In recent projects, the function of startup and self startup is useful. Here is a summary for everyone to learn and discuss!
Actual combat drill
Test mobile phone: Hongmi mobile phone Redmi 6A Android version 9
Huawei mobile phone DUA-AL00 Android version 8.1.0
Xiaomi tablet MI PAD 4 Android version 8.1.0
The above models have been tested successfully
For the basic idea of startup and self startup:
- To realize the self startup of app, we use broadcast to realize this requirement. The app first needs to register a broadcast about receiving and sending startup (android.intent.action.BOOT_COMPLETED); Moreover, it must be noted that this broadcast must be statically registered, not dynamically registered (this kind of broadcast that receives the startup broadcast must be statically registered, so that the application can still receive the startup broadcast before it runs
, dynamic broadcasting will not work. - After the broadcast is statically registered, you can process it in the onReceive method
- Key points
(1) After installing the application, run it once, then shut down and start it again.
(2) For andorid4 After 0, you need to start the APP once before you can receive the broadcast after startup. The main purpose is to prevent malicious programs
(3) As far as possible, only one APP of the same device is allowed to realize the function of startup and self startup, otherwise there should be a conflict between the two
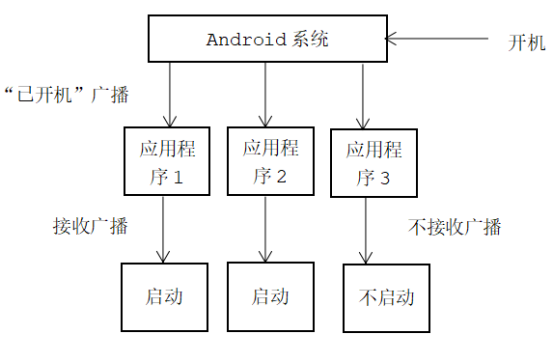
Concrete implementation
//Join permission <uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
For the processing in onReceive method, some models do not work. Here are two implementation methods:
1. Start the application according to the package name
2. Specify the class and jump to the corresponding Activity
