Implement a simple CA system, which includes the following functions:
- Certificate generation: after the user provides a Certificate Signing Request (CSR) and public key, the system will automatically generate a certificate for the user and issue it through email. Two types of certificates for SSL and code signing are supported.
- Certificate revocation: after a user initiates a certificate revocation request, the system will update the Certificate Revocation List (CRL), but considering the system load, the user's revocation request will be temporarily recorded and then updated in days. The client can use the CRL Distribution Point (CRL Distribution Point: the distribution point of the system is: CRL Distribution Point )Get the latest CRL list. www.biyezuopin.vip
- Certificate audit: the user's certificate request can only be issued after being audited by the administrator, so we have written a simple administrator audit function.
- Key pair generation: Based on the jsrssign plug-in of Vue, we support the client to generate RSA public-private key pairs locally.
- User authentication: including login, logout, registration, authentication and other common operations, which are realized by Token.
- Code signature: in addition to the self generating function of CA system, this project also provides a simple code signature tool written in Java to verify the project results.
Final results
Interface documentation
In order to unify front and back-end requests, all requests use POST
CSR file upload
Interface: / api/ca/file
Document identification: CSR_FILE
Return: this interface will return the user's public key and personal information parsed through CSR
{"header":{"code":200,"msg":"ok"},"country":"CN","province":"ShanXi","locality":"TaiYuan","organization":"Zhongbei University","common_name":"junebao.top","email_address":"","organizational_unit":"IT","public_key":"-----BEGIN RSA PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEArj/g1UgvJAEnXxlDFHU6\naMwaaZ7BP4zXXWLnfw5kiVTjMPL4kS21do82bygUg4tCPM3pnDt5BpibGwsAZhQ8\nNH527z0Is2yHUT8S/RWT7t7AAJ06NdsEzdyaKAzVHa3xfq6zjVHc11nn0eLB0M0G\nahteIZebHjNhMX3dyVbvUx9e0iAjPDxbCvficbBDhQ1fzZbUoxmS175ENDuoNRY1\nory8+fFAnRhwTJn12mhB/U+QaHiBIfzhC7exMffcUJYK8WYqt0W0+3oS47gyiCt7\nlEyhzQ9UoJVA7O5zsmB39xPLXHIsRLkuAZBIB9YLibZOse5gRCVgd6OYJTyhHbGl\nRwIDAQAB\n-----END RSA PUBLIC KEY-----\n"}
functional design
System flow
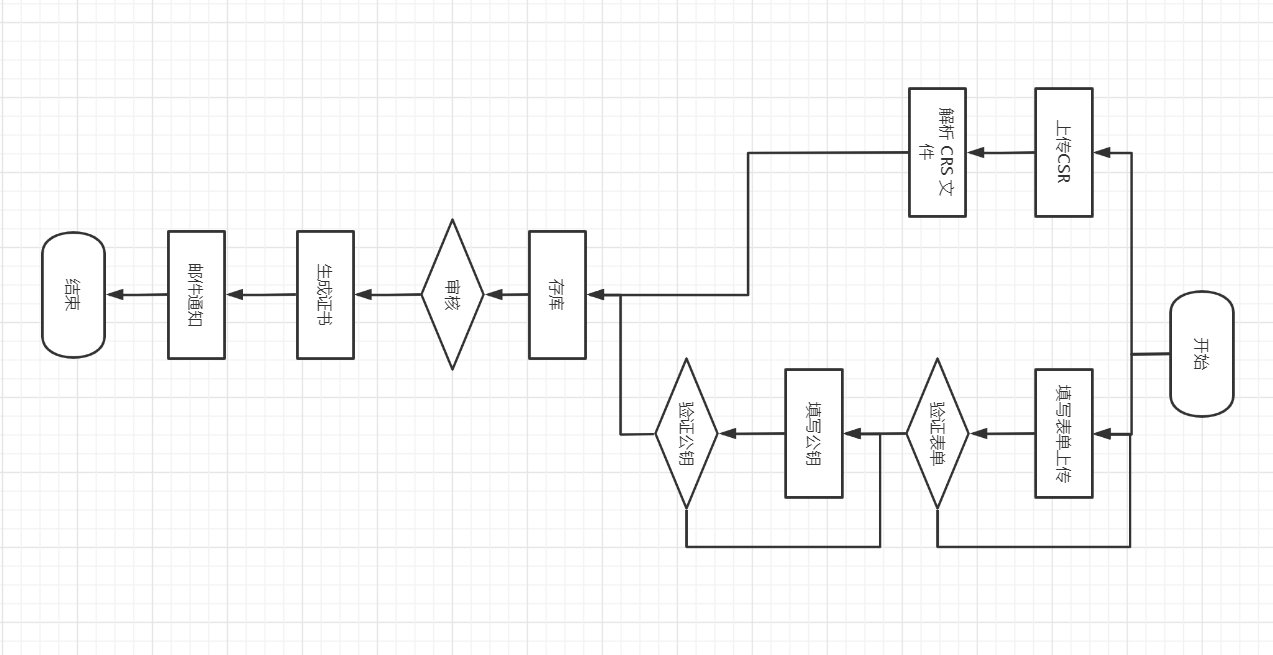
Database design
CREATE TABLE `ca_requests` ( `id` int unsigned NOT NULL AUTO_INCREMENT, `created_at` datetime DEFAULT NULL COMMENT 'Field creation time', `updated_at` datetime DEFAULT NULL COMMENT 'Update time', `deleted_at` datetime DEFAULT NULL COMMENT 'Delete time', `user_id` int NOT NULL COMMENT 'User requesting certificate ID', `state` int unsigned NOT NULL COMMENT 'Certificate status (1: to be approved, 2: approved, 3: failed)', `public_key` text CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL COMMENT 'Public key', `country` varchar(20) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL COMMENT 'country', `province` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL COMMENT 'State and city', `locality` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL COMMENT 'region', `organization` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL COMMENT 'organization', `organization_unit_name` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL COMMENT 'department', `common_name` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL COMMENT 'full name', `email_address` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL COMMENT 'mailbox', PRIMARY KEY (`id`), KEY `idx_ca_requests_deleted_at` (`deleted_at`) ) ENGINE=InnoDB AUTO_INCREMENT=46 DEFAULT CHARSET=utf8;
CREATE TABLE `certificates` ( `id` int unsigned NOT NULL AUTO_INCREMENT, `created_at` datetime DEFAULT NULL, `updated_at` datetime DEFAULT NULL, `deleted_at` datetime DEFAULT NULL, `user_id` int NOT NULL COMMENT 'Certificate owner ID', `state` int unsigned NOT NULL COMMENT 'Status (1 = in use, 2 = revoked or expired)', `request_id` int NOT NULL COMMENT 'Certificate request ID', `expire_time` bigint NOT NULL COMMENT 'Expiration timestamp', PRIMARY KEY (`id`), KEY `idx_certificates_deleted_at` (`deleted_at`) ) ENGINE=InnoDB AUTO_INCREMENT=39 DEFAULT CHARSET=utf8;
CREATE TABLE `crls` ( `id` int unsigned NOT NULL AUTO_INCREMENT, `created_at` datetime DEFAULT NULL, `updated_at` datetime DEFAULT NULL, `deleted_at` datetime DEFAULT NULL, `certificate_id` int NOT NULL COMMENT 'certificate ID', `input_time` bigint NOT NULL COMMENT 'Add timestamp', PRIMARY KEY (`id`), KEY `idx_crls_deleted_at` (`deleted_at`) ) ENGINE=InnoDB AUTO_INCREMENT=17 DEFAULT CHARSET=utf8 COMMENT='Certificate revocation list';
CREATE TABLE `user_tokens` ( `id` int unsigned NOT NULL AUTO_INCREMENT, `created_at` datetime DEFAULT NULL, `updated_at` datetime DEFAULT NULL ON UPDATE CURRENT_TIMESTAMP, `deleted_at` datetime DEFAULT NULL, `user_id` int NOT NULL, `token` varchar(64) NOT NULL, `expire_time` bigint NOT NULL, PRIMARY KEY (`id`), KEY `idx_user_tokens_deleted_at` (`deleted_at`) ) ENGINE=InnoDB AUTO_INCREMENT=81 DEFAULT CHARSET=utf8;
CREATE TABLE `users` ( `id` int unsigned NOT NULL AUTO_INCREMENT, `created_at` datetime DEFAULT NULL, `updated_at` datetime DEFAULT NULL, `deleted_at` datetime DEFAULT NULL, `username` varchar(16) NOT NULL, `password` varchar(255) NOT NULL, `email` varchar(255) DEFAULT NULL, `authority` int DEFAULT NULL COMMENT 'Permission, 1 means system administrator', PRIMARY KEY (`id`), UNIQUE KEY `username` (`username`), KEY `idx_users_deleted_at` (`deleted_at`) ) ENGINE=InnoDB AUTO_INCREMENT=62 DEFAULT CHARSET=utf8;
background knowledge
PKI
encryption algorithm
Symmetric encryption: 3DES
3DES (or Triple DES) is the general name of Triple Data Encryption Algorithm (TDEA) block cipher. It is equivalent to applying DES encryption algorithm three times to each data block. Due to the enhancement of computer computing ability, the key length of the original des password becomes easy to be brutally cracked; 3DES is designed to provide a relatively simple method, that is, by increasing the key length of DES to avoid similar attacks, rather than designing a new block cipher algorithm.
