class
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 20:29:28
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Person {
//Property, also known as member variable, is placed outside the method in the class
String name;//name
int age;//Age
double height;//height
double weight;//weight
//Method, behavior
//sleep
public void sleep(){
System.out.println("I was sleeping!");
}
//having dinner
public void eat(){
System.out.println("I'm having a meal!");
}
}
object
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 20:36:32
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Test01 {
public static void main(String[] args) {
//Create an object (instance) of the Person class
Person p = new Person();
//His name is sqlboy
p.name = "sqlboy";
//Age 25
p.age = 25;
//Height 178
p.height = 178;
//Weight 145
p.weight = 145;
//sqlboy starts eating
p.eat();
//sqlboy began to sleep
p.sleep();
}
}
Local and member variables
Distinguishing type | local variable | Member variable |
---|
Different location in code | Method, variables defined in the code block | Variables defined outside the method in the class |
Code scope is different | Current method or code block | Many methods of the current class |
Is there a default value | No default value | There are default values |
Do you want to initialize | It must be initialized, or an error will be reported | No, it is recommended to assign value when using |
Different locations in memory | Stack memory | Heap memory |
Different action time | The current method or current code block is executed from the beginning to the end | Current object from creation to destruction |
- Default value of member variable
Type category | Type subclass | Default value |
---|
Basic type | byte | (byte)0 |
Basic type | short | (short)0 |
Basic type | int | 0 |
Basic type | long | 0L |
Basic type | float | 0.0f |
Basic type | double | 0.0d |
Basic type | char | '\u0000' (null) |
Basic type | boolean | false |
reference type | / | null |
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 21:00:34
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Test02 {
//If a member variable is defined and not initialized, the default value will be used
byte a;
short b;
int c;
long d;
float e;
double f;
char g;
boolean h;
Object i;
public static void main(String[] args) {
//Create Test02 object
Test02 t = new Test02();
//Call the print method of t to print
t.print();
}
//Print
public void print(){
System.out.println(a+"\t"+b+"\t"+"\t"+c+"\t"+d+"\t"+e+"\t"+f+"\t"+g+"\t"+h+"\t"+i);
}
}
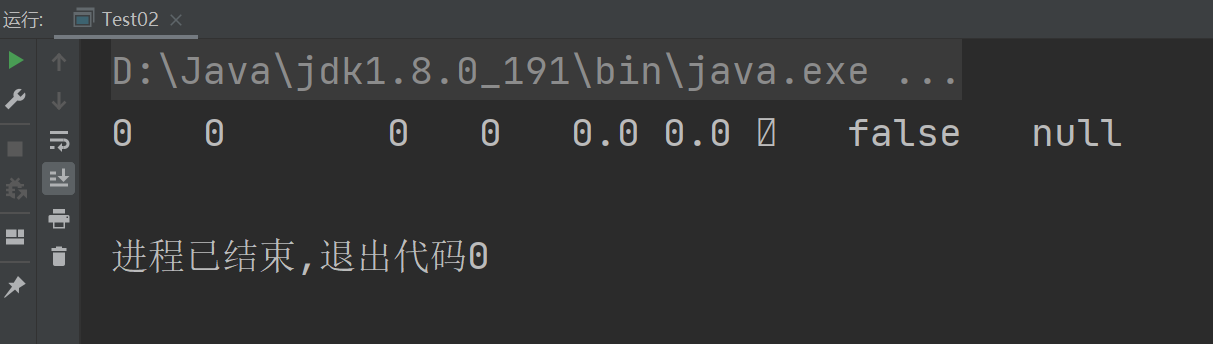
constructor
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 21:10:41
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Person1 {
//Define attributes
String name;
int age;
//Empty constructor, default constructor
//When there are other overloaded constructors, it is not shown to write out this constructor, then the parameterless constructor does not exist
public Person1(){};
//Constructor Overload
public Person1(String name, int age){
this.name = name;
this.age = age;
}
public static void main(String[] args) {
/*
Process of creating objects:
1.When encountering Person1 for the first time, load the class (only once)
2.Create an object to make room in the heap for this object
3.Initialize the properties of the object
new Keyword is actually calling a method, which is called constructor
When calling the constructor, if there is no constructor written in your class, the system will assign a default empty constructor by default
Difference between constructor and method:
1.There is no return value type for the method
2.A return statement cannot be inside a method body
3.The constructor name is very special and must be consistent with the class name
Function of constructor: it is not to create an object. Before calling the constructor, the object has been created and the properties have
The default initialized value. The constructor is called to assign values to attributes
Note: generally, the initialization operation is not carried out in the empty constructor, because in that case, the properties of each object are the same
We just need to ensure that the empty constructor exists, and there is no need to write anything in it
*/
Person1 p1 = new Person1("sqlboy", 25);
System.out.println("My name is"+p1.name+"!");//My name is sqlboy!
