Five years of Android development, during which do stop and stop (to do background development, server management), when returning to Android, found that very strange, many controls used to write smoothly, now seems to forget something, always open the project, open the project, sometimes not necessarily find it.
To sum up, it is still due to the lack of a proper code base to encapsulate some customized controls or modules with low coupling in the usual development projects, or to write fewer blogs in peacetime. If you are a programming ape who has just learned to develop, or a big bird who has several years of development experience, you should also start sorting out your own code, which is not in vain for the years of knocking code in this lifetime. At the same time, in the interview, it will bring you a lot of impressions. So, I started to prepare my own code base, put it on github or microblog, hoping to communicate with you gods. Next, let me put one or two custom controls.
Customize a set of Dialog universal prompt boxes:
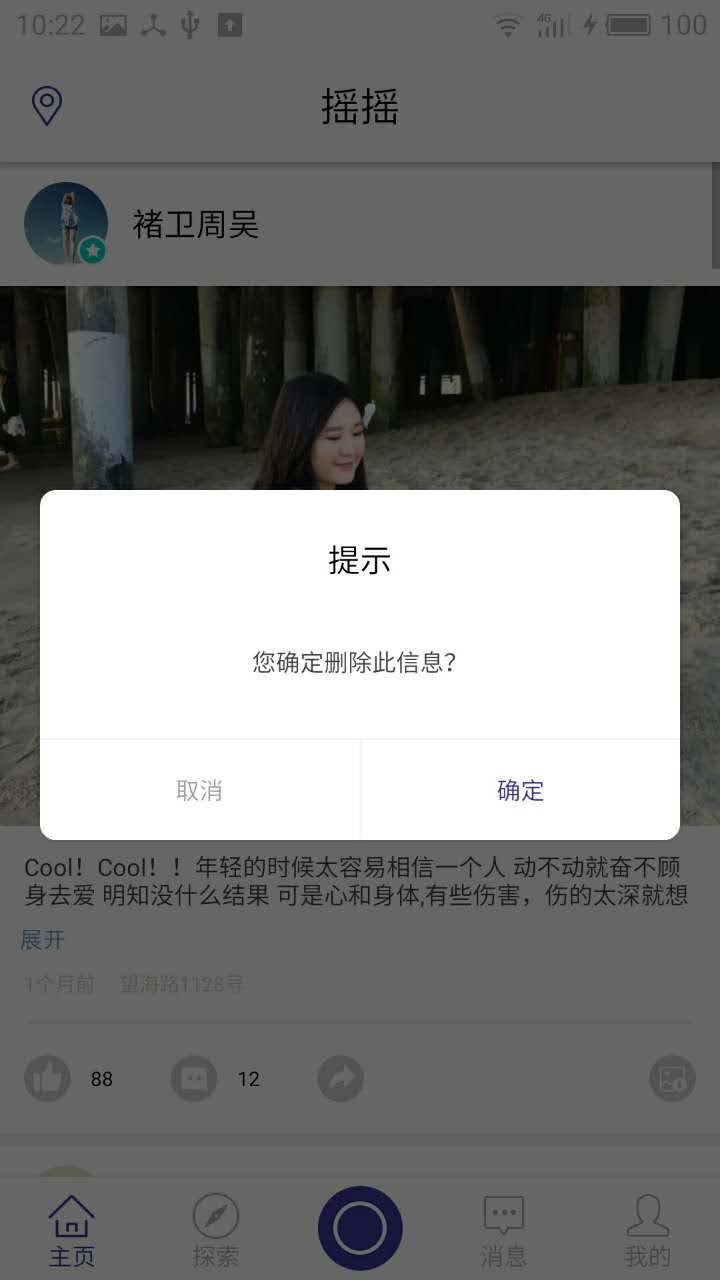
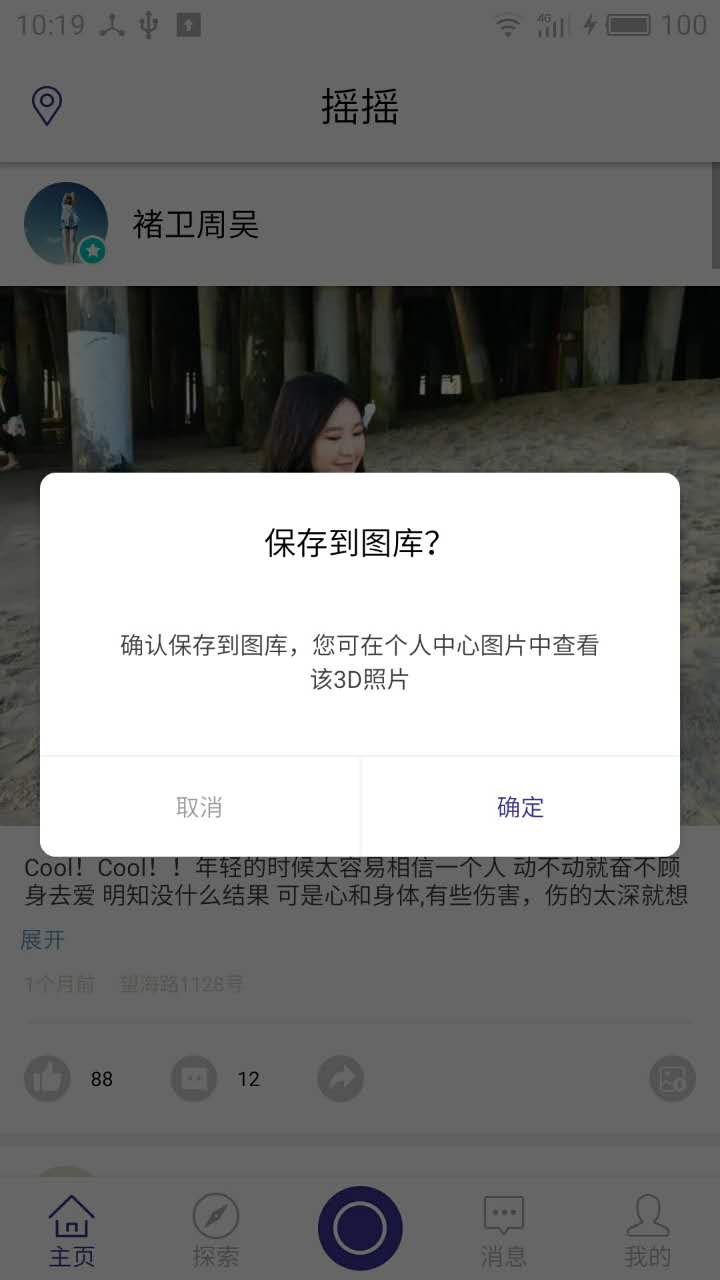
Appeal prompt boxes are all of a kind, of course, you may not be satisfied with, or inconsistent with the style of your designer's requirements, it doesn't matter, you just go in and modify the layout of dialog. Of course, I hope that when I customize these controls, I can use xml to render them, and try not to use pictures to do background and so on. Each app's prompt box style is basically the same, not every page's prompt box is different, if it really changes too much, we can re-customize a dialog. Others only need to set up information:
new CommomDialog(mContext, R.style.dialog, "Are you sure you want to delete this information?", new CommomDialog.OnCloseListener() { @Override public void onClick(boolean confirm) { if(confirm){ Toast.makeText(this,"Click OK", Toast.LENGTH_SHORT).show(); dialog.dismiss(); } } }) .setTitle("Tips").show();
Let's first look at the CommomDialog class. When this class is defined, the methods in it are as chained as possible to facilitate later calls.
