03_Java basic syntax
The Scanner class cannot get the char type directly from the keyboard
Process control
- Process control statements are statements used to control the execution order of statements in the program. Statements can be combined into small logic modules that can complete certain functions.
- Its process control mode adopts three basic process structures specified in structured programming, namely:
- Sequential structure: the program is executed line by line from top to bottom without any judgment and jump.
- Branch structure:
- Selectively execute a piece of code according to conditions.
- There are two branch statements: if... else and switch case.
- Cyclic structure
- Execute a piece of code repeatedly according to the loop conditions.
- There are three loop statements: while, do... While and for.
- Note: jdk1 5 provides a foreach loop to easily traverse sets and array elements.
Sequential structure
The execution order of sequential structure is generally from top to bottom
Branching structure
if-else
The three structures of if else are as follows:
If else nested structure
/* If else in branch structure 1, Three structures First: if(Conditional expression){ Execute expression } Second: choose one from two if(Conditional expression){ Execute expression 1 }else{ Execute expression 2 } Third: choose one from n if(Conditional expression){ Execute expression 1 }else if(Conditional expression){ Execute expression 2 }else if(Conditional expression){ Execute expression 3 } ... else{ Execute expression n } */ class IfTest { public static void main(String[] args) { //Example 1 int heartBeats = 79; if(heartBeats < 60 || heartBeats > 100){ System.out.println("Further examination is required"); } System.out.println("End of inspection"); //Example 2 int age = 23; if(age < 18){ System.out.println("You can also watch cartoons"); }else{ System.out.println("You can watch adult movies"); } //Example 3 if(age < 0){ System.out.println("The data you entered is illegal"); }else if(age < 18){ System.out.println("Adolescence"); }else if(age < 35){ System.out.println("Young adults"); }else if(age < 60){ System.out.println("Middle age"); }else if(age < 120){ System.out.println("Old age"); }else{ System.out.println("Are you going to be an immortal~~"); } } }
If else exercise 1
/* Yue Xiaopeng took the Java exam. He and his father Yue buqun made a commitment: If: When the score is 100 points, a BMW will be rewarded; When the score is (80, 99], an iphone xs max will be rewarded; When the score is [60,80], reward an iPad; At other times, there is no reward. Please input Yue Xiaopeng's final grade from the keyboard and judge it explain: 1. else Structure is optional. That is, else is optional. Write it according to your needs 2. For conditional expressions: > If multiple conditional expressions are mutually exclusive (or have no intersection), it doesn't matter which judgment and execution statement is declared above or below. > If there is an intersection relationship between multiple conditional expressions, you need to consider which structure should be declared on it according to the actual situation. > If there is an inclusive relationship between multiple conditional expressions, it is usually necessary to declare the small range on the large range. Otherwise, those with small scope will have no chance to implement. */ import java.util.Scanner; class IfTest { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Please enter Yue Xiaopeng's final grade:(0-100)"); int score = scan.nextInt(); if(score == 100){ System.out.println("Reward one BMW");//be my wife! BMW <---> MSN }else if(score > 80 && score <= 99){ System.out.println("Reward one iphone xs max"); }else if(score >= 60 && score <= 80){ System.out.println("Reward one iPad"); }else{ System.out.println("There's no reward"); } } }
If else exercise 2
