- 1. Bean configuration
- 2. Bean instantiation
- Constructor instantiation
- Static factory instantiation
- Instance factory instantiation
- 3. Scope of bean
- singleton scope
- prototype scope
- 4. Bean life cycle
- 5. Bean assembly method
- XML based assembly
- Annotation based assembly
- automatic assembly
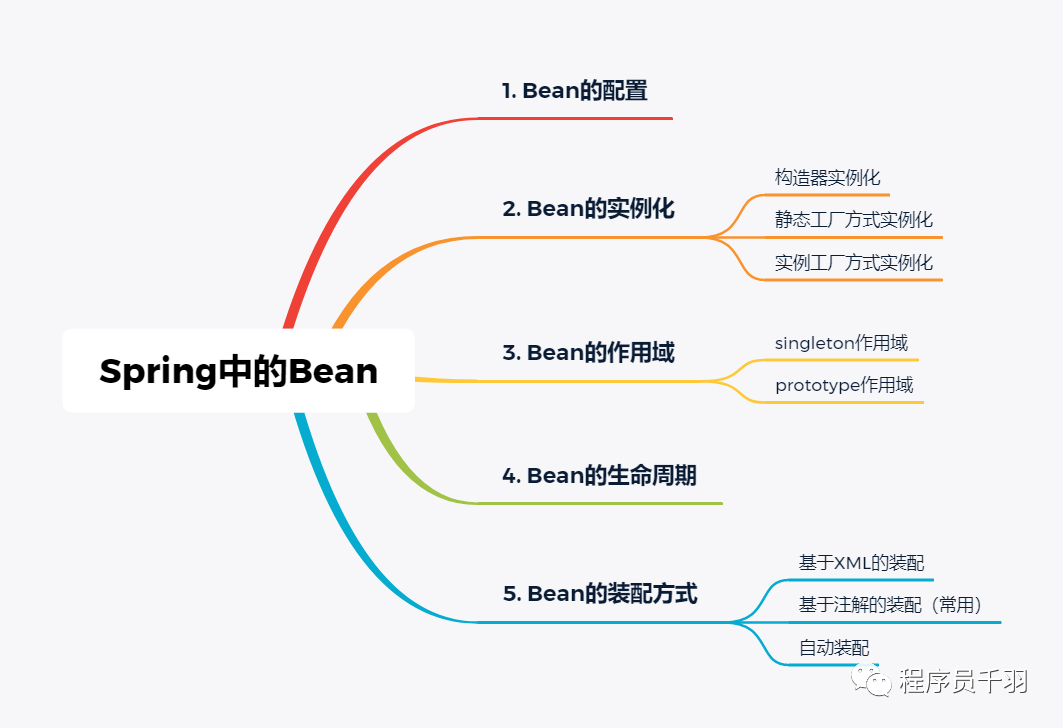
"GitHub: https://github.com/nateshao/ssm/tree/master/102-spring-bean
1. Bean configuration
What is a Bean in Spring?
"If Spring is regarded as a large factory, the beans in the Spring container are the products of the factory. If you want to use this factory to produce and manage beans, you need to tell it in the configuration file which beans are needed and how to assemble these beans together.
Tip: the essence of beans is classes in Java, while beans in Spring are actually references to entity classes to produce Java class objects, so as to realize the production and management of beans.
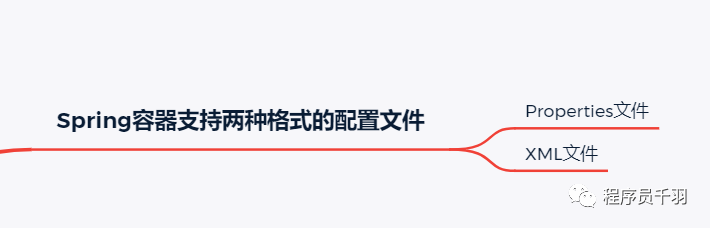
In actual development, the most commonly used configuration method is XML file format. This configuration method registers and manages the dependencies between beans through XML files.
The root element of the XML configuration file is < beans >, which contains multiple < Bean > sub elements. Each < Bean > sub element defines a Bean and describes how the Bean is assembled into the Spring container. The common attributes of < beans > elements are shown in the following table:
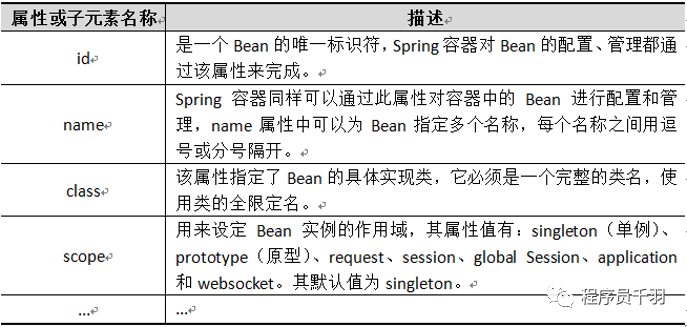
In the configuration file, an ordinary Bean only needs to define id (or name) and class attributes. The way to define a Bean is as follows:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="bean1" class="com.nateshao.Bean1" /> <bean name="bean2" class="com.nateshao.Bean2" /> </beans>
Tip: if id and name are not specified in the Bean, Spring will use the class value as id.