/** * @author Martin-harry * @date 2022/3/4 * @address * @Desc Define self starting BroadcastReceiver */ public class AutoStartBroadReceiver extends BroadcastReceiver { private static final String ACTION = "android.intent.action.BOOT_COMPLETED"; @Override public void onReceive(Context context, Intent intent) { Log.e("Receive broadcast", "onReceive: "); Log.e("Receive broadcast", "onReceive: " + intent.getAction()); //Power on if (ACTION.equals(intent.getAction())) { Log.e("Receive broadcast", "onReceive: Started..."); //The first method: according to the package name // PackageManager packageManager = context.getPackageManager(); // Intent mainIntent = packageManager.getLaunchIntentForPackage("com.harry.martin"); // mainIntent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // context.startActivity(mainIntent); // context.startService(mainIntent); //The second method: specify the class and jump to the corresponding activity Intent mainIntent = new Intent(context, LoginActivity.class); /** * Intent.FLAG_ACTIVITY_NEW_TASK * Intent.FLAG_ACTIVITY_CLEAR_TOP */ mainIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); context.startActivity(mainIntent); // context.startService(mainIntent); } } }
Register broadcast
<receiver android:name=".receiver.AutoStartBroadReceiver" android:exported="true"> <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED" /> <category android:name="android.intent.category.HOME" /> </intent-filter> </receiver> <activity android:name=".mvp.view.Activity.LoginActivity" android:enabled="true" android:exported="true"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Scenario test
After completing the above work, here is the permission management operation for some mobile applications
ComponentName localComponentName = new ComponentName(MyApp.getInstance(), MyInstalledReceiver.class); int i = MyApp.getInstance().getPackageManager().getComponentEnabledSetting(localComponentName); // Log.e("self start > > > >", "oncreate:" + I); getAutostartSettingIntent(this);