/* Programming: input three integers from the keyboard and store them into variables num1, num2 and num3 respectively, They are sorted (using if else if else) and output from small to large. explain: 1. if-else Structures can be nested with each other. 2. If the execution statement in the if else structure has only one line, the corresponding pair of {} can be omitted. However, it is not recommended to omit. When there are no curly braces, else is the proximity principle, which is paired with the nearest if above else. */ import java.util.Scanner; class IfTest2 { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Please enter the first integer:"); int num1 = scanner.nextInt(); System.out.println("Please enter the second integer:"); int num2 = scanner.nextInt(); System.out.println("Please enter the third integer:"); int num3 = scanner.nextInt(); if(num1 >= num2){ if(num3 >= num1) System.out.println(num2 + "," + num1 + "," + num3); else if(num3 <= num2) System.out.println(num3 + "," + num2 + "," + num1); else System.out.println(num2 + "," + num3 + "," + num1); }else{ if(num3 >= num2) System.out.println(num1 + "," + num2 + "," + num3); else if(num3 <= num1) System.out.println(num3 + "," + num1 + "," + num2); else System.out.println(num1 + "," + num3 + "," + num2); } } }
The formula for obtaining the value of [a,b] interval using random numbers is as follows:
Formula: (int)(Math.random() * (b-a + 1)*a)
If else exercise 3
/* As we all know, men should be married and women should be married. If the woman's parents want to marry their daughter, of course, they must put forward certain conditions: Height: above 180cm; Wealth: wealth of more than 10 million; Shuai: Yes. If these three conditions are met at the same time, then: "I must marry him!!!" If the three conditions are true, then: "marry, less than the top, more than the bottom." If the three conditions are not met, then: "do not marry!" */ import java.util.Scanner; class IfExer1 { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Please enter your height:(cm)"); int height = scan.nextInt(); System.out.println("Please enter your wealth:(must)"); double wealth = scan.nextDouble(); /* Mode 1: System.out.println("Please enter whether you are handsome: (true/false) "); boolean isHandsome = scan.nextBoolean(); if(height >= 180 && wealth >= 1 && isHandsome){ System.out.println("I must marry him!!! "); }else if(height >= 180 || wealth >= 1 || isHandsome){ System.out.println("Marry, not enough than the top, more than the bottom. "); }else{ System.out.println("Don't marry! "); } */ //Mode 2: System.out.println("Please enter whether you are handsome:(yes/no)"); String isHandsome = scan.next(); if(height >= 180 && wealth >= 1 && isHandsome.equals("yes")){ System.out.println("I must marry him!!!"); }else if(height >= 180 || wealth >= 1 || isHandsome.equals("yes")){ System.out.println("Marry, not enough than the top, more than the bottom."); }else{ System.out.println("Don't marry!"); } } }
switch-case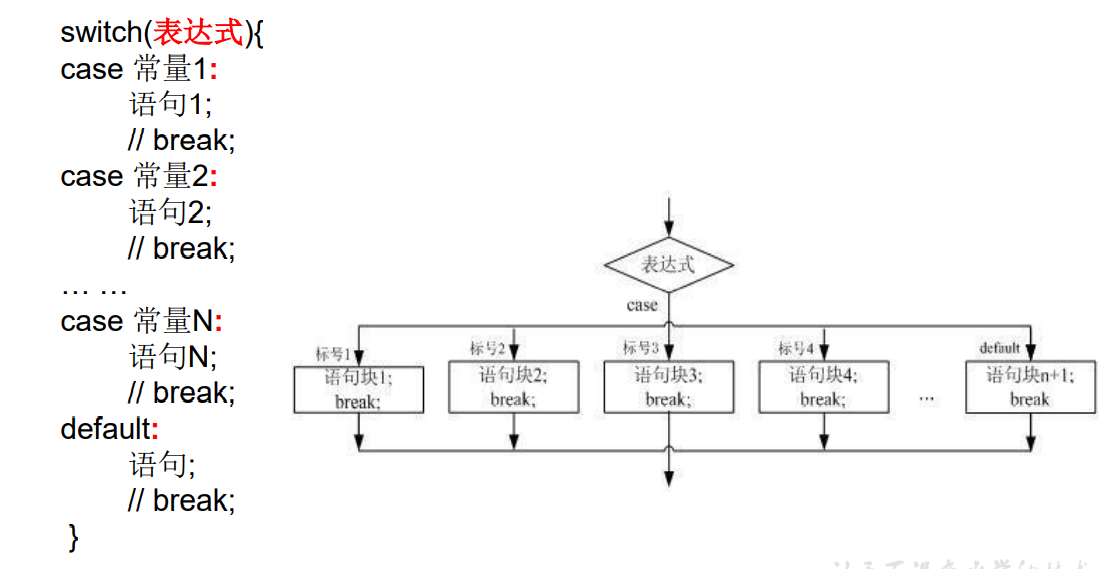
Relevant rules of switch statement
- The value of the expression in switch (expression) must be one of the following types: byte, short, char, int, enumeration (jdk 5.0), String (jdk 7.0);
- The value in the case clause must be a constant, not a variable name or an uncertain expression value;
- For the same switch statement, the constant values in all case clauses are different from each other;
- Break statement is used to make the program jump out of the switch statement block after executing a case branch; If there is no break, the program will execute to the end of switch in sequence
- The default clause is optional. At the same time, the location is also flexible. When there is no matching case, execute default