2. Bean instantiation
What are the ways to instantiate beans?
"In object-oriented programs, if you want to use an object, you need to instantiate it first. Similarly, in Spring, you also need to instantiate beans if you want to use beans in containers. There are three ways to instantiate beans: constructor instantiation, static factory instantiation and instance factory instantiation (the most commonly used one is constructor instantiation).
Constructor instantiation
"Constructor instantiation means that the Spring container instantiates a Bean through the default constructor in the class corresponding to the Bean. Next, demonstrate the use of constructor instantiation:
- Create a Web project and import relevant maven packages;
- Create a Java class named Beanl;
- Create Spring configuration file beans 1 XML and configure Beanl entity class Bean;
Bean1.java
public class Bean1 { }
InstanceTest1.java
public class InstanceTest1 { public static void main(String[] args) { String xmlPath = "beans1.xml"; ApplicationContext applicationContext = new ClassPathXmlApplicationContext(xmlPath); Bean1 bean = (Bean1) applicationContext.getBean("bean1"); System.out.println(bean); } }
beans1.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd"> <bean id="bean1" class="com.nateshao.instance.constructor.Bean1" /> </beans>
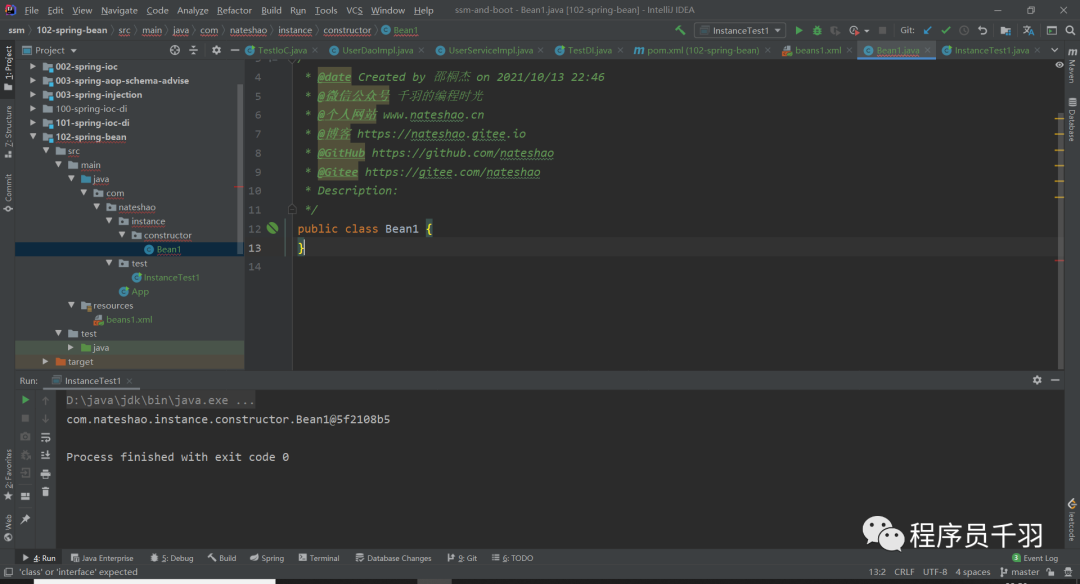
Static factory instantiation
"Static factory is another way to instantiate a Bean. This method requires you to create a static factory method to create an instance of a Bean. Next, demonstrate the use of static factory instantiation:
- Create a Java class named Bean2;
- Create a Java factory class and use static methods to obtain Bean2 instances in the class;
- Create Spring configuration file beans2 XML and configure the factory class Bean;
Bean2.java
public class Bean2 { }
MyBean2Factory.java
