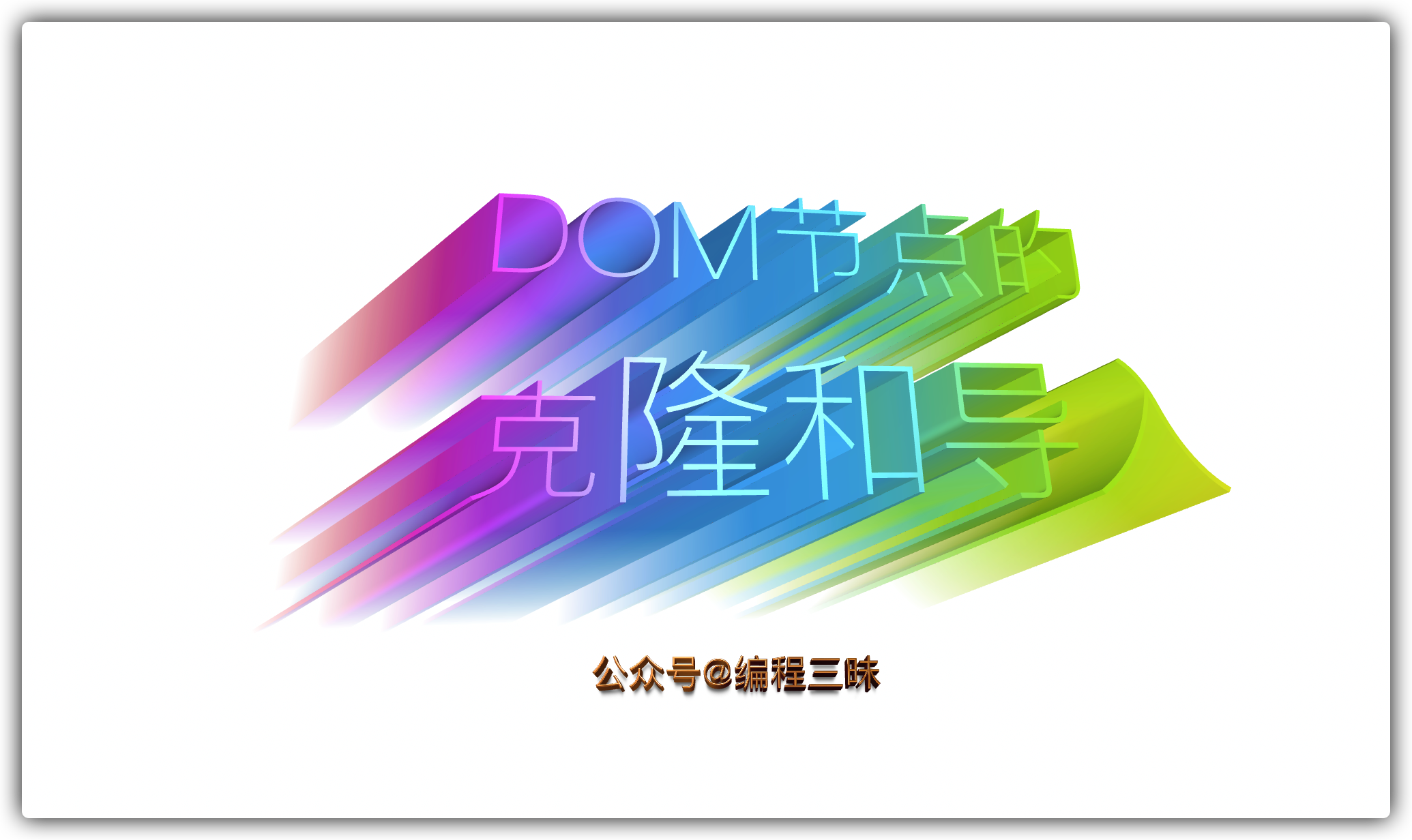
preface
When using JS to operate DOM nodes, we often use the operation of cloning (or importing) nodes. What methods can achieve the effect of node cloning (or importing)?
Today, let's summarize the methods that can achieve the effect of node cloning (or import).
node.cloneNode()
When it comes to cloning nodes, the first thing we can think of must be node Clonenode() method.
grammar
The syntax is as follows:
let cloneNode = targetNode.cloneNode(deep);
- cloneNode finally clones the generated node copy.
- targetNode the target node to be cloned.
