When we collect specific data, it often takes a long time. Sometimes, because of some things, it is impossible to wait for the results in front of the computer for a long time, so the program needs to automatically send us e-mail and other notices after a period of time, and execute exit program or shutdown and other aftercare work to save resources or power, So how does this process need to be implemented. Based on this collection program, this essay adds the realization of these functions and introduces some processing skills.
Click to get the official version of devaexpress
1, Mail configuration
If we need to realize the notification methods such as sending e-mail or sending SMS, we need to enter the parameters involved in these processing processes into the system in advance. It's ok if we can't hard code them. However, for scalability, I prefer to use the configuration interface to configure the parameters.
In the processing of parameter configuration, in the blog< Implementation of parameter configuration management function of Winform development framework - based on settingsprovider Net >And< Realizing dynamic personalized configuration management of menu list in Winform interface >All of them have been introduced in detail, based on settingsprovider Net can realize our convenient configuration function, which can be configured in XML file or saved in database for processing as needed.
The following interface is also needed to configure my email collection interface.
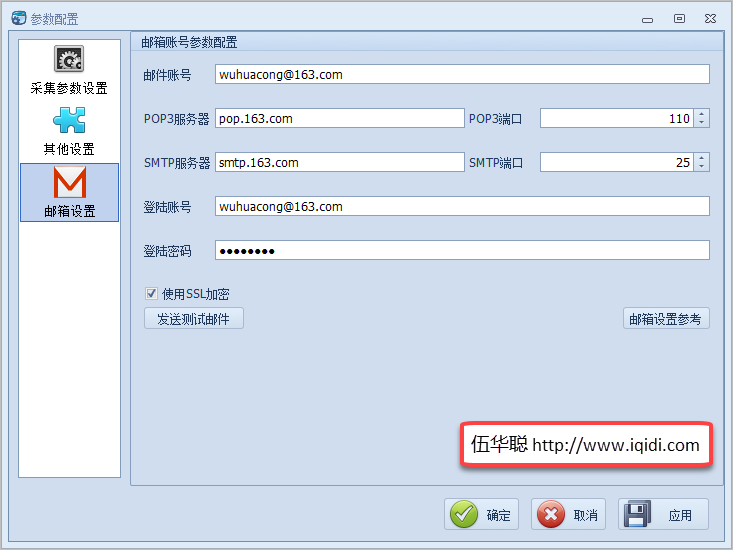
Two additional function buttons are placed in this. One is the mailbox setting reference, and the other is to send the test email. The former is used to assist in filling in some parameters, and the latter is to verify whether the user account receives the test email.
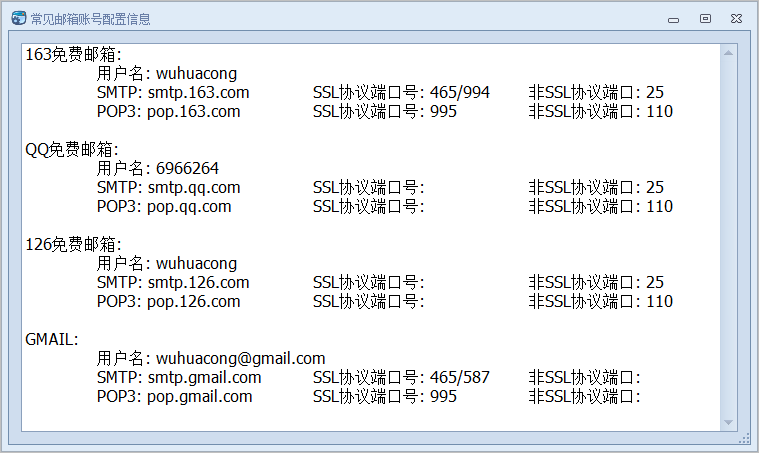
After sending the test email successfully, we will verify whether you have received it, so as to check whether the parameters provided under are correct.
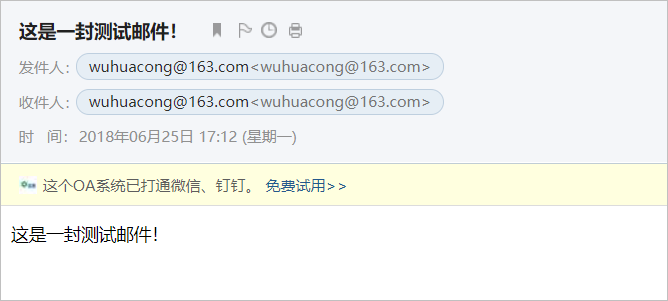
2, Set timing processing
After completing the above steps, we have basically completed half of the workload, and the rest is to ask the system to send a notice to us and deal with the aftermath at the right time.