/** * Get the Intent of the self startup management page * * @param context context * @return Management page of Intent returned from Startup */ public static Intent getAutostartSettingIntent(Context context) { ComponentName componentName = null; String brand = Build.MANUFACTURER; Intent intent = new Intent(); intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); switch (brand.toLowerCase()) { case "samsung"://Samsung componentName = new ComponentName("com.samsung.android.sm", "com.samsung.android.sm.app.dashboard.SmartManagerDashBoardActivity"); break; case "huawei"://Huawei Log.e("Self start management >>>>", "getAutostartSettingIntent: Huawei"); componentName = new ComponentName("com.huawei.systemmanager", "com.huawei.systemmanager.appcontrol.activity.StartupAppControlActivity"); // componentName = new ComponentName("com.huawei.systemmanager", "com.huawei.systemmanager.startupmgr.ui.StartupNormalAppListActivity");// At present, it is universal break; case "xiaomi"://millet // componentName = new ComponentName("com.miui.securitycenter", "com.miui.permcenter.autostart.AutoStartManagementActivity"); componentName = new ComponentName("com.android.settings","com.android.settings.BackgroundApplicationsManager"); break; case "vivo"://VIVO // componentName = new ComponentName("com.iqoo.secure", "com.iqoo.secure.safaguard.PurviewTabActivity"); componentName = new ComponentName("com.iqoo.secure", "com.iqoo.secure.ui.phoneoptimize.AddWhiteListActivity"); break; case "oppo"://OPPO // componentName = new ComponentName("com.oppo.safe", "com.oppo.safe.permission.startup.StartupAppListActivity"); componentName = new ComponentName("com.coloros.oppoguardelf", "com.coloros.powermanager.fuelgaue.PowerUsageModelActivity"); break; case "yulong": case "360"://360 componentName = new