Introduction to cloud function
Cloud base, a one-stop efficient development platform: https://curl.qcloud.com/JcBqSTMl
The Serverless Cloud Function (SCF) is the Serverless execution environment provided by Tencent cloud.
Simply write a simple, single purpose cloud function to associate it with events generated by Tencent cloud infrastructure and other cloud services.
Using cloud functions, you can run back-end code in the form of functions to respond to SDK calls or HTTP requests. The code will be stored in the cloud and run in a managed environment without managing or operating your own server.
Initialize cloud function project
Tencent cloud development provides detailed development documents and cli tools (CloudBase CLI) to help users quickly and conveniently deploy projects and manage cloud development resources.
If you haven't initialized the cloud function project, you can use the CLI tool for initialization (recommended), or you can directly build the project based on the source code. You can refer to this section:
CLI tools: https://docs.cloudbase.net/cli-v1/intro
Cloud function: https://docs.cloudbase.net/cloud-function/introduce
Cloud function + TypeScript
The cloud function. Node has been provided JS function template, but there is no official support for TypeScript.
Students who are used to developing in the type detection and code prompt environment of TS need to manually create a cloud function development environment of TypeScript.
The whole process is roughly as follows: write cloud function code using TypeScript = > compile TS file as JS = > Modify cloud function deployment configuration = > upload and deploy cloud function
Next, we will use the basic template node app in the cloud function console (create a blank function using the helloworld example) to introduce the process of cloud function + TypeScript.
1, Transform cloud function directory structure
The directory structure of cloud development after node app is initialized with TCB CLI tool should be as follows:
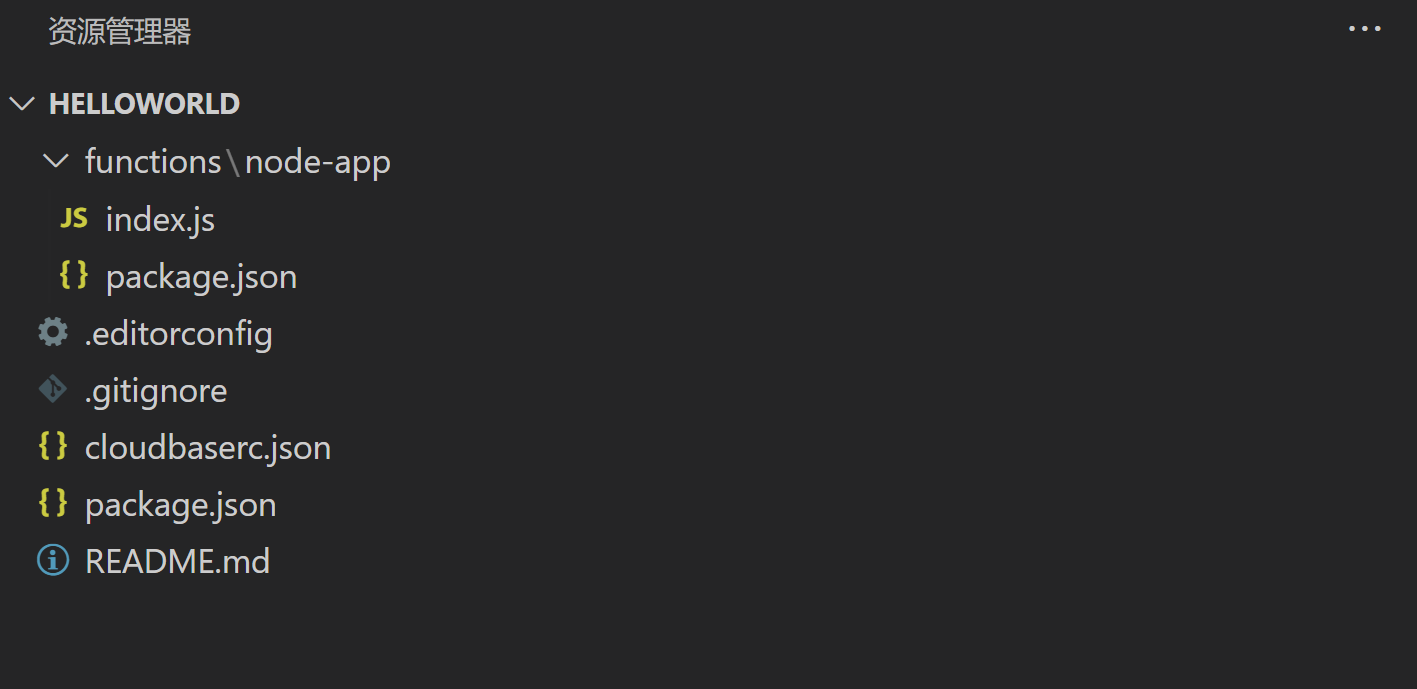
The configuration file of cloud development environment is cloudbaserc JSON, where the subdirectory functions \ node app is the root directory of the new "node app" cloud function
We should write TypeScript files in src directory and generate the compiled and converted js files in dist directory. Therefore, we need to modify the directory structure and create dist and src folders respectively
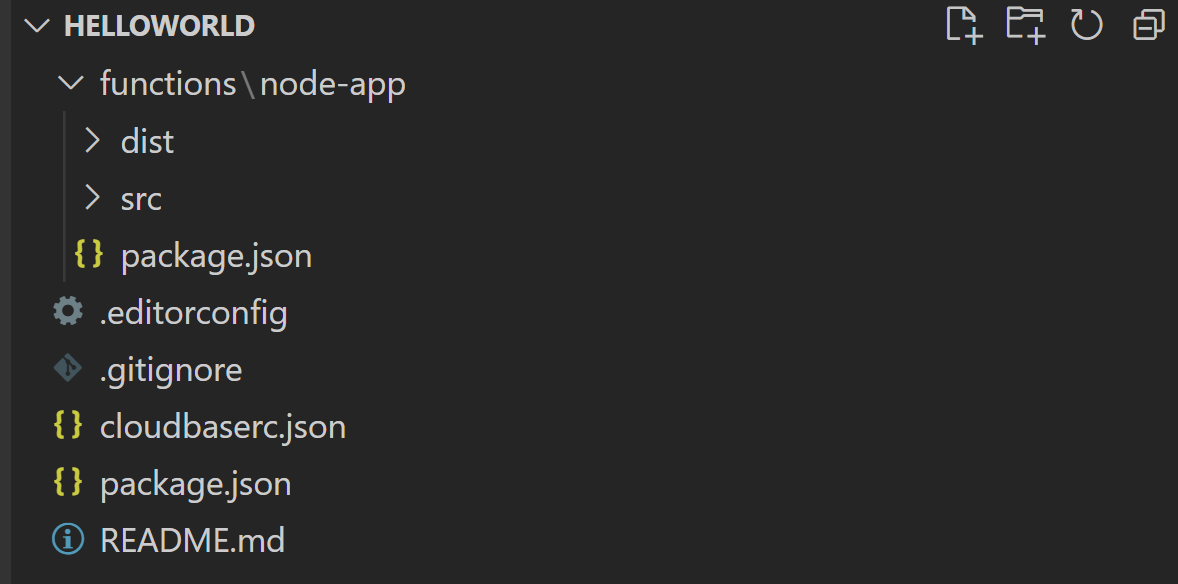
2, Configure TypeScript environment
Configure TypeScript tool and install TypeScript
npm install -g typescript
perhaps
yarn global add typescript
Use the tsc -v command to ensure successful installation and output the version number
Write tsconfig. In the cloud function directory JSON configuration file
