August 24, 2018 17:20:30
Abstract factory pattern
definition
Lend me a morning pencil and return it to you tomorrow.
Now I can return your morning pencil. You are no longer here. Tomorrow is still tomorrow.
Abstract Factory pattern provides an interface to create a series of related or interdependent objects without specifying their specific classes—— Design patterns: the foundation of Reusable Object-Oriented Software
Usage scenario
First, we should understand two new concepts: product family and product hierarchy.
Product family refers to the family composed of functionally related products in different product hierarchy.
Product hierarchy, that is, the inheritance structure of products. The specific products that inherit (or realize) the same abstract product belong to the same product hierarchy.
In the following code examples, Chenguang stationery and true color stationery are two product families. Chenguang pencil and true color pencil that inherit (or implement) pencil Abstract products are one product hierarchy, and Chenguang pencil and true color pencil that inherit (or implement) rubber Abstract products are another product hierarchy. [I used inheritance (or implementation) because there are interfaces in Java that are implemented, and abstract classes are inherited. Abstract products can be either interfaces or abstract classes. In order not to mislead myself, I specially added it.] examples include: Intel's motherboard, chipset and CPU are a product family, and AMD's motherboard, chipset and CPU are also a product family, There are three product level structures: motherboard, chipset and CPU.
When we think about code examples and things we often use, we think of stationery, morning light and true color. After checking, I didn't expect that the turnover of small stationery exceeded one billion.
Now our goal is to earn him 100 million first and open a blog Park Stationery (product family). They all have the product hierarchy. We can directly inherit (or implement) their abstract product classes. It's more convenient. This is the benefit of the abstract factory pattern. Add a product family without destroying the original code structure, which conforms to the opening and closing principle.
However, only the old products are not competitive. Otherwise, we will add a new product, that is, a new product grade structure. For the time being, it is a special smart pen for code farmer cheating to help you easily cope with the written test. At this time, we will add new members to the stationery (product family) in the blog Park, and we need to add relevant codes for creating new products in the specific factory class of stationery in the blog park, It does not comply with the opening and closing principle. This is the disadvantage of the abstract factory pattern.
To sum up, in combination with other situations, the abstract factory mode can be used in the following cases:
1. A system should not rely on the details of how product instances are created, combined and expressed, which is important for all types of factory patterns.
2. The system has more than one product, and only one product family is used at a time.
3. Products belonging to the same product family will be used together, and this constraint should be reflected in the system design.
4. All products appear as their own inherited (or implemented) interfaces, so that the client does not depend on the specific implementation.
Point 1, no doubt. Points 2 and 3 say that I choose Chenguang stationery. What I use is Chenguang pencil. I need to use Chenguang eraser, which should be used together. I can't use Chenguang pencil but use real color eraser. This constraint seems a little far fetched in this example, but think about this scenario: the final exam needs the same stationery. There are two options, one is Chenguang, the other is true color. They are matched. Chenguang's pencil is matched with Chenguang's rubber, and the true color pencil is matched with the true color rubber, so it's comfortable. Point 4: interface oriented programming to reduce coupling.
In points 2 and 3, it is more appropriate to use the Intel and AMD product families mentioned above. Intel's CPU cannot match AMD's motherboard, and AMD's CPU cannot match Intel's motherboard. Therefore, when assembling the computer, there is this constraint by default: only products in the same product family can be used.
The origin of the abstract factory pattern is used to create Windows constructs belonging to different operating systems. For example: command Button and Text box (Text) are both Windows builds. In the Windows environment of UNIX operating system and Windows operating system, the two builds have different local implementations, and their details are different. When building Windows, you can only select the Button and Text of one operating system. If not, neither operating system can be used. What do I want this window to do?
