preface
I came to the library early this morning and thought about a simple and powerful tool of wechat. Recently, there are still a lot of wechat news, such as applet and timeline. This is not the focus. The focus is to see a Python based wechat open source library: itchat. I played all day. Python once said to me, "time is running out, hurry to use python.".
Let's use itchat to make a program like this: undefined private chat withdrawn information can be collected and sent to the file assistant of personal wechat, including: undefined(1) who: who sent the undefined(2) when: when to send the message undefined(3) what: what information undefined(4) which: what kind of information, including text, picture, voice, video, sharing Location, attachment undefined
code implementation
# -*-encoding:utf-8-*- import os import re import shutil import time import itchat from itchat.content import * # Note: text, voice, video, picture, location, business card, sharing and attachment can be withdrawn # {msg_id:(msg_from,msg_to,msg_time,msg_time_rec,msg_type,msg_content,msg_share_url)} msg_dict = {} # Temporary directory for file storage rev_tmp_dir = "/home/alic/RevDir/" if not os.path.exists(rev_tmp_dir): os.mkdir(rev_tmp_dir) # The expression has a problem | accept the MSG of information and note_ ID inconsistency coincidence solution face_bug = None # Store the received messages in the dictionary. When a new message is received, clean up the over time messages in the dictionary | do not accept information without recall function # [TEXT, PICTURE, MAP, CARD, SHARING, RECORDING, ATTACHMENT, VIDEO, FRIENDS, NOTE] @itchat.msg_register([TEXT, PICTURE, MAP, CARD, SHARING, RECORDING, ATTACHMENT, VIDEO]) def handler_receive_msg(msg): global face_bug # Get the local timestamp and format the local timestamp e: 2017-04-21 21:30:08 msg_time_rec = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()) # Message ID msg_id = msg['MsgId'] # Message time msg_time = msg['CreateTime'] # The nickname of the message sender | you can also use remarkmame to make comments here, but you or the person without comments is None msg_from = (itchat.search_friends(userName=msg['FromUserName']))["NickName"] # Message content msg_content = None # Shared links msg_share_url = None if msg['Type'] == 'Text' \ or msg['Type'] == 'Friends': msg_content = msg['Text'] elif msg['Type'] == 'Recording' \ or msg['Type'] == 'Attachment' \ or msg['Type'] == 'Video' \ or msg['Type'] == 'Picture': msg_content = r"" + msg['FileName'] # Save file msg['Text'](rev_tmp_dir + msg['FileName']) elif msg['Type'] == 'Card': msg_content = msg['RecommendInfo']['NickName'] + r" My business card" elif msg['Type'] == 'Map': x, y, location = re.search( "<location x=\"(.*?)\" y=\"(.*?)\".*label=\"(.*?)\".*", msg['OriContent']).group(1, 2, 3) if location is None: msg_content = r"latitude->" + x.__str__() + " longitude->" + y.__str__() else: msg_content = r"" + location elif msg['Type'] == 'Sharing': msg_content = msg['Text'] msg_share_url = msg['Url'] face_bug = msg_content # Update dictionary msg_dict.update( { msg_id: { "msg_from": msg_from, "msg_time": msg_time, "msg_time_rec": msg_time_rec, "msg_type": msg["Type"], "msg_content": msg_content, "msg_share_url": msg_share_url } } ) # After receiving the note notification message, judge whether to withdraw and carry out corresponding operations @itchat.msg_register([NOTE]) def send_msg_helper(msg): global face_bug if re.search(r"\<\!\[CDATA\[.*A message was withdrawn\]\]\>", msg['Content']) is not None: # Gets the id of the message old_msg_id = re.search("\<msgid\>(.*?)\<\/msgid\>", msg['Content']).group(1) old_msg = msg_dict.get(old_msg_id, {}) if len(old_msg_id) < 11: itchat.send_file(rev_tmp_dir + face_bug, toUserName='filehelper') os.remove(rev_tmp_dir + face_bug) else: msg_body = "Tell you a secret~" + "\n" \ + old_msg.get('msg_from') + " Withdrawn " + old_msg.get("msg_type") + " news" + "\n" \ + old_msg.get('msg_time_rec') + "\n" \ + "Withdrawn what ⇣" + "\n" \ + r"" + old_msg.get('msg_content') # If it is sharing, there is a link if old_msg['msg_type'] == "Sharing": msg_body += "\n That's the link➣ " + old_msg.get('msg_share_url') # Send recall message to file assistant itchat.send(msg_body, toUserName='filehelper') # If there are documents, send them back if old_msg["msg_type"] == "Picture" \ or old_msg["msg_type"] == "Recording" \ or old_msg["msg_type"] == "Video" \ or old_msg["msg_type"] == "Attachment": file = '@fil@%s' % (rev_tmp_dir + old_msg['msg_content']) itchat.send(msg=file, toUserName='filehelper') os.remove(rev_tmp_dir + old_msg['msg_content']) # Delete dictionary old messages msg_dict.pop(old_msg_id) if __name__ == '__main__': itchat.auto_login(hotReload=True,enableCmdQR=2) itchat.run()
The program can be run directly on the terminal. If the code scanning on the terminal is successful, you can log in successfully. At the same time, it can also be packaged and run in the window system (note to modify the path, and it is recommended to use the relative path).
➜ ~ python wx.py Getting uuid of QR code. Downloading QR code. Please scan the QR code to log in. Please press confirm on your phone. Loading the contact, this may take a little while. �[3;J Login successfully as AlicFeng Start auto replying.
design sketch
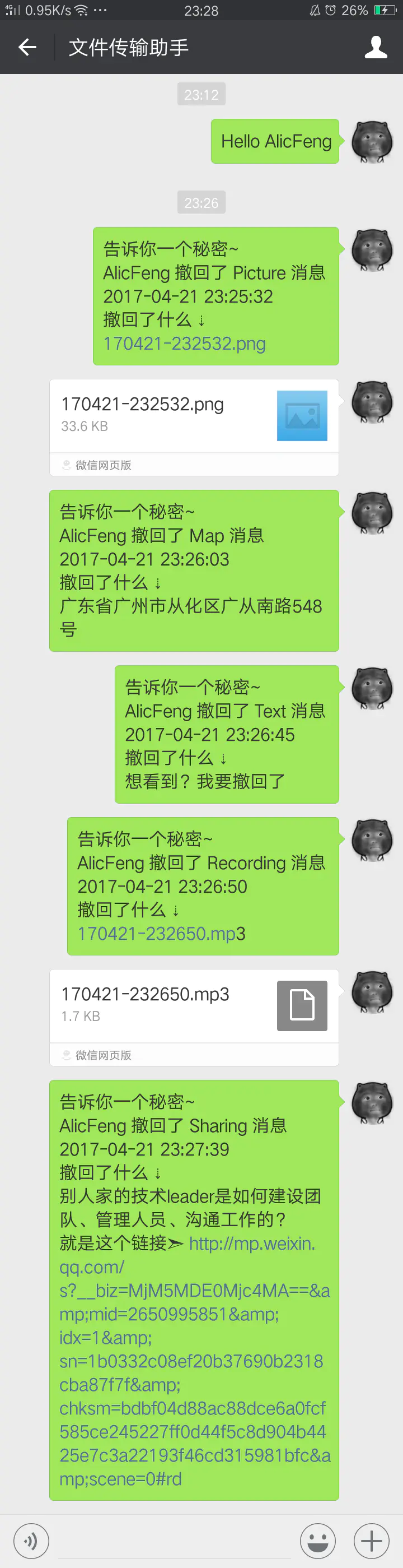
tchat
The above are all trivial matters of programming logic. I'd better record the open source library of itchat wechat.
- Introduction undefineditchat is an open source wechat personal ID interface. It becomes very simple to call wechat using python. Simply use the itchat code to build an instant messaging based on wechat, which is better reflected in the convenience of expanding more communication functions of personal wechat on other platforms.
- install
pip3 install itchat
- Itchat - Hello world defined sends a message to the file assistant in just three lines of code
import itchat itchat.auto_login(hotReload=True) itchat.send('Hello AlicFeng', toUserName='filehelper')
- View client
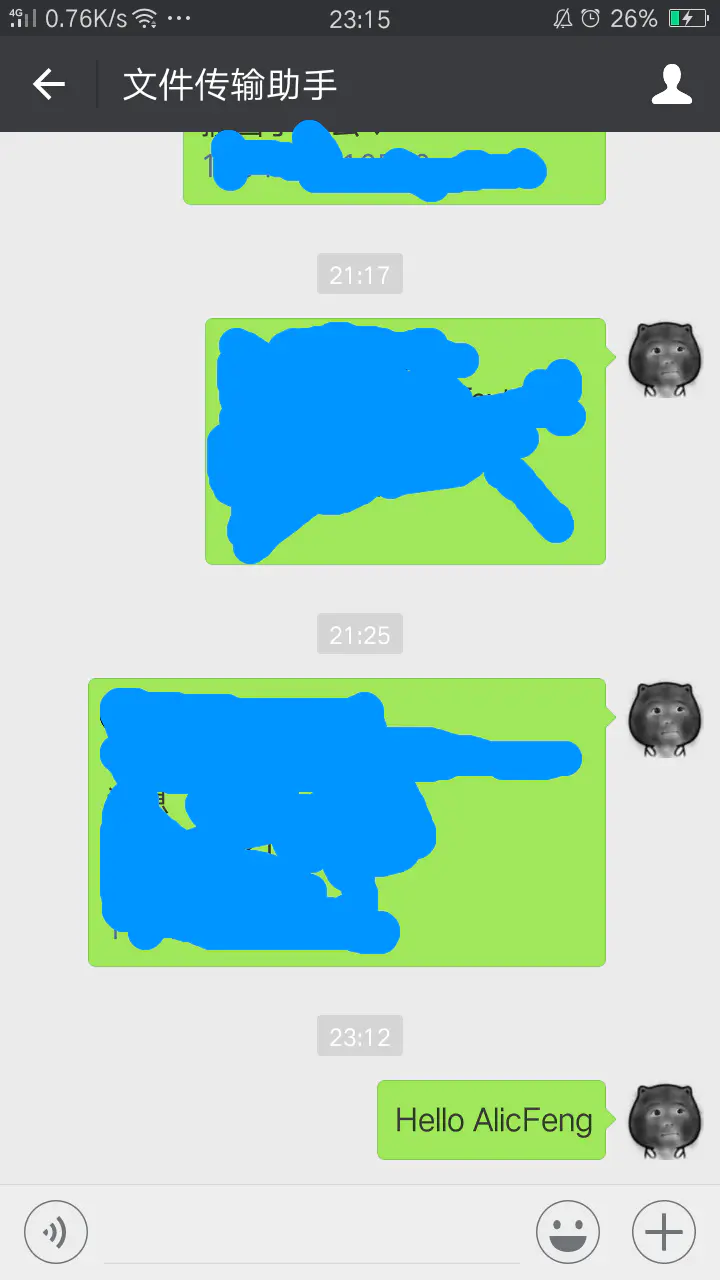
If you find an error or don't understand, you can put it forward in the comment area for everyone to communicate!
If the article is helpful to you, like + pay attention, your support is my biggest motivation