1. Demand analysis
Using C + + to realize address book management system
The following functions need to be realized in the system:
Add contact: add a new contact to the address book. The information includes (name, gender, age, contact number, home address, and records up to 1000 people.
Show contacts: displays all contact information in the address book
Delete contact: delete the specified contact by name
Find contact: find the specified contact by name
Modify contact: modify the specified contact according to the name
Empty contact: clear all information in the address book
Exit address book: exit the currently used address book
2. Create project
The steps to create a project are as follows:
· create a new project
· add files
2.1 create a new project
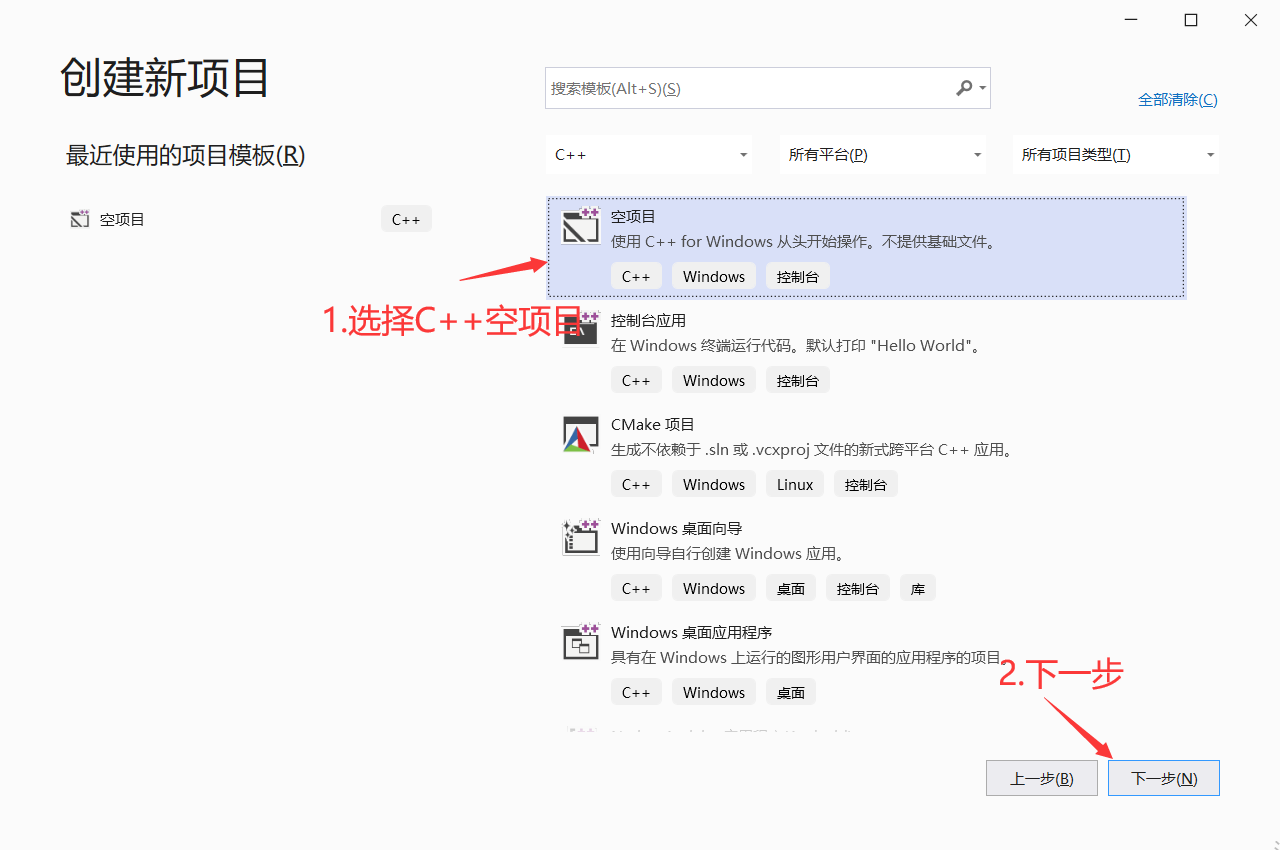
2.2 adding files
3. Menu function
Function Description: the interface for users to select functions
The menu effect diagram is as follows:
Steps:
·The encapsulated function void showmenu() displays this interface
Calling display function in main function
Reference code:
void showMenu() { cout << "*****************************" << endl; cout << "***** 1.Add a Contact *****" << endl; cout << "***** 2.Show contacts *****" <<endl; cout << "***** 3.Delete Contact *****" << endl; cout << "***** 4.find contact *****" << endl; cout << "***** 5.Modify contact *****" << endl; cout << "***** 6.Empty contacts *****" << endl; cout << "***** 0.Exit address book *****" << endl; cout << "*****************************" << endl; } int main() { //Menu call showMenu(); system("pause"); return 0; }
4. Exit function
Function Description: exit the address book system
Idea: enter different functions according to different choices of users, and build the framework through switch branch structure
Reference code:
int main() { //Create user selected variables int select = 0; while (true) { //Menu call showMenu(); cin >> select; switch (select) { case 1://Add a Contact break; case 2://Show contacts break; case 3://Delete Contact break; case 4://find contact break; case 5://Modify contact break; case 6://Empty contacts break; case 0://Exit address book cout << "Welcome to use again!" << endl; system("pause"); return 0; break; default: break; } } system("pause"); return 0; }
5. Add contact
Function Description: realize the function of adding contacts, and the upper limit of contacts is 1000; Contact information includes (name, gender, age, contact number, home address)
Steps:
·Design contact structure
·Design address book structure
·Create address book in main function
·Encapsulate add contact function
·Test add contact function
5.1 design contact structure
Contact information includes: name, gender, age, contact number, home address
Reference code:
struct person { string m_Name; //full name int m_Sex; //Gender: 1 male, 2 female int m_Age;//Age string m_Phone; //Telephone string m_Addr; //Home address };
5.2 design address book structure
The maximum number is 1000, and the number of existing contacts is recorded
Reference code:
#define MAX 1000 / / maximum number of people //Address book structure struct addressbooks { struct person personArray[MAX]; //An array of contacts saved in the address book int m_size ; //Number of people already in the address book };
5.3 create address book in main function
After the add contact function is encapsulated, create an address book variable in the main function, which is the address book that needs to be maintained all the time.
Reference code:
//Create address book structure variable Addressbooks abs; //Initialize the number of people in the address book abs.m_size = 0;
5.4 encapsulating and adding contact functions
Idea: first, check whether the address book is full. If it is full, you cannot continue to add it. If it is not full, enter the contact data one by one
Reference code:
//Add a Contact void addPerson(Addressbooks * abs) { //Judge whether the address book is full. If it is full, it will not be added if (abs->m_size == MAX) { cout << "The address book is full and cannot be added" << endl; return; } else //Add specific contacts { //full name cout << "Please enter your name" << endl; string name; cin >> name; abs->personArray[abs->m_size].m_Name = name; //Gender cout << "Please enter gender:" << endl; cout << "1 -- male" << endl; cout << "2 -- female" << endl; int sex = 0; while (true) { //If you enter 1 or 2, you can exit the cycle. If the input is wrong, you can re-enter it cin >> sex; if (sex == 1 || sex == 2) { abs->personArray[abs->m_size].m_Sex; break; } cout << "Input error, please re-enter" << endl; } //Age cout << "Please enter age" << endl; int age = 0; cin >> age; abs->personArray[abs->m_size].m_Age = age; //contact number cout << "Please enter the contact number" << endl; string phone; cin >> phone; abs->personArray[abs->m_size].m_Phone = phone; //Home address cout << "Please enter your home address" << endl; string address; cin >> address; abs->personArray[abs->m_size].m_Addr = address; //Number of contacts updated abs->m_size++; cout << "Added successfully" << endl; system("pause"); //Please press any key to continue system("cls");//Clear screen } }
5.5 test add contact function
Select 1 in the selection interface to add a contact
Add in switch statement
case 1://Add a Contact addPerson(&abs); break;
design sketch
6. Show contacts
Function Description: displays the existing contact information in the address book
Steps:
· encapsulate and display contact function
· test and display contact function
6.1 encapsulated display contact function
Idea: when there is no one in the current address book, it will prompt that the address book is empty and the number of people is greater than 0 to display the information in the address book
Reference code:
//Show contact function void showPerson(Addressbooks * abs) { //Judge whether the address book is empty. If it is empty, it will prompt that the address book is empty //If it is not empty, the circular output information if (abs->m_size == 0) { cout << "Current address book is empty" << endl; } else { for (int i = 0; i < abs->m_size; i++) { cout << "full name:" << abs->personArray[i].m_Name << "\t"; cout << "Gender:" << (abs->personArray[i].m_Sex == 1?"male":"female") << "\t"; cout << "Age:" << abs->personArray[i].m_Age << "\t"; cout << "contact number:" << abs->personArray[i].m_Phone << "\t"; cout << "Home address:" << abs->personArray[i].m_Addr << endl; } } system("pause"); //press any key to continue system("cls");//Clear screen }
6.2 test and display contact function
In the switch case statement, add the following to case2:
case 2://Show contacts showPerson(&abs); break;
design sketch
7. Delete Contact
Function Description: delete the specified contact by name
Steps:
·Encapsulates a function that detects whether a contact exists
·Encapsulate delete contact function
·Test delete contact function
7.1 whether the package inspection contact exists
Design idea: before deleting a contact, judge whether the contact entered by the user exists. If it exists, return to the location and delete it. If it does not exist, return - 1
Reference code:
//Check whether the contact exists. If it exists, return the specific subscript position. If it does not exist, return - 1 int isExist(Addressbooks * abs,string name) { for (int i = 0; i < abs->m_size; i++) { //If found, return the subscript i if (abs->personArray[i].m_Name == name) { return i; } } return -1; }
7.2 encapsulating and deleting contact function
Design idea: according to the search function, if it is found, it will be deleted; if it is not found, it will prompt that there is no such person
Reference code:
//Delete specified contact void deletePerson(Addressbooks* abs) { cout << "Please enter the name of the contact you want to delete" << endl; string name; cin >> name; //ret == -1, not found; ret != -1. I found it int ret=isExist(abs, name); if (ret != -1) { //Found, delete for (int i = ret; i < abs->m_size; i++) { //Data forward abs->personArray[i] = abs->personArray[i + 1]; } abs->m_size--;//Number of contacts updated cout << "Delete succeeded!" << endl; } else { cout << "No one was found" << endl; } system("pause");//press any key to continue system("cls");//Clear screen }