package com.nateshao.instance.static_factory; /** * @date Created by Shao Tongjie on 2021/10/13 22:53 * @Programming time of WeChat official account * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class MyBean2Factory { //Create a Bean2 instance using your own factory public static Bean2 createBean(){ return new Bean2(); } }
beans2.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd"> <bean id="bean2" class="com.nateshao.instance.static_factory.MyBean2Factory" factory-method="createBean" /> </beans>
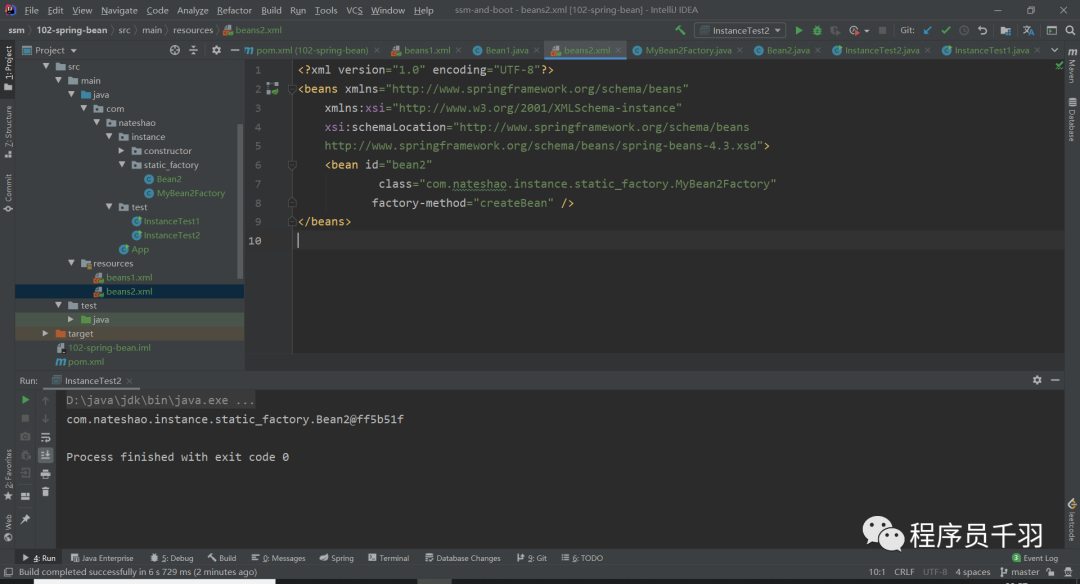
Instance factory instantiation
"The instance factory directly creates Bean instances. In the configuration file, configure an instance factory through the factory Bean property, and then use the factory method property to determine which method in the factory to use. Next, demonstrate the use of instance factory instantiation:
- Create a Java class named Bean3;
- Create a Java factory class and use non static methods to obtain Bean3 instances in the class;
- Create Spring configuration file beans3 XML and configure the factory class Bean;
- Create test classes and test programs.
Bean3.java
public class Bean3 { }
MyBean3Factory.java
package com.nateshao.instance.factory; /** * @date Created by Shao Tongjie on 2021/10/13 23:00 * @Programming time of WeChat official account * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class MyBean3Factory { public MyBean3Factory() { System.out.println("bean3 Factory instantiation"); } //Method for creating Bean3 instance public Bean3 createBean(){ return new Bean3(); } }
beans3.java
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd"> <!-- Configuration factory --> <bean id="myBean3Factory" class="com.nateshao.instance.factory.MyBean3Factory" /> <!-- use factory-bean Property points to the configured instance factory, use factory-method Property determines which method in the factory is used--> <bean id="bean3" factory-bean="myBean3Factory" factory-method="createBean" /> </beans>
InstanceTest3.java
