ArcGIS API for JavaScript 4.18 adds a new development method @ arcgis/core based on ES Modules. This article will introduce how to use this method for development.
Write in front
With the development of front-end mainstream frameworks Vue and react, do you also use ArcGIS API for JavaScript in Vue or react projects when developing WebGIS projects? Before, when we used ArcGIS API for JavaScript in Vue or react projects, we always recommended the AMD method of ESRI loader, The sample code is as follows (only the use in react project is demonstrated here, and the use in Vue is shown in the second half of the article):
//Use the then() method of ESRI loader directly to chain callback import React,{Component} from 'react'; import esriLoader from 'esri-loader'; import './App.css'; class App extends Component{ state = { } componentDidMount = () => { const _self = this; const options = { url: 'https://js.arcgis.com/4.14/init.js', / / the API address here can be the CDN provided on the official website or the address of offline deployment can be configured here css: 'https://js.arcgis.com/4.14/esri/themes/light/main.css' }; esriLoader.loadModules([ "esri/Map", "esri/views/MapView"], options) // Pass in the class you want to use .then(([Map, MapView ]) => { // doSomeThing let map = new Map({ basemap: 'osm' }); let view = new MapView({ container: "app", map: map, center: [104.072044,30.663279], zoom: 10 }); }) .catch(err => { console.error('Map initialization failed', err); }) } render () { return ( <div className='App' id='app'> </div> ) } } export default App; //Or like this //Use async and await of ES7 directly import React, { Component } from 'react'; import { loadModules } from 'esri-loader'; import './App.css'; const options = { url: 'https://js.arcgis.com/4.17/', css: 'https://js.arcgis.com/4.17/esri/themes/light/main.css', }; class App extends Component { componentDidMount = () => { this.initMap(); }; initMap = async () => { const [Map, MapView] = await loadModules( ['esri/Map', 'esri/views/MapView'], options ); const map = new Map({ basemap: 'osm', }); const view = new MapView({ container: 'mapview', map: map, zoom: 10, }); }; render() { return <div id="mapview"></div>; } } export default App;
Above, we have demonstrated two ways of using ArcGIS API for JavaScript development through ESRI loader in React project. For the use of Vue, please refer to the following article, which has a detailed introduction process. The list of articles is as follows:
- [Fan Wai] Vue is developed using ArcGIS JS API 4.14
- [Fan Wai] ArcGIS JS API 4.14 is used in React
- [Fan Wai] ArcGIS JS API development using @ arcgis/cli scaffold
No matter how we optimize the above methods, there is a problem: if we need an API module in ArcGIS API for JavaScript somewhere in the component code, we need to load it asynchronously through the loadModules method of ESRI loader, and then we can carry out the corresponding function development. This is actually very inconvenient for our code writing. We prefer an API module of ArcGIS API for JavaScript. We can directly introduce it at the top of the component code, so that this API module can be used anywhere in the component code. In fact, such a way of use cannot be realized through ESRI loader, unless you seal the interface of ArcGIS API for JavaScript according to the needs of the project.
However, with the release of ArcGIS API for JavaScript 4.18, this embarrassing situation has been broken. ArcGIS API for JavaScript 4.18 adds a new way of use - @ arcgis/core. In this way, we can directly introduce the required API module at the top of the component, and then use it anywhere in the component code.
