Hello, I'm Xiaoxuan
A few days ago, someone asked me about java inheritance backstage
The question is, do C inherit A and B inherit A and C inherit B?
catalogue
- What is inheritance?
- Inherited syntax?
- Inheritance type?
- Inherited features?
- Inherit keywords?
- Inheritance considerations?
What is inheritance?
Inheritance is one of the most remarkable features of object-oriented. Inheritance is to derive a new class from an existing class. The new class can absorb the data properties and behaviors of the existing class and expand new capabilities.
Inherited syntax?
class Subclass extends Parent class {}
Subclasses are also called derived classes; The parent class is also called super class;
Inheritance type?
Single inheritance, multiple inheritance, different classes inherit the same class;
Note that java inheritance does not have much inheritance.
Inherited features?
- Subclasses have non private properties and methods of the parent class
- Subclasses can extend the parent class;
- Subclasses can implement the methods of the parent class in their own way;
Inherit keywords?
- extends keyword
In Java, class inheritance is a single inheritance, that is, a subclass can only have one parent class, so extensions can only inherit one class.
- implements keyword
Using the implements keyword can make java have the feature of multi inheritance in a disguised way. If the scope of use is class inheritance interface, you can inherit multiple interfaces at the same time (the interface is separated by commas).
public interface A { public void eat(); public void sleep(); } public interface B { public void show(); } public class C implements A,B { }
Inheritance considerations?
Code error:
class A {} class B {} class C extends A,B {}
Correct writing:
class A {} class B extends A {} class C extends B {}
In the inheritance relationship, if you want to instantiate a subclass object, you will call the parent class construction first by default to initialize the attributes in the parent class, and then call the subclass construction to initialize the attributes in the subclass, that is, by default, the subclass will find the parameterless construction method in the parent class.
About constructor supplement:
If there is no parameter constructor defined, there is no parameter constructor by default; On the basis of parametric constructors, both constructors can only have parametric constructors if they are defined.
package com.edu.java; class Animal { private String name; public Animal(String myName) { name = myName; } public Animal(){ System.out.println("I'm an animal"); } public void doing(){ System.out.println(name+"What are you doing"); } } class tager extends Animal { String name; // Here is the parameter constructor of tag. If no parameter constructor is defined, there is only a parameter constructor. public tager(String myName) { this.name = myName; System.out.println("I'm tiger 01"+name); } public tager() { System.out.println("I'm tiger 02"+name); } public void doing(){ System.out.println(name+"What are you doing"); } } class Test{ public static void main(String[] args) { tager tager = new tager(); tager tager2 = new tager("aa"); } }
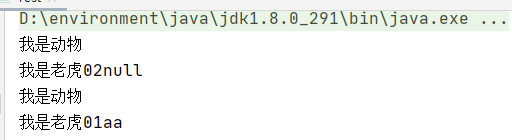
The default call is parameterless construction. If the parent class does not have parameterless construction at this time, the child class must call the construction method of the specified parameter through super().
If the parent class has a parameterless constructor, the child class constructor can call the parameterless constructor of the parent class without using super. After executing the parent constructor, execute the child constructor.
The following is an example code of B inheriting A, C inheriting B and C inheriting A:
package com.edu.java; // A class Animal { private String name; private int id; public Animal(String myName, int myid) { name = myName; id = myid; } public Animal(){ } public void doing(){ System.out.println(name+"What are you doing"); } public void begoodat(){ System.out.println(name+"What are you good at"); } public void introduction() { System.out.println("hello everyone! I am"+ id + "number" + name + "."); } } // B inherits A class tager extends Animal { String name; // Here is the parameter constructor of tag. If no parameter constructor is defined, there is only a parameter constructor. public tager(String myName) { this.name = myName; } public tager() { } // For the convenience of testing, we define a parameterless constructor here // If the parent class has only a parameterless constructor, the parameterless constructor of the child class can only use super to call the parameterless constructor of the parent class. public void doing() { System.out.println(name+"Eating meat"); } public void begoodat() { System.out.println(name+"Good at running"); } public void type(){ System.out.println(name+"It belongs to the cat family"); } public void xingge(){ System.out.println(name+"Fierce character"); } } // C inherits B class wolf extends tager{ public wolf(String myName) { super(myName); } public void doing(String name) { System.out.println(name+"Eating meat"); } public void begoodat(String name) { System.out.println(name+"Good at running"); } public void type(String name){ System.out.println(name+"It belongs to the dog family"); } public void live(String name){ System.out.println(name+"It belongs to social animals"); } } //C inherits A class wolf1 extends Animal{ public void doing(String name) { System.out.println(name+"Eating meat"); } public void begoodat(String name) { System.out.println(name+"Good at running"); } public void type(String name){ System.out.println(name+"It belongs to social animals"); } public void tixing(String name){ System.out.println(name+"Slender body"); } } class Test{ public static void main(String[] args) { tager tager = new tager("laohu"); tager.begoodat(); tager.doing(); tager.type(); // If there are parameters in the doing() of the subclass, if there is no parameter in the main, there will be a doing() method without parameters in the parent class. If not, it will be reported directly. // tager.begoodat("laohu"); // tager.doing("laohu"); System.out.println("==========================="); wolf wolf = new wolf("lang"); wolf.begoodat(); //Call the method of the parent class wolf.type(); //Call the method of the parent class wolf.xingge();//Call the method of the parent class wolf.begoodat("lang"); //Call methods of subclasses System.out.println("==========================="); // Because B inherits A and C inherits B, because there are extended methods in B, such as xingge() // It cannot be implemented in C's direct inheritance of A, but C's direct inheritance of A adds another tixing() method wolf1 wolf1 = new wolf1(); wolf1.tixing("xiaolang"); wolf1.begoodat("xiaolang"); } }
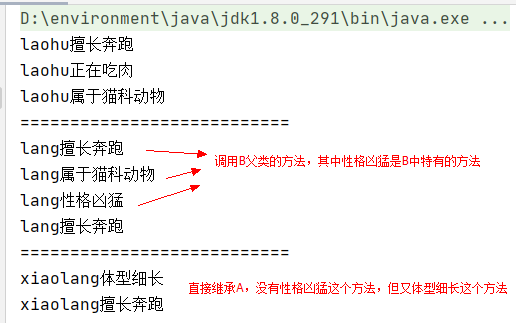
Because B may extend the function of A in the middle. If C directly inherits A, C is at the same level as B.