/* Branch structure 2: switch case 1.format switch((expression){ case Constant 1: Execute statement 1; //break; case Constant 2: Execute statement 2; //break; ... default: Execute statement n; //break; } 2.explain: ① Match the constants in each case in turn according to the value in the switch expression. Once the match is successful, it enters the corresponding case structure and calls its execution statement. After the execution statement is called, the execution statements in other case structures (i.e. case penetration) will continue to be executed downward until the break keyword or the end of this switch case structure is encountered. In a switch case structure, if there is no break statement in the case execution statement, there will be multiple output values. If only one value needs to be output, the break statement will be added to the case execution statement. ② break,It can be used in the switch case structure to indicate that once this keyword is executed, it will jump out of the switch case structure ③ switch The expression in the structure can only be one of the following six data types: byte ,short,char,int,Enumeration type (new in JDK5.0), String type (new in JDK7.0) ④ case Only constants can be declared later. Scope cannot be declared. Examples are in the following code ⑤ break Keywords are optional. ⑥ default:Equivalent to else in if else structure default The structure is optional and the position is flexible. */ class SwitchCaseTest { public static void main(String[] args) { int number = 5; switch(number){ case 0: System.out.println("zero"); break; case 1: System.out.println("one"); break; case 2: System.out.println("two"); break; case 3: System.out.println("three"); break; default: System.out.println("other"); //break; } String season = "summer"; switch (season) { case "spring": System.out.println("in the warm spring , flowers are coming out with a rush"); break; case "summer": System.out.println("Summer heat"); break; case "autumn": System.out.println("fresh autumn weather"); break; case "winter": System.out.println("Snow in winter"); break; default: System.out.println("Season input error"); break; } //**************The following two cases fail to compile********************* //Situation 1 /* boolean isHandsome = true; switch(isHandsome){ case true: System.out.println("I'm so handsome ~ ~ "); break; case false: System.out.println("I'm so ugly ~ ~ "); break; default: System.out.println("Incorrect input ~ ~ "); } */ //Situation II /* int age = 10; switch(age){ case age > 18: System.out.println("Adult) "; break; default: System.out.println("Minors "); } */ } }
Switch case exercise 1
/* Example: for students whose scores are greater than 60, output "qualified". If the score is lower than 60, the output is "unqualified". Note: if the execution statements of multiple cases in the switch case structure are the same, you can consider merging. */ class SwitchCaseTest1 { public static void main(String[] args) { /* int score = 78; switch(score){ case 0: case 1: case 2: ... case 100: } */ /*Use if else int score = 78; if(score >= 60){ }else{ } */ int score = 78; switch(score / 10){ //When the execution statements are the same, merging can be performed case 0: case 1: case 2: case 3: case 4: case 5: System.out.println("fail,"); break; case 6: case 7: case 8: case 9: case 10: System.out.println("pass"); break; } //Better solution: switch(score / 60){ case 0: System.out.println("fail,"); break; case 1: System.out.println("pass"); break; } } }
Switch case exercise 2
/* Programming: input "month" and "day" of 2019 from the keyboard, and the date input through program output is the day of 2019. 2 15: 31 + 15 5 7: 31 + 28 + 31 + 30 + 7 .... Note: break is optional in switch case */ import java.util.Scanner; class SwitchCaseTest2 { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Please enter 2019 month: "); int month = scan.nextInt(); System.out.println("Please enter 2019 day: "); int day = scan.nextInt(); //Define a variable to hold the total number of days int sumDays = 0; //Mode 1: redundancy /* if(month == 1){ sumDays = day; }else if(month == 2){ sumDays = 31 + day; }else if(month == 3){ sumDays = 31 + 28 + day; }else if(month == 4){ sumDays = 31 + 28 + 31 + day; } //... else{//month == 12 //sumDays = ..... + day; } */ //Mode 2: redundancy /* switch(month){ case 1: sumDays = day; break; case 2: sumDays = 31 + day; break; case 3: sumDays = 31 + 28 + day; break; ... } */ //Use the penetration of case statement without writing break statement switch(month){ case 12: sumDays += 30; case 11: sumDays += 31; case 10: sumDays += 30; case 9: sumDays += 31; case 8: sumDays += 31; case 7: sumDays += 30; case 6: sumDays += 31; case 5: sumDays += 30; case 4: sumDays += 31; case 3: sumDays += 28; case 2: sumDays += 31; case 1: sumDays += day; } System.out.println("2019 year" + month + "month" + day + "The first day of the year" + sumDays + "day"); } }