package com.nateshao.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * @date Created by Shao Tongjie on 2021/10/13 23:05 * @Programming time of WeChat official account * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class InstanceTest3 { public static void main(String[] args) { // Define profile path String xmlPath = "beans3.xml"; // ApplicationContext instantiates the Bean when loading the configuration file ApplicationContext applicationContext = new ClassPathXmlApplicationContext(xmlPath); System.out.println(applicationContext.getBean("bean3")); } }
Operation results
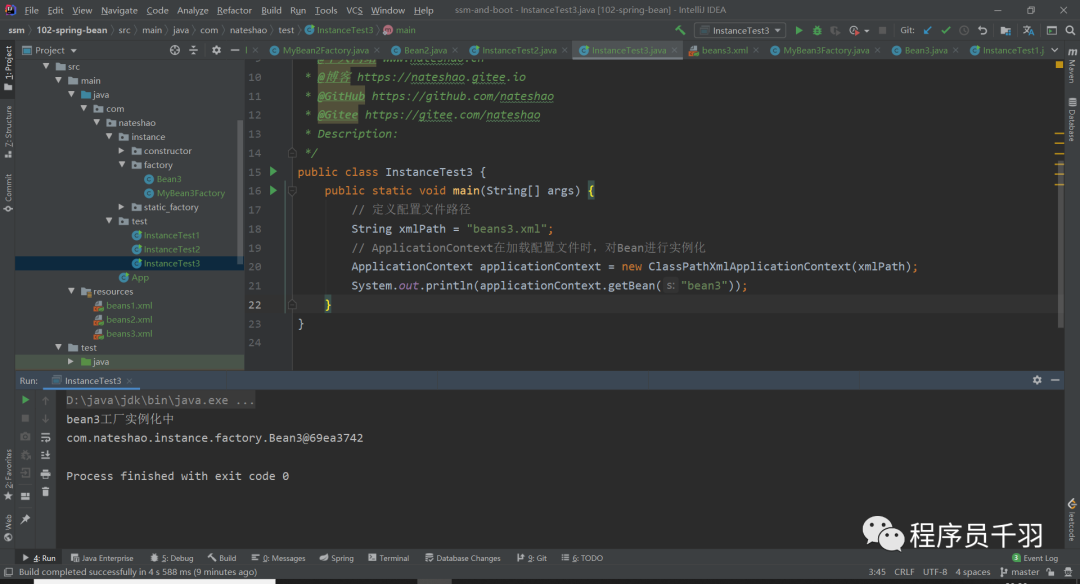
3. Scope of bean
Type of scope
Spring 4.3 defines seven scopes for Bean instances, as shown in the following table:
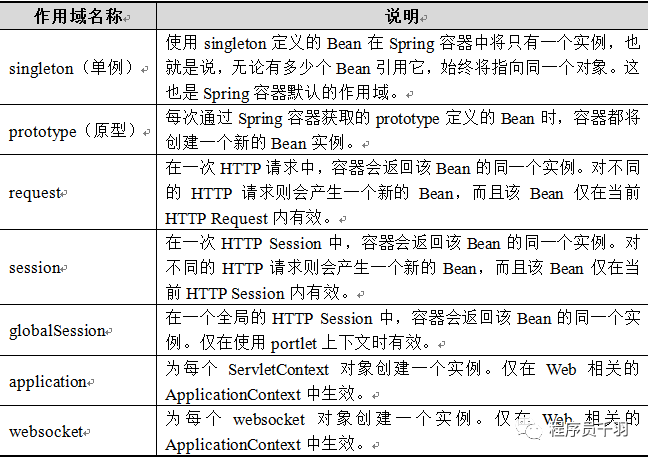
Note: singleton and prototype are the two most commonly used scopes among the seven scopes in the above table.
singleton scope
Singleton is the default scope of the Spring container. When the scope of a Bean is singleton, there will only be one shared Bean instance in the Spring container. Singleton scope is the best choice for beans without session state (such as Dao component and Service component).
In the Spring configuration file, you can use the scope attribute of the < Bean > element to define the scope of a Bean as a singleton.
How to configure singleton scope in Spring?
such as
<bean id="scope" class="com.nateshao.scope.Scope" scope="singleton"/>
prototype scope
The prototype scope should be used for beans that need to maintain session state (such as the Action class of Struts 2). When using the prototype scope, the Spring container will create a new instance for each request to the Bean.
In the Spring configuration file, the scope attribute of the < Bean > element is also used to define the scope of the Bean as prototype.
How to configure the prototype scope in Spring?
such as
<bean id="scope" class="com.nateshao.scope.Scope" scope=" prototype "/>
Code example 4: ScopeTest
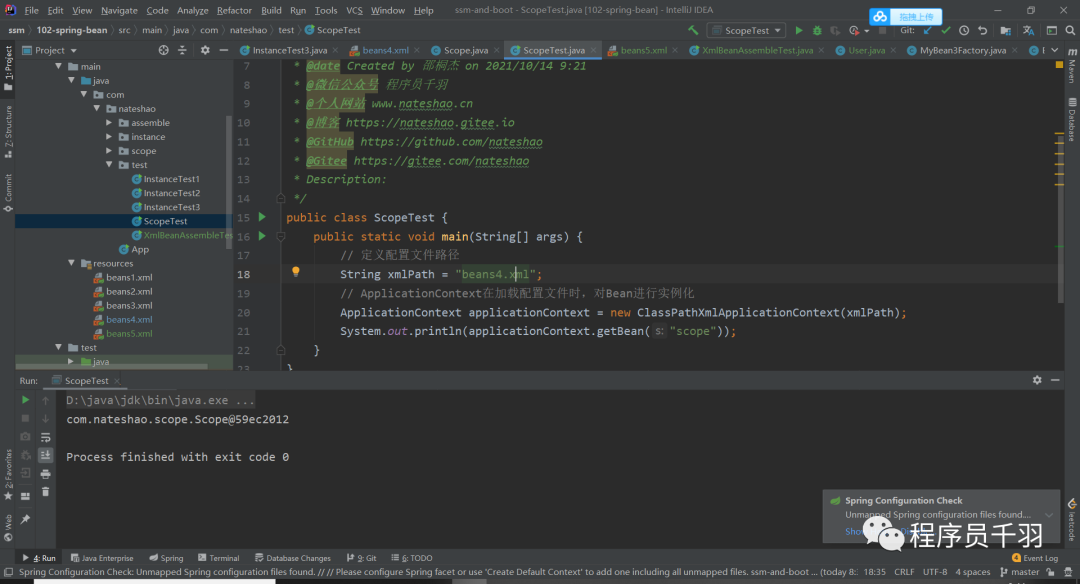
4. Bean life cycle
What's the point of understanding the life cycle of beans in Spring?