tsconfig.json
{ "compilerOptions": { "module": "commonjs", "target": "es6", "lib": [ "ES6", "dom" ], "sourceMap": true, "outDir": "./dist", "rootDir": "./src", "importHelpers": true, "strict": true, "allowSyntheticDefaultImports": true }, "exclude": [ "node_modules" ], "include": [ "src/index.ts", "src/**/*" ] }
Install some necessary dependencies
yarn add @types/node tslib
3, Writing TypeScript source code
Write index. In the src directory ts
const hello : string = "Hello World" exports.main = async (event : any, context : any) => { console.log(hello) return event }
Use the tsc command to compile the ts file to the dist directory
At this point, your directory should have the following structure:
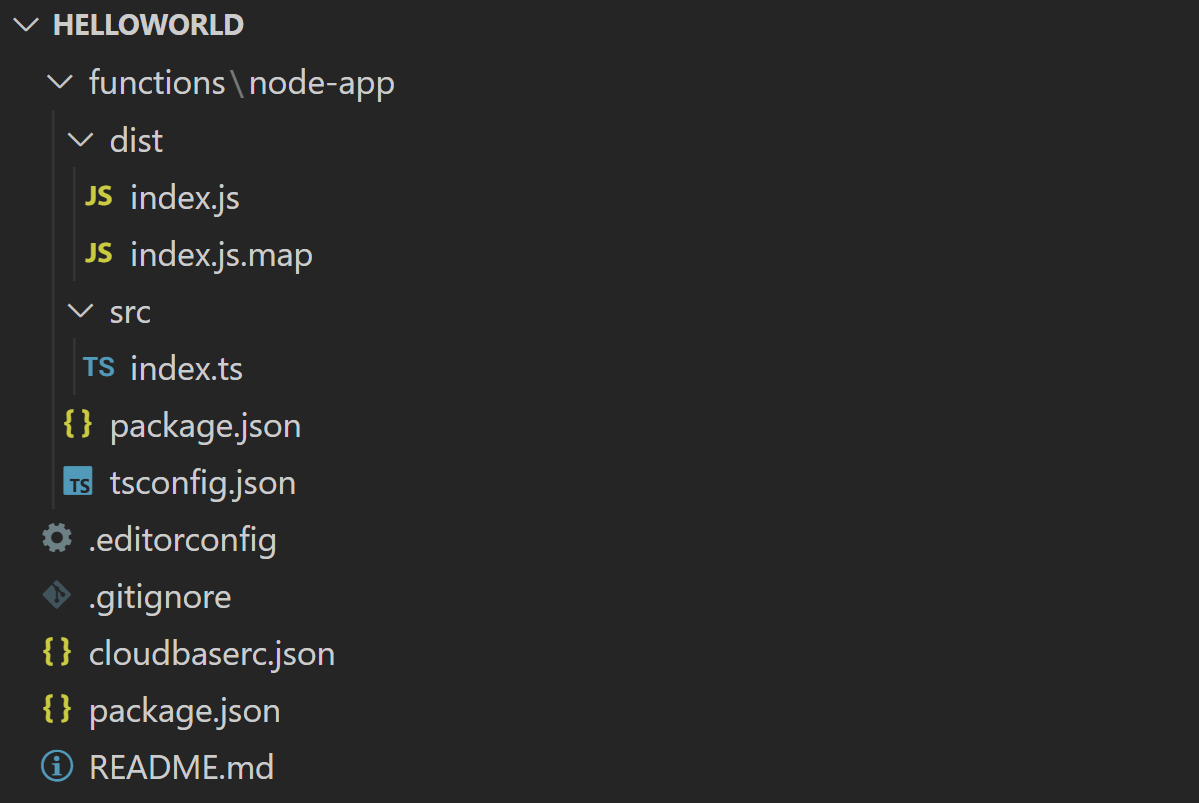
4, Modify cloud function deployment configuration
At this time, directly uploading and deploying cloud functions cannot work normally, because the cloud function will not find the entry file, so it is necessary to modify the configuration of cloud functions
In cloudbaserc JSON, find the function field and configure it:
"installDependency": true // Indicates that the package is automatically installed after the cloud function is uploaded JSON dependency "ignore": [ // Ignore local dependency files that do not need to be uploaded and deployed, TypeScript source files in src directory, etc "*.md", "node_modules", "node_modules/**/*", "src", "src/**/*" ]
