1, Foreword
this article will mainly explain the basic theory of Web automated testing in Python, the construction of Webdriver environment and some basic common operations of automated testing. In addition, there is a portal of a series of articles below, which is still being updated. Interested partners can also go to check it. Without much talk, let's have a look~
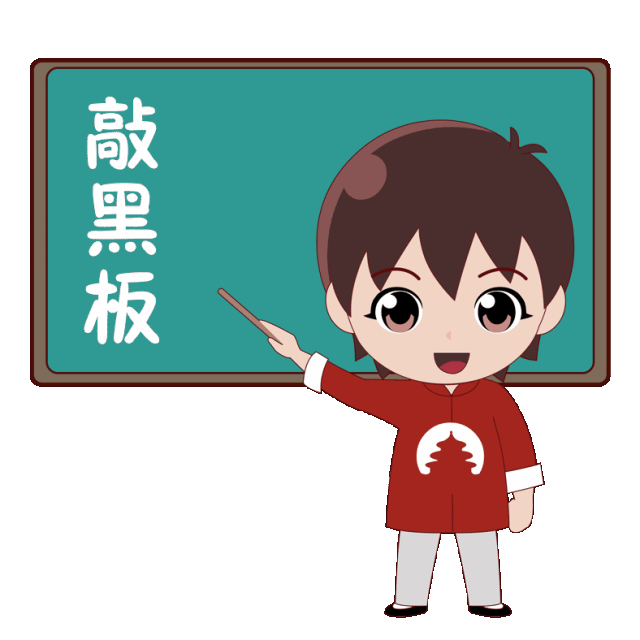
Series of articles:
Series 1: [Python automated test 1] meet the beauty of Python
Series 2: [Python automated test 2] Python installation and configuration and basic use of PyCharm
series 3: [Python automated test 3] get to know data types and basic syntax
series 4: [Python automated test 4] string knowledge summary
Series 5: [Python automated test 5] list and tuple knowledge summary
series article 6: [Python automated test 6] dictionary and collection knowledge summary
series article 7: [Python automated test 7] data operator knowledge collection
series 8: [Python automated test 8] explanation of process control statement
series 9: [Python automated test 9] function knowledge collection
series article 10: [Python automated test 10] file basic operation
series article 11: [Python automated test 11] module, package and path knowledge collection
article series 12: [Python automated test 12] knowledge collection of exception handling mechanism
series article 13: [Python automated test 13] collection of class, object, attribute and method knowledge
series article 14: Advanced Python test automation
article series 15: [Python automated testing 15] core concepts and functions of unittest testing framework
article series 16: [Python automated test 16] test case data separation
Article series: [Python automated test 17] openpyxl secondary packaging and data driving
series article 18: [Python automated test 18] configuration file analysis and practical application
article series 19: [Python automated test 19] explanation of logging system
article series 20: [Python automated testing 20] construction of interface automated testing framework model
article series 21: [Python automated testing 21] interface automated testing practice I_ Interface concept, project introduction and test process Q & A
series article 22: [Python automated test 22] interface Automated Test Practice II_ Interface framework modification and use case optimization
series article 23: [Python automated testing 23] interface automated testing practice III_ Dynamic parameterization and data forgery
article series 24: [Python automated testing 24] interface automated testing practice IV_ Python operation database
series 25: [Python automated testing 25] interface automated testing practice V_ Database assertion, Interface Association and related management optimization
series article 26: [Python automated test 26] interface automated test practice 6_ pytest framework + allure explanation
2, Detailed explanation of Web automation test
2.1 what is Web automated testing
what is Web automated testing? Easily understood, Web automated testing is to replace manual testing operations through code or tools. It belongs to simulated manual operation. There are many tools and methods for Web automated testing, mainly including selenium, cypress, playwright, etc. selenium is the main stream at present, and there are many advantages of using selenium:
""" selenium Advantages of 1,selenium Rich data, you can search the data at any time when you have questions and difficulties 2,selenium It's relatively easy to find a job, HR More willing to choose test engineers who will mainstream tools and frameworks 3,Support multiple languages(Java / Python / go / js) 4,Support remote and multi browser 5,The first choice of the industry at present and the mainstream of the industry """
2.2 selenium installation and environment construction
2.2.1 installation foundation dependency and steps
selenium installation requires certain dependencies, which is a necessary condition for installing selenium for automatic testing. There are three main dependencies:
""" selenium Installation dependency: 1,browser (Suggest Google Chrome / Firefox) 2,selenium webdriver 3,python binding """ """ selenium Installation steps: 1,pip install selenium 2,download Webdriver,Chrome The browser can be downloaded through official download or image download, which corresponds to the version of the browser (At present, after testing, the known version 71 is a very compatible version) 3,Put Python Installation directory or anywhere else """
2.2.2 Webdriver Download
driver download:
corresponding driver version and download link of Google Browser:
super easy to use domestic image source: Domestic image source of Chrome browser
chrome historical version: Chrome historical version