7.3 test the function of deleting contacts
In the switch case statement, case3 adds:
case 3://Delete Contact deletePerson(&abs); break;
design sketch
8. Find contacts
Function Description: view the specified contact information by name
Steps:
·Encapsulate find contact function
·Test function function
8.1 encapsulating the contact lookup function
Idea: judge whether the contact specified by the user exists, whether there is display information, and whether there is no prompt. Check whether there is no such person.
Reference code:
//find contact void findPerson(Addressbooks * abs) { cout << "Please enter the contact you want to find" << endl; string name; cin >> name; int ret = isExist(abs, name); if (ret != -1) { cout << "full name:" << abs->personArray[ret].m_Name << "\t"; cout << "Gender:" << (abs->personArray[ret].m_Sex == 1?"male":"female") << "\t"; cout << "Age:" << abs->personArray[ret].m_Age << "\t"; cout << "contact number:" << abs->personArray[ret].m_Phone << "\t"; cout << "Home address:" << abs->personArray[ret].m_Addr << endl; } else { cout << "No one was found" << endl; } system("pause"); system("cls"); }
8.2 test the function of finding contacts
In the switch case statement, add the following to case4:
case 4://find contact findPerson(&abs); break;
design sketch
9. Modify contact
Function Description: re modify the specified contact according to the name
Steps:
·Encapsulating and modifying contact functions
·Test function function
9.1 encapsulating and modifying contact functions
Idea: find the contact entered by the user. If the search is successful, modify it, and if the search fails, you will be prompted that there is no such person
Reference code:
//Modify contact void modifyPerson(Addressbooks* abs) { cout << "Please enter the contact name you want to modify" << endl; string name; cin >> name; int ret = isExist(abs, name); if (ret != -1) { //Modify name cout << "Please enter your name" << endl; string name; cin >> name; abs->personArray[ret].m_Name = name; //Modify gender cout << "Please enter gender\n1 -- male\n2 -- female" << endl; int sex; cin >> sex; while (true) { if (sex == 1 || sex == 2) { abs->personArray[ret].m_Sex = sex; break; } else { cout << "Please re-enter" << endl; } } //Modify age int age = 0; cout << "Please enter age" << endl; cin >> age; abs->personArray[ret].m_Age = age; //Modify contact number cout << "Please enter the contact number" << endl; string phone; cin >> phone; abs->personArray[ret].m_Phone = phone; //Modify home address cout << "Please enter your home address" << endl; string address; cin >> address; abs->personArray[ret].m_Addr = address; cout << "Modified successfully" << endl; } else { cout << "No one was found" << endl; } system("pause"); system("cls"); }
9.2 test and modify contact function
In the switch case statement, add the following to case5:
case 5://Modify contact modifyPerson(&abs); break;
design sketch
10. Empty contacts
Function Description: clear all information in the address book
Steps:
·Encapsulate empty contact function
·Test the function of clearing contacts
10.1 encapsulate empty contact function
Idea: just set the number of contacts to 0
Reference code:
//Empty contacts void cleanPerson(Addressbooks* abs) { cout << "Are you sure to clear the address book?\n1.determine\n2.cancel" << endl; int flag = 0; while (true) { cin >> flag; if (flag == 1) { abs->m_size = 0; cout << "Contacts to empty" << endl; break; } else if (flag == 2) { cout << "Cancelled, address book not empty" << endl; break; } else { cout << "Input error, please re-enter" << endl; } } system("pause");//press any key to continue system("cls");//Clear screen }
10.2 test the function of clearing contacts
In the switch case statement, add the following to case6:
case 6://Empty contacts cleanPerson(&abs); break;
design sketch