Switch case exercise 3
/* Enter the year, month and day respectively from the keyboard to judge the day of the year Note: criteria for judging whether a year is a leap year: 1)It can be divided by 4, but not by 100 or 2)It can be divided by 400 explain: 1. Any structure that can use switch case can be converted to if else. On the contrary, it does not hold. 2. When we write the branch structure, we find that we can use switch case (at the same time, there are not many values of expressions in switch), When you can also use if else, we prefer to use switch case. Reason: the execution efficiency of switch case is slightly higher. */ import java.util.Scanner; class SwitchCaseExer { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Please enter year: "); int year = scan.nextInt(); System.out.println("Please enter month: "); int month = scan.nextInt(); System.out.println("Please enter day: "); int day = scan.nextInt(); //Define a variable to hold the total number of days int sumDays = 0; switch(month){ case 12: sumDays += 30; case 11: sumDays += 31; case 10: sumDays += 30; case 9: sumDays += 31; case 8: sumDays += 31; case 7: sumDays += 30; case 6: sumDays += 31; case 5: sumDays += 30; case 4: sumDays += 31; case 3: //sumDays += 28; //Determine whether year is a leap year if((year % 4 == 0 && year % 100 != 0 ) || year % 400 == 0){ sumDays += 29; }else{ sumDays += 28; } case 2: sumDays += 31; case 1: sumDays += day; } System.out.println(year + "year" + month + "month" + day + "The first day of the year" + sumDays + "day"); } }
Note:
Enter the student's grade, and then output the exercises of the grade
Comparison of switch and if statements
if and switch statements are very similar. In what specific scenario, which statement should be applied?
-
If there are few specific values to judge, and they conform to byte, short, char, int, String, enumeration and other types. Although both statements can be used, the swtich statement is recommended. Because the efficiency is slightly higher.
-
Other situations: for interval judgment and boolean judgment, if is used. If is used in a wider range. In other words, if you use switch case, it can be rewritten as if else. Otherwise, it does not hold.
Cyclic structure
-
Loop structure: repeatedly execute the function of specific code when some conditions are met
-
Circular statement classification
-
for loop
-
while loop
-
Do while loop
-
Cycle structure diagram:
for loop
-
Syntax format
for (① initialization part; ② loop condition part; ④ iteration part)
③ circulating body part;
}
-
Execution process: ① - ② (enter the cycle when the cycle conditions are met) - ③ - ④ - ② - ③ - ④ - ② - ③ - ④ -... - ② (exit the cycle when the cycle conditions are not met)
As shown in the figure below:
-
explain:
- ② The loop condition part is a boolean type expression. When the value is false, exit the loop
- ① The initialization part can declare multiple variables, but they must be of the same type, separated by commas
- ④ There can be multiple variable updates, separated by commas
for loop exercise 1
/* For Use of circular structure 1, Four elements of circular structure ① Initialization condition ② Loop condition -- > is of boolean type ③ Circulatory body ④ Iterative condition 2, Structure of for loop for(①;②;④){ ③ } Execution process: ① - ② - ③ - ④ - ② - ③ - ④ -- ② */ class ForTest { public static void main(String[] args) { /* System.out.println("Hello World!"); System.out.println("Hello World!"); System.out.println("Hello World!"); System.out.println("Hello World!"); System.out.println("Hello World!"); */ for(int i = 1;i <= 5;i++){//i:1,2,3,4,5 System.out.println("Hello World!"); } //i: Valid within a for loop. The for loop fails. //System.out.println(i); //practice: int num = 1; for(System.out.print('a');num <= 3;System.out.print('c'),num++){ System.out.print('b'); } //Output result: abcbc System.out.println(); //Example: traverse even numbers within 100, output the sum of all even numbers, and output the number of even numbers int sum = 0;//Record the sum of all even numbers int count = 0;//Record the number of even numbers for(int i = 1;i <= 100;i++){ if(i % 2 == 0){ System.out.println(i); sum += i; count++; } //System.out.println("the sum is:" + sum "); } System.out.println("The sum is:" + sum); System.out.println("Number:" + count); } }
