Although the path object of photos can be obtained through the system album, you can't know more detailed information, such as picture name, file size, file path, etc., so you can't carry out personalized customization development. In order to open more file information, Android has designed a special media sharing library to allow developers to obtain more detailed media information through content components. For pictures, the path of album media library is mediastore Images. Media. EXTERNAL_ CONTENT_ URI, so you can traverse from the media library to get the picture list details through the content parser. To facilitate code management, first declare the following object variables:
private List<ImageInfo> mImageList = new ArrayList<ImageInfo>(); // Picture list private Uri mImageUri = MediaStore.Images.Media.EXTERNAL_CONTENT_URI; // Uri of album private String[] mImageColumn = new String[]{ // Array of field names for media library MediaStore.Images.Media._ID, // number MediaStore.Images.Media.TITLE, // title MediaStore.Images.Media.SIZE, // file size MediaStore.Images.Media.DATA}; // File path
Then query the picture information of the media library according to the content parser. For simplicity, only the first six pictures with the smallest file size are selected. The list loading code example is as follows:
// Load picture list private void loadImageList() { mImageList.clear(); // Empty picture list // Query the album of the system through the content parser and return the cursor of the result set. "_sizeasc" indicates that it is arranged in ascending order of file size Cursor cursor = getContentResolver().query(mImageUri, mImageColumn, null, null, "_size asc"); if (cursor != null) { // Next, traverse the result set and add it to the picture list one by one. For simplicity, select only the first six pictures for (int i=0; i<6 && cursor.moveToNext(); i++) { ImageInfo image = new ImageInfo(); // Create a picture information object image.setId(cursor.getLong(0)); // Set picture number image.setName(cursor.getString(1)); // Set picture name image.setSize(cursor.getLong(2)); // Set the file size of the picture image.setPath(cursor.getString(3)); // Set the file path of the picture mImageList.add(image); // Add to picture list } cursor.close(); // Close database cursor } }
Note that the above code obtains the file path in string format, while the MMS sending application requires A Uri type path object. Originally, the code "Uri.parse(path)" could convert the string into A Uri object, but from Android 7 Starting from 0, the system does not allow other applications to directly access the path in the old format. The file provider FileProvider must be used to obtain the legal Uri path, which is equivalent to that application A declares to share A file, and then application B can access the shared file. To do this, you need to configure the FileProvider again. The detailed configuration steps are described below. First, create a new xml folder in the res directory and create a file in the folder_ paths. xml, and then fill the following contents into the xml file to define several external file directories:
<paths> <external-path path="Android/data/com.example.chapter07/" name="files_root" /> <external-path path="." name="external_storage_root" /> </paths>
Then open androidmanifest XML, add the following provider tag at the end of the application node to declare the provider component of the current application. The configuration example of the added tag is as follows:
<!-- compatible Android7.0,To access a file Uri Change the mode to FileProvider --> <provider android:name="androidx.core.content.FileProvider" android:authorities="com.example.chapter07.fileProvider" android:exported="false" android:grantUriPermissions="true"> <meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths" /> </provider>
The above provider is changeable in two places. One is the authorities attribute, which specifies the authorization string, which is the unique identification of each provider; The other is the resource attribute of metadata, which indicates the path resource of the file provider, that is, the file just defined_ paths. xml. Go back to the active source code of the page and add the following code before sending MMS. The purpose is to build Uri objects according to the string path. Note that it is for Android 7 Compatible processing above 0.
Uri uri = Uri.parse(path); // Creates a Uri object based on the specified path // Compatible with Android 7 0, change the Uri method of accessing the file to FileProvider if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) { // Obtain the Uri access method of the file through the FileProvider uri = FileProvider.getUriForFile(this, BuildConfig.APPLICATION_ID + ".fileProvider", new File(path)); }
As can be seen from the above code, Android 7 0 starts calling the getUriForFile method of FileProvider to obtain the Uri object. The second parameter of the method is the authorization string of the File provider (BuildConfig.APPLICATION_ID value is the package name of the current application), and the third parameter is the File object of File type. After running the App, the test page will automatically load the six pictures found from the media library, and the interface effect of filling in all the information is shown in the figure below.
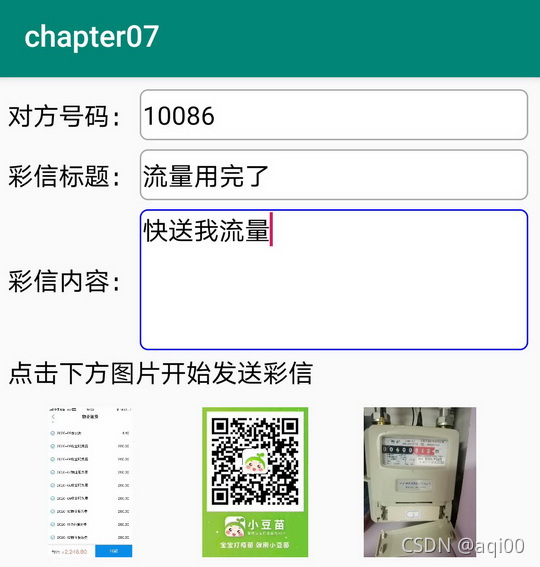
Finally, click a picture to select it as an MMS attachment. An application selection window as shown in the following figure pops up at the bottom of the interface.
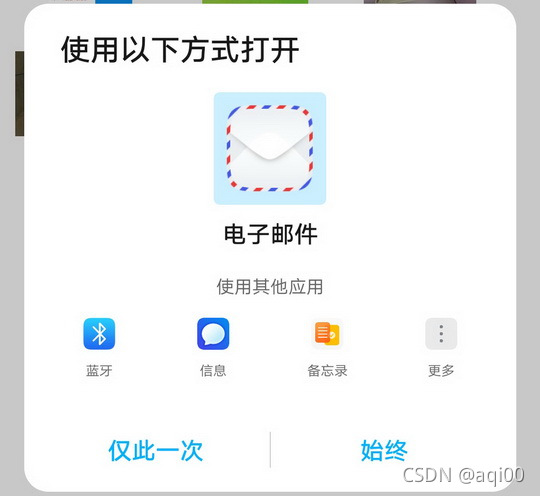
Select the message icon and click this button only once to jump to the system MMS sending page as shown in the figure below.
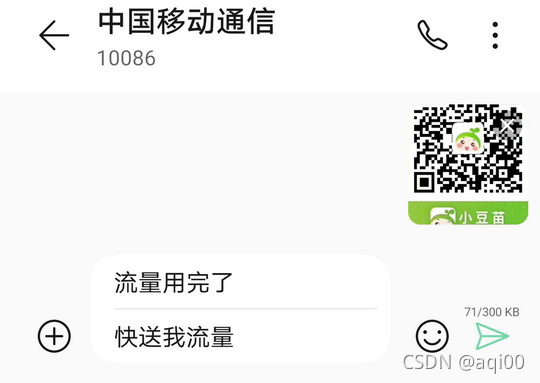
Click here to view the full directory of Android Development notes