If we are timed, we need to specify a time range. It is only appropriate to use the TimeSpanEdit control of devaexpress. After we only need to determine the data of hour, minute and second, we can determine the final time of our task execution according to this time range.
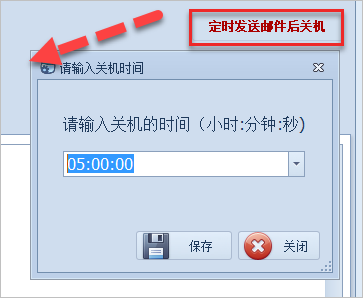
This small pop-up form is only used to obtain the time range entered by the user, and there is no specific logic.
After inputting the shutdown time, we can pop up a countdown window according to the shutdown time, covering the interface of the main program.
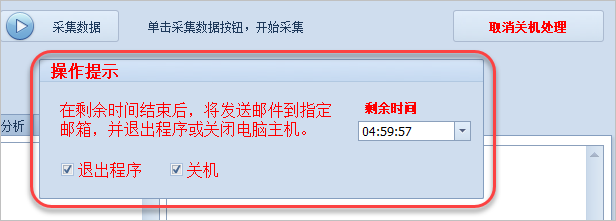
Finally, after we reach the trigger point of time, we can send email notification and exit the program or shut down.
The above is the whole process of processing. How about the processing code? Let's analyze the specific code process.
private bool isShutdown = false; private TimerHelper timerHelper; private void btnSendAndShutdown_Click(object sender, EventArgs e) { btnSendAndShutdown.Enabled = false; try { if (!isShutdown) { //Get the time of shutdown FrmShutdownTime frmTime = new FrmShutdownTime(); if (frmTime.ShowDialog() != System.Windows.Forms.DialogResult.OK) return; //Convert to final time TimeSpan timeSpan = frmTime.timeShutdown.TimeSpan; this.endTime = DateTime.Now.Add(timeSpan); //Timer auxiliary class handles timed work timerHelper = new TimerHelper(1000, true); timerHelper.Execute += () => { //Process and judge the event every second if (ShutdownEvent != null) { ShutdownEvent(sender, e); } }; //Display shutdown panel groupShutdown.BringToFront(); groupShutdown.Visible = true; } else { //Close panel CloseShutDownGroup(); } isShutdown = !isShutdown; this.btnSendAndShutdown.Text = isShutdown ? "Cancel shutdown processing" : "Shut down after sending mail regularly"; } finally { btnSendAndShutdown.Enabled = true; } }
The above main processing logic is placed on the processing events of the timer
ShutdownEvent(sender, e);
This triggered event is an event defined in the main window. It is used to count down and send notifications.
private event EventHandler ShutdownEvent;
Then we can initialize the event processing in the form. The initialization code is as follows.
//Event that closes or exits the program this.ShutdownEvent += (s, e)=> { #region timing processing operation this.Invoke(new MethodInvoker(delegate() { //Judge whether the current remaining time enters the notification process var ts = endTime.Subtract(DateTime.Now); if (ts.TotalSeconds > 1) { //Update countdown timeLeft.TimeSpan = new TimeSpan(ts.Days, ts.Hours, ts.Minutes, ts.Seconds); } else { //Close the panel and exit the timer CloseShutDownGroup(); //Send mail SendMail(); //Or exit the host program if (chkShutdown.Checked) { Process.Start("shutdown.exe", "-s");//Shut down } else if (chkExitApp.Checked) { Application.ExitThread(); } } })); #endregion
The final logic is to send mail and exit the program or shut down:
//Send mail SendMail(); //Close the host or exit the program if (chkShutdown.Checked) { Process.Start("shutdown.exe", "-s");//Shut down } else if (chkExitApp.Checked) { Application.ExitThread(); }
The shutdown operation is used to execute the command line to realize the shutdown processing, which is very convenient.
For the processing of this Shutdown command, I will list some function descriptions below.
Parameters of shutdown command:
shutdown.exe -s: shutdown
shutdown.exe -r: shutdown and restart
shutdown.exe -l: log off the current user
shutdown.exe -s -t time: set shutdown countdown
shutdown.exe -h: Hibernate
shutdown.exe -t time: set the countdown to shutdown. The default value is 30 seconds.
shutdown.exe -a: cancel shutdown
shutdown.exe -f: forcibly shut down the application without warning
shutdown.exe -m \ computer name: control remote computer
shutdown.exe -i: displays the "remote Shutdown" graphical user interface, but it must be the first parameter of Shutdown
shutdown.exe -c "message content": enter the message content in the shutdown dialog box
shutdown.exe -d [u][p]:xx:yy: list the reason code for system shutdown: u is the user code, p is a planned shutdown code, xx is a primary reason code (positive integer less than 256), yy is a secondary reason code (positive integer less than 65536)
For example, if your computer needs to shut down at 12:00, you can select "start → run" and enter "at 12:00 Shutdown -s". In this way, the computer will appear the "system shutdown" dialog box at 12:00, with a 30 second countdown by default and prompt you to save your work.