"The significance of understanding the life cycle of a Bean in Spring is that you can use a Bean to complete some related operations at a specific time during its lifetime. There may be many such times, but generally, some related operations are often performed in the Bean's position (after initialization) and predestruction (before destruction).
The Spring container can manage the life cycle of some scopes of beans. Relevant instructions are as follows:
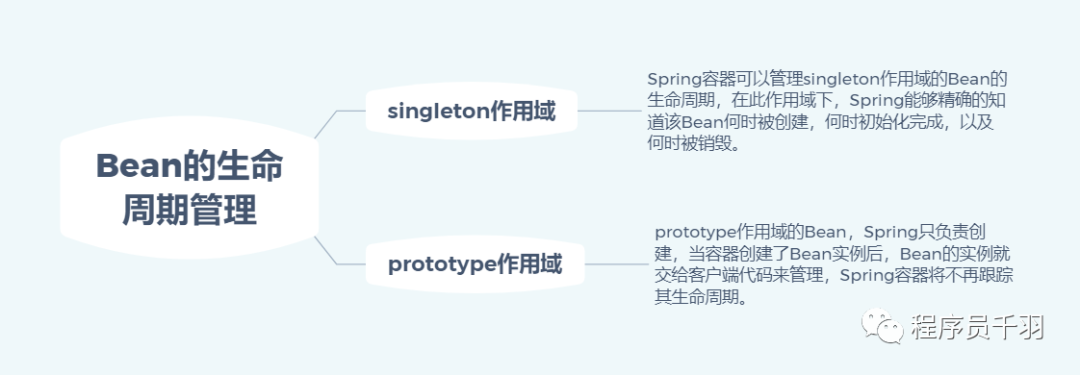
The life cycle process of beans in Spring container is shown in the following figure;

5. Bean assembly method
What is Bean assembly?
"Bean assembly can be understood as dependency injection. Bean assembly means bean dependency injection. The Spring container supports various forms of bean assembly, such as XML based assembly, Annotation based assembly and automatic assembly (Annotation based assembly is the most commonly used). This section will mainly explain the use of these three assembly methods.
XML based assembly
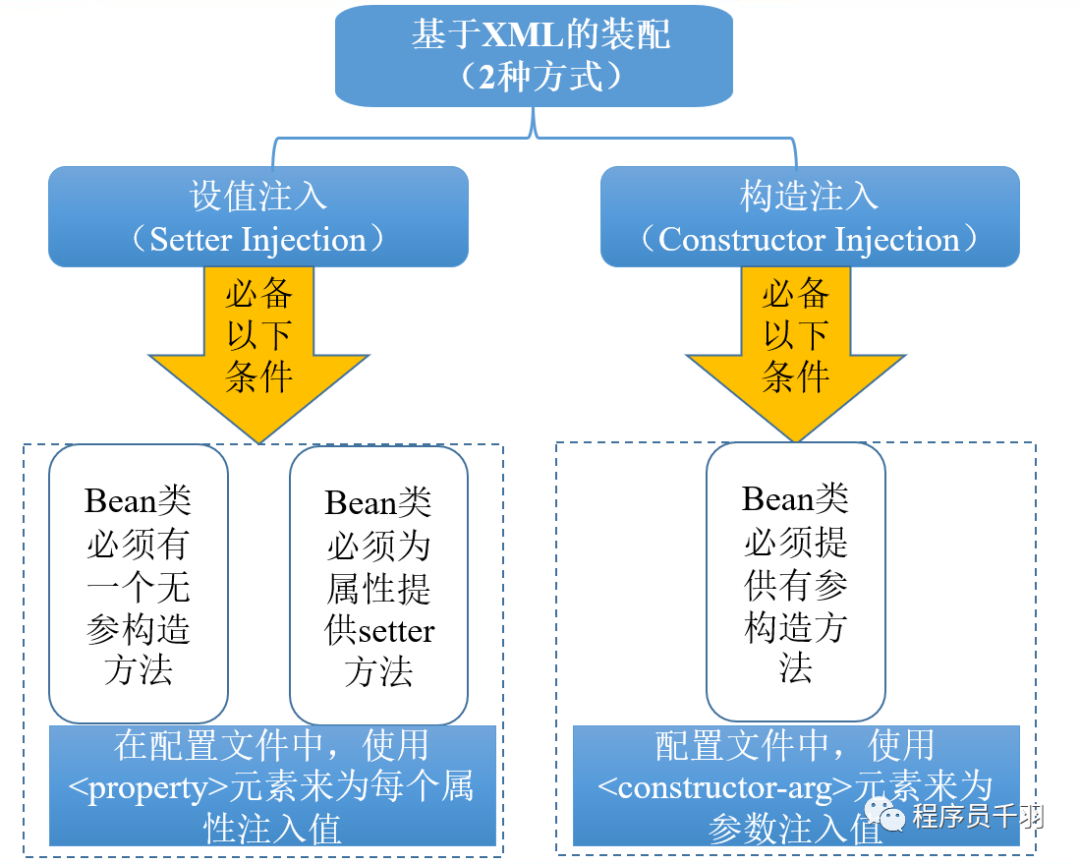
XML based assembly is used as follows:
- Create Java classes, provide parameterized and nonparametric constructs and property setter methods;
- Create Spring configuration file beans5 XML, two methods are used to configure beans
- Create test classes and test programs.
User.java
package com.nateshao.assemble; import java.util.List; /** * @date Created by Shao Tongjie on 2021/10/14 9:24 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class User { private String username; private int password; private List<String> list; /** * 1.Using construct injection * 1.1 Provides a parametric construction method with all parameters. */ public User(String username, Integer password, List<String> list) { super(); this.username = username; this.password = password; this.list = list; } /** * 2.Use set point injection * 2.1 Provide default null parameter construction method; * 2.2 Provide setter methods for all properties. */ public User() { super(); } public void