Google official version: Official version of chrom e
corresponding driver version and download link of Edge browser:
Edge browser: Edge browser
corresponding driver version and download link of IE browser:
official version of IE: Github official version
corresponding driver version and download link of Safari browser:
Safari browser: Safari browser
precautions:
the driver must be compatible with the browser version. For example, if the browser version is 71, download the driver of 71
after downloading, put it into the corresponding Python root directory
2.2.3 inspection of successful installation
after the installation is completed, we need to check whether the installation is successful. You can enter the following code and run it to check whether the installation is successful:
# Import selenium package from selenium import webdriver # Open Google browser, Chrome initial capitalization driver = webdriver.Chrome()
2.3 basic use of selenium
first, briefly understand the basic usage syntax of selenium in automated testing. The syntax is a fixed syntax. Just keep it in mind
# Import selenium package from selenium import webdriver # Open Google browser, Chrome initial capitalization driver = webdriver.Chrome() # We need to pay special attention to window maximization. Generally speaking, after we open a browser, the first step is to maximize the window to prevent element positioning failure driver.maximize_window() # Use get to request Baidu page driver.get("https://www.baidu.com") # Print the current browser address print(driver.current_url) # Get page title print(driver.title) # Go to another website driver.get("https://www.iqiyi.com") # back off driver.back() # forward driver.forward() # Refresh driver.refresh() # Close current window driver.close() # Close the entire browser and close the driver chromedriver at the same time driver.quit()
2.4 selenium element positioning
2.4.1 what is element positioning?
we need to know what an element is before we need to locate it. An element is a mark customized by developers in the layout of the web page. We can find a specific precise position by developing the marked elements. This process is called element positioning. For example, if we want to use automatic software to control input, the first step is to find the input box first, Then input can be carried out. We need to locate elements before performing various simulated manual operations such as click, double click and drag
2.4.2 element positioning mode
in selenium, there are 8 major element positioning methods. Refer to the following:
""" selenium Positioning mode of eight elements: 1,id 2,name 3,class name 4,link text 5,partial link text 6,tag name 7,xpath 8,css selector """ """ In fact, there are 30 positioning methods, but in most cases, these 8 methods can be used for accurate positioning """
the way of element positioning is very simple. Taking Baidu's input box as an example, there are two main positioning methods. The author has only one of them to introduce the screenshot below:
""" Mode 1: 1,Press select when entering Baidu page F12 2,Click the check element arrow and click the position of the text input box again 3,View element results -- When selected, there will be a line of special marks in light blue or other colors in Google browser by default """
the first method is as follows:
the second method is as follows:
""" Mode 2: 1,Right click the text input box when entering Baidu page 2,Select"inspect"Button -- Select to automatically navigate to the corresponding row """
2.4.3 Baidu search through element positioning
Baidu search is realized through element positioning. The specific method of elements needs to be determined according to the actual situation. In this search, Baidu's search box can view id elements, so we can use id element positioning. Interested students can also try other existing methods:
""" Realization idea: 1,Initialize browser 2,Open Baidu interface 3,Positioning Baidu text input box 4,input data 5,Click Baidu button """ import time from selenium import webdriver # Initialize a Google browser driver = webdriver.Chrome() # Open Baidu interface driver.get("https://www.baidu.com") # maximize window driver.maximize_window() # Locate the text input box below Baidu through the element id and the value is kw input_text = driver.find_element("id", "kw") # Enter text input_text.send_keys("How to marry Bai Fumei to the peak of life") # Through the element id, the value is su to locate the baidu button baidu_button = driver.find_element("id", "su") # Click the "Baidu click" button baidu_button.click() # Forced wait for 3 seconds time.sleep(3) # Close browser driver.quit()
well, that's all the content of this article. Have you learned it? I hope it can help you!