Specific operation
1. Initialize a Vue or React project demo (if you won't create it, just read the three articles mentioned above, which are described in detail). Here, for demonstration, I created a React project demo. After the project demo is created, open the command line window in the root directory of the project demo as shown on the official website, and then install the package @ arcgis/core:
npm install @arcgis/core
After the installation, we can install it in package The version number after installation can be seen in the JSON file, which is actually the version number of the API:
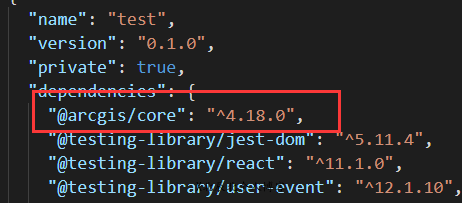
2. After the installation is completed, we open package. In the root directory of the project JSON file, modify the project startup command:
"scripts": { "start": "npm run copy && react-scripts start", "build": "npm run copy && react-scripts build", "copy": "ncp ./node_modules/@arcgis/core/assets ./public/assets" },
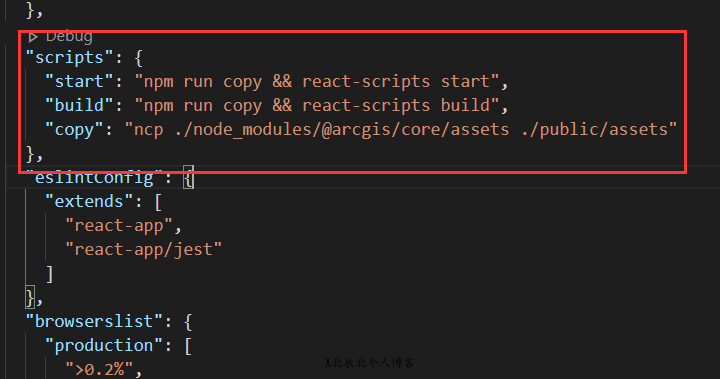
In the above startup commands, we modified the default start and build commands, and then added a copy command, which mainly copies the static resources in the @ arcgis/core package to the public directory of the project.
You can see that the ncp command is used in the copy command, so we need to install the tool globally through the following commands:
npm install ncp -g
3. And then in the app. Of React project JS or index JS file, import the style file package required by ArcGIS API for JavaScript through the following command, as follows:
import '@arcgis/core/assets/esri/themes/light/main.css';
4. Finally, the required API module is introduced at the top of the component code file. Here we only create a two-dimensional map, so the code is directly written in app JS file, as follows:
import Map from '@arcgis/core/Map'; import MapView from '@arcgis/core/views/MapView';
5. Write a life cycle function, and then write the code to instantiate the two-dimensional map in the function, as follows:
componentDidMount = () => { this.initMap(); }; initMap = () => { const map = new Map({ basemap: 'osm', }); const view = new MapView({ container: 'mapview', map: map, zoom: 10, }); };
6. Finally, you can see the results as follows:
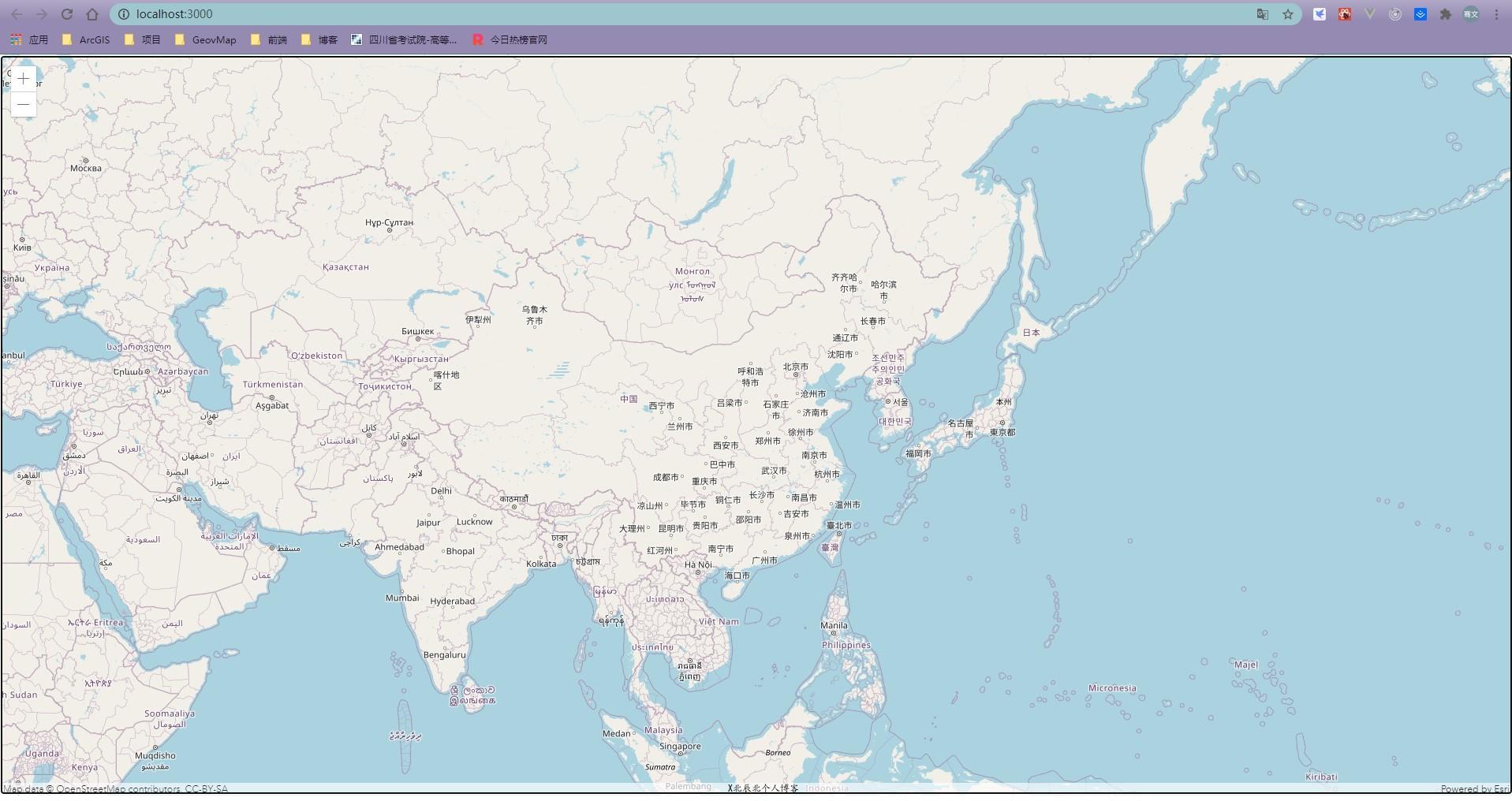
7. The above is all about the introduction of @ arcgis/core mode.
summary
With the emergence of @ arcgis/core, the current development method of using ArcGIS API for JavaScript in Vue or React projects has changed from the previous one to the current two methods: ESRI loader and @ arcgis/core. Because it is a new way of use, it is only a beta version at present, but it can meet most of the development needs. As for the functions currently developed by bloggers, there have been no problems, so you can rest assured to use it. However, in @ arcgis/core, there is no way to use a specific version of API, because if you install @ arcgis/core through NPM install, the API contained in it is the latest version 4.18 by default. Here is just a guess, maybe through npm install @arcgis/core@4.17.0 In this way, you can install the API of version 4.17, but you haven't tried it, maybe not, because @ arcgis/core only appeared on 4.18. Interested partners can try it by themselves.
Finally, let's take a look at the difference between the ES Modules mode of @ arcgis/core and the AMD mode of traditional ESRI loader. The following figure is a screenshot of the official website, which roughly compares the differences between the two modes:
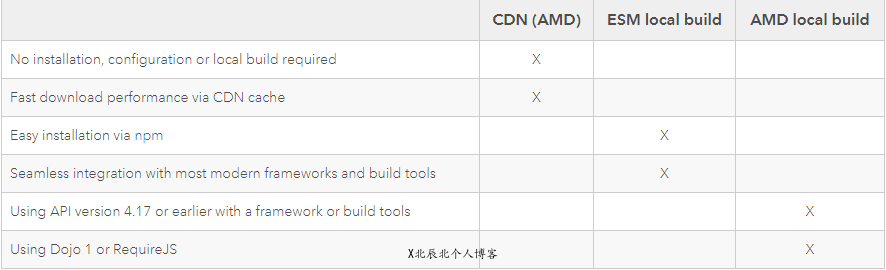