An example of a complete configuration file is as follows:
{ "version": "2.0", "envId": "helloworld-*****", "$schema": "https://framework-1258016615.tcloudbaseapp.com/schema/latest.json", "functionRoot": "./functions", "functions": [ { "name": "node-app", "timeout": 5, "envVariables": {}, "runtime": "Nodejs10.15", "memorySize": 128, "handler": "index.main", "installDependency": true, "ignore": [ "*.md", "node_modules", "node_modules/**/*", "src", "src/**/*" ] } ], "framework": { "name": "node-starter", "plugins": { "function": { "use": "@cloudbase/framework-plugin-function", "inputs": {} } } }, "region": "ap-shanghai" }
Then write the cloud function entry file index. In the cloud function root directory js
"use strict"; const index = require('./dist/index') exports.main = async function (event, context) { return await index.main(event, context) }
5, Upload and deploy cloud functions
Use tcb fn deploy to directly deploy cloud functions, and debug on the console
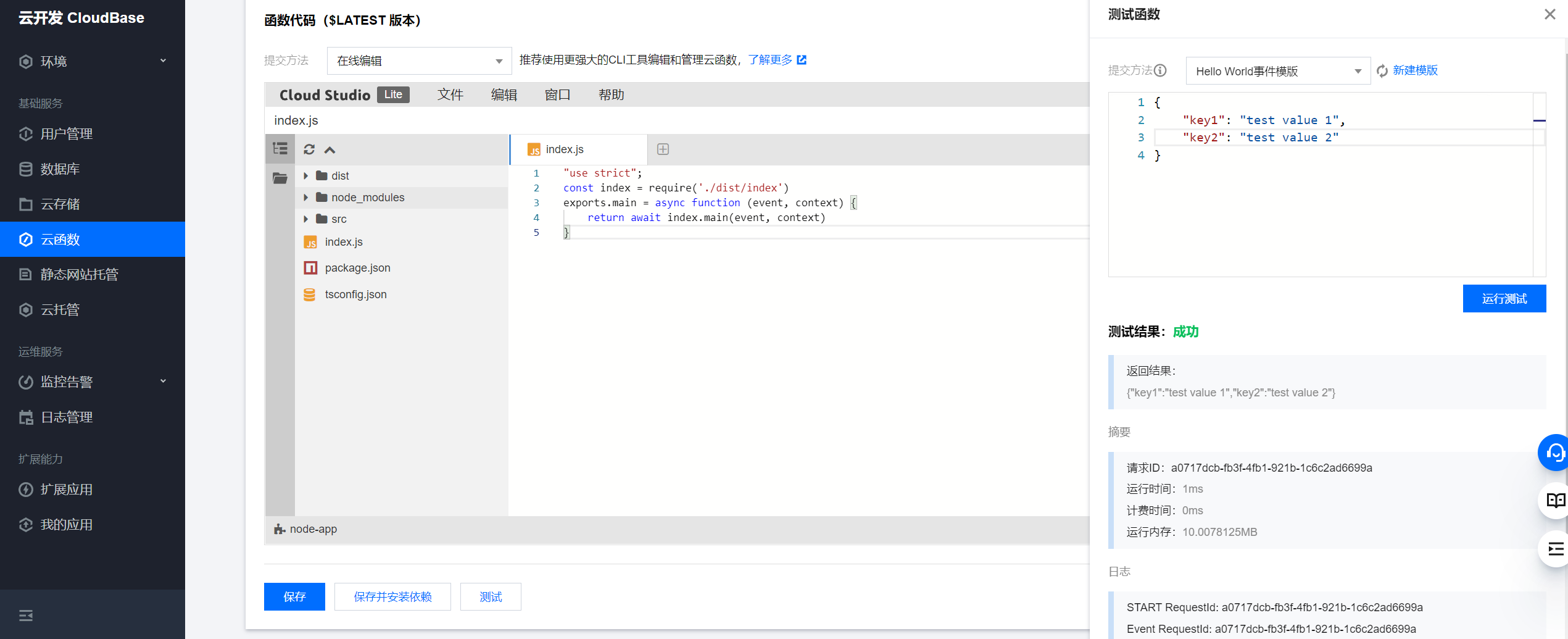
The test results are successful, indicating that cloud function + TypeScript has been successfully implemented
Cloud base, a one-stop efficient development platform: https://curl.qcloud.com/JcBqSTMl
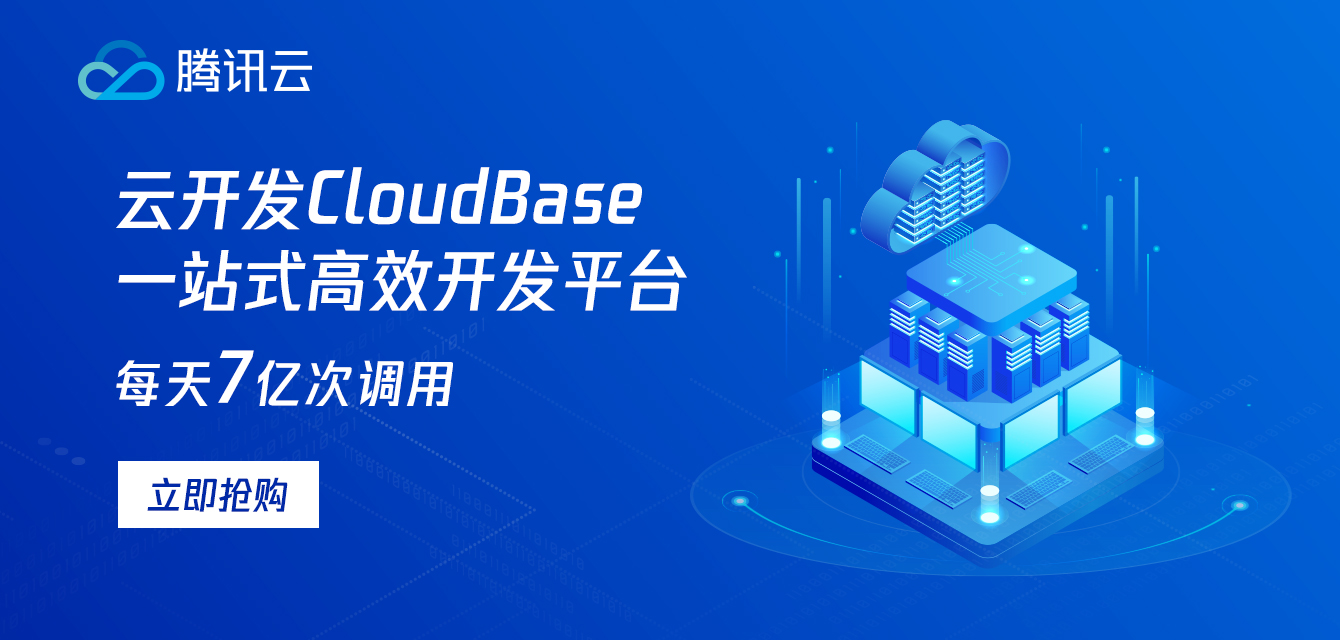
This article is an original article of Titan notes, which is synchronously published to Tencent cloud + community