fmt.Println((&s2).name) }
Where do you think the error will be reported?

oh Yes (& S2) Name has a problem
Because & is the address character and * is the pointer dereference character. Therefore, the member variable can be accessed only by dereferencing the pointer.
Do you wonder why pointers and references can get member variables? In fact, it is dereference, which is the strength of our GO language. It can dereference automatically, but unfortunately, it can only dereference level B pointers. If it is a multi-level pointer, you have to rely on yourself.
3, The difference between type alias and type definition
package main import "fmt" type Int1 int type Int2 = int func main() { var i = 0 var i1 Int1 = i var i2 Int2= i fmt.Println(i, i1, i2) }
Can you see the problem at a glance?
But when they are put together, can you immediately distinguish which is the type alias and which is the type definition?
type Int1 int
It defines that a new type is created based on the int type, and
type Int2 = int
Is to use Int2 as an alias for int type.
Therefore, the following errors will be reported:

This is because Go is a strongly typed language, so we need to cast int into Int1 type to compile.
4, A note for slicing
package main import "fmt" func main() { a := []int{7, 8, 9} fmt.Printf("%+v\n", a) fun1(a) fmt.Printf("%+v\n", a) fun2(a) fmt.Printf("%+v\n", a) } func fun1(a []int) { a = append(a, 5) } func fun2(a []int) { a[0] = 8 }
Take a look at the running results:
[7 8 9] [7 8 9] [8 8 9]
what?!! No append in? This is because append may lead to memory reallocation, so we can no longer determine whether the slice before append and the slice after append refer to the same memory space.
Therefore, the slice a after the execution of fun1 to append is not the same as the outer slice a, so looking at the outer slice a, you can find that the content of the outer slice has not changed.
5, Several ways of Golang string connection
package main import ( "bytes" "fmt" "strings" ) func main() { str1 := "abc" str2 := "def" strs := []string{"Regan","Yue"} fmt.Println(str1+str2) sprintf := fmt.Sprintf("abc%s", "accc") fmt.Println(sprintf) join := strings.Join(strs, "-") fmt.Println(join) var buffer bytes.Buffer buffer.WriteString("hello") buffer.WriteString("world") fmt.Println(buffer.String()) }
Four ways of connecting strings are mentioned here. The first one uses the + sign to connect directly, and the second one uses FMT Sprintf (), the third is to use strings Join(), the fourth is to use buffer Writestring() to connect strings.