The implementation effect is as follows: after obtaining the keyboard height, manually move the input box:

Although the pop-up of the input box is not smooth enough, it seems a little stiff, but we can add appropriate animation to improve it.
The method is to cover a PopupWindow with a width of 0 and a height of match [parent] for the current activity. Set the mSoftInputMode of PopupWindow to soft [input] adjust [resize]. After the keyboard pops up, calculate the height of the keyboard pop-up according to the change of the height of the PopupWindow content area.
The core code is as follows:
public class HeightProvider extends PopupWindow implements OnGlobalLayoutListener { private Activity mActivity; private View rootView; private HeightListener listener; private int heightMax; // Record the maximum height of the pop content area public HeightProvider(Activity activity) { super(activity); this.mActivity = activity; // Basic configuration rootView = new View(activity); setContentView(rootView); // Monitor global Layout changes rootView.getViewTreeObserver().addOnGlobalLayoutListener(this); setBackgroundDrawable(new ColorDrawable(0)); // Set width to 0 and height to full screen setWidth(0); setHeight(LayoutParams.MATCH_PARENT); // Set keyboard pop-up mode setSoftInputMode(LayoutParams.SOFT_INPUT_ADJUST_RESIZE); setInputMethodMode(PopupWindow.INPUT_METHOD_NEEDED); } public HeightProvider init() { if (!isShowing()) { final View view = mActivity.getWindow().getDecorView(); // Delay loading popupwindow, if not, error will be reported view.post(new Runnable() { @Override public void run() { showAtLocation(view, Gravity.NO_GRAVITY, 0, 0); } }); } return this; } public HeightProvider setHeightListener(HeightListener listener) { this.listener = listener; return this; } @Override public void onGlobalLayout() { Rect rect = new Rect(); rootView.getWindowVisibleDisplayFrame(rect); if (rect.bottom > heightMax) { heightMax = rect.bottom; } // The difference between the two is the height of the keyboard int keyboardHeight = heightMax - rect.bottom; if (listener != null) { listener.onHeightChanged(keyboardHeight); } } public interface HeightListener { void onHeightChanged(int height); } }
The usage code is as follows:
public class MainActivity extends AppCompatActivity { private EditText etBottom; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); etBottom = findViewById(R.id.etBottom); new HeightProvider(this).init().setHeightListener(new HeightProvider.HeightListener() { @Override public void onHeightChanged(int height) { etBottom.setTranslationY(-height); } }); } } <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:layout_width="match_parent" android:layout_height="match_parent" android:scaleType="centerCrop" android:src="@drawable/test" /> <EditText android:id="@+id/etBottom" android:layout_width="match_parent" android:layout_height="50dp" android:layout_alignParentBottom="true" android:background="#ffabcdef" /> </RelativeLayout>
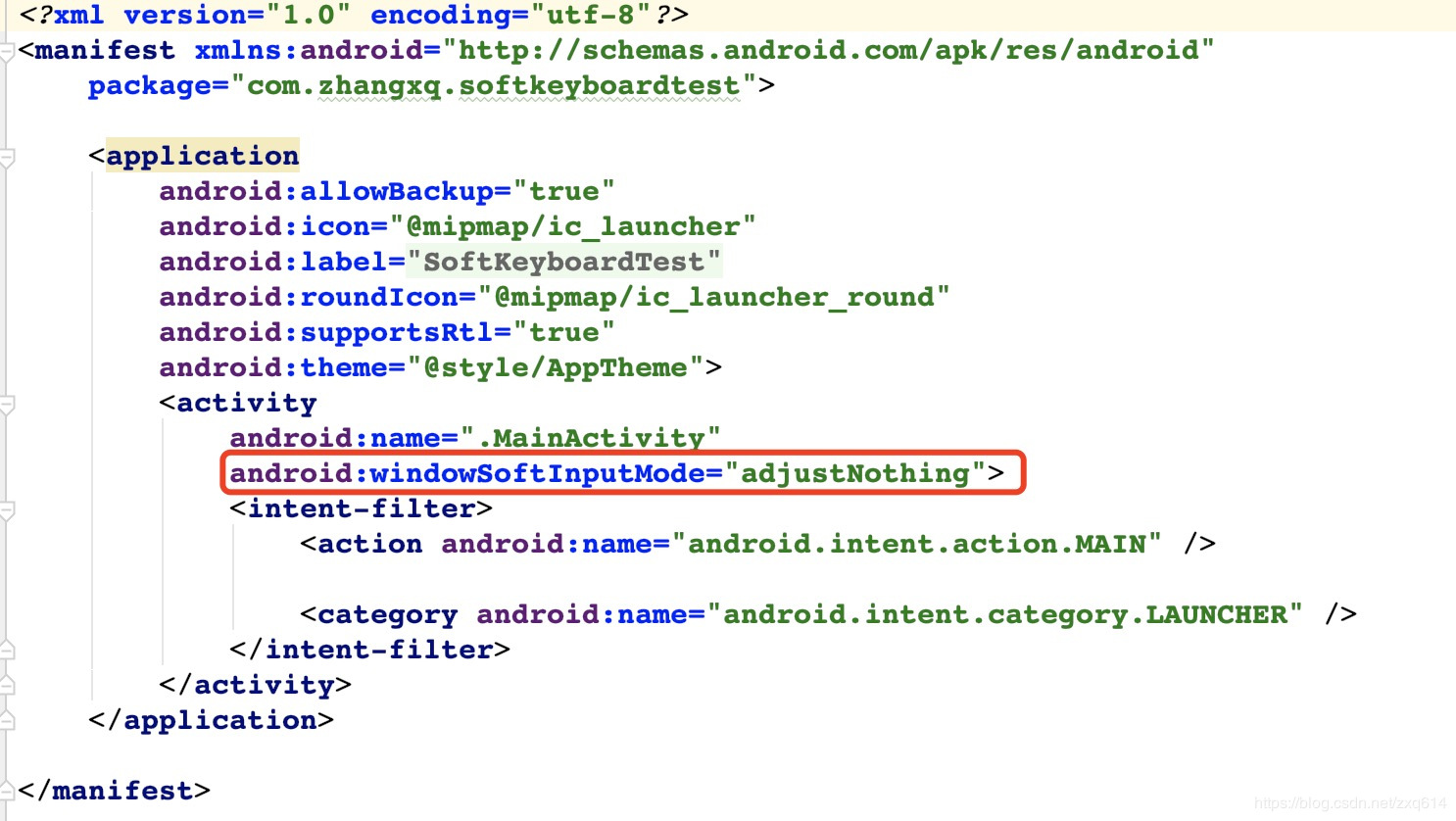
It is very simple to use. You can use the highprovider as a tool class.