for loop exercise 2
/* Write a program to cycle from 1 to 150 and print a value on each line, In addition, print "foo" on each multiple line of 3, Print "biz" on each multiple line of 5, Print out "baz" on each multiple line of 7. */ class ForTest1 { public static void main(String[] args) { for(int i = 1;i <= 150;i++){ System.out.print(i + " "); //Separate if structures are used instead of else if because when a number is a multiple of 3, 5 and 7 at the same time, it can be output as required if(i % 3 == 0){ System.out.print("foo "); } if(i % 5 == 0){ System.out.print("biz "); } if(i % 7 == 0){ System.out.print("baz "); } //Line feed System.out.println(); } } }
for loop exercise 3
/* Title: enter two positive integers m and n to find their maximum common divisor and minimum common multiple. For example, the maximum common divisor of 12 and 20 is 4 and the minimum common multiple is 60. Note: use of break keyword: once break is executed in the loop, it will jump out of the loop */ import java.util.Scanner; class ForTest{ public static void main(String[] args){ Scanner scan = new Scanner(System.in); System.out.println("Please enter the first positive integer:"); int m = scan.nextInt(); System.out.println("Please enter the second positive integer:"); int n = scan.nextInt(); //Get the maximum common divisor //1. Get the smaller of the two numbers int min = (m <= n)? m : n; //2. Traversal for(int i = min;i >= 1 ;i--){ if(m % i == 0 && n % i == 0){ System.out.println("The maximum common divisor is:" + i); break;//Once the break is executed in the loop, it jumps out of the loop } } //Get least common multiple //1. Get the larger of the two numbers int max = (m >= n)? m : n; //2. Traversal for(int i = max;i <= m * n;i++){ if(i % m == 0 && i % n == 0){ System.out.println("Least common multiple:" + i); break; } } } }
while Loop
-
Syntax format
-
① Initialization part
while(② cycle condition part)
③ circulating body part;
④ iterative part;
}
-
-
Execution process:
- ① - ② (enter the cycle when the cycle conditions are met) - ③ - ④ - ② - ③ - ④ - ② - ③ - ④ -... - ② (exit the cycle when the cycle conditions are not met)
-
explain:
- Be careful not to forget the declaration part. Otherwise, the cycle will not end and become an dead cycle.
- for loops and while loops can be converted to each other
/* While Use of recycling 1, Four elements of circular structure ① Initialization condition ② Loop condition -- > is of boolean type ③ Circulatory body ④ Iterative condition 2, Structure of while loop ① while(②){ ③; ④; } Execution process: ① - ② - ③ - ④ - ② - ③ - ④ -- ② explain: 1.When writing a while loop, be careful not to lose the iteration condition. Once lost, it may lead to a dead cycle! 2.We write programs to avoid dead cycles. 3.for Loop and while loop can be converted to each other! Difference: the scope of the initialization condition part of the for loop and the while loop is different. for The conditions of loop initialization can only be used in the for loop. When the conditions of while loop initialization are out, the while loop can still be used Algorithm: finiteness. */ class WhileTest{ public static void main(String[] args) { //Traverse all even numbers within 100 int i = 1; while(i <= 100){ if(i % 2 == 0){ System.out.println(i); } i++; } //After the while loop is out, it can still be called. System.out.println(i);//101 } }
do-while Loop
-
Syntax format
① Initialization part;
do{
③ Circulating body part
④ Iterative part
}while(② cycle condition part);
-
Execution process: ① - ③ - ④ - ② - ③ - ④ - ② - ③ - ④ -... ②
-
Note: the do while loop executes the loop body at least once.
