screenshot
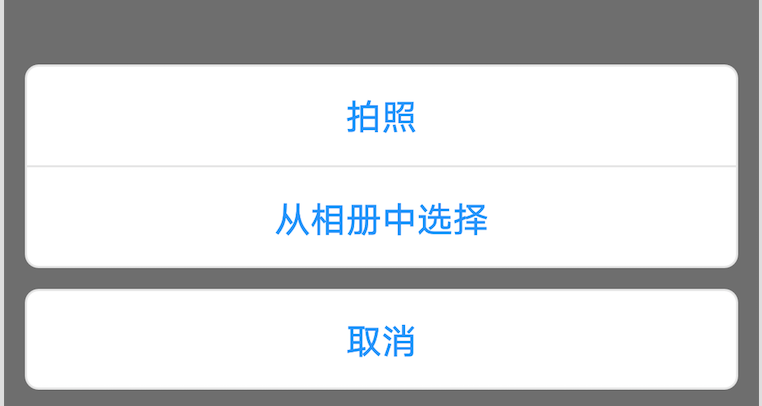
pw.png
Realization
1,BasePopupWindow.java
1.1. Realize dynamic loading of different layout s
1.2. Whether the background is translucent after the dynamic configuration is popped up and restored when it is closed (monitor ondismiss and use the window class to change color)
1.3. Some basic method abstraction methods
1.4. For more complex styles and dynamic effects, you can continue to expand this type
/** * Created by wujn on 2018/10/29. * Version : v1.0 * Function: base popuwindow */ public abstract class BasePopupWindow extends PopupWindow implements View.OnClickListener{ /** * context */ private Context context; /** * Top most background view */ private View vBgBasePicker; /** * Content viewgroup */ private LinearLayout llBaseContentPicker; public BasePopupWindow(Context context) { super(context); this.context = context; View parentView = View.inflate(context, R.layout.base_popup_window_picker, null); vBgBasePicker = parentView.findViewById(R.id.v_bg_base_picker); llBaseContentPicker = (LinearLayout) parentView.findViewById(R.id.ll_base_content_picker); /*** * Add layout to interface */ llBaseContentPicker.addView(View.inflate(context, bindLayout(), null)); setContentView(parentView); //Set the width of the PopupWindow pop-up this.setWidth(ViewGroup.LayoutParams.MATCH_PARENT); //Set PopupWindow pop-up height this.setHeight(ViewGroup.LayoutParams.WRAP_CONTENT); //Add the following code in the PopupWindow, so that the pop-up window will not be blocked when the keyboard pops up. this.setInputMethodMode(PopupWindow.INPUT_METHOD_NEEDED); this.setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_RESIZE); setFocusable(true);//Set get focus setTouchable(true);//Settings can be touched setOutsideTouchable(true);//Set the outside to click ColorDrawable dw = new ColorDrawable(0xffffff); setBackgroundDrawable(dw); //Animating the SelectPicPopupWindow pop-up this.setAnimationStyle(R.style.BottomDialogWindowAnim); initView(parentView); initListener(); initData(); vBgBasePicker.setOnClickListener(this); //Need screen translucency setBackgroundHalfTransition(isNeedBackgroundHalfTransition()); } /** * Initialize layout * * @return */ protected abstract int bindLayout(); /** * Initialize view * * @param parentView */ protected abstract void initView(View parentView); /** * Initialization data */ protected abstract void initData(); /** * Initialize listening */ protected abstract void initListener(); /** * In order to adapt to the above display problems of 7.0 system (displayed at the bottom of the control) * * @param anchor */ @Override public void showAsDropDown(View anchor) { if (Build.VERSION.SDK_INT >= 24) { Rect rect = new Rect(); anchor.getGlobalVisibleRect(rect); int h = anchor.getResources().getDisplayMetrics().heightPixels - rect.bottom; setHeight(h); } super.showAsDropDown(anchor); if(isNeedBgHalfTrans){ backgroundAlpha(0.5f); } } /** * Display at the bottom of the screen * * @param layoutid rootview */ public void showAtLocation(@LayoutRes int layoutid) { showAtLocation(LayoutInflater.from(context).inflate(layoutid, null), Gravity.TOP | Gravity.CENTER_HORIZONTAL, 0, 0); if(isNeedBgHalfTrans){ backgroundAlpha(0.5f); } } /** * Top view click event monitoring * * @param v */ @Override public void onClick(View v) { switch (v.getId()) { case R.id.v_bg_base_picker: dismiss(); break; } } /** * Whether to set background translucency * */ public boolean isNeedBackgroundHalfTransition(){ return false; } private boolean isNeedBgHalfTrans = false; private void setBackgroundHalfTransition(boolean isNeed){ isNeedBgHalfTrans = isNeed; if(isNeedBgHalfTrans){ this.setOnDismissListener(new OnDismissListener() { @Override public void onDismiss() { backgroundAlpha(1f); } }); } } /** * Set the background transparency of the add screen * @param bgAlpha */ private void backgroundAlpha(float bgAlpha) { WindowManager.LayoutParams lp = ((Activity)context).getWindow().getAttributes(); lp.alpha = bgAlpha; //0.0-1.0 ((Activity)context).getWindow().setAttributes(lp); } }
2,base_popup_window_picker.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <View android:id="@+id/v_bg_base_picker" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_weight="1" /> <LinearLayout android:id="@+id/ll_base_content_picker" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:orientation="vertical" /> </LinearLayout>
3. Animation style when entering and exiting