public class CommomDialog extends Dialog implements View.OnClickListener{ private TextView contentTxt; private TextView titleTxt; private TextView submitTxt; private TextView cancelTxt; private Context mContext; private String content; private OnCloseListener listener; private String positiveName; private String negativeName; private String title; public CommomDialog(Context context) { super(context); this.mContext = context; } public CommomDialog(Context context, int themeResId, String content) { super(context, themeResId); this.mContext = context; this.content = content; } public CommomDialog(Context context, int themeResId, String content, OnCloseListener listener) { super(context, themeResId); this.mContext = context; this.content = content; this.listener = listener; } protected CommomDialog(Context context, boolean cancelable, OnCancelListener cancelListener) { super(context, cancelable, cancelListener); this.mContext = context; } public CommomDialog setTitle(String title){ this.title = title; return this; } public CommomDialog setPositiveButton(String name){ this.positiveName = name; return this; } public CommomDialog setNegativeButton(String name){ this.negativeName = name; return this; } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.dialog_commom); setCanceledOnTouchOutside(false); initView(); } private void initView(){ contentTxt = (TextView)findViewById(R.id.content); titleTxt = (TextView)findViewById(R.id.title); submitTxt = (TextView)findViewById(R.id.submit); submitTxt.setOnClickListener(this); cancelTxt = (TextView)findViewById(R.id.cancel); cancelTxt.setOnClickListener(this); contentTxt.setText(content); if(!TextUtils.isEmpty(positiveName)){ submitTxt.setText(positiveName); } if(!TextUtils.isEmpty(negativeName)){ cancelTxt.setText(negativeName); } if(!TextUtils.isEmpty(title)){ titleTxt.setText(title); } } @Override public void onClick(View v) { switch (v.getId()){ case R.id.cancel: if(listener != null){ listener.onClick(this, false); } this.dismiss(); break; case R.id.submit: if(listener != null){ listener.onClick(this, true); } break; } } public interface OnCloseListener{ void onClick(Dialog dialog, boolean confirm); } }
Customize the listening event, set the message, return the handle, return this;
Look at the R.layout.dialog_commom xml file again
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/bg_round_white" android:orientation="vertical" > <TextView android:id="@+id/title" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center_horizontal" android:padding="12dp" android:layout_marginTop="12dp" android:text="Tips" android:textSize="16sp" android:textColor="@color/black"/> <TextView android:id="@+id/content" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:layout_gravity="center_horizontal" android:lineSpacingExtra="3dp" android:layout_marginLeft="40dp" android:layout_marginTop="20dp" android:layout_marginRight="40dp" android:layout_marginBottom="30dp" android:text="Check in successfully and get 200 points" android:textSize="12sp" android:textColor="@color/font_common_1"/> <View android:layout_width="match_parent" android:layout_height="1dp" android:background="@color/commom_background"/> <LinearLayout android:layout_width="match_parent" android:layout_height="50dp" android:orientation="horizontal"> <TextView android:id="@+id/cancel" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/bg_dialog_left_white" android:layout_weight="1.0" android:gravity="center" android:text="@string/cancel" android:textSize="12sp" android:textColor="@color/font_common_2"/> <View android:layout_width="1dp" android:layout_height="match_parent" android:background="@color/commom_background"/> <TextView android:id="@+id/submit" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/bg_dialog_right_white" android:gravity="center" android:layout_weight="1.0" android:text="@string/submit" android:textSize="12sp" android:textColor="@color/font_blue"/> </LinearLayout> </LinearLayout>
I use rounded corners for the whole background, so it doesn't seem particularly rigid. android:background="@drawable/bg_round_white"
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <solid android:color="@color/white" /> <corners android:radius="8dp" /> </shape>
Of course, the bottom two buttons also need to be rounded.
The bottom left button: android:background="@drawable/bg_dialog_left_white"
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <solid android:color="@color/white" /> <corners android:bottomLeftRadius="8dp" /> </shape>
Right-down button: android:background="@drawable/bg_dialog_right_white"
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <solid android:color="@color/white" /> <corners android:bottomRightRadius="8dp" /> </shape>
The style displayed should also be set up:
<style name="dialog" parent="@android:style/Theme.Dialog"> <item name="android:windowFrame">@null</item> <!--Frame--> <item name="android:windowIsFloating">true</item> <!--Does it float now? activity Above--> <item name="android:windowIsTranslucent">false</item> <!--translucent--> <item name="android:windowNoTitle">true</item> <!--No title--> <item name="android:windowBackground">@android:color/transparent</item> <!--Background transparency--> <item name="android:backgroundDimEnabled">true</item> <!--vague--> </style>
This is a basic success. By setting the header, body, button name and click event, you can control your prompt box at will.
Later, I will put up my own code base and learn with you.