Do while exercises
/* do-while Use of recycling 1, Four elements of circular structure ① Initialization condition ② Loop condition -- > is of boolean type ③ Circulatory body ④ Iterative condition 2, Do while loop structure: ① do{ ③; ④; }while(②); Execution process: ① - ③ - ④ - ② - ③ - ④ -- ② explain: 1.do-while The loop will execute the loop body at least once! 2.In development, use for and while more. Less use of do while */ class DoWhileTest { public static void main(String[] args) { //Traverse even numbers within 100 and calculate the sum of all even numbers and the number of even numbers int num = 1; int sum = 0;//Record sum int count = 0;//Number of records do{ if(num % 2 == 0){ System.out.println(num); sum += num; count++; } num++; }while(num <= 100); System.out.println("The sum is:" + sum); System.out.println("Number:" + count); //*************Realize that do while executes the loop body at least once*************** int number1 = 10; while(number1 > 10){ System.out.println("hello:while"); number1--; } int number2 = 10; do{ System.out.println("hello:do-while"); number2--; }while(number2 > 10); } }
Circular exercise:
/* Title: Read an uncertain number of integers from the keyboard, and judge the number of positive and negative numbers. Enter 0 to end the program. explain: 1. Structure that does not limit the number of times in the loop condition part: for(;) Or while(true) 2. How many ways to end the cycle? Method 1: the loop condition part returns false Method 2: execute break in the loop body */ import java.util.Scanner; class ForWhileTest { public static void main(String[] args) { Scanner scan = new Scanner(System.in); int positiveNumber = 0;//Record the number of positive numbers int negativeNumber = 0;//Record the number of negative numbers for(;;){//while(true){ int number = scan.nextInt(); //Judge the positive and negative of number if(number > 0){ positiveNumber++; }else if(number < 0){ negativeNumber++; }else{ //Once the break is executed, the loop jumps out break; } } System.out.println("The number of positive numbers entered is:" + positiveNumber); System.out.println("The number of negative numbers entered is:" + negativeNumber); } }
Nested loops (multiple loops)
- A nested loop is formed when one loop is placed in another loop. Among them, for, while, do... While can be used as outer loop or inner loop.
- In essence, nested loops treat the inner loop as the loop body of the outer loop. Only when the loop condition of the inner loop is false, will it completely jump out of the inner loop, end the current loop of the outer loop and start the next loop.
- If the number of outer layer cycles is m times and the number of inner layer cycles is n times, the inner layer cycle body actually needs to execute m*n times.
practice
/* Use of nested loops 1.Nested loop: declaring one loop structure A in the loop body of another loop structure B constitutes A nested loop 2. Outer circulation: circulation structure B Inner circulation: circulation structure A 3. explain ① The inner loop structure is traversed once, which is only equivalent to the execution of the outer loop body once ② Suppose that the outer loop needs to be executed m times and the inner loop needs to be executed n times. At this time, the loop body of the inner loop has been executed m * n times 4. skill: The outer loop controls the number of rows and the inner loop controls the number of columns */ class ForForTest { public static void main(String[] args) { //****** //System.out.println("******"); for(int i = 1;i <= 6;i++){ System.out.print('*'); } System.out.println("\n"); /* ****** ****** ****** ****** */ for(int j = 1;j <= 4;j++ ){ for(int i = 1;i <= 6;i++){ System.out.print('*'); } System.out.println(); } /* i((line number) j(*) * 1 1 ** 2 2 *** 3 3 **** 4 4 ***** 5 5 */ for(int i = 1;i <= 5;i++){//Number of control rows for(int j = 1;j <= i;j++){//Number of control columns System.out.print("*"); } System.out.println(); } /* i((line number) The law of j(*) is: i + j = 5, in other words: j = 5 - i; **** 1 4 *** 2 3 ** 3 2 * 4 1 */ for(int i = 1;i <= 4;i++){ for(int j = 1;j <= 5 - i;j++){ System.out.print("*"); } System.out.println(); } /* * ** *** **** ***** **** *** ** * */ //slightly /*It is equivalent to the use of interval compensation method Use the blank character before * to replace it ----* ---* * --* * * -* * * * * * * * * * * * * * * * * * * */ class Test1{ public static void main(String[] args){ for( int i = 0; i < 5 ; i++){ //Output the blank part, that is, the part of "-" for(int j = 0 ; j < 4-i ; j++){ System.out.print(" "); } //Output upper part* for(int k = 0 ; k <i + 1; k++){ System.out.print("* "); } //Line feed System.out.println(); } //Lower half for(int i = 0 ; i < 4 ; i++){ for(int j = 0 ; j < i+1 ; j++){ System.out.print(" "); } for(int k = 0 ; k<4-i ; k++){ System.out.print("* "); } //Line feed System.out.println(); } } } } }
99 multiplication table exercise