role
Abstract Factory: the common interface of concrete factory (product family)
Concrete Factory: product family
Abstract Product: product hierarchy
Concrete Product role:
Illustration
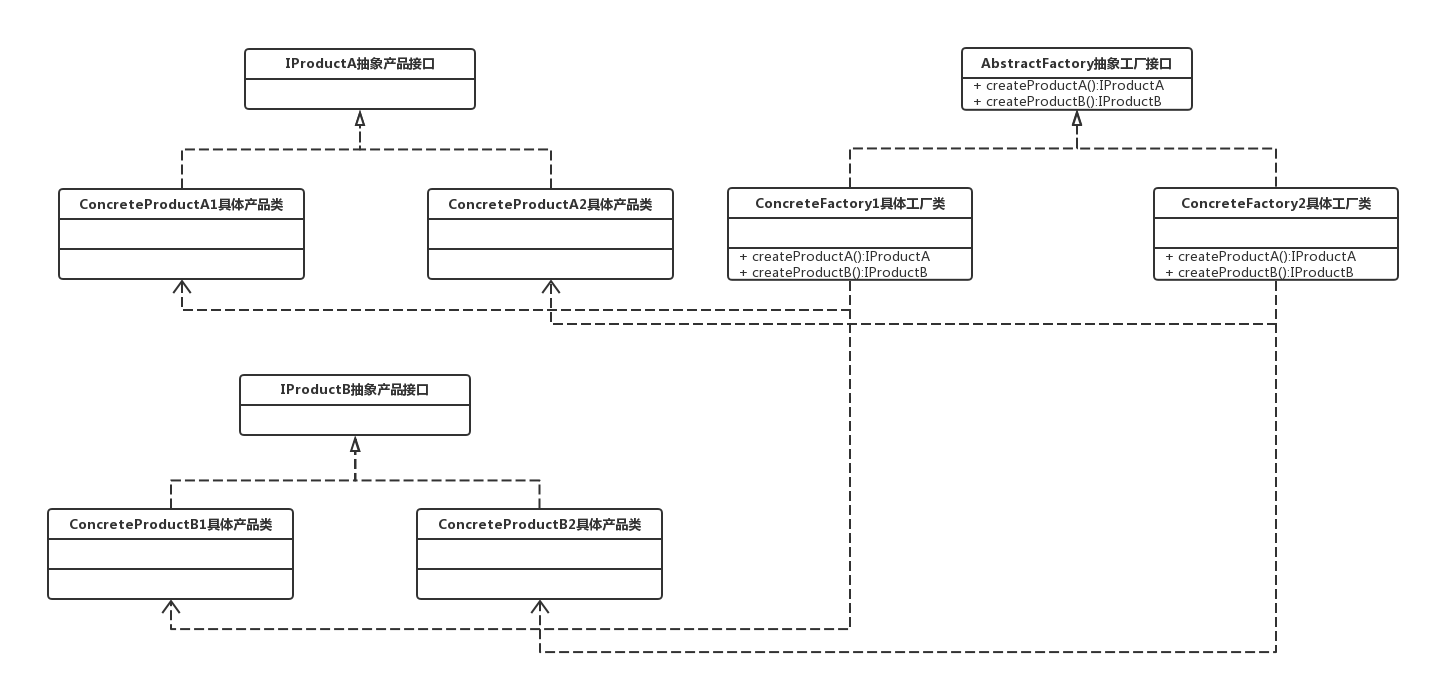
Code example
Code example class dependency diagram:
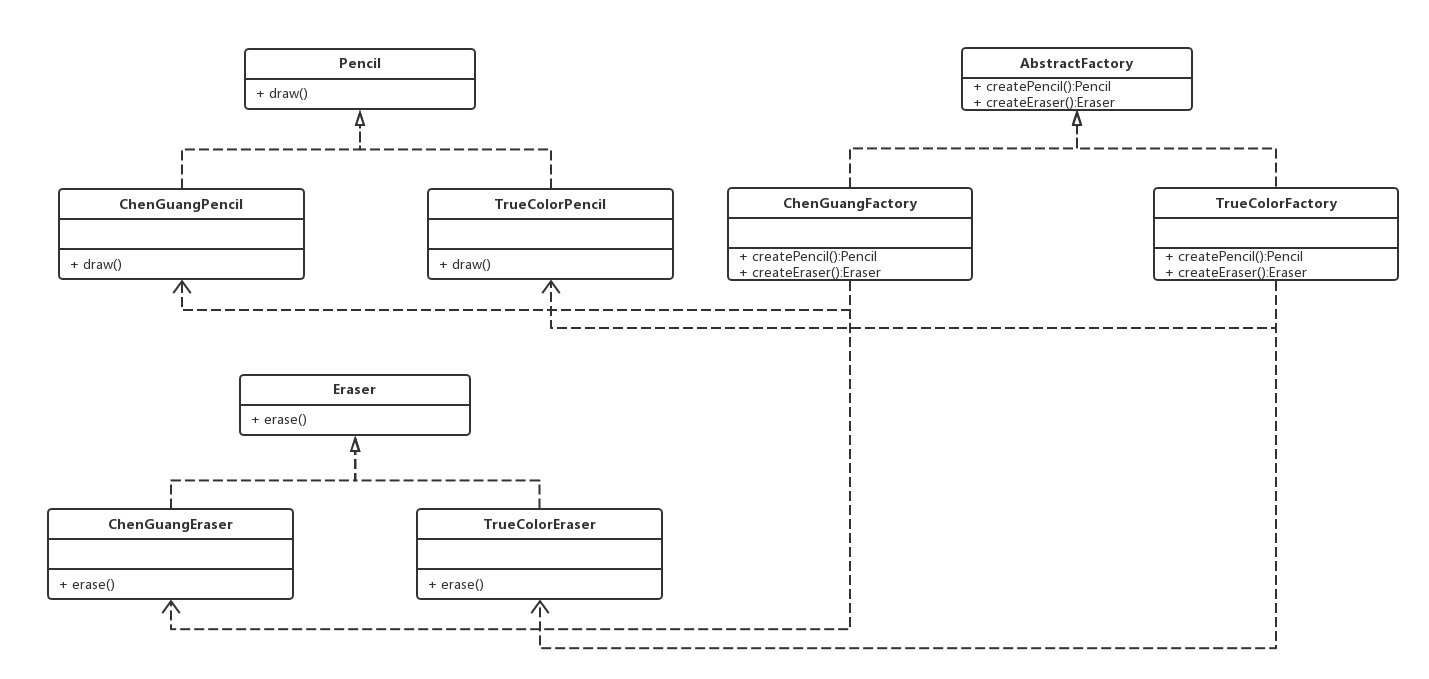
Abstract factory role (AbstractFactory.java)
public interface AbstractFactory { public Pencil createPencil(); public Eraser createEraser(); }
Specific factory roles (ChenGuangFactory.java, TrueColorFactory.java)
// Chenguang factory public class ChenGuangFactory implements AbstractFactory { @Override public Pencil createPencil() { return new ChenGuangPencil(); } @Override public Eraser createEraser() { return new ChenGuangEraser(); } } // True color factory public class TrueColorFactory implements AbstractFactory{ @Override public Pencil createPencil() { return new TrueColorPencil(); } @Override public Eraser createEraser() { return new TrueColorEraser(); } }
Abstract product roles (Pencil.java, Eraser.java)
// pencil public interface Pencil { public void draw(); } // rubber public interface Eraser { public void erase(); }
Specific product roles (ChenGuangPencil.java, truecolorencil.java, ChenGuangEraser.java, TrueColorEraser.java)
// Morning pencil public class ChenGuangPencil implements Pencil { @Override public void draw() { System.out.println("Draw with a morning pencil."); } } // True color pencil public class TrueColorPencil implements Pencil { @Override public void draw() { System.out.println("Draw with a true color pencil."); } } // Morning light rubber public class ChenGuangEraser implements Eraser { @Override public void erase() { System.out.println("Use the morning eraser to erase the drawing drawn by the morning pencil."); } } // True color rubber public class TrueColorEraser implements Eraser { @Override public void erase() { System.out.println("Use a true color eraser to erase the picture drawn by a true color pencil."); } }