- The deep optional parameter indicates whether deep cloning is required, that is, whether the child nodes under the targetNode need to be cloned. The default is false.
give an example:
<body> <div id="container"> <div class="header">This is the head</div> <div class="body"> <div class="content">Content I</div> <div class="content">Content II</div> </div> </div> <script> const target = document.querySelector(".body"); const cloneNode1 = target.cloneNode(); console.log("cloneNode1.outerHTML\n\n",cloneNode1.outerHTML); const cloneNode2 = target.cloneNode(true); console.log("cloneNode2.outerHTML\n\n", cloneNode2.outerHTML); </script> </body>
The operation results are as follows:
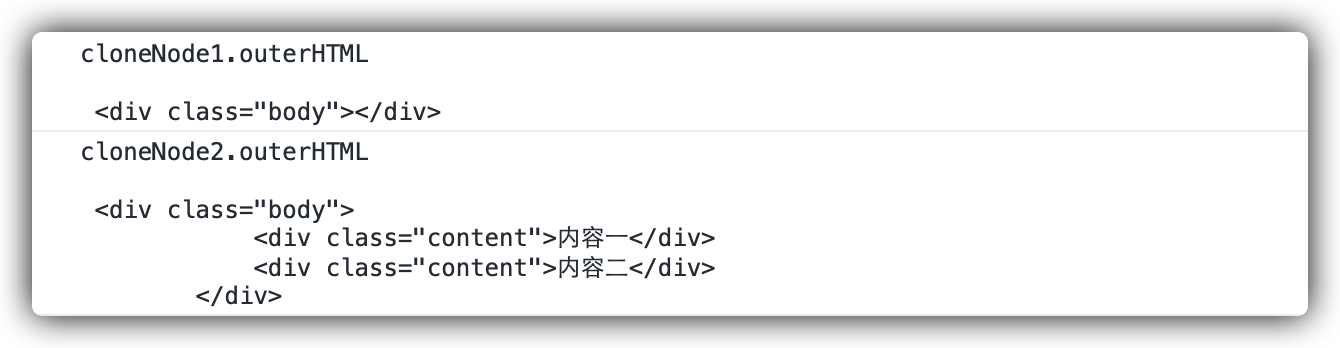
document.importNode()
Copy a node of an external document, and then insert the copied node into the current document. The syntax is as follows:
let node = document.importNode(externalNode, deep);
- Node is the node object imported externally into the current document.
- externalNode is the target node to be imported in the external document.
- Whether deep copy is deep copy. The default is false.
give an example:
<!--iframe.html--> <body> <h1>This is Iframe page</h1> <div id="container"> <div class="header">This is Iframe Content header</div> <div class="body">This is Iframe Content subject</div> </div> </body> <!--index.html--> <body> <div id="container"> <div class="header">Content header</div> <div class="body">Content subject</div> </div> <iframe id="iframe_ele" src="./iframe.html"></iframe> <script> window.onload = function () { const iframeEle = document.getElementById('iframe_ele'); const iframeContainer = iframeEle.contentDocument.getElementById("container"); const importedNode = document.importNode(iframeContainer, true); document.body.appendChild(importedNode); } </script> </body>
The final effect is as follows:
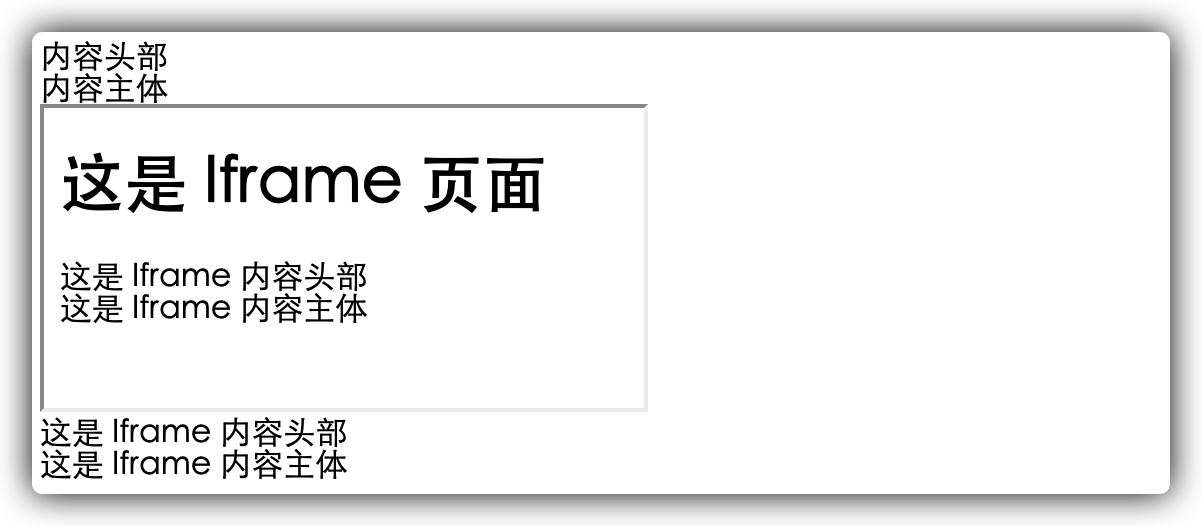
document.adoptNode()
Get a node from other document documents. The node and all nodes in its subtree will be deleted from the original document (if there is this node), and its ownerDocument attribute will become the current document document. Then you can insert this node into the current document. Syntax:
let node = document.adoptNode(externalNode);
- Node the node object obtained from the external document.