/* Application of nested loops 1: multiplication table 1 * 1 = 1 2 * 1 = 2 2 * 2 = 4 . . . 9 * 1 = 9 . . . 9 * 9 = 81 */ class NineNineTable { public static void main(String[] args) { for(int i = 1;i <= 9;i++){ for(int j = 1;j <= i;j++){ System.out.print(i + " * " + j + " = " + (i * j) + " "); } System.out.println(); } } }
Output prime within 0-100
/* 100 The output of all prime numbers within. Prime: prime, a natural number that can only be divided by 1 and itself. -- > From 2 to the end of the number - 1, it cannot be divided by the number itself. The smallest prime number is: 2 */ class PrimeNumberTest { public static void main(String[] args) { boolean isFlag = true;//Identify whether i is completely divided by j. once it is completely divided, modify its value for(int i = 2;i <= 100;i++){//Traverse natural numbers within 100 for(int j = 2;j < i;j++){//j: Removed by i if(i % j == 0){ //i is divided by j isFlag = false; } } // if(isFlag == true){ System.out.println(i); } //Reset isFlag isFlag = true; } } } //Optimization mode 1 /* 100000 The output of all prime numbers within. Implementation mode I Prime: prime, a natural number that can only be divided by 1 and itself. -- > From 2 to the end of the number - 1, it cannot be divided by the number itself. For primenumbertest Optimization of prime number output in Java file */ class PrimeNumberTest1 { public static void main(String[] args) { boolean isFlag = true;//Identify whether i is completely divided by j. once it is completely divided, modify its value int count = 0;//Record the number of prime numbers //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long start = System.currentTimeMillis(); for(int i = 2;i <= 100000;i++){//Traversing natural numbers within 100000 //Optimization 2: it is effective for natural numbers that are prime numbers. //for(int j = 2;j < i;j++){ for(int j = 2;j <= Math.sqrt(i);j++){//j: Removed by i if(i % j == 0){ //i is divided by j isFlag = false; break;//Optimization 1: it is only effective for natural numbers that are not prime numbers. } } // if(isFlag == true){ //System.out.println(i); count++; } //Reset isFlag isFlag = true; } //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long end = System.currentTimeMillis(); System.out.println("The number of prime numbers is:" + count); System.out.println("The time spent is:" + (end - start));//17110 - optimization 1: break:1546 - optimization 2: 13 } } //Optimization mode II /* 100000 The output of all prime numbers within. Implementation mode 2 Prime: prime, a natural number that can only be divided by 1 and itself. -- > From 2 to the end of the number - 1, it cannot be divided by the number itself. For primenumbertest Optimization of prime number output in Java file */ class PrimeNumberTest2 { public static void main(String[] args) { int count = 0;//Record the number of prime numbers //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long start = System.currentTimeMillis(); label:for(int i = 2;i <= 100000;i++){//Traversing natural numbers within 100000 //Using: m = a * b, then a=m/b, b=m/a, //Therefore, when a midpoint is taken between a and B, m/a and m/b are equivalent to symmetry for(int j = 2;j <= Math.sqrt(i);j++){//j: Removed by i if(i % j == 0){ //i is divided by j continue label; } } //All that can perform this step are prime numbers count++; } //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long end = System.currentTimeMillis(); System.out.println("The number of prime numbers is:" + count); System.out.println("The time spent is:" + (end - start));//17110 - optimization 1: break:1546 - optimization 2: 13 } }
Use of special Keywords: break, continue
break statement
Use of labels
label:for(int i = 1;i <= 4;i++){ for(int j = 1;j <= 10;j++){ if(j % 4 == 0){ //break;// By default, the nearest loop of this keyword will pop up. //continue; //break label;// End the one-layer loop structure of the specified ID continue label;//End the cycle of the one-layer cycle structure of the specified ID } System.out.print(j); } System.out.println(); }
continue Statement
Example:
/* break Use of and continue keywords Scope of use Functions used in the loop (different points) Same point break: switch-case In cyclic structure End current cycle You cannot declare an execution statement after a keyword continue: In cyclic structure End the current cycle You cannot declare an execution statement after a keyword */ class BreakContinueTest { public static void main(String[] args) { for(int i = 1;i <= 10;i++){ if(i % 4 == 0){ break;//123 //continue;//123567910 //System.out.println("delireba offers me tonight!!!"); } System.out.print(i); } System.out.println("\n"); //****************************** label:for(int i = 1;i <= 4;i++){ for(int j = 1;j <= 10;j++){ if(j % 4 == 0){ //break;// By default, the nearest loop of this keyword will pop up. //continue; //break label;// End the one-layer loop structure of the specified ID continue label;//End the cycle of the one-layer cycle structure of the specified ID } System.out.print(j); } System.out.println(); } } }