setUsername(String username) { this.username = username; } public void setPassword(Integer password) { this.password = password; } public void setList(List<String> list) { this.list = list; } @Override public String toString() { return "User [username=" + username + ", password=" + password + ", list=" + list + "]"; } }
beans5.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd"> <!--1.Assembly using construction injection User example --> <bean id="user1" class="com.nateshao.assemble.User"> <constructor-arg index="0" value="tom" /> <constructor-arg index="1" value="123456" /> <constructor-arg index="2"> <list> <value>"constructorvalue1"</value> <value>"constructorvalue2"</value> </list> </constructor-arg> </bean> <!--2.Assembly using set point injection User example --> <bean id="user2" class="com.nateshao.assemble.User"> <property name="username" value="Zhang San"></property> <property name="password" value="654321"></property> <!-- injection list aggregate --> <property name="list"> <list> <value>"setlistvalue1"</value> <value>"setlistvalue2"</value> </list> </property> </bean> </beans>
XmlBeanAssembleTest.java
package com.nateshao.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * @date Created by Shao Tongjie on 2021/10/14 9:27 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class XmlBeanAssembleTest { public static void main(String[] args) { // Define profile path String xmlPath = "beans5.xml"; // ApplicationContext instantiates the Bean when loading the configuration file ApplicationContext applicationContext = new ClassPathXmlApplicationContext(xmlPath); System.out.println(applicationContext.getBean("user1")); System.out.println(applicationContext.getBean("user2")); } }
Operation results:
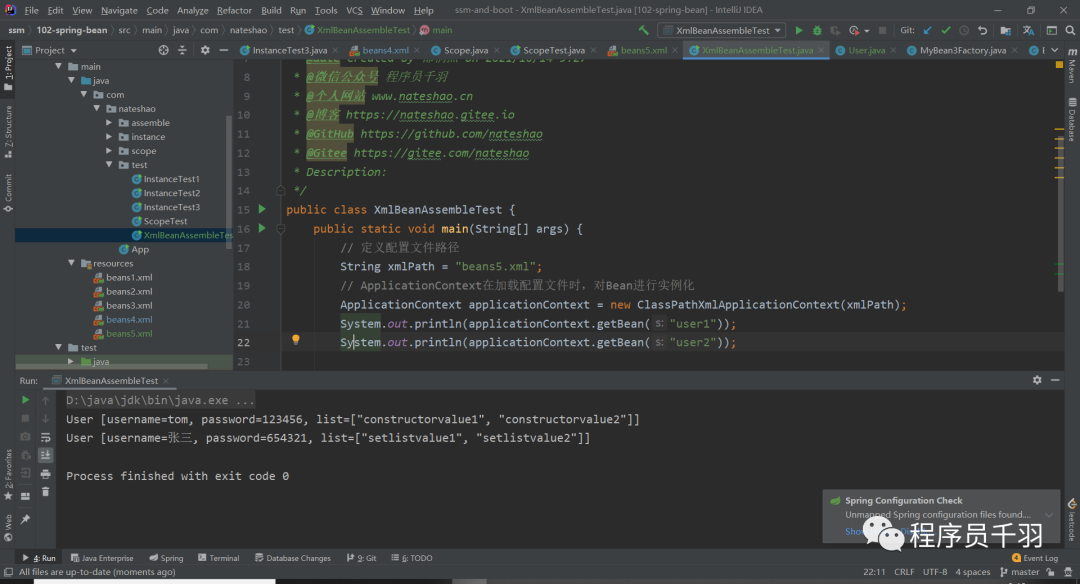
Annotation based assembly
"XML based assembly may cause the XML configuration file to be too bloated, which will bring some difficulties to subsequent maintenance and upgrading. Therefore, Spring provides comprehensive support for Annotation technology.