ComponentName("com.yulong.android.coolsafe", "com.yulong.android.coolsafe.ui.activity.autorun.AutoRunListActivity"); break; case "meizu"://Meizu componentName = new ComponentName("com.meizu.safe", "com.meizu.safe.permission.SmartBGActivity"); break; case "oneplus"://One plus componentName = new ComponentName("com.oneplus.security", "com.oneplus.security.chainlaunch.view.ChainLaunchAppListActivity"); break; case "letv"://letv intent.setAction("com.letv.android.permissionautoboot"); default://other intent.setAction("android.settings.APPLICATION_DETAILS_SETTINGS"); intent.setData(Uri.fromParts("package", context.getPackageName(), null)); break; } intent.setComponent(componentName); return intent; }
About authorization operation of Hongmi mobile phone:
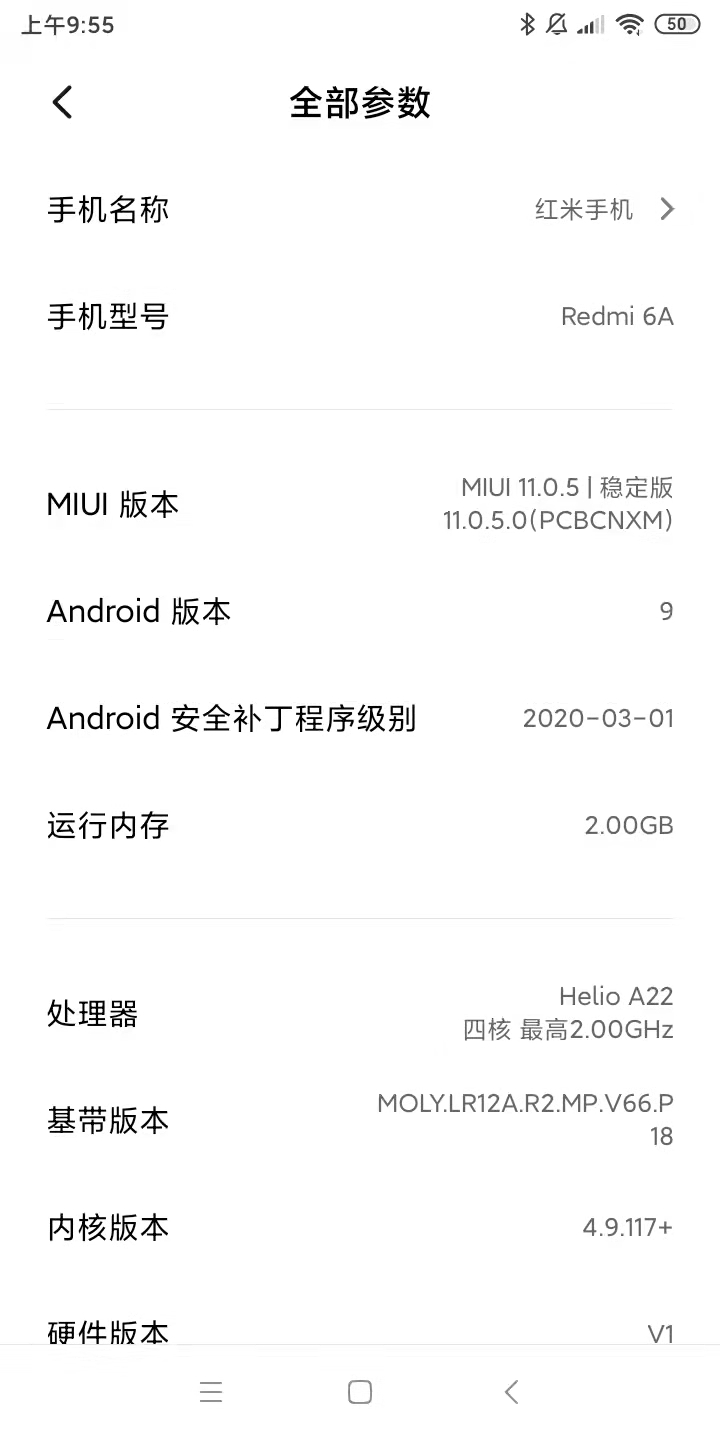
Find the mobile housekeeper application in Hongmi mobile phone and open the application management
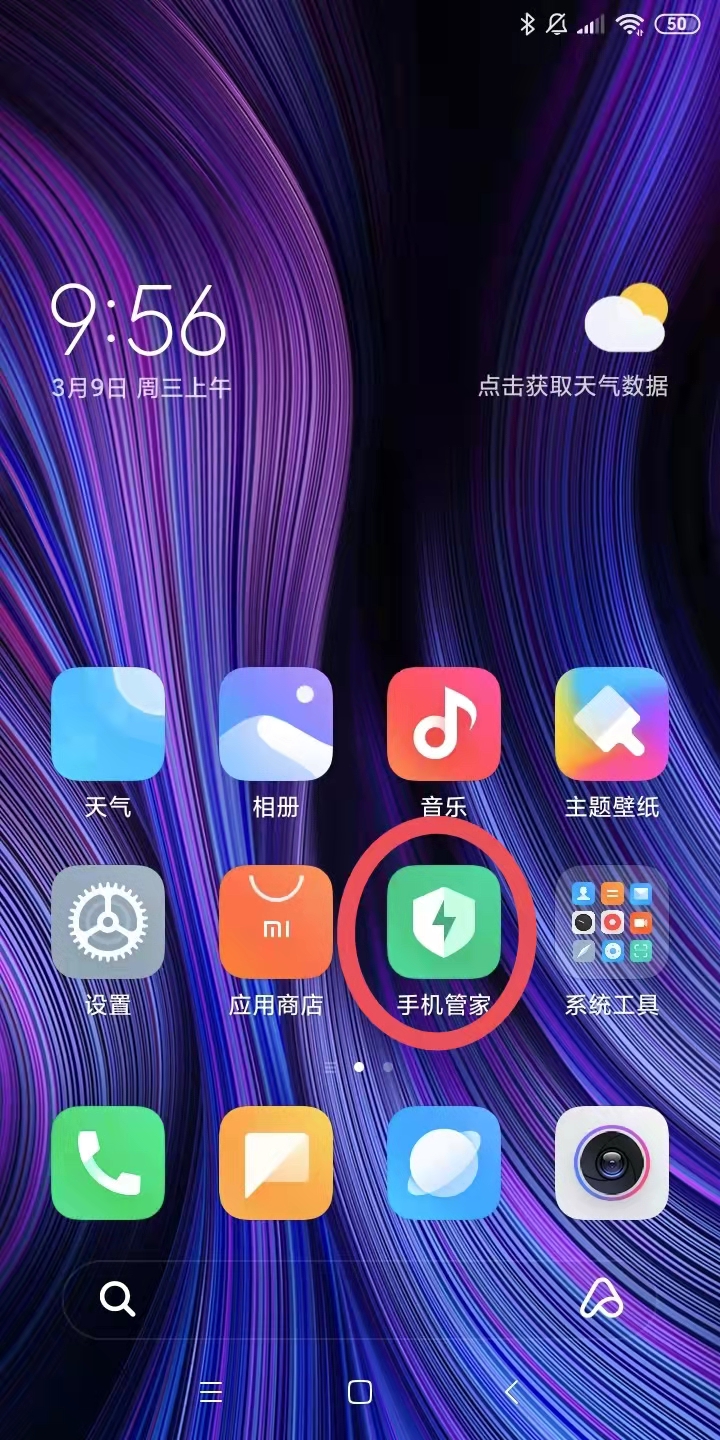