Use the labeled continue statement to output prime numbers
/* 100000 The output of all prime numbers within. Implementation mode 2 Prime: prime, a natural number that can only be divided by 1 and itself. -- > From 2 to the end of the number - 1, it cannot be divided by the number itself. For primenumbertest Optimization of prime number output in Java file */ class PrimeNumberTest2 { public static void main(String[] args) { int count = 0;//Record the number of prime numbers //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long start = System.currentTimeMillis(); label:for(int i = 2;i <= 100000;i++){//Traversing natural numbers within 100000 for(int j = 2;j <= Math.sqrt(i);j++){//j: Removed by i if(i % j == 0){ //i is divided by j continue label; } } //All that can perform this step are prime numbers count++; } //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long end = System.currentTimeMillis(); System.out.println("The number of prime numbers is:" + count); System.out.println("The time spent is:" + (end - start));//17110 - optimization 1: break:1546 - optimization 2: 13 } }
return statement
- Special process control statement 3: return
- Return is not specifically used to end a loop. Its function is to end a method. When a method executes a return statement, the method will be terminated.
- Unlike break and continue, return directly ends the entire method, no matter how many layers of loops the return is in
Description of special process control statement
- break can only be used in switch statements and loop statements.
- continue can only be used in circular statements.
- The two functions are similar, but continue is to terminate this cycle and break is to terminate this layer of cycle
- There can be no other statements after break and continue, because the program will never execute the following statements.
- The label statement must immediately follow the head of the loop. Label statements cannot be used before acyclic statements.
- Many languages have goto statements. Goto statements can transfer control to any statement in the program at will, and then execute it. But it makes the program error prone. break and continue in Java are different from goto.
###Measure the advantages and disadvantages of a function code:
1. Correct line
2. Readability
3. Robustness
Recognize the nearest loop of this keyword.
//continue;
//break label;// End the one-layer loop structure of the specified ID continue label;//End the cycle of the one-layer cycle structure of the specified ID } System.out.print(j); } System.out.println(); } }
}
==Use labeled continue Statement to implement the output prime== ```java /* 100000 The output of all prime numbers within. Implementation mode 2 Prime: prime, a natural number that can only be divided by 1 and itself. -- > From 2 to the end of the number - 1, it cannot be divided by the number itself. For primenumbertest Optimization of prime number output in Java file */ class PrimeNumberTest2 { public static void main(String[] args) { int count = 0;//Record the number of prime numbers //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long start = System.currentTimeMillis(); label:for(int i = 2;i <= 100000;i++){//Traversing natural numbers within 100000 for(int j = 2;j <= Math.sqrt(i);j++){//j: Removed by i if(i % j == 0){ //i is divided by j continue label; } } //All that can perform this step are prime numbers count++; } //Gets the number of milliseconds between the current time and 1970-01-01 00:00:00 long end = System.currentTimeMillis(); System.out.println("The number of prime numbers is:" + count); System.out.println("The time spent is:" + (end - start));//17110 - optimization 1: break:1546 - optimization 2: 13 } }
return statement
- Special process control statement 3: return
- Return is not specifically used to end a loop. Its function is to end a method. When a method executes a return statement, the method will be terminated.
- Unlike break and continue, return directly ends the entire method, no matter how many layers of loops the return is in
Description of special process control statement
- break can only be used in switch statements and loop statements.
- continue can only be used in circular statements.
- The two functions are similar, but continue is to terminate this cycle and break is to terminate this layer of cycle
- There can be no other statements after break and continue, because the program will never execute the following statements.
- The label statement must immediately follow the head of the loop. Label statements cannot be used before acyclic statements.
- Many languages have goto statements. Goto statements can transfer control to any statement in the program at will, and then execute it. But it makes the program error prone. break and continue in Java are different from goto.
###Measure the advantages and disadvantages of a function code:
1. Correct line
2. Readability
3. Robustness
4. High efficiency (time) and low storage (space)