Main notes
- @Component: used to describe Bean in Spring. It is a generalized concept and only represents a component.
- @Repository: used to identify the class of the data access layer (DAO) as a Bean in Spring.
- @Service: used to identify the class of the business layer (service) as a Bean in Spring.
- @Controller: used to identify the class of the control layer (controller) as a Bean in Spring.
- @Autowired: used to label the Bean's attribute variables, attribute setter methods and construction methods, and cooperate with the corresponding annotation processor to complete the automatic configuration of the Bean.
- @Resource: its function is the same as Autowired@ There are two important attributes in a resource: name and type. Spring resolves the name attribute to the Bean instance name and the type attribute to the Bean instance type.
- @Qualifier: when used in conjunction with the @ Autowired annotation, the default assembly by Bean type will be modified to assembly by Bean instance name, which is specified by the parameters of the @ qualifier annotation.
Annotation based assembly is used as follows:
- Create the interface UserDao and define the method;
- Create the interface implementation class UserDaoImpl and declare the class with @ Repository;
- Create the UserService interface and define the method;
- Create the interface implementation class UserServiceImpl and declare the class with @ Service,
- Inject UserDao attribute with @ Resource;
- Create a Controller class, declare it with @ Controller, and use @ Resource
- Inject UserService attribute;
- Create Spring configuration file and configure Bean;
- Create test classes and test programs.
UserDao.java
public interface UserDao { public void save(); }
UserDaoImpl.java
package com.nateshao.annotation; import org.springframework.stereotype.Repository; /** * @date Created by Shao Tongjie on 2021/10/14 10:12 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ @Repository public class UserDaoImpl implements UserDao{ @Override public void save() { System.out.println("userdao...save..."); } }
UserService.java
public interface UserService { public void save(); }
UserServiceImpl.java
package com.nateshao.annotation; import org.springframework.stereotype.Service; import javax.annotation.Resource; /** * @date Created by Shao Tongjie on 2021/10/14 10:14 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ @Service("userService") public class UserServiceImpl implements UserService{ @Resource(name="userDao") private UserDao userDao; @Override public void save() { //Call the save method in userDao this.userDao.save(); System.out.println("userservice....save..."); } public void setUserDao(UserDao userDao) { this.userDao = userDao; } }
UserController.java