Open authorization management, set self startup permission for your application, and allow the application to pop up the interface in the background
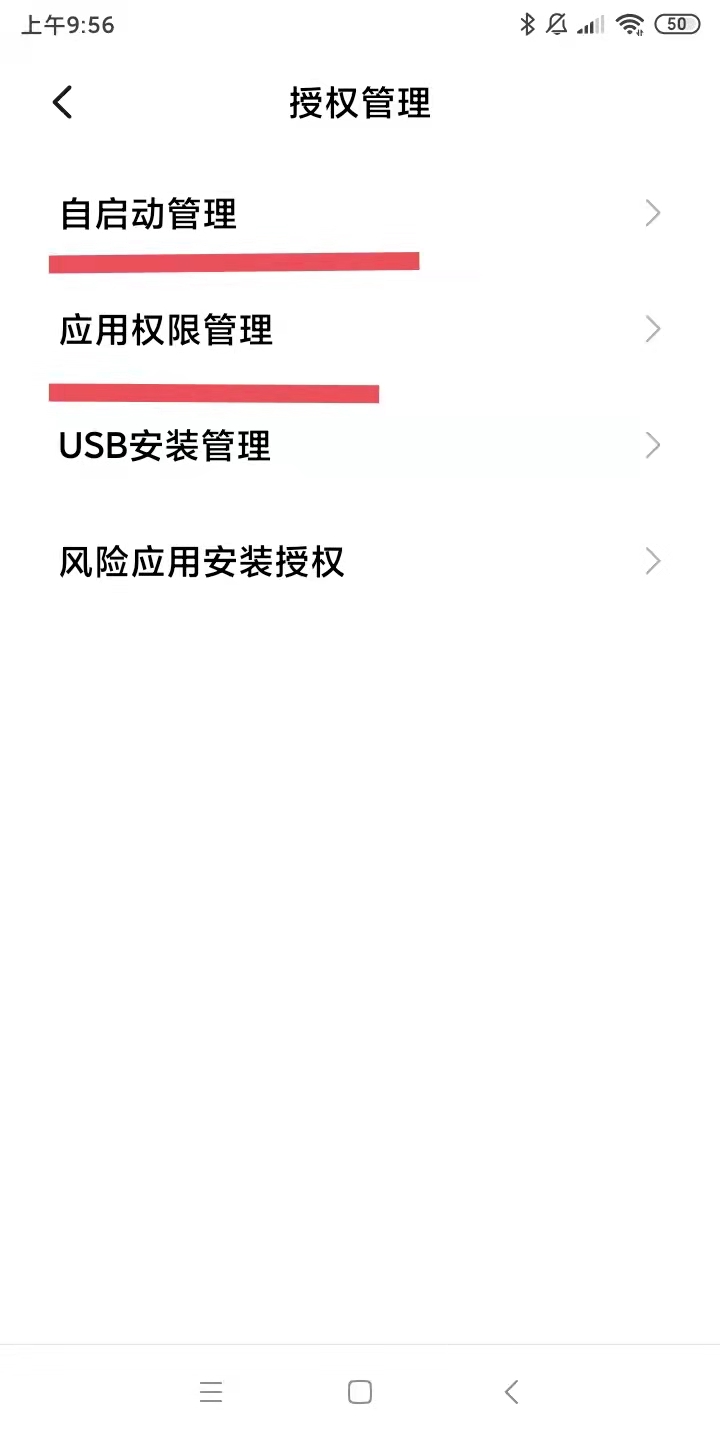
About Huawei mobile phone authorization:
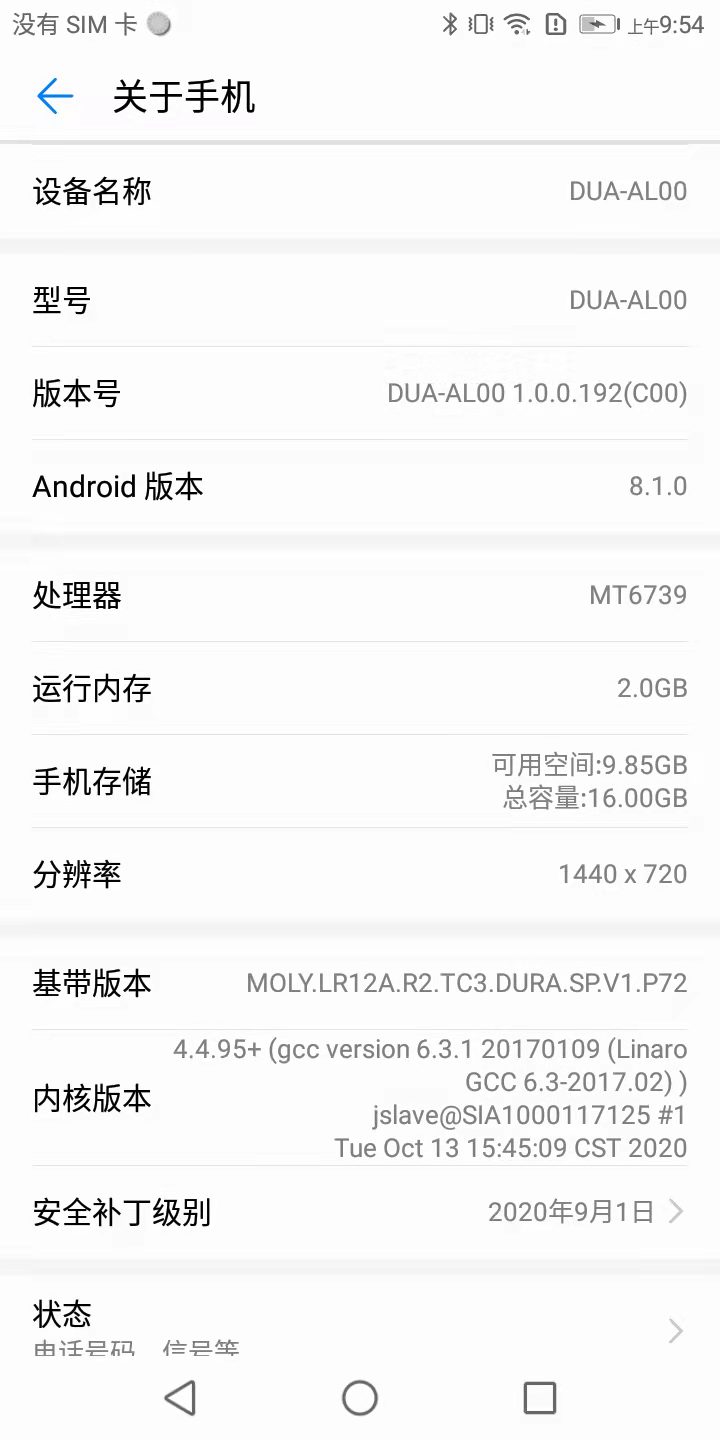
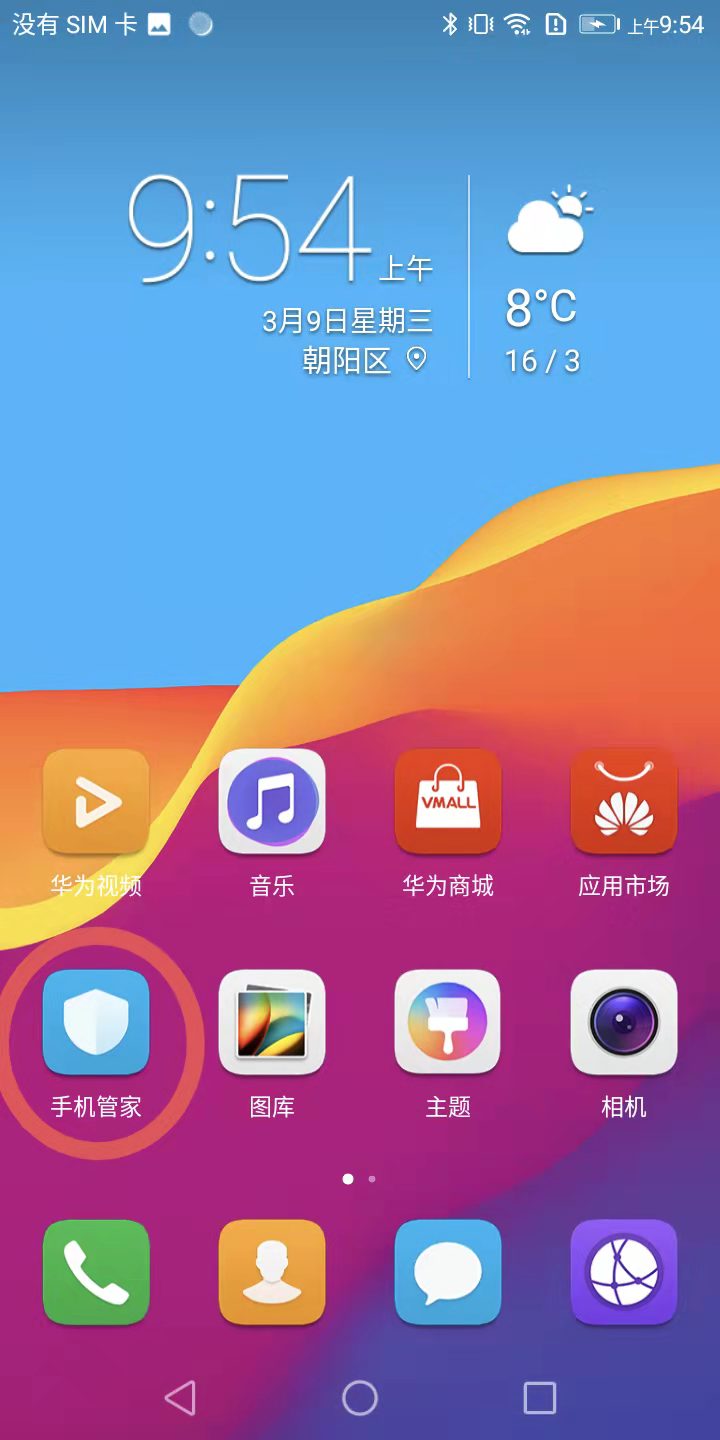
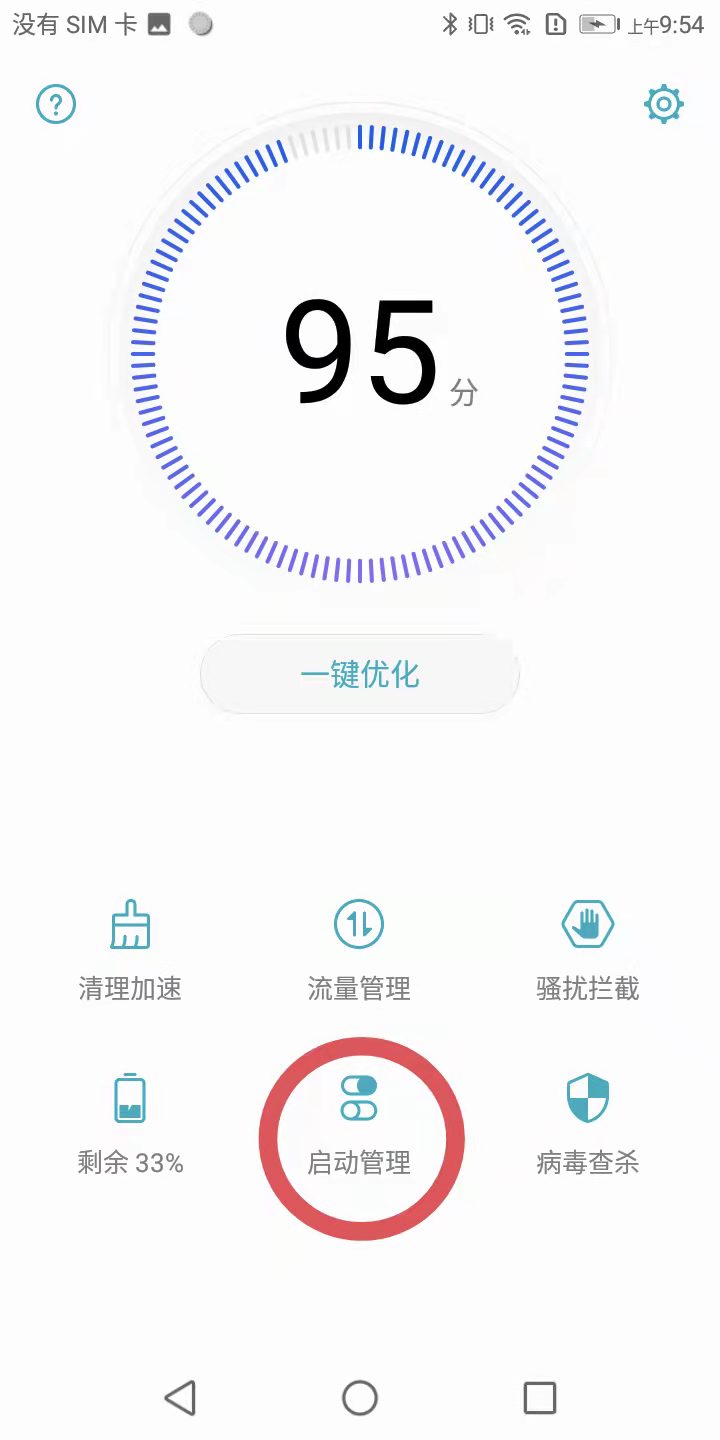
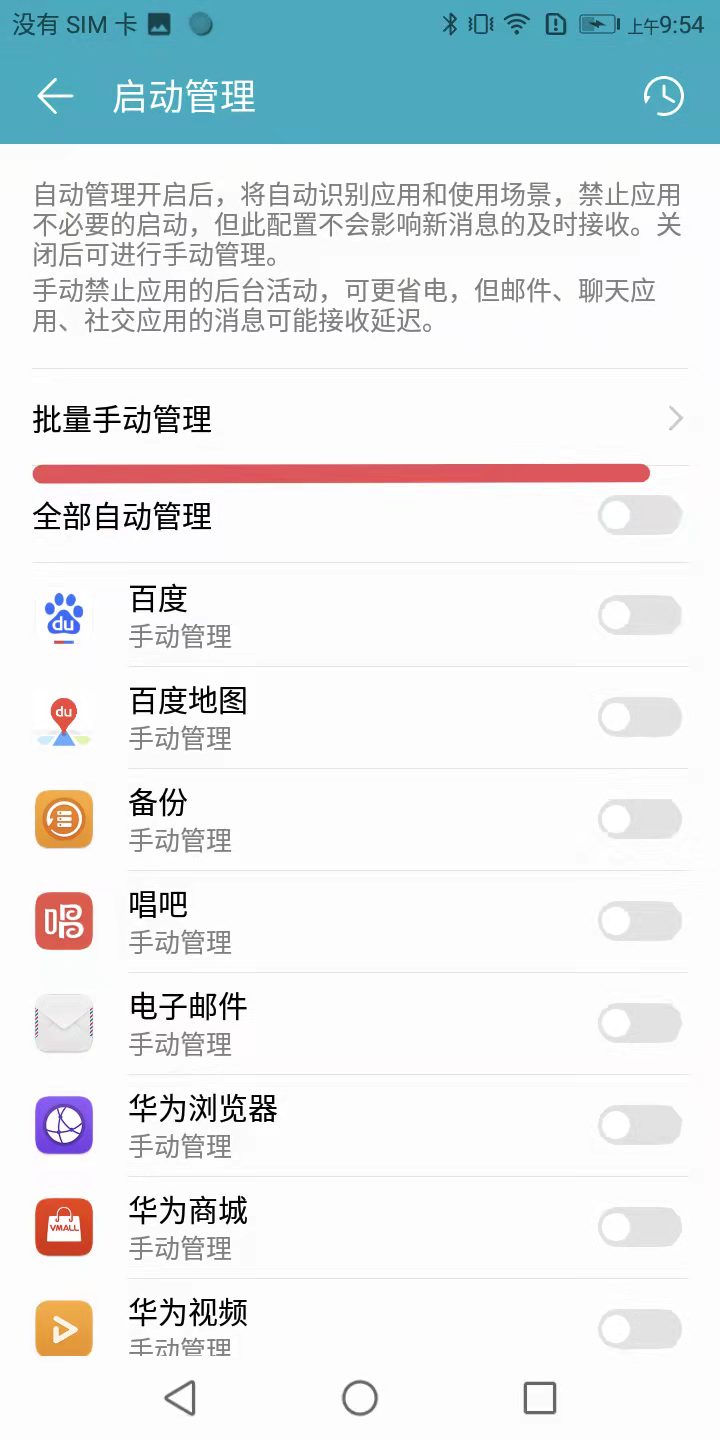
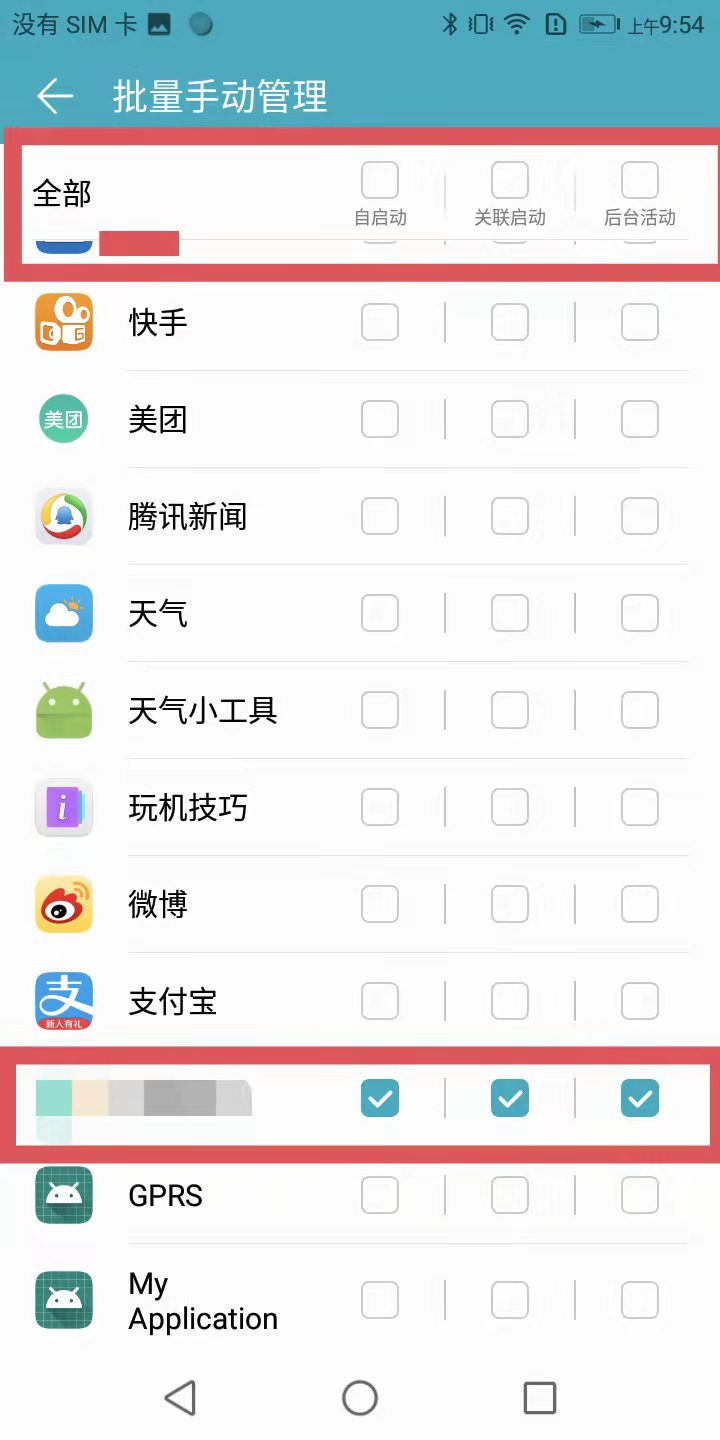
summary
When I was testing, I had to wait for a period of time for the red rice mobile phone to start automatically. If any boss knows how to solve it, I can comment below. Thank you
Digression:
In another way, it's best to let the system desktop application start you, Launcher
PackageName: comp.xxx.launcher
launcher is an app written in java, but it is a system application. However, if you take this code, it seems that you need fw's help or you can work it out by yourself
Specifically, add an intent in the MainActivity of the Launcher to jump to the package name of your app