Test class (AbstractFactoryTest.java)
public class AbstractFactoryTest { public static void main(String[] args) { // 1. Morning light AbstractFactory factory = new ChenGuangFactory(); Pencil pencil = factory.createPencil(); pencil.draw(); // Draw with a morning pencil. Eraser eraser = factory.createEraser(); eraser.erase(); // Use the morning eraser to erase the drawing drawn by the morning pencil. // 2. True color AbstractFactory factoryT = new TrueColorFactory(); Pencil pencilT = factoryT.createPencil(); pencilT.draw(); // Draw with a true color pencil. Eraser eraserT = factoryT.createEraser(); eraserT.erase(); // Use a true color eraser to erase the picture drawn by a true color pencil. } }
Test results:

advantage
1. It has the same advantages as simple factory mode and factory method mode: the client does not need to know the details of object creation
2. When multiple objects in a product family are designed to work together, it ensures that clients always use only products in the same product family.
3. Add product family to comply with the opening and closing principle.
shortcoming
1. When adding product grade structure, it does not comply with the opening and closing principle.
summary
Abstract factory pattern adds the concepts of product family and product hierarchy on the basis of factory method pattern, and only uses the constraints in the same product family, which makes the abstract factory pattern conform to some scenes in real life and become a practical design pattern. In addition, the abstract factory pattern realizes high cohesion and low coupling, which also provides a basis for its wide application.
finish
(should we charge some advertising fees for Chenguang and real color???)
reference resources:
Abstract factory pattern in JAVA and pattern
August 25, 2018 18:31:58