System.out.println("I this year"+p1.age+"Years old!");//I'm 25 years old!
}
}
this
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 21:34:03
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Person2 {
String name;
int age;
public Person2(){}
public Person2(String name, int age){
this.name = name;//this refers to the current object
this.age = age;//this refers to the current object
}
public void eat(){
int age = 10;
System.out.println(age);//According to the proximity principle, age refers to the nearest age and the local variable age, so the output is 10
System.out.println(this.age);//Age of the current object, member variable age
System.out.println(name);//There is no duplicate name. It is the member variable name directly
}
public void play(){
/*this.*/eat();//Methods in the same class can call each other, and this can be ignored without writing
System.out.println("I'm going to play after dinner!");
}
public static void main(String[] args) {
Person2 p2 = new Person2("sqlboy", 25);
p2.play();
}
}
static
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 21:59:47
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Person3 {
String name;
int age;
//static modifier attribute
static int count;//Direct class name can be called
//static modification method
//static and public are modifiers. They are in parallel and have no order. Whoever writes first can write later
public static void print(){
System.out.println("I am a static method, which can be called directly by the class name!");
}
public static void main(String[] args) {
/*
When the class is loaded, the static content will be loaded into the static field of the method area,
Static content precedes the existence of objects. This static content is shared by all objects of this class
*/
System.out.println(Person3.count);//0
Person3.print();//I am a static method, which can be called directly by the class name!
}
}
- summary
- When the class is loaded, it is loaded into the static field in the method area
- Exists before object
- Access method: object name Property name class name Attribute name (recommended)
Code block
- Class composition: attributes, methods, constructors, code blocks, and internal classes
- Code block classification: ordinary block, construction block, static block, synchronous block (multithreading)
package com.yuzhenc.oop;
/**
* @author: yuzhenc
* @date: 2022-02-22 22:14:54
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Test03 {
//attribute
int a;
static int sa;
//method
public void a(){
System.out.println("-----a");
{
//Ordinary blocks limit the scope of local variables
System.out.println("This is an ordinary block");
System.out.println("----000000");
int num = 10;
System.out.println(num);
}
//System.out.println(num);
//if(){}
//while(){}
}
public static void b(){
System.out.println("------b");
}
//Tectonic block
{
System.out.println("------This is a building block");
}
//Static block
static{
System.out.println("-----This is a static block");
//Only methods can be used in static blocks: static attributes, static methods
System.out.println(sa);
b();
}
//constructor
public Test03(){
System.out.println("This is an empty constructor");
}
public Test03(int a){
this.a = a;
}
//This is a main method, which is the entry of the program:
public static void main(String[] args) {
Test03 t = new Test03();
t.a();
Test03 t2 = new Test03();
t2.a();
}
}
- Code block execution order
- The static block is executed first and only once when the class is loaded, so it is generally used to write projects in the future: create factories and put the initialization information of the database into the static block, which is generally used to perform some global initialization operations
- Re execute building blocks (not commonly used)
- Re execution constructor
- Common block in re execution method
package
- Function of package: to solve the problem of duplicate names (in fact, the package corresponds to the directory on the drive letter)
- Package name definition
- All lowercase names
- For intermediate use separate
- Generally, the company domain name is written upside down
- Add module name
- Keywords in the system cannot be used
- The location of the package declaration is usually on the first line of non annotative code
- After the package is imported, if you want to use the class with the same name under other packages, you must write the package manually
//Declaration package
package com.yuzhenc.oop;
import java.util.Date;Guide bag: it is for positioning
/**
* @author: yuzhenc
* @date: 2022-02-22 22:25:02
* @desc: com.yuzhenc.oop
* @version: 1.0
*/
public class Test04 {
//This is a main method, which is the entry of the program:
public static void main(String[] args) {
new Person();
new Date();
new java.sql.Date(1000L);//After the package is imported, if you want to use the class with the same name under other packages, you must write the package manually
System.out.println(Math.random());//java. Packages under Lang can be used directly
}
}
- summary
- Classes under different packages need to import packages: import * *; For example: import Java util. Date;
- After the package is imported, if you want to use the class with the same name under other packages, you must write the package manually
- Classes under the same package can be used directly without importing packages
- In Java Classes under Lang package can be used directly without importing package