1, Foreword
this article will mainly explain the dynamic parameterized form of use case design in interface testing. In addition, there is a portal of a series of articles below, which is still being updated. Interested partners can also go to check it. Let's have a look together without saying much~
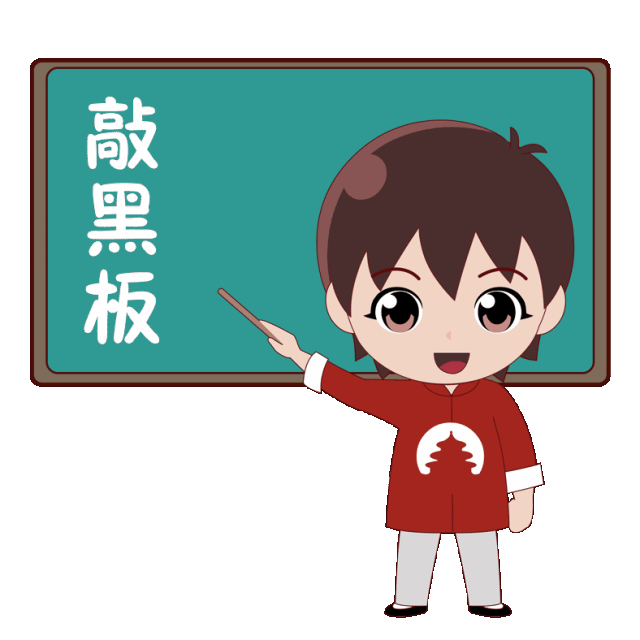
Series of articles:
Series 1: Meet the beauty of Python automation 1
Series 2: [Python automated test 2] Python installation and configuration and basic use of PyCharm
series 3: [Python automated test 3] get to know data types and basic syntax
series 4: [Python automated test 4] string knowledge summary
Series 5: [Python automated test 5] list and tuple knowledge summary
series article 6: [Python automated test 6] dictionary and collection knowledge summary
series article 7: [Python automated test 7] data operator knowledge collection
series 8: [Python automated test 8] explanation of process control statement
series 9: [Python automated test 9] function knowledge collection
series article 10: [Python automated test 10] file basic operation
series article 11: [Python automated test 11] module, package and path knowledge collection
article series 12: [Python automated test 12] knowledge collection of exception handling mechanism
series article 13: [Python automated test 13] collection of class, object, attribute and method knowledge
series article 14: [Python automated test 14] basic and advanced exercises of Python automated test
article series 15: [Python automated testing 15] core concepts and functions of unittest testing framework
article series 16: [Python automated test 16] test case data separation
series article 17: [Python automated test 17] openpyxl secondary packaging and data driving
series article 18: [Python automated test 18] configuration file analysis and practical application
article series 19: [Python automated test 19] explanation of logging system
article series 20: [Python automated testing 20] construction of interface automated testing framework model
article series 21: [Python automated testing 21] interface automated testing practice I_ Interface concept, project introduction and test process Q & A
series article 22: [Python automated test 22] interface Automated Test Practice II_ Interface framework modification and use case optimization
2, Dynamic parameterization
for example, when designing a test case of registration interface, we will encounter an obvious bottleneck. The mobile phone number is not registered. The registration can be successfully completed when the automatic test case is executed for the first time, but it will be found that the mobile phone number already exists when the test case is executed for the second time, and the execution of the test case will fail, In the face of this situation, there are the following solutions:
""" Solution: 1,Each time it is opened manually excel After deletion, re-enter a new mobile phone number 2,Query the mobile phone number in the database. If the mobile phone number already exists, delete the mobile phone number in the database 3,The last number on an existing phone number+1 4,Randomly generate a mobile phone number Train of thought analysis: 1,Although the first method can be solved, it needs to be replaced manually every time, which is not convenient for maintenance 2,Although the mobile phone number can be queried and deleted, the real project will not easily delete the database, and a registered mobile phone number will be associated with a table, and in most cases, the test does not have permission to delete 3,This is also a solution. We can use the 11th digit every time+1,The disadvantage is that it will eventually encounter numbers that may conflict with other mobile phone numbers, resulting in execution failure, but the efficiency is much higher than 1 and 2 4,The final method is also dynamic parameterization. Mark the data to be replaced. When the loop traverses the mark, replace the mark with the randomly generated number, so that it can pass smoothly when executing the use case """
dynamic parameterization is to solve this kind of problem, so that the mobile phone number can be changed continuously and randomly, so that every execution will not cause use case failure due to the repetition of mobile phone number (using dynamic parameterization may still be random to the registered number, but the probability is very low), and data forgery is required to randomly generate a mobile phone number.
3, Data forgery
in the field of automated testing, data forgery is not something that destroys the system security, but hopes to automatically generate the data of test cases and the data conforms to certain rules, such as mobile phone number, e-mail, etc. Data forgery can be used in automatic registration module, login or other text input boxes to detect certain input rules, etc.
data forgery Library in Python - faker library, which can be installed through pip first:
import faker # Initialize the faker object and specify that the generation rule area is China fk = faker.Faker(locale="zh_CN") result = fk.phone_number() print(f"cell-phone number:{result}") # Randomly generate an address company = fk.company() print(f"address:{company}") # Randomly generate a company address = fk.address() print(f"company:{address}") # Randomly generate a city city = fk.city() print(f"city:{city}")
in addition to the standard forgery library, we can also formulate a rule in the way we want:
def generate_new_phone(): phone = "1" + random.choice(["3", "5", "7", "8", "9"]) for i in range(9): num = random.randint(0, 9) phone += str(num) return phone print(f"Functional mobile number:{generate_new_phone()}")
simple package forgery library function. If you write data forgery code in the framework, you can put it in common and import it from common to generate some data:
import faker def generate_new_phone(): fk = faker.Faker(locale="zh_CN") result = fk.phone_number() return result
# Modified fragment code (if you have any questions about fragment code, please refer to the preceding article): @ddt class TestLogin(unittest.TestCase): @list_data(data) def test_login_success(self, case_data): json_data = case_data["json"] if "#new_phone#" in json_data: new_phone = data_forgery.generate_new_phone() json_data = json_data.replace("#new_phone", new_phone)
well, that's all the content of this article. Have you learned it? I hope it can help you!