- externalNode is the node object in the external document to be imported.
give an example:
<!--iframe.html--> <body> <h1>This is Iframe page</h1> <div id="container"> <div class="header">This is Iframe Content header</div> <div class="body">This is Iframe Content subject</div> </div> </body> <!--index.html--> <body> <div id="container"> <div class="header">Content header</div> <div class="body">Content subject</div> </div> <iframe id="iframe_ele" src="./iframe.html"></iframe> <script> window.onload = function () { const iframeEle = document.getElementById('iframe_ele'); const iframeContainer = iframeEle.contentDocument.getElementById("container"); const node = document.adoptNode(iframeContainer); document.body.appendChild(node); } </script> </body>
effect:
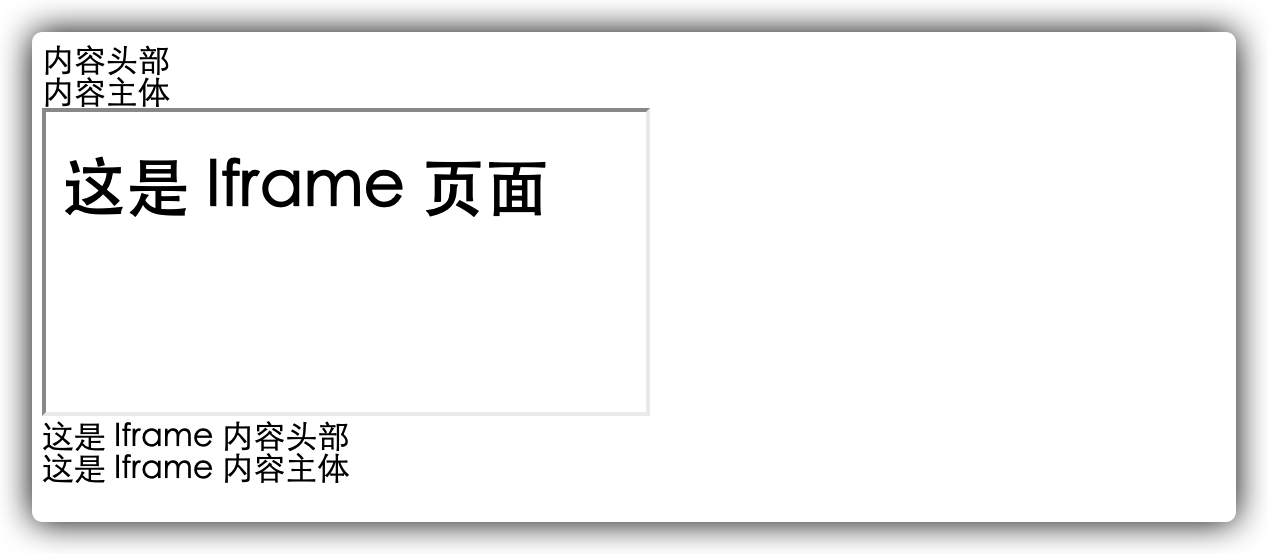
summary
The above is a summary of the methods of cloning (or importing) DOM nodes using JS.
~
~End of this article, thank you for reading!
~
Learn interesting knowledge, make interesting friends and shape interesting souls!
Hello, I'm Programming samadhi Hermit Wang, my official account is " Programming samadhi "Welcome to pay attention and hope you can give us more advice!