<!--animanation--> <!-- Bottom dialog Pop up animation style--> <style name="BottomDialogWindowAnim" parent="android:Animation"> <item name="android:windowEnterAnimation">@anim/popup_bottom_enter_anim</item> <item name="android:windowExitAnimation">@anim/popup_bottom_exit_anim</item> </style>
Enter the animation: pop? Bottom? Enter? Anim.xml
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <translate android:duration="200" android:fromYDelta="100%p" android:toYDelta="0" /> <alpha android:duration="200" android:fromAlpha="0.0" android:toAlpha="1.0" /> </set>
Exit Animation: pop? Bottom? Exit? Anim.xml
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:duration="200" android:fromYDelta="0" android:toYDelta="50%p" /> <alpha android:duration="200" android:fromAlpha="1.0" android:toAlpha="0.0" /> </set>
4. layout imitating IOS: pop > Window > album > or > camera.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:padding="10dp"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@drawable/bg_shape_corner_white" android:orientation="vertical"> <Button android:id="@+id/btnCamera" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@null" android:text="Photograph" android:textColor="@color/dodgerblue" android:textSize="@dimen/normal_text_size" /> <View android:layout_width="match_parent" android:layout_height="1dp" android:background="@color/gray_1" /> <Button android:id="@+id/btnAlbum" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@null" android:text="Choose from album" android:textColor="@color/dodgerblue" android:textSize="@dimen/normal_text_size" /> </LinearLayout> <LinearLayout android:layout_marginTop="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@drawable/bg_shape_corner_white" android:orientation="vertical"> <Button android:id="@+id/btnCancel" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@null" android:text="@string/cancel" android:textColor="@color/dodgerblue" android:textSize="@dimen/normal_text_size" /> </LinearLayout> </LinearLayout>
Background style of item
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <solid android:color="@color/white" /> <corners android:radius="6dp"/> <stroke android:width="1dp" android:color="@color/gray_1" /> </shape>
5. popuwindow class imitating IOS: albumorcamerapapopupwindow
public class AlbumOrCameraPopupWindow extends BasePopupWindow { private Button btnCamera; private Button btnAlbum; private Button btnCancel; private OnCameraOrAlbumSelectListener onCameraOrAlbumSelectListener; public AlbumOrCameraPopupWindow(Context context,OnCameraOrAlbumSelectListener onCameraOrAlbumSelectListener) { super(context); this.onCameraOrAlbumSelectListener = onCameraOrAlbumSelectListener; } @Override public boolean isNeedBackgroundHalfTransition(){ return true; } @Override protected int bindLayout() { return R.layout.popup_window_album_or_camera; } @Override protected void initView(View parentView) { btnCamera = (Button) parentView.findViewById(R.id.btnCamera); btnAlbum = (Button) parentView.findViewById(R.id.btnAlbum); btnCancel = (Button) parentView.findViewById(R.id.btnCancel); } @Override protected void initData() { } @Override protected void initListener() { btnCamera.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { dismiss(); if(onCameraOrAlbumSelectListener != null){ onCameraOrAlbumSelectListener.OnSelectCamera(); } } }); btnAlbum.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { dismiss(); if(onCameraOrAlbumSelectListener != null){ onCameraOrAlbumSelectListener.OnSelectAlbum(); } } }); btnCancel.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { dismiss(); } }); } }
Select monitor interface of camera or album
public interface OnCameraOrAlbumSelectListener { public void OnSelectCamera(); public void OnSelectAlbum(); }
6. Specific use
private AlbumOrCameraPopupWindow ACWindow; private void initListener(){ ACWindow = new AlbumOrCameraPopupWindow(this, onCameraOrAlbumSelectListener); btnIosPopupWindow.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if(ACWindow != null && !ACWindow.isShowing()){ ACWindow.showAtLocation(R.layout.activity_view_popup_window); } } }); } /**Choose a camera or album*/ private OnCameraOrAlbumSelectListener onCameraOrAlbumSelectListener = new OnCameraOrAlbumSelectListener() { @Override public void OnSelectCamera() { LogUtil.i("OnSelectCamera..."); ToastUtil.showShort(instance, "camera"); } @Override public void OnSelectAlbum() { LogUtil.i("OnSelectAlbum..."); ToastUtil.showShort(instance, "Choose from album"); } };
Further to CV Engineer~~~