1. configuration:
1.1 add permissions and FileProvider in Android manifest.xml:
-------------------------------------------------------------------------------------------------------------------- <uses-permission android:name="android.permission.INTERNET"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.REQUEST_INSTALL_PACKAGES" /> -------------------------------------------------------------------------------------------------------------------- <provider android:name="androidx.core.content.FileProvider" android:authorities="com.fengzhi.wuyemanagement.fileprovider" android:grantUriPermissions="true" android:exported="false"> <meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths" /> </provider>
1.2 create a new file (path: res\xml\file_paths.xml):
<paths> <external-path path="." name="external_storage_root" /> </paths>
1.3 build.gradle:
implementation "com.lzy.net:okgo:3.0.4"//okgo network request implementation 'com.google.code.gson:gson:2.8.2'//gson implementation "org.permissionsdispatcher:permissionsdispatcher:4.3.1"//Jurisdiction annotationProcessor "org.permissionsdispatcher:permissionsdispatcher-processor:4.3.1"//Jurisdiction
2. Click the button to update as an example:
2.1 core code:
private int version; /* Update progress bar */ private ProgressBar mProgress; private AlertDialog mDownloadDialog; -------------------------------------------------------------------------------------------------------------------- //Click the button, check the authority,,, and check the update method @NeedsPermission({Manifest.permission.READ_EXTERNAL_STORAGE, Manifest.permission.WRITE_EXTERNAL_STORAGE, Manifest.permission.REQUEST_INSTALL_PACKAGES}) protected void checkUpdate() { showLoadingDialog("Checking for updates..."); version = AppUpdateUtil.getAppVersionCode(this);//Check current version number // Call the method,,, the specific implementation of the interface, receive the passed parameters, and then call your own method, requestAppUpdate(version, new DataRequestListener<UpdateAppBean>() { @Override public void success(UpdateAppBean data) { // When the returned json getStatus is 0, download the apk file. Here is the method to download the apk file updateApp(data.getData().getApk_url()); } @Override public void fail(String msg) { // When the returned json getStatus is 1, the prompt: "it is the latest version! " SToast(msg); dismissLoadingDialog(); } }); } //Check the version number, first request (post),, UpdateAppBean generates according to the server return private void requestAppUpdate(int version, final DataRequestListener<UpdateAppBean> listener) { OkGo.<String>post(Const.HOST_URL + Const.UPDATEAPP).params("version", version).execute(new StringCallback() { @Override public void onSuccess(Response<String> response) { Gson gson = new Gson(); UpdateAppBean updateAppBean = gson.fromJson(response.body(), UpdateAppBean.class); if (updateAppBean.getStatus() == 0) { listener.success(updateAppBean); } else { listener.fail(updateAppBean.getMsg()); } } @Override public void onError(Response<String> response) { listener.fail("Server connection failed"); dismissLoadingDialog(); } }); } //If there is a new version, prompt for a new version, and then download the apk file private void updateApp(String apk_url) { dismissLoadingDialog(); DialogUtils.getInstance().showDialog(this, "New version found, Download update?", new DialogUtils.DialogListener() { @Override public void positiveButton() { downloadApp(apk_url); } }); } //Download apk file and jump (second request, get) private void downloadApp(String apk_url) { OkGo.<File>get(apk_url).tag(this).execute(new FileCallback() { @Override public void onSuccess(Response<File> response) { String filePath = response.body().getAbsolutePath(); Intent intent = IntentUtil.getInstallAppIntent(mContext, filePath); // After testing, you must use startactivity instead of stratactivityfor result mContext.startActivity(intent); dismissLoadingDialog(); mDownloadDialog.dismiss(); mDownloadDialog=null; } @Override public void downloadProgress(Progress progress) { // showDownloadDialog(); // mProgress.setProgress((int) (progress.fraction * 100)); if (mDownloadDialog == null) { // Build software download dialog AlertDialog.Builder builder = new AlertDialog.Builder(mContext); builder.setTitle("Updating"); // Add progress bar to download dialog final LayoutInflater inflater = LayoutInflater.from(mContext); View v = inflater.inflate(R.layout.item_progress, null); mProgress = (ProgressBar) v.findViewById(R.id.update_progress); builder.setView(v); mDownloadDialog = builder.create(); mDownloadDialog.setCancelable(false); mDownloadDialog.show(); } mProgress.setProgress((int) (progress.fraction * 100)); } }); }
2.2 DataRequestListener:
public interface DataRequestListener<T> { //Request successful void success(T data); //request was aborted void fail(String msg); }
Next is the tool class, from github, reference, https://github.com/vondear/RxTool
2.3 AppUpdateUtil:
/** * Get App version code * * @param context context * @return App Version code */ public static int getAppVersionCode(Context context) { return getAppVersionCode(context, context.getPackageName()); }
2.4 IntentUtil:
public class IntentUtil { /** * Get the intention to install app (support 7.0) * * @param context * @param filePath * @return */ public static Intent getInstallAppIntent(Context context, String filePath) { //Local path to apk file File apkfile = new File(filePath); if (!apkfile.exists()) { return null; } Intent intent = new Intent(Intent.ACTION_VIEW); Uri contentUri = FileUtil.getUriForFile(context, apkfile); intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) { intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION); } intent.setDataAndType(contentUri, "application/vnd.android.package-archive"); return intent; }
2.5 FileUtil:
/** * Convert files to URIs (7.0 supported) * * @param mContext * @param file * @return */ public static Uri getUriForFile(Context mContext, File file) { Uri fileUri = null; if (Build.VERSION.SDK_INT >= 24) { fileUri = FileProvider.getUriForFile(mContext, mContext.getPackageName() + ".fileprovider", file); } else { fileUri = Uri.fromFile(file); } return fileUri; }
3. Problems encountered
9.0 mobile phone authorities configuration error, resulting in unable to install,
terms of settlement:
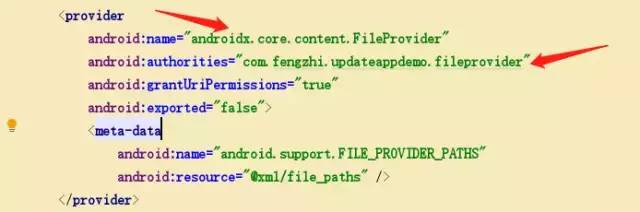
1. Android x is used in the project, and the file provider of Android x must be used in the configuration of Android manifest.xml
2. The authors here are the same as the ones in FileUtil.java. I just capitalized the letter P, resulting in an error