If you want to shut down in the way of countdown, you can enter "Shutdown.exe -s -t 3600", which means automatic shutdown after 60 minutes, and "3600" means 60 minutes.
When sending e-mail, we usually use an encapsulated class for e-mail sending.
/// <summary> ///Send mail /// </summary> private bool SendMail() { //statistical data btnSumaryData_Click(null, null); string title = string.Format("{0}Period statistical results-{1}", Portal.gc.CurrentQSNumber, DateTime.Now); //Get the content displayed by the control and convert its newline List<string> list = new List<string>(); list.AddRange(txtShenxiao.Lines); list.AddRange(this.txtShenxiao2.Lines); string content = string.Join("<br>", list); //Send mail var success = SendMailHelper.SendMail(title, content); return success; }
When we send mail, we first get the user's mail configuration information, then send mail to send assistant class to realize the sending and processing of the content. The specific code is shown below.
public class SendMailHelper { /// <summary> ///Send mail results /// </summary> public static bool SendMail(string title, string content) { //Get configured mail information string creator = "";// LoginUserInfo.Name; ISettingsStorage store = new DatabaseStorage(creator); SettingsProvider settings = new SettingsProvider(store); bool result = false; EmailParameter parameter = settings.GetSettings<EmailParameter>(); if (parameter != null) { //Use the mail auxiliary class to send the mail content EmailHelper email = new EmailHelper(parameter.SmtpServer, parameter.LoginId, parameter.Password); email.Subject = title; email.Body = content; email.IsHtml = true; email.Charset = "gb2312"; email.From = parameter.Email; email.FromName = parameter.Email; email.AddRecipient(parameter.Email); result = email.SendEmail(); } return result; }
The above is the whole process, which also involves the way of accessing controls between processes, as shown in the following code.
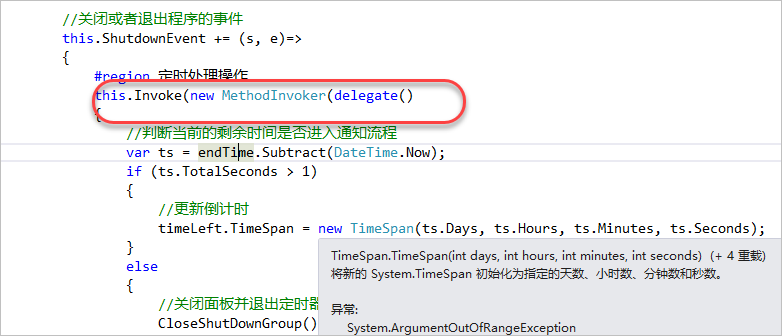
For this detailed introduction, please refer to my earlier essays< On data binding and assignment in multithreading >, these details need us to test step by step and find the best solution. I hope the idea of this essay will give you some inspiration.
Of course, if the system is larger and more systematic, we can also consider using EasyNetQ to realize information notification.
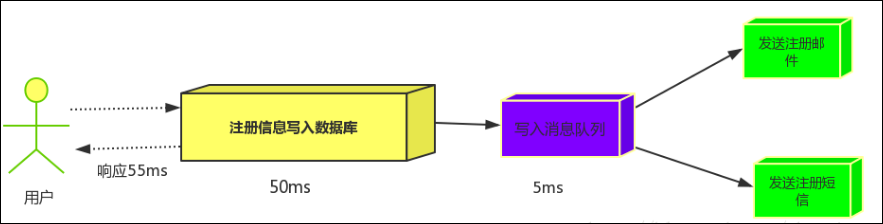
This kind of notice can be better extended. For details, please refer to the following essay< Using EasyNetQ component to operate RabbitMQ Message Queuing service >, but generally small programs don't have to be so troublesome. Just use a timer to process them.
DevExpress Universal The second major version of this year, v21.0, was officially released in October 2. This version officially supports visual studio 2022 & Net6 is also perfectly compatible with Microsoft's latest release of Windows 11, which comprehensively solves the problems of various user scenarios. Keep pace with the times and never stop!
This article is reproduced from: Blog Park - Wu Huacong
Devaxpress technical exchange group 5:742234706 welcome to join the group discussion