package com.nateshao.annotation; import org.springframework.stereotype.Controller; import javax.annotation.Resource; /** * @date Created by Shao Tongjie on 2021/10/14 10:17 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ @Controller("userController") public class UserController { @Resource(name="userService") private UserService userService; public void save(){ this.userService.save(); System.out.println("userController...save..."); } public void setUserService(UserService userService) { this.userService = userService; } }
beans.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd"> <!-- use context Namespace ,Turn on the corresponding annotation processor in the configuration file --> <context:annotation-config /> <!--Three are defined respectively Bean example --> <bean id="userDao" class="com.nateshao.annotation.UserDaoImpl" /> <bean id="userService" class="com.nateshao.annotation.UserServiceImpl" /> <bean id="userController" class="com.nateshao.annotation.UserController" /> </beans>
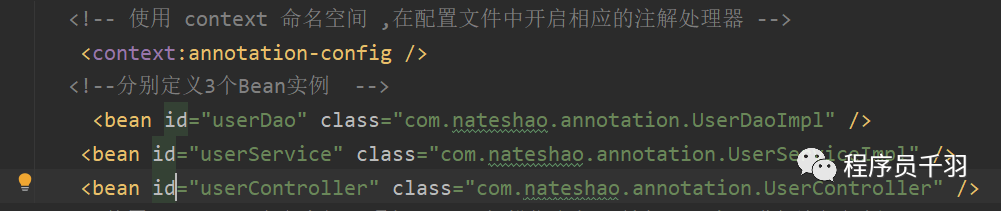
AnnotationAssembleTest.java
package com.nateshao.test; import com.nateshao.annotation.UserController; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * @date Created by Shao Tongjie on 2021/10/14 10:20 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class AnnotationAssembleTest { public static void main(String[] args) { // Define profile path String xmlPath = "beans6.xml"; // Load profile ApplicationContext applicationContext = new ClassPathXmlApplicationContext(xmlPath); // Get UserController instance UserController userController = (UserController) applicationContext.getBean("userController"); // Call the save() method in UserController userController.save(); } }
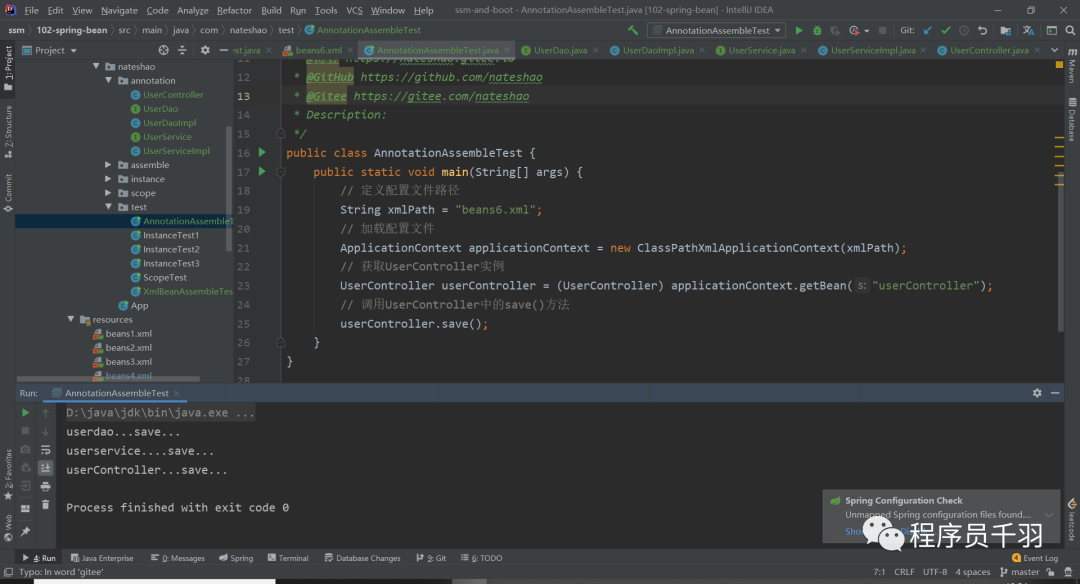
Tip: in addition to configuring beans through the < Bean > element in the example, you can also configure all beans under a package in the form of package scanning:
<context:component-scan base-package="com.nateshao.annotation" />
automatic assembly
"The so-called automatic assembly means that a Bean is automatically injected into the properties of other beans. The element of Spring contains an autowire Property. We can automatically assemble beans by setting the Property value of autowire. The autowire Property has five values, and its values and descriptions are shown in the following table:

Automatic assembly, using the following methods:
- Modify UserServiceImple and UserController in the previous section and add setter methods of class properties respectively;
- Modify the Spring configuration file and use the autowire attribute to configure the Bean;
- Retest the program.
<bean id="userDao" class="com.nateshao.annotation.UserDaoImpl" /> <bean id="userService" class="com.nateshao.annotation.UserServiceImpl" autowire="byName" /> <bean id="userController" class="com.nateshao.annotation.UserController" autowire="byName" />
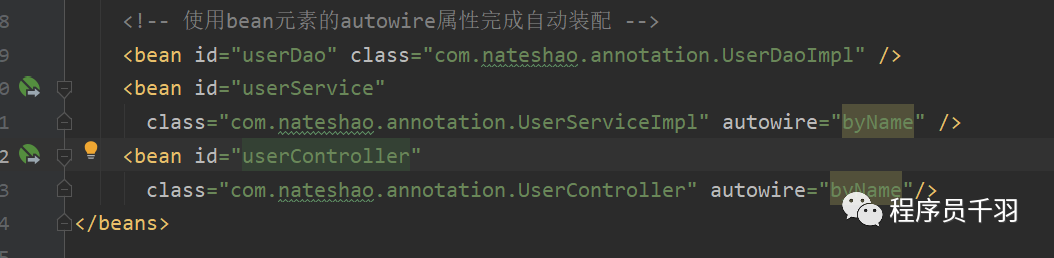