Asymmetric encryption RSA
Hash algorithm
MD5
SHA256
X.509
10. 509 is the format standard of public key certificate in cryptography. X509 certificate has been used in many applications including TLS/SSL
In Internet protocol, it is also used in many non online application scenarios, such as electronic signature service. X509 certificate contains public key, identity information (such as network host name, organization name or individual name, etc.) and signature information (it can be the signature of certificate issuing authority CA or self signature). For a certificate signed by a trusted certificate issuing authority or verified by other means, the owner of the certificate can use the certificate and the corresponding private key to create a secure communication and digitally sign the document In addition, in addition to the function of the certificate itself, x509 also comes with a certificate revocation list and a certificate validity verification algorithm from the certificate issuing authority that finally signs the certificate to the final trusted point.
In X.509, an organization obtains a signed certificate by initiating a certificate signing request (CSR). First, we need to generate a pair of key pairs, then use the private key to sign the CSR and save the private key safely. CSR further contains the identity information of the request initiator, the public key used to verify the request, and the distinguished name of the requested certificate. CSR may also contain other information about identity certificate required by ca. The CA then issues a certificate for the distinguished name and binds a public key Organizations can distribute trusted root certificates to all members so that they can use the company's PKI system.
Certificate format
Version Number: the Version Number of the specification, which is currently version 3 and the value is 0x2;
Serial Number: a column number maintained by CA and assigned to each certificate issued by CA, which is used to track and revoke the certificate. As long as you have the issuer information and Serial Number, you can uniquely identify a certificate, with a maximum of 20 bytes;
Signature Algorithm: the algorithm used for digital signature, such as sha256 with RSA encryption ccdsa with sha2s6;
Issuer: the identification information of the certificate issuing unit, such as "C=CN, St = Beijing, l = Beijing, o = org example.com,CN=ca.org. example.com ”;
Validity: the validity period of the certificate, including the start and end time.
Subject: the Distinguished Name of the certificate owner, such as "C=CN, ST=Beijing, L=Beijing,CN=person.org.example.com";
SubJect Public Key Info: information related to the protected public key:
Public Key Algorithm: the algorithm adopted by the public key;
Subject Unique Identifier: the content of the public key.
Issuer Unique Identifier: the unique information representing the issuer. It is only supported in version 2 and 3. Optional;
Subject Unique Identifier: represents the unique information of the entity with the certificate. It is only supported in version 2 and 3. Optional:
Extensions (optional): optional extensions. It may include: Subject Key Identifier: the secret key identifier of the entity, which distinguishes multiple pairs of secret keys of the entity;
Basic Constraints: 1. Indicate whether it belongs to CA;Authority Key Identifier: the public key identifier of the certificate issuer;
CRL Distribution Points: the issuing address of the revocation file;
Key Usage: the purpose or function information of the certificate.
certificate revocation
In public key infrastructure (PKI), CSR is a file sent by the certificate applicant to the CA organization to issue digital certificates for it. Its common format is PKCS Generally, 10 and SPKAC will contain a user's public key and necessary user information. In X.509 standard, it is listed as follows:
Before generating the certificate, the applicant should form a key pair and keep the private key properly. For the information in CSR, the private key signature should be used to prevent tampering. Therefore, a standard CSR contains the following three parts of information:
- Authentication request information: contains the main applicant information and public key
- Signature algorithm ID:
- CSR signature: the applicant should sign the CSR information with his own private key to ensure that it is not tampered with.
The system allows the applicant to quickly submit personal information and public key by filling in the form, and also allows the applicant to upload CSR files directly.
Method of generating CSR through openSSL locally:
# Generate random private key openssl genrsa -des3 -out server.key 2048 # Issue CSR using private key openssl req -new -nodes -keyout server.key -out server.csr
Module division and personnel division
modular | content | |
---|---|---|
Certificate request | 1. CSR file upload 2. CSR form filling | |
Public key submission User certificate query | ||
Certificate generation | 1. List to be reviewed 2. Issue certificates 3. Reject the request | |
Login registration authentication | 1. Login 2. Registration 3. Logout | |
certificate revocation | 1. Certificate revocation request 